Array vs ArrayList: Unpacking Performance Issues in Java
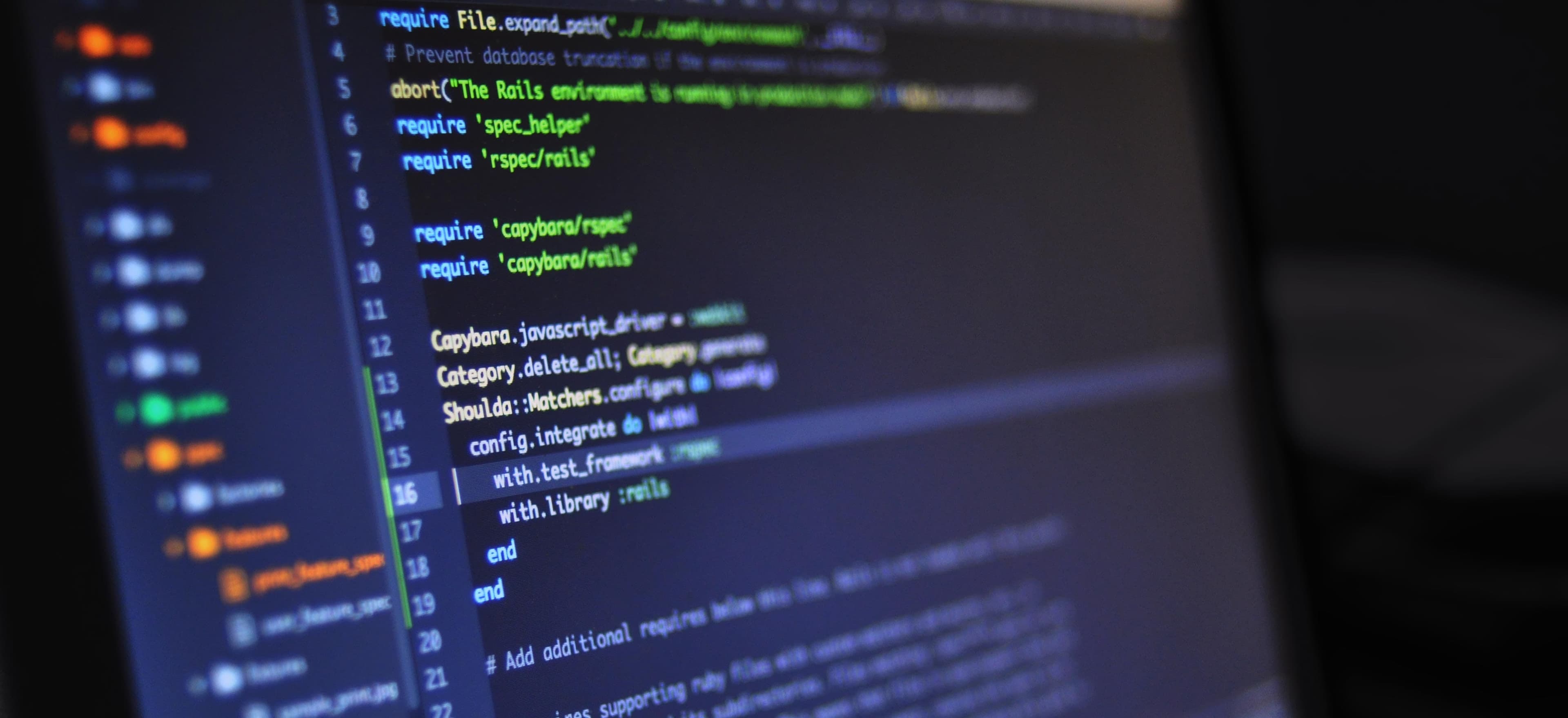
- Published on
Array vs ArrayList: Unpacking Performance Issues in Java
When it comes to data handling in Java, two primary structures often come to mind: Arrays and ArrayLists. Both serve the purpose of storing multiple values, yet their performance characteristics, flexibility, and use cases differ considerably. This blog post will delve into the key differences between Arrays and ArrayLists in Java, focusing on their performance implications, scenarios for use, and code examples to illustrate these concepts.
Understanding Arrays
An Array in Java is a data structure that holds a fixed number of elements of the same data type. Arrays provide a direct mechanism for storing and accessing data, making them memory-efficient and fast when it comes to indexing.
Key Characteristics of Arrays
-
Fixed Size: Once defined, the size of an array cannot be changed. For instance, if you define an array to hold five integers, it will always be capable of holding only those five integers.
-
Direct Access: Arrays allow for fast access to elements using an index, which is why they are often preferred in scenarios where the number of elements is known during compile-time.
-
Homogeneous Data Types: Arrays can only store elements of a defined data type.
Example of Using Arrays
Here’s a simple code snippet demonstrating how to declare and initialize an array in Java:
public class ArrayExample {
public static void main(String[] args) {
int[] numbers = new int[5]; // An integer array with fixed size 5
// Initializing the array
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i * 10; // Assigning values to the array
}
// Displaying the elements
for (int number : numbers) {
System.out.println(number);
}
}
}
In this example, we define an integer array numbers
of size 5 and populate it via a loop. The primary reason to use an array here is the predictable structure and access speed.
Understanding ArrayLists
On the other hand, an ArrayList is a part of Java's collection framework. It is a resizable array implementation of the List interface, which allows for dynamic-sized arrays that can grow as needed.
Key Characteristics of ArrayLists
-
Dynamic Resizing: Unlike arrays, ArrayLists can grow and shrink in size, adapting to the number of elements you add or remove.
-
Flexibility: ArrayLists can store elements of different types if you use the
Object
class, but generics allow you to maintain type safety. -
Performance: While access time is also O(1) for ArrayLists, adding or removing elements can be O(n) in the worst case, especially if it requires resizing the backing array.
Example of Using ArrayList
Here’s a simple code snippet showing how to utilize an ArrayList in Java:
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>(); // Declare an ArrayList
// Adding values to the ArrayList
for (int i = 0; i < 5; i++) {
numbers.add(i * 10); // Adding elements dynamically
}
// Displaying the elements
for (int number : numbers) {
System.out.println(number);
}
}
}
In this example, the ArrayList
numbers
is dynamic. You can add elements without worrying about the defined size, which presents a significant advantage when exact sizes are not known at compile-time.
Performance Comparison
When comparing performance between Arrays and ArrayLists, several factors come into play:
Access Time
Both data structures allow for constant time access (O(1)) to their elements. Whether you are using an index in an Array or the get()
method in an ArrayList, the underlying array provides a fast retrieval mechanism.
Memory Consumption
Arrays typically consume less memory due to the absence of additional features that ArrayLists possess. An ArrayList has a backing array, and when it exceeds its capacity, it has to create a new array and copy existing elements to it, which adds overhead.
Adding/Removing Elements
Adding an element to an Array is not feasible when you reach its capacity. You must create a new array to accommodate additional elements. In contrast, with an ArrayList, you can efficiently add elements (amortized O(1)), but you may incur a performance cost if the backing array needs resizing. Removing an element, particularly from the middle of an ArrayList, requires shifting elements, leading to a potential O(n) time complexity.
Use Cases
-
Use arrays when you have a fixed size and performance is a priority. This is common in data structures like heaps, stacks, and matrices.
-
Use ArrayLists when you need flexibility with the size and do not require frequent additions and deletions. Suitable for dynamic data collections where elements are often added or removed.
Summary and Conclusion
In conclusion, both Arrays and ArrayLists have their unique advantages and disadvantages in Java. Arrays are ideal for fixed-size data needs with efficient memory usage, while ArrayLists offer flexible sizing and easier data manipulation. The choice between them should depend on your specific requirements regarding performance, memory usage, and functionality.
For further reading on Java collections, you may check the Java Collections Framework Documentation and explore the differences in more detail.
When optimizing performance in your Java applications, always consider where your data is maintained and manipulated. Ultimately, understanding these structures will inform better design choices and lead to more efficient coding practices.
Happy coding!
Checkout our other articles