Overcoming Common Pitfalls in Software Development
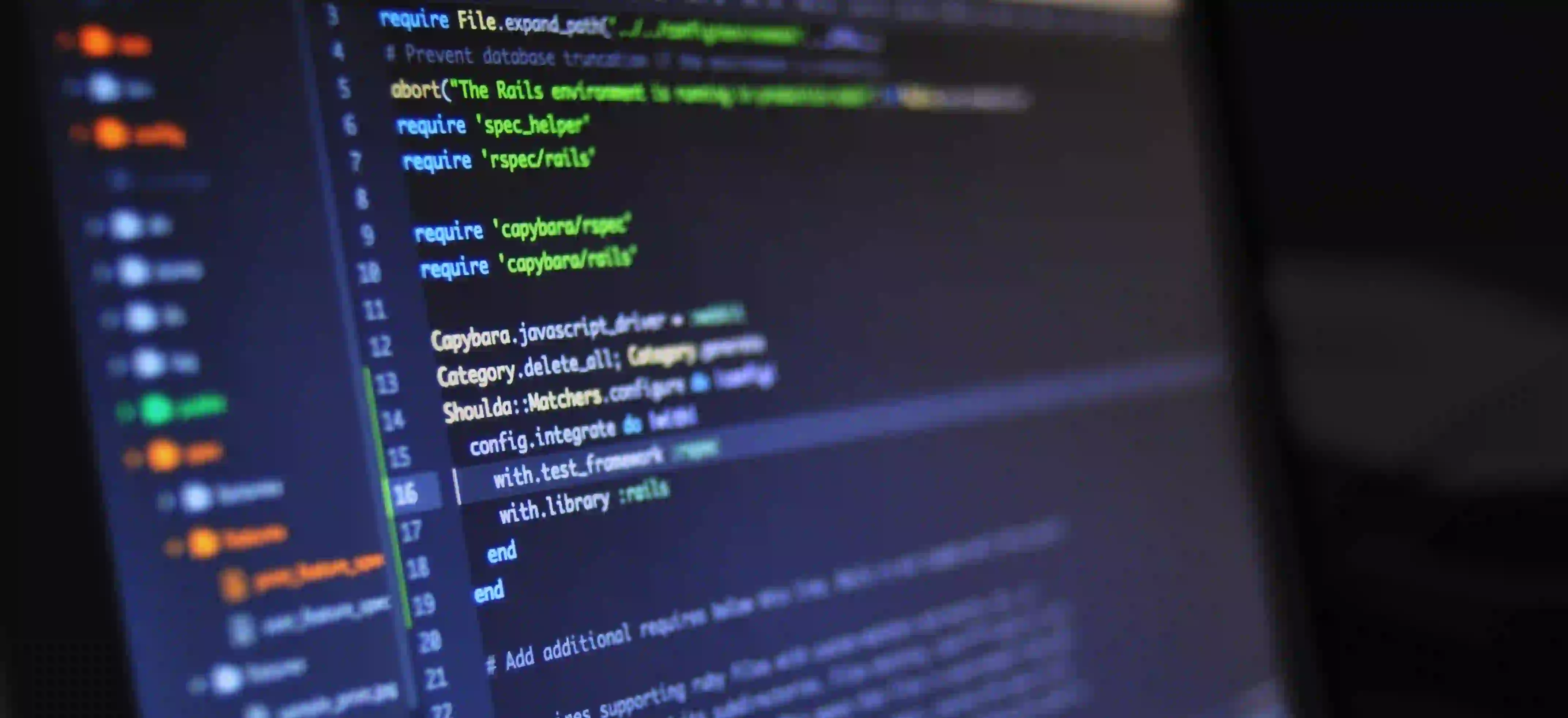
Overcoming Common Pitfalls in Software Development
Software development is both a science and an art. It requires meticulous attention to detail, an understanding of user needs, and constant adaptability in the face of new challenges. Despite having extensive frameworks, tools, and best practices at our disposal, developers frequently encounter certain pitfalls that can derail projects or hinder their progress. In this blog post, we'll discuss some common pitfalls in software development and provide actionable strategies to overcome them.
Understanding Common Pitfalls
-
Inadequate Planning
This is perhaps the most significant pitfall. It is all too easy to dive straight into coding, especially if you have a clear vision. However, without proper planning, projects can quickly spiral out of control.
- Solution: Adopt Agile methodologies. Agile promotes iterative development and constant feedback. Tools like Trello or Jira can help keep your projects organized and ensure everyone is on the same page.
☕snippet.java// Example of an initial planning phase public class ProjectPlanning { private List<String> goals; public ProjectPlanning() { this.goals = new ArrayList<>(); } public void addGoal(String goal) { this.goals.add(goal); } public List<String> getGoals() { return goals; } }
In this example, we create a simple
ProjectPlanning
class. It keeps track of your project goals—an essential first step before you start coding. -
Lack of Documentation
It is essential to maintain clear documentation throughout the project lifecycle. Many developers consider documentation a chore, leading to a lack of clarity and onboarding difficulties for new team members.
- Solution: Use inline comments and maintain a separate documentation space, utilizing tools such as Markdown or Javadoc.
☕snippet.java/** * Calculates the sum of two integers. * * @param a the first integer * @param b the second integer * @return the sum of a and b */ public int sum(int a, int b) { return a + b; }
Here, we've added Javadoc comments to provide clarity on the
sum
method. Keeping documentation close to the code can aid future developers. -
Ignoring Unit Tests
Not utilizing unit tests can lead to code that is difficult to maintain. Even seasoned developers often overlook testing due to time constraints. However, the long-term benefits are undeniable.
- Solution: Implement unit tests early in the development process using frameworks like JUnit.
☕snippet.javaimport static org.junit.jupiter.api.Assertions.assertEquals; import org.junit.jupiter.api.Test; public class MathUtilsTest { @Test public void testSum() { MathUtils utils = new MathUtils(); assertEquals(5, utils.sum(2, 3)); } }
This code snippet showcases a basic unit test for the
sum
method. By creating tests, you ensure that your code behaves as expected even as you refactor or add new features. -
Scope Creep
Scope creep occurs when an unplanned expansion of a project takes place. It adds unnecessary complexity and often leads to project delays.
- Solution: Define clear project boundaries and stick to them. Regularly review project requirements with stakeholders to manage expectations.
☕snippet.javapublic class ProjectScope { private String projectName; private List<String> features; public ProjectScope(String projectName) { this.projectName = projectName; this.features = new ArrayList<>(); } public void addFeature(String feature) { if (features.size() < 5) { // Example limit on features features.add(feature); } else { System.out.println("Feature limit reached for: " + projectName); } } }
In this example, we've implemented a feature limit to manage scope. By doing so, you can curb scope creep effectively.
-
Neglecting Code Reviews
Skipping code reviews can lead to unchecked errors, non-standard coding practices, and a lack of shared knowledge among team members.
- Solution: Establish a mandatory code review process. Utilize tools like GitHub for pull requests, allowing peers to review code efficiently.
☕snippet.java// Sample code that would undergo a code review process public void processPayment(double amount) { if (amount <= 0) { throw new IllegalArgumentException("Amount must be positive"); } // Assume payment processing logic here }
Before this code snippet is merged, a peer's review can ensure that it meets coding standards and is error-free.
-
Failure to Communicate
Poor communication can severely affect project timelines and outcomes. Teams may miss deadlines or fail to understand requirements due to inadequate info sharing.
- Solution: Foster a culture of open communication. Utilize platforms like Slack or Microsoft Teams to maintain constant dialogue.
☕snippet.java// Example of a simple messaging system for team communication public class TeamChat { private List<String> messages; public TeamChat() { this.messages = new ArrayList<>(); } public void sendMessage(String message) { messages.add(message); notifyMembers(message); } private void notifyMembers(String message) { System.out.println("New message: " + message); } }
This basic
TeamChat
class highlights the importance of keeping communication open. By notifying team members of new messages, you cultivate a communicative environment. -
Underestimating Maintenance
Maintenance can consume more time than initial development. Developers may build a feature and move on, neglecting the fact that it requires ongoing support.
- Solution: Write maintainable code. Stick to coding standards and conduct regular maintenance check-ups on your software to address issues.
☕snippet.javapublic void updateFeature() { // Feature update logic with a focus on maintainability }
Here, the dummy method
updateFeature
represents ongoing maintenance. Writing clear, maintainable code is key to reducing future workload. -
Choosing the Wrong Tools
The ever-evolving tech landscape means new tools are popping up frequently. Choosing the wrong tool can lead to inefficiencies and frustration.
- Solution: Evaluate your options carefully. Engage with the community and research the functionality of tools before integrating them into your stack.
-
Ignoring User Feedback
Sometimes developers fall into the trap of thinking they know what users want without actually soliciting user feedback. This can lead to the development of features that may not meet the users' needs.
- Solution: Utilize tools like SurveyMonkey or UserTesting to gather user feedback regularly.
-
Not Future-Proofing Code
Software projects need to adapt to changing requirements over time. If developers do not take future possibilities into account, they may need to perform significant overhauls down the line.
- Solution: Design with flexibility and scalability in mind. Use design patterns like the Strategy or Observer pattern to allow for easier modifications in the future.
Bringing It All Together
Overcoming these common pitfalls is essential for successful software development. The key lies in following best practices, prioritizing communication, and remaining open to feedback. Being mindful of these aspects will not only enhance the quality of your projects but will also create a more enjoyable development experience.
For more tips on best practices in Java programming and software development, consider reading Effective Java or Clean Code.
By implementing these strategies, you can navigate the complexities of software development more efficiently and effectively, paving the way to successful project outcomes.