Understanding Java Finalizers: Why They're Problematic
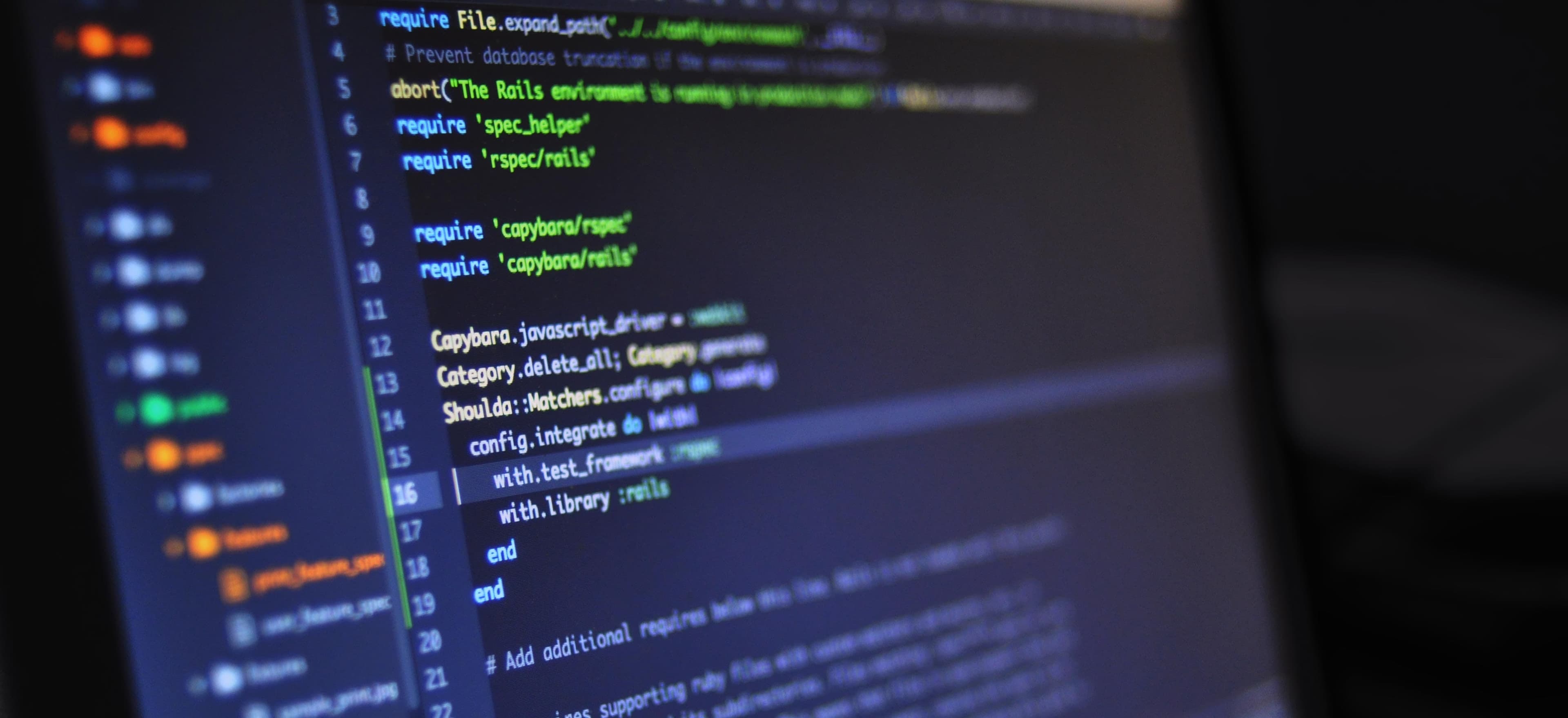
- Published on
Understanding Java Finalizers: Why They're Problematic
Java is a powerful programming language known for its memory management capabilities, thanks in large part to its automatic garbage collection. However, one feature of Java that has raised some eyebrows over the years is the use of finalizers. This blog post will explore Java finalizers, dissect the reasons they can be problematic, and discuss best practices for resource management in Java applications.
What are Java Finalizers?
In Java, a finalizer is a mechanism provided by the Object
class that allows developers to define a cleanup method, known as finalize()
, which the garbage collector calls before reclaiming the object's memory.
Syntax of Finalizers
Here's a simple example illustrating how a finalizer is defined:
public class Resource {
// Resource constructor
public Resource() {
System.out.println("Resource acquired.");
}
// Finalizer
@Override
protected void finalize() throws Throwable {
try {
System.out.println("Resource released.");
// Release resources (like closing files or network connections)
} finally {
super.finalize();
}
}
}
public class Main {
public static void main(String[] args) {
Resource resource = new Resource();
resource = null; // Lose reference to the resource
System.gc(); // Suggest garbage collection
}
}
Key Takeaways
- Automatic Cleanup: The intention behind finalizers is to automatically handle the cleanup when an object is no longer reachable.
- Invocation Timing: There is no guarantee when the
finalize()
method will be executed, leading to uncertainty in resource management.
The Problems with Finalizers
While the concept of finalizers may seem helpful, they can introduce a series of problems:
1. Unpredictable Timing
Finalizers do not run immediately after an object becomes unreachable. The garbage collector runs at its discretion, meaning resources might remain allocated for longer than expected. In performance-critical applications, this can lead to issues.
2. Performance Overheads
The presence of a finalizer adds overhead. When an object with a finalizer is no longer reachable, it requires extra steps during garbage collection, which can slow down performance. Additionally, the object must wait for two rounds of garbage collection—first to mark it for finalization, and second for actual collection.
3. Potential Memory Leaks
Finalizers can inadvertently lead to memory leaks. If a finalizer holds a reference to another object, it can prevent that object from being reclaimed. For example:
public class LeakyResource {
private LeakyResource badReference;
// Finalizer
@Override
protected void finalize() throws Throwable {
try {
// Keeping a reference to another object
// may cause memory leaks
badReference = new LeakyResource();
} finally {
super.finalize();
}
}
}
4. Exception Handling Challenges
If an exception is thrown in a finalizer, it is ignored (though it's logged). This can lead to silent failures where critical cleanup actions are not performed, further complicating resource management.
5. Lack of Control
Developers lose control over the cleanup process. This can lead to unforeseen issues, especially when using objects that rely on external resources.
6. Alternative Solutions Exist
Java provides several alternative approaches to handle resource management, which are generally more effective. These include try-with-resources statements and explicit resource management through proper design patterns.
Recommended Practices for Resource Management
Given the pitfalls of finalizers, here are a few recommended practices for managing resources effectively in Java:
Utilize Try-with-Resources
The try-with-resources statement ensures that resources are cleaned up promptly after use. This feature is particularly useful for working with I/O operations.
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
// Resources will be automatically closed here
Explicit Clean Up
For classes that manage resources, implement a method to release them explicitly.
public class DatabaseConnection {
private Connection connection;
public DatabaseConnection() {
// Establish connection
}
public void close() {
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
Weak References
If you are using external resources that might need to be released, consider using weak references. This allows the garbage collector to reclaim memory while also providing a cleanup opportunity when necessary.
Avoid Finalizers
As a general rule, avoid finalizers. For a detailed discussion about why finalizers are discouraged, consider reading this Java Best Practices article.
Lessons Learned
Java finalizers, once considered a novel feature for automatic cleanup, have proven to be problematic for various reasons. From their unpredictability and performance impacts to the potential for memory leaks, the drawbacks often outweigh the benefits.
Instead, developers should embrace modern alternatives, such as try-with-resources and explicit resource management techniques. By doing so, they can ensure their applications manage resources effectively and maintain optimal performance.
For further reading and resources, consider these links:
By focusing on best practices, Java developers can create robust applications that are easier to maintain and free from the complications traditionally associated with finalizers.