Why Just-In-Time Compilation Can Slow Down Your App Performance
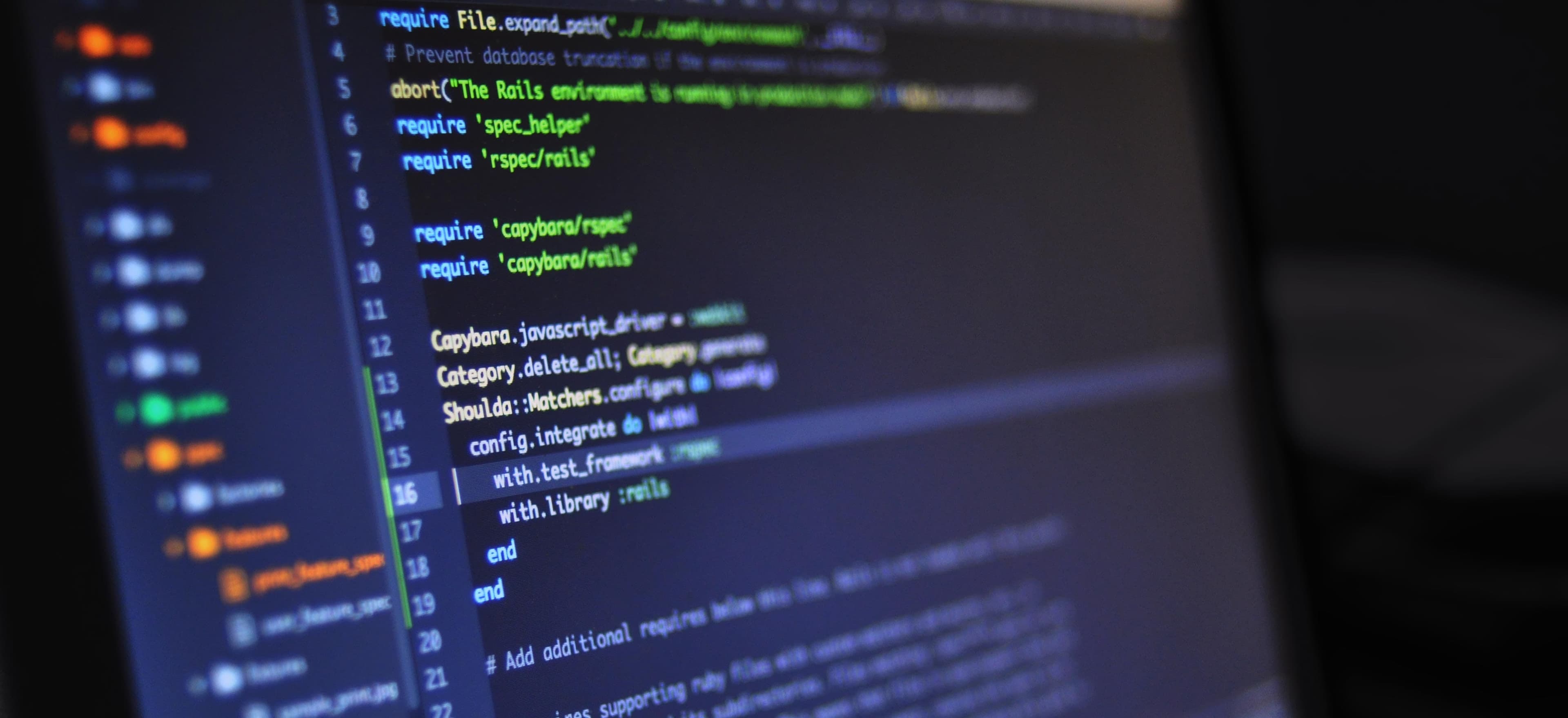
- Published on
Why Just-In-Time Compilation Can Slow Down Your App Performance
Java, known for its "write once, run anywhere" philosophy, utilizes a unique approach to execute code by employing Just-In-Time (JIT) compilation. While JIT compilation enhances runtime performance by converting bytecode to native machine code, it can sometimes lead to unexpected slowdowns in application performance. In this post, we explore why this occurs and what you can do to mitigate these effects.
Understanding Just-In-Time Compilation
JIT compilation is an integral part of the Java Virtual Machine (JVM) that aims to minimize the overhead of interpreting bytecode. Instead of processing Java bytecode line by line, JIT compilers convert frequently executed bytecode sequences into native machine code. This typically results in faster execution times, particularly for long-running applications.
How JIT Compilation Works
When a Java application starts, the JVM begins executing the bytecode using an interpreter. During runtime, the JVM identifies "hot spots" in the code—methods or loops that are executed frequently. The JIT compiler compiles these sections into native code on-the-fly, replacing interpreted bytecode with compiled code as needed.
Here's a simplified view of the JIT compilation process:
- Start Application: The JVM starts executing Java bytecode.
- Identify Hot Spots: The JVM monitors execution to detect frequently-called methods.
- Compile to Native Code: Once a method is hot, the JIT compiler compiles it into native code.
- Replace Bytecode: The JVM replaces bytecode execution with the newly compiled native code for performance.
Example code snippet for JIT hot spot detection:
public class HotSpotExample {
public static void main(String[] args) {
for (int i = 0; i < 10_000; i++) {
compute(i);
}
}
public static void compute(int value) {
// Simulate computation
int sum = 0;
for (int j = 0; j < value; j++) {
sum += j;
}
System.out.println("Sum of " + value + " is: " + sum);
}
}
The Benefits of JIT Compilation
The primary benefit of JIT compilation is improved execution speed. By converting bytecode to native code, applications can run significantly faster, especially in longer tasks or when handling large sets of data. Additionally, the adaptive nature of JIT allows for optimizations based on runtime context—something static compilation cannot achieve.
Where JIT Compilation Can Lead to Slowdowns
Despite its advantages, JIT compilation can introduce performance issues. Here are some common scenarios that may lead to excessive compilation overhead:
1. Warm-up Time
JIT compilation can result in initial latency, referred to as "warm-up time." When a Java application starts, it may run slower until the JIT compiler has time to compile frequently used methods.
Example Scenario
A web service that requires quick responses may suffer during its initial requests. As the service warms up, responses slow down until the critical methods are compiled.
To mitigate warm-up time, consider these approaches:
- Pre-load important classes and methods.
- Use a JIT compiler optimization flag to optimize the compiled methods ahead of time.
2. Over-Optimization
The JVM uses heuristics to determine which methods to compile. In some cases, it might over-optimize code paths based on incorrect assumptions about execution frequency.
For example, a rarely accessed method might be compiled, consuming unnecessary resources. As a result, you'll see delay when transitioning between interpreted and compiled modes.
3. Increased Memory Usage
Compiled native code occupies more memory than interpreted bytecode. If your application continually compiles many methods, it can lead to high memory consumption. This situation slows down garbage collection and increases the risk of out-of-memory errors.
4. De-optimization
While JIT might compile a method for efficiency, if usage patterns change at runtime, the JVM might de-optimize the method, reverting it back to bytecode. This process can add significant overhead, especially in applications with dynamic execution flows.
5. JIT Compiler Warm-Up Configuration
JIT compilation itself can be tuned through various flags (like -XX:CompileThreshold
) to define how many times a method must be called before being compiled. An inappropriate threshold can lead to frequent recompilation or delayed compilation.
Best Practices for Optimizing JIT Compilation
To get the most out of JIT compilation while avoiding pitfalls, consider the following best practices:
1. Profiling Your Application
Use profiling tools to monitor method execution and detect hot spots. This allows you to have better insights into what should be optimized vs. over-optimized.
Resource Suggestion: Use Java Profilers or VisualVM for in-depth analysis.
2. Optimize Algorithmic Complexity
Before diving into compilation configurations, ensure that your application algorithms are efficient. Choose data structures and algorithms that minimize time complexity.
3. Avoid Excessive Method Calls
Breaking down large tasks into smaller methods can lead to excessive method calls and JIT performance bottlenecks. Consider whether you can combine smaller methods for better optimization.
4. Use Static Analysis Tools
Incorporate static analysis tools to catch performance issues during development. Tools like FindBugs or PMD may help identify potential performance bottlenecks before your application is deployed.
5. Tune JVM Options
Employ tuning with appropriate JVM options. Use flags to optimize code compilation and memory management. For instance, -XX:MaxInlineSize
can control method inlining based on size.
java -XX:MaxInlineSize=100 -jar YourApp.jar
Wrapping Up
While JIT compilation plays a crucial role in boosting Java application performance through dynamic optimization, it is not without challenges. The warm-up time, memory usage increases, and potential for over-optimization can hurt performance.
From algorithm optimization to deep profiling, integrating these best practices into your development strategy can help mitigate the performance costs associated with JIT compilation.
As a Java developer, understanding the intricacies of JIT compilation can help you write applications that not only run efficiently but also scale effectively—ensuring that you harness Java's full potential.
Whether you're developing a small application or a large enterprise system, learning how to navigate JIT compilation intricacies can make all the difference in your application's performance and user experience.
For further reading on tuning JVM options, refer to the official Oracle documentation.
For additional insights into JVM performance enhancements, see this Java Performance Tuning guide.