Boost Your App's Security: Mastering Logging Techniques
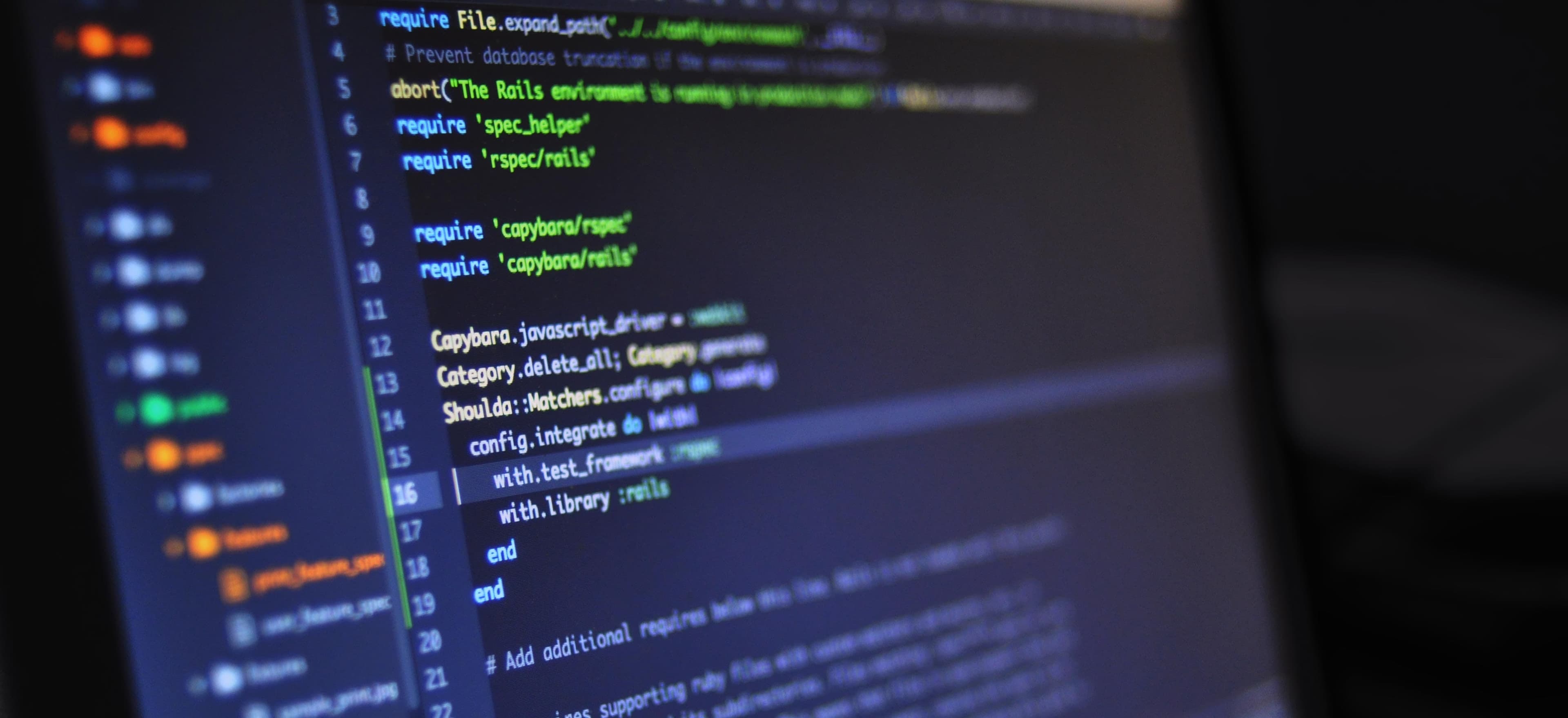
- Published on
Boost Your App's Security: Mastering Logging Techniques
In the world of application development, security is often at the forefront of considerations. As developers, our responsibility extends beyond merely writing functional code; we must also ensure that our applications are resilient against various vulnerabilities. A key component of building secure applications is effective logging. In this post, we’ll explore how mastering logging techniques can significantly enhance your app's security, especially when using Java.
Understanding Logging
Before delving into specifics, let's clarify what logging means in the context of software development. Logging refers to the systematic recording of events that occur when an application runs. These records, or log entries, can provide critical insights into the behavior of applications and potential security threats.
Why Is Logging Important?
- Monitoring and Analysis: Logs enable developers and security teams to monitor application behavior in real time.
- Incident Response: In the event of a security incident, logs play a crucial role in investigating and rectifying the issue.
- Compliance: Many regulatory frameworks require organizations to maintain detailed logging for security audits and accountability.
Java Logging Frameworks
Java offers several logging frameworks to facilitate logging, including:
- Java Util Logging (JUL): The built-in logging framework provided by the Java Development Kit (JDK).
- Log4j: A popular logging library that is highly configurable and performs well in various environments.
- SLF4J (Simple Logging Facade for Java): An abstraction layer that allows the use of various logging frameworks while providing a simple interface.
In this post, we will primarily focus on Log4j, given its flexibility and widespread adoption.
Getting Started with Log4j
To get started, first, add the Log4j dependency to your Maven pom.xml
file:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.17.0</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.17.0</version>
</dependency>
This ensures that you have the required libraries for Log4j to function properly.
Basic Configuration
Log4j allows you to configure logging through XML, JSON, or YAML based configuration files. Here’s a simple example of using a log4j2.xml
configuration file:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n"/>
</Console>
<File name="FileLogger" fileName="app.log" append="false">
<PatternLayout pattern="%d{ISO8601} [%t] %-5p %c %x - %m%n"/>
</File>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
<AppenderRef ref="FileLogger"/>
</Root>
</Loggers>
</Configuration>
Commentary
- Appenders: Different outputs for your logs (console, file, etc.) ensure visibility.
- PatternLayout: Here, we structure how the log message is displayed, providing context for each log entry.
- Loggers: The root logger determines the default logging level (
info
in this case), controlling the amount of information recorded.
For detailed information on configuring Log4j, visit Apache Log4j Documentation.
Essential Logging Practices for Security
Incorporating logging into your Java application is the first step. However, to fully leverage its benefits in enhancing security, adhere to the following practices:
1. Log Important Events
Always log significant events such as user logins, access to sensitive data, and administrative actions. Here’s a code snippet demonstrating how to log user login attempts:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class UserService {
private static final Logger logger = LogManager.getLogger(UserService.class);
public void login(String username) {
logger.info("User {} attempted to log in.", username);
// Logic for user login
logger.info("User {} logged in successfully.", username);
}
}
Commentary
This snippet captures both successful and failed login attempts. Such logs are crucial in detecting potential brute-force attacks.
2. Avoid Logging Sensitive Data
While it's important to log events, be mindful of sensitive information such as passwords, credit card details, and personal identification information.
public void createUser(String username, String password) {
logger.info("Creating user {}", username);
// Avoid logging password
// Logic to save user
}
Commentary
By avoiding logging sensitive information, you minimize the risk of exposing user data in the event of a log breach.
3. Implement Log Rotation and Retention Policies
Logs can grow quickly, consuming valuable disk space. Implement sensor rotation to archive old logs and set expiration to manage your storage effectively.
<File name="RollingFile" fileName="app.log" filePattern="app-%d{yyyy-MM-dd}-%i.log" append="false">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n"/>
<Policies>
<TimeBasedTriggeringPolicy interval="1" modulate="true"/>
<SizeBasedTriggeringPolicy size="10MB"/>
</Policies>
</File>
Commentary
The above configuration rotates logs daily and also when the size exceeds 10MB, ensuring you have consistent log hygiene.
4. Monitor Log Files
Integration with monitoring tools like ELK Stack (Elasticsearch, Logstash, and Kibana) can enhance your ability to analyze logs. This provides insights into patterns and potential breaches effectively.
Beyond the Basics - Log Anomaly Detection
As applications become more sophisticated, so do attacks. Consider employing machine learning algorithms to analyze logs for unusual patterns. This approach can help detect breaches more effectively than traditional methods.
For insights on implementing log anomaly detection, check out this detailed guide.
Closing the Chapter
Mastering logging techniques is essential for enhancing your app’s security. By implementing thoughtful logging practices, you not only create a trail that can help during investigations but also build a robust defense mechanism against vulnerabilities. Remember to choose the right logging framework, log significant events, protect sensitive data, manage log storage, and consider innovative methods like anomaly detection.
Taking these steps will not only improve your application’s security posture but also foster trust with users who rely on your software.
Happy coding, and may your logs guide you towards a secure application!
Feel free to leave comments or questions below. Share your own experiences with logging in your Java applications!
Checkout our other articles