Mastering String Permutations: Common Pitfalls in Java
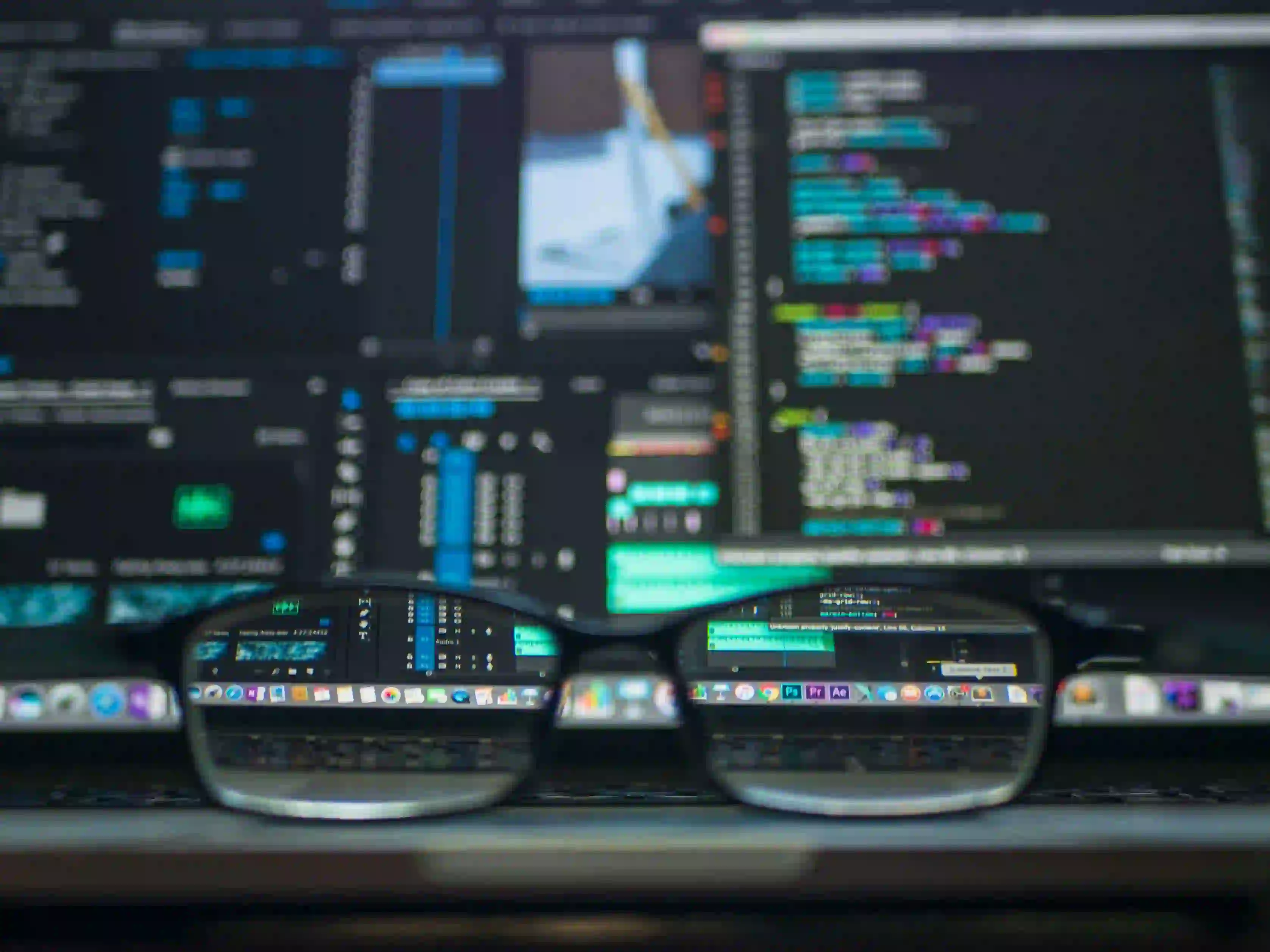
Mastering String Permutations: Common Pitfalls in Java
When dealing with string permutations in Java, developers frequently encounter various challenges. String permutations can be useful in a multitude of applications, such as generating anagrams, solving puzzles, and arranging data efficiently. However, numerous pitfalls can deter developers from achieving optimal performance and clean, understandable code. In this blog post, we will explore string permutations, dissect the common challenges that arise, and illustrate how to navigate through them with practical Java code snippets and clear commentary.
Understanding Permutations
Before diving into the code, let's clarify what a permutation is. A permutation of a string is a rearrangement of its characters. For example, the permutations of the string "ABC" include:
- ABC
- ACB
- BAC
- BCA
- CAB
- CBA
The number of permutations of a string of n characters is given by n!. However, when the string contains duplicate characters, the number of unique permutations is n! divided by the factorial of the frequency of each duplicate character.
The Approach
We will use a recursive approach to generate permutations. The recursion will systematically build permutations by swapping characters and tracking which characters have been used.
Basic Implementation
Here’s a basic implementation for generating all permutations of a string in Java:
import java.util.HashSet;
import java.util.Set;
public class Permutation {
public static void permute(String str) {
permuteHelper("", str);
}
private static void permuteHelper(String prefix, String remaining) {
int n = remaining.length();
if (n == 0) {
System.out.println(prefix);
} else {
for (int i = 0; i < n; i++) {
// Recursively call the function with the prefix and the remaining substring
permuteHelper(prefix + remaining.charAt(i), remaining.substring(0, i) + remaining.substring(i + 1, n));
}
}
}
public static void main(String[] args) {
String test = "ABC";
permute(test);
}
}
Commentary on the Code
-
Recursion Base Case: The
permuteHelper
function checks if theremaining
string is empty, indicating that a full permutation has been formed. If true, it prints the permutation. -
Recursive Case: The loop iterates through every character in the
remaining
string. The function concatenates the current character to theprefix
and calls itself with a newremaining
string minus the chosen character. This effectively builds the permutation one character at a time.
Common Pitfalls
1. Handling Duplicates
One of the common pitfalls arises when the input string contains duplicate characters. The previous method generates duplicate permutations, which can be undesirable. To avoid this, we can utilize a Set
to track already used characters.
Here is an improved version that eliminates duplicates:
import java.util.HashSet;
import java.util.Set;
public class UniquePermutation {
public static void permuteUnique(String str) {
Set<String> results = new HashSet<>();
permuteHelper("", str, results);
for (String permutation : results) {
System.out.println(permutation);
}
}
private static void permuteHelper(String prefix, String remaining, Set<String> results) {
int n = remaining.length();
if (n == 0) {
results.add(prefix);
} else {
for (int i = 0; i < n; i++) {
permuteHelper(prefix + remaining.charAt(i), remaining.substring(0, i) + remaining.substring(i + 1, n), results);
}
}
}
public static void main(String[] args) {
String test = "AAB";
permuteUnique(test);
}
}
Why We Use a Set
Using a Set
ensures that only unique permutations are collected. When a completed permutation is found, it is added to the results set. The benefit of this approach is that it automatically filters out duplicates.
2. Efficiency Concerns
The naive recursive approach may not be efficient for longer strings due to its exponential time complexity. Optimizing the function can save significant runtime.
The optimization can be achieved using iteration:
import java.util.ArrayList;
import java.util.List;
public class IterativePermutation {
public static List<String> permute(String str) {
List<String> result = new ArrayList<>();
result.add(""); // Start with an empty prefix
for (char c : str.toCharArray()) {
List<String> newPerm = new ArrayList<>();
for (String s : result) {
for (int i = 0; i <= s.length(); i++) {
newPerm.add(s.substring(0, i) + c + s.substring(i));
}
}
result = newPerm; // Update the result with new permutations
}
return result;
}
public static void main(String[] args) {
List<String> permutations = permute("ABC");
for (String p : permutations) {
System.out.println(p);
}
}
}
Explanation of the Iterative Method
-
Initialization: Start with a list containing an empty string, as we need to build up from there.
-
Outer Loop: Iterate over each character in the original string.
-
Inner Loop: For each current permutation, insert the new character in every possible position.
This method is more efficient in terms of space and time complexity compared to the recursive version.
3. Performance Tuning
For significantly long strings, memoization could enhance performance by caching results of previous computations.
To read more about performance optimizations in algorithms, refer to Java Performance Tuning Best Practices.
The Last Word
String permutations are a fundamental concept in programming and have practical applications across various domains. By understanding the common pitfalls, such as handling duplicates and optimization, developers can write cleaner, more efficient Java code.
Remember, whether you are taking the recursive or iterative route, the key is to always ensure the flexibility and performance of your solution. Implementing these solutions offers you not only the ability to generate permutations effectively but also gives insight into good coding practices in Java.
For deeper insights on recursion and its applications, check out Mastering Recursion in Java.
Happy coding!