Mastering GraphQL Mutations: Handling No Data Returns
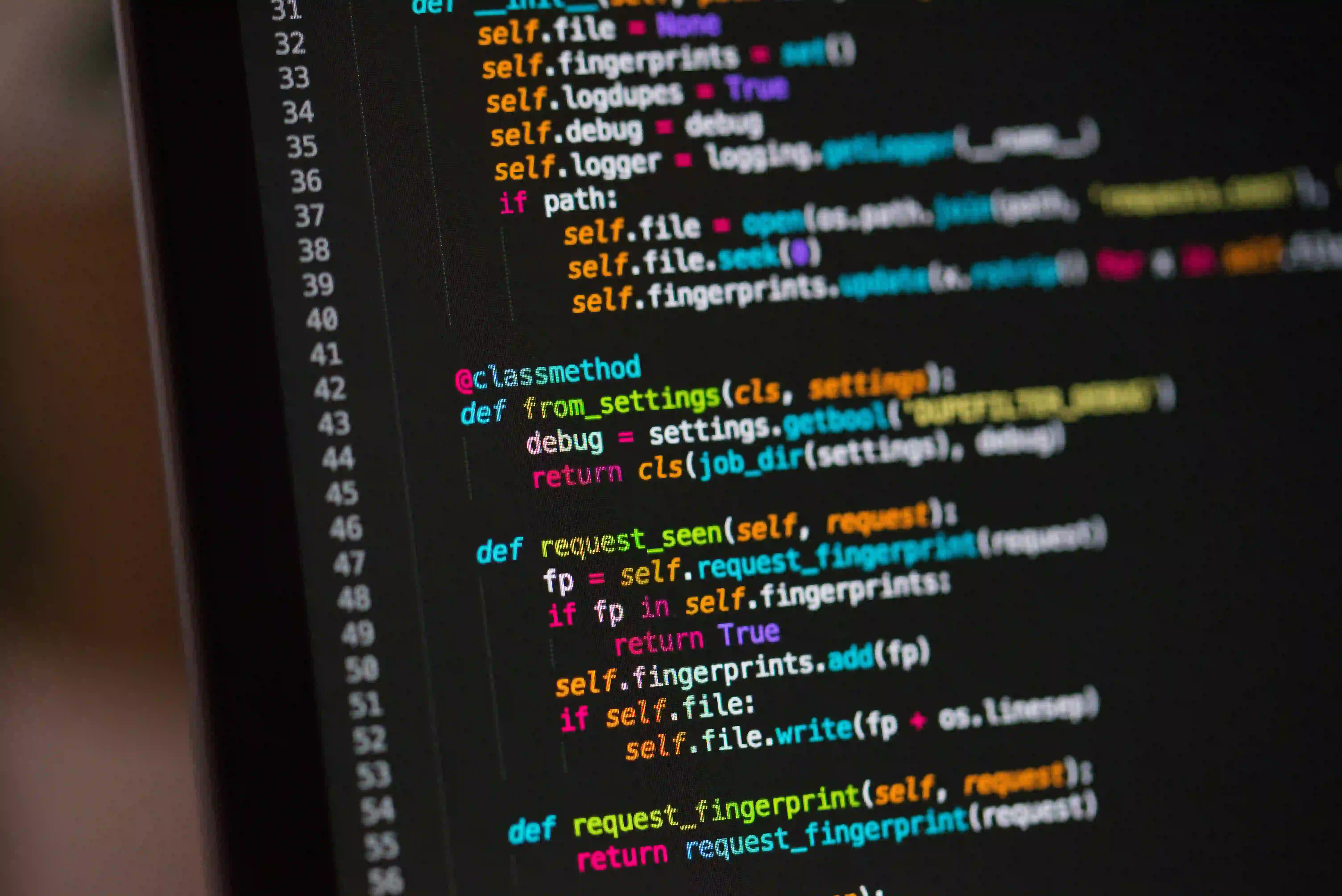
Mastering GraphQL Mutations: Handling No Data Returns
GraphQL has rapidly gained popularity among developers for its flexibility and efficiency in how we communicate between client and server. While queries are often the star of the show, mutations deserve just as much attention, especially when considering scenarios when no data is returned. In this post, we'll delve deep into GraphQL mutations, focusing on how to manage cases where your mutation might not yield any data.
What Are GraphQL Mutations?
Before we dig deeper, let's clarify what a mutation is. In GraphQL terminology, a mutation is a way to modify server-side data. Unlike a query, which is read-only, mutations are designed for creating, updating, or deleting data in your API.
Basic Structure of a Mutation
Here's a simple example of how a mutation might look in GraphQL:
mutation {
createUser(name: "John Doe", email: "johndoe@example.com") {
id
name
}
}
In this example, we are creating a user and asking for the user’s ID and name to be returned. This is typical behavior for many mutations. However, what happens when our mutation doesn't return data, or if we specifically want to handle that?
Understanding No Data Returns
Mutations can sometimes result in a "No Data" scenario. Here are a few common situations when this might occur:
- Deletion Operations: When you delete an item, it often makes sense not to return any data.
- Success Acknowledgements: For mutations that perform actions like logging or notifications, returning data may not be necessary.
- Error Handling: In some cases, errors can occur which prevent achieving the desired outcome.
Example: Deleting a User Without Returning Data
Let's illustrate a mutation that deletes a user and does not return any data:
mutation {
deleteUser(id: "123") {
success
message
}
}
In this case, you might design your server to respond with confirmation information instead of the deleted user itself:
{
"data": {
"deleteUser": {
"success": true,
"message": "User deleted successfully"
}
}
}
This approach allows the client to know that the operation was successful or to handle potential problems accordingly.
Crafting Mutations without Data Returns
When designing your GraphQL schema, mutations that do not return data can be crafted in a way that enhances usability and clarity. Consider the following guidelines:
- Use Explicit Types: Even if no data is returned, define a return type that indicates the operation's success.
- Provide Feedback: Consider returning a status message, especially in case of errors.
- Model Responses: Structure your responses clearly, so clients can effortlessly interpret them.
Example: GraphQL Schema for Mutations
Here’s an example of a GraphQL schema using SDL (Schema Definition Language) that employs a mutation returning only a message:
type Mutation {
deleteUser(id: ID!): DeleteResponse!
}
type DeleteResponse {
success: Boolean!
message: String!
}
Implementing the Mutation
Once you've set up your GraphQL schema, you can implement the resolver function. Below is an example using JavaScript with Apollo Server, showcasing how to handle the mutation logic while returning user-friendly messages, without any actual user data being returned.
Resolver Example
const resolvers = {
Mutation: {
deleteUser: async (_, { id }, { dataSources }) => {
const userDeleted = await dataSources.userAPI.deleteUserById(id);
if (userDeleted) {
return {
success: true,
message: "User deleted successfully"
};
} else {
return {
success: false,
message: "User not found"
};
}
}
}
};
Commentary
- Here, the resolver calls a data source method to delete a user by ID.
- Depending on whether deletion was successful, it returns an appropriate success flag and message.
- This provides clarity and usability from the client-side perspective.
Handling Errors Gracefully
Implementing error handling within your mutation is crucial. As a best practice, always account for various scenarios that might cause your mutation to fail.
Example of Error Handling
You can enhance the previous resolver to include more extensive error handling:
const resolvers = {
Mutation: {
deleteUser: async (_, { id }, { dataSources }) => {
try {
const userDeleted = await dataSources.userAPI.deleteUserById(id);
if (userDeleted) {
return {
success: true,
message: "User deleted successfully"
};
} else {
return {
success: false,
message: "User not found"
};
}
} catch (error) {
console.error("Error deleting user:", error);
return {
success: false,
message: "An error occurred while deleting the user"
};
}
}
}
};
Key Points
- Try/Catch: Wrapping your mutation logic in a try/catch block allows you to catch any unexpected errors during execution.
- Logging: Always log errors for server-side investigations without exposing sensitive information to the client.
Client-Side Implementation
On the client side, you can utilize libraries such as Apollo Client to manage the execution of these mutations. Using the example mutation deleteUser
, here's how you might execute it in your application:
import { useMutation } from '@apollo/client';
import gql from 'graphql-tag';
const DELETE_USER = gql`
mutation DeleteUser($id: ID!) {
deleteUser(id: $id) {
success
message
}
}
`;
function DeleteUserComponent({ userId }) {
const [deleteUser, { data, loading, error }] = useMutation(DELETE_USER);
const handleDelete = () => {
deleteUser({ variables: { id: userId } });
};
return (
<div>
<button onClick={handleDelete}>Delete User</button>
{loading && <p>Deleting...</p>}
{data && <p>{data.deleteUser.message}</p>}
{error && <p>An error occurred: {error.message}</p>}
</div>
);
}
My Closing Thoughts on the Matter
Mastering GraphQL mutations involves understanding not just how to send and receive data, but also how to structure responses effectively—even when no data is present. By focusing on user experience, thoughtful error handling, and providing meaningful success messages, we can build robust APIs that gracefully manage data modification tasks.
For more in-depth information on GraphQL, consider visiting the official GraphQL documentation. Whether you're new to GraphQL or looking to sharpen your skills, there's always more to learn and develop.
Happy coding!