Mastering Java Scheduling: Common Pitfalls to Avoid
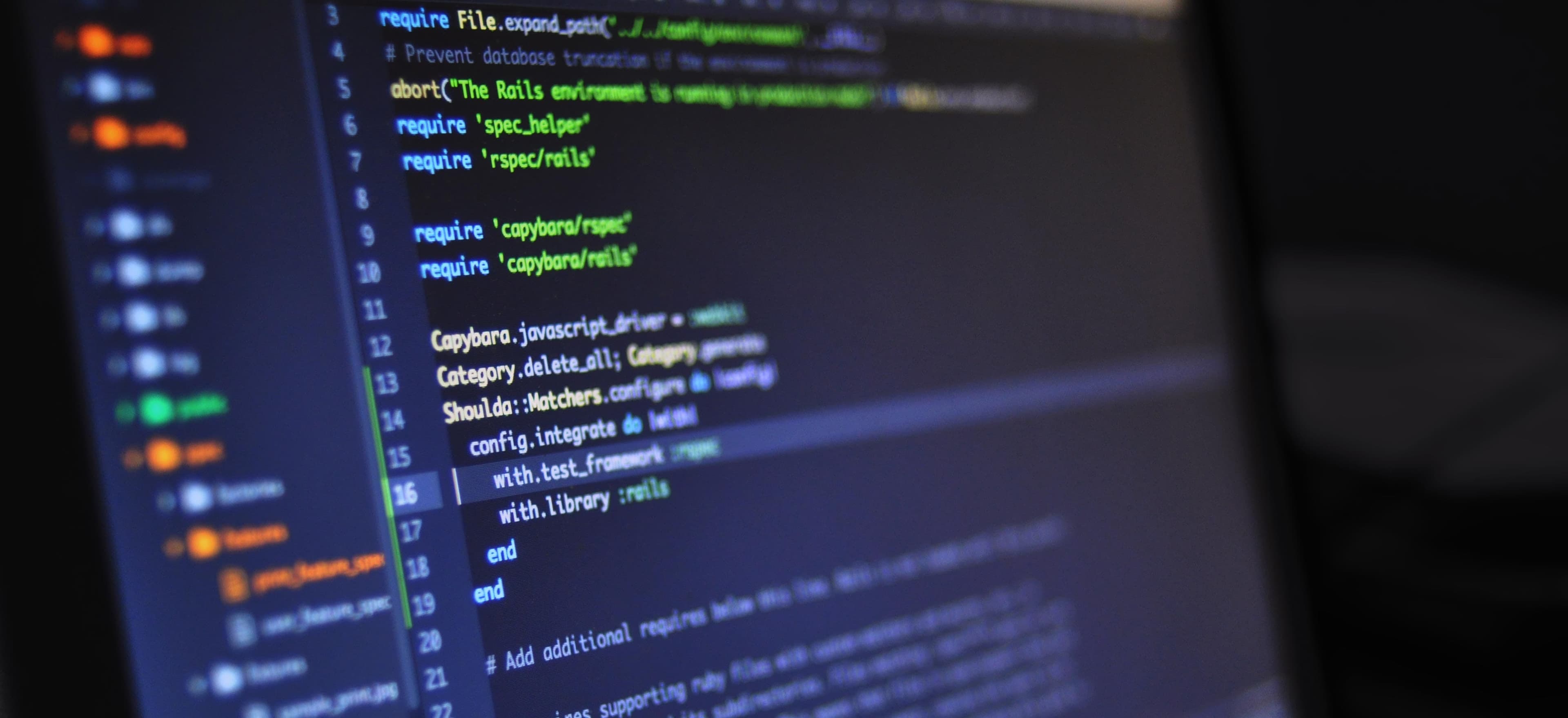
- Published on
Mastering Java Scheduling: Common Pitfalls to Avoid
Java is a versatile programming language that offers robust capabilities for scheduling tasks. From simple applications needing delayed execution of a function to complex systems requiring intricate workflows, Java provides several tools to manage scheduling. However, as with any technology, pitfalls exist that can lead to inefficiencies, errors, or system failures. In this blog post, we will explore common pitfalls in Java scheduling, supported by code examples and practical insights.
Understanding Task Scheduling in Java
Task scheduling in Java generally revolves around the following APIs:
java.util.Timer
andjava.util.TimerTask
: A simple way to schedule a task for future execution in a background thread.java.util.concurrent.ScheduledExecutorService
: A more advanced solution that supports multiple concurrent scheduled tasks.- Quartz Scheduler: A robust job scheduling library that offers powerful scheduling capabilities.
Each of these has its specific use cases and potential pitfalls that developers should be conscious of.
Common Pitfall 1: Overusing Timer
and TimerTask
One of the most common pitfalls when working with Java scheduling is overusing Timer
and TimerTask
for complex scheduling needs. While simple and easy to implement, Timer
has issues managing concurrent tasks.
Why It Matters:
When a task scheduled by a Timer throws an unchecked exception, the Timer thread becomes inactive leading to halted execution of subsequent tasks.
Example:
import java.util.Timer;
import java.util.TimerTask;
public class TimerExample {
public static void main(String[] args) {
Timer timer = new Timer();
timer.schedule(new TimerTask() {
public void run() {
System.out.println("Running task...");
throw new RuntimeException("Simulated error");
}
}, 0);
}
}
In this example, the subsequent executions of the task scheduled with timer
will not occur because the first task has thrown an exception, stopping the entire Timer thread.
Solution:
Consider using ScheduledExecutorService
instead, as it can handle exceptions without stopping subsequent tasks.
Common Pitfall 2: Ignoring Thread Pool Management
Another common mistake is the improper management of thread pools when using ScheduledExecutorService
. A poorly managed executor can lead to resource exhaustion, particularly if too many threads are created without limits.
Why It Matters:
Creating excessive threads can exhaust system resources, causing performance degradation or application crashes.
Example:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ScheduledExecutorExample {
public static void main(String[] args) {
ScheduledExecutorService executor = Executors.newScheduledThreadPool(10);
executor.scheduleAtFixedRate(() -> {
System.out.println("Task executed");
}, 0, 1, TimeUnit.SECONDS);
// Proper shutdown is crucial.
// executor.shutdown();
}
}
Solution:
Choose an appropriate number for your thread pool based on the expected workload. Additionally, always make sure to shut down the executor service using executor.shutdown()
to release resources properly.
Common Pitfall 3: Misunderstanding Time Boundaries
Many developers miscalculate the time intervals for scheduled tasks. Considering time zones or relying on incorrect calculations can lead to missed deadlines or unexpected behavior.
Why It Matters:
A task scheduled to execute at the wrong time can disrupt workflows, leading to larger issues in applications that rely on accurate timings, such as cron jobs.
Example:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class TimeBoundaryExample {
public static void main(String[] args) {
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
executor.schedule(() -> System.out.println("Time-sensitive task executed"),
25, TimeUnit.SECONDS);
}
}
Solution:
Always account for time zones and daylight saving time changes when scheduling tasks. The java.time
package introduced in Java 8 makes it easier to work with dates and times correctly (Learn more about java.time
).
Common Pitfall 4: Forgetting to Handle Task Completion
When tasks finish their execution, it's crucial to handle the outcome appropriately. Neglecting to check the result of scheduled tasks can lead to ignored errors or unwanted results.
Why It Matters:
Failing to process results from these tasks may result in unexpected behaviors down the line.
Example:
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class TaskCompletionExample {
public static void main(String[] args) {
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
Future<?> future = executor.schedule(() -> {
System.out.println("Task executed");
// Throwing exception for demonstration
throw new RuntimeException("Task error");
}, 1, TimeUnit.SECONDS);
try {
// This will not get to the completion - it'll throw an exception.
future.get();
} catch (Exception e) {
System.err.println("Caught exception: " + e.getMessage());
}
}
}
Solution:
Always utilize the Future.get()
method adequately to catch exceptions thrown by the tasks. This practice ensures that you remain aware of errors and can act on them as necessary.
Common Pitfall 5: Underestimating Performance Implications
Lastly, developers often underestimate the performance implications of scheduling tasks, especially in high-throughput applications. For example, creating a large number of scheduled tasks can impact overall application performance.
Why It Matters:
Performance bottlenecks can severely impact user experience and application stability, making it critical to understand expected workloads.
Example:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class PerformanceExample {
public static void main(String[] args) {
ScheduledExecutorService executor = Executors.newScheduledThreadPool(10);
for (int i = 0; i < 1000; i++) {
executor.schedule(() -> {
// Simulating a demanding task
System.out.println("Executing task...");
}, 1, TimeUnit.MILLISECONDS);
}
executor.shutdown();
}
}
Solution:
Evaluate and monitor task loads. Implement back-off strategies or throttling mechanisms where necessary, ensuring that scheduled tasks remain efficient without overwhelming the application.
Wrapping Up
Mastering Java scheduling involves understanding the tools available, their appropriate use cases, and the pitfalls to avoid. From proper thread pool management to effectively handling task completion, being conscientious will pay dividends in performance and reliability.
For a deeper dive into Java scheduling, consider exploring the official Java concurrency documentation or integrating a third-party library like Quartz Scheduler for more advanced use cases.
Whether you're building simple applications or complex systems, avoiding these common pitfalls will empower you to leverage Java's scheduling capabilities effectively. Happy coding!