Combatting DukeScript's Compatibility Challenges in Projects
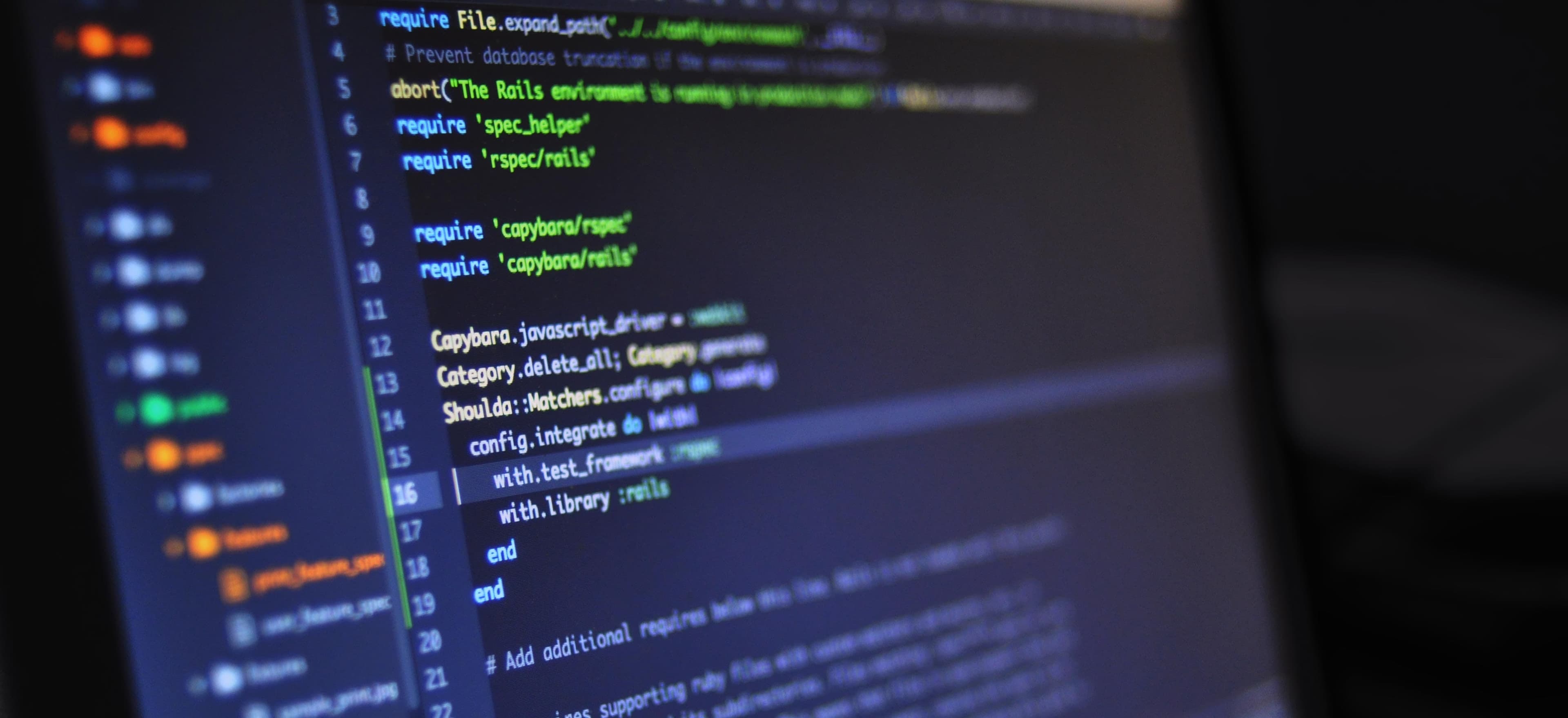
- Published on
Combatting DukeScript's Compatibility Challenges in Projects
DukeScript is a remarkable tool that allows developers to create cross-platform applications using Java. It leverages the power of JavaFX, enabling developers to write code once and deploy it across various platforms, including desktop and mobile. However, like any technology, DukeScript comes with its own set of compatibility challenges that developers must navigate. In this blog post, we'll explore these challenges and offer solutions to effectively mitigate them.
Understanding DukeScript Compatibility
DukeScript is built on top of the Java Virtual Machine (JVM) and connects to native mobile functionalities through a set of lightweight wrappers. While this is an attractive feature, it also leads to some compatibility issues due to differences in dependency management, rendering engines, and platform-specific features.
Key Compatibility Issues:
-
Platform Differences: Applications built with DukeScript may behave differently on Android, iOS, or desktop platforms even though they use the same code base.
-
Missing Features: Not all JavaFX features are supported in DukeScript, which can lead to missing functionalities or unexpected behaviors.
-
Dependency Management: Managing dependencies in a multi-platform environment can become complex, especially when implementing libraries that are not fully compatible with DukeScript.
-
Rendering Issues: Differences in rendering engines among platforms can cause inconsistencies in UI layouts.
Best Practices for Overcoming DukeScript Compatibility Challenges
To effectively combat these challenges, developers can adopt a series of best practices. Here’s a detailed look at strategies that will help improve compatibility in your DukeScript projects.
1. Use Common Libraries
Utilizing libraries that are known to work well with DukeScript is essential. Not all Java libraries integrate seamlessly with DukeScript, so choose wisely. Libraries like Gson for JSON handling and JFXtras for additional JavaFX components are designed to maintain compatibility with DukeScript.
import com.google.gson.Gson;
// Example of using Gson for JSON serialization in DukeScript
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public static void main(String[] args) {
User user = new User("John Doe", 30);
Gson gson = new Gson();
// Serialize User object into JSON format
String json = gson.toJson(user);
System.out.println(json); // Outputs: {"name":"John Doe","age":30}
}
}
Why? Using compatible libraries ensures foundational functionalities aren’t compromised across different platforms.
2. Adhere to Platform Guidelines
Each platform has its own set of design and functionality guidelines. For instance, mobile devices might prioritize touch events, while desktops utilize keyboard and mouse interactions. When creating DukeScript applications, ensure you are aware of these guidelines:
import javafx.scene.input.MouseEvent;
import javafx.scene.input.TouchEvent;
// Handling different input on various platforms
public void handleInput(MouseEvent mouseEvent, TouchEvent touchEvent) {
if (mouseEvent != null) {
// Handle mouse events for desktop
System.out.println("Mouse Clicked at: " +
mouseEvent.getX() + ", " +
mouseEvent.getY());
} else if (touchEvent != null) {
// Handle touch events for mobile
System.out.println("Screen touched at: " +
touchEvent.getTouchPoint().getX() + ", " +
touchEvent.getTouchPoint().getY());
}
}
Why? Adhering to platform-specific guidelines helps improve user experience and reduces compatibility issues.
3. Modularize Your Code
Creating modular code can enhance compatibility across different platforms. Rather than hard-coding platform-specific logic, use interfaces and abstract classes that define behaviors. This not only organizes your code but also makes it easier to manage.
public interface PlatformHandler {
void initialize();
void render();
}
public class MobileHandler implements PlatformHandler {
public void initialize() {
System.out.println("Mobile App Initialized");
}
public void render() {
System.out.println("Rendering mobile UI");
}
}
public class DesktopHandler implements PlatformHandler {
public void initialize() {
System.out.println("Desktop App Initialized");
}
public void render() {
System.out.println("Rendering desktop UI");
}
}
// Usage
public class Application {
private PlatformHandler platformHandler;
public Application(PlatformHandler handler) {
this.platformHandler = handler;
platformHandler.initialize();
}
public void start() {
platformHandler.render();
}
}
Why? Using a modular approach allows easy updates and enhancements, enhancing compatibility across platforms.
4. Use Conditional Compilation
In situations where certain features are only applicable to specific platforms, use conditional compilation to streamline the process. This involves setting constants in build configurations to segregate platform-specific code.
#ifdef ANDROID
public void platformSpecificFeature() {
System.out.println("This feature is Android specific.");
}
#else
public void platformSpecificFeature() {
System.out.println("This feature is not available on this platform.");
}
#endif
Why? Conditional compilation allows code specific to a platform to be included or excluded, simplifying maintenance.
Testing is Crucial
As you implement these strategies, rigorous testing becomes crucial. You should set up continuous integration (CI) tools that can run automated tests across different platforms. This will help identify compatibility issues early in the development process.
5. Cross-Platform Testing Tools
Explore tools designed for DukeScript apps, such as the JUnit framework, which allows you to write repeatable tests. Ensure tests cover various scenarios, including input handling, UI rendering, and component interactions.
Example of a simple JUnit test:
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
class UserTest {
@Test
void testUserJsonSerialization() {
User user = new User("John Doe", 30);
Gson gson = new Gson();
String json = gson.toJson(user);
Assertions.assertEquals("{\"name\":\"John Doe\",\"age\":30}", json);
}
}
Why? Automated testing ensures application reliability across various platforms while saving time in the long run.
The Last Word
While compatibility challenges in DukeScript projects can be discouraging, adopting a structured approach to building your applications will greatly mitigate these issues. By choosing common libraries, adhering to platform guidelines, modularizing your code, implementing conditional compilation, and rigorously testing your applications, you significantly enhance the compatibility of your DukeScript projects.
DukeScript can truly be an asset in your development toolkit, allowing you to harness the power of Java while working across diverse platforms. Equip yourself with the right strategies, and you will be well on your way to mastering DukeScript compatibility challenges.
For more insights about Java development and cross-platform technologies, check out Baeldung's Java Tutorial and Oracle's Java Documentation.
Checkout our other articles