Mastering JDK 9: Overcoming Stream API Confusion
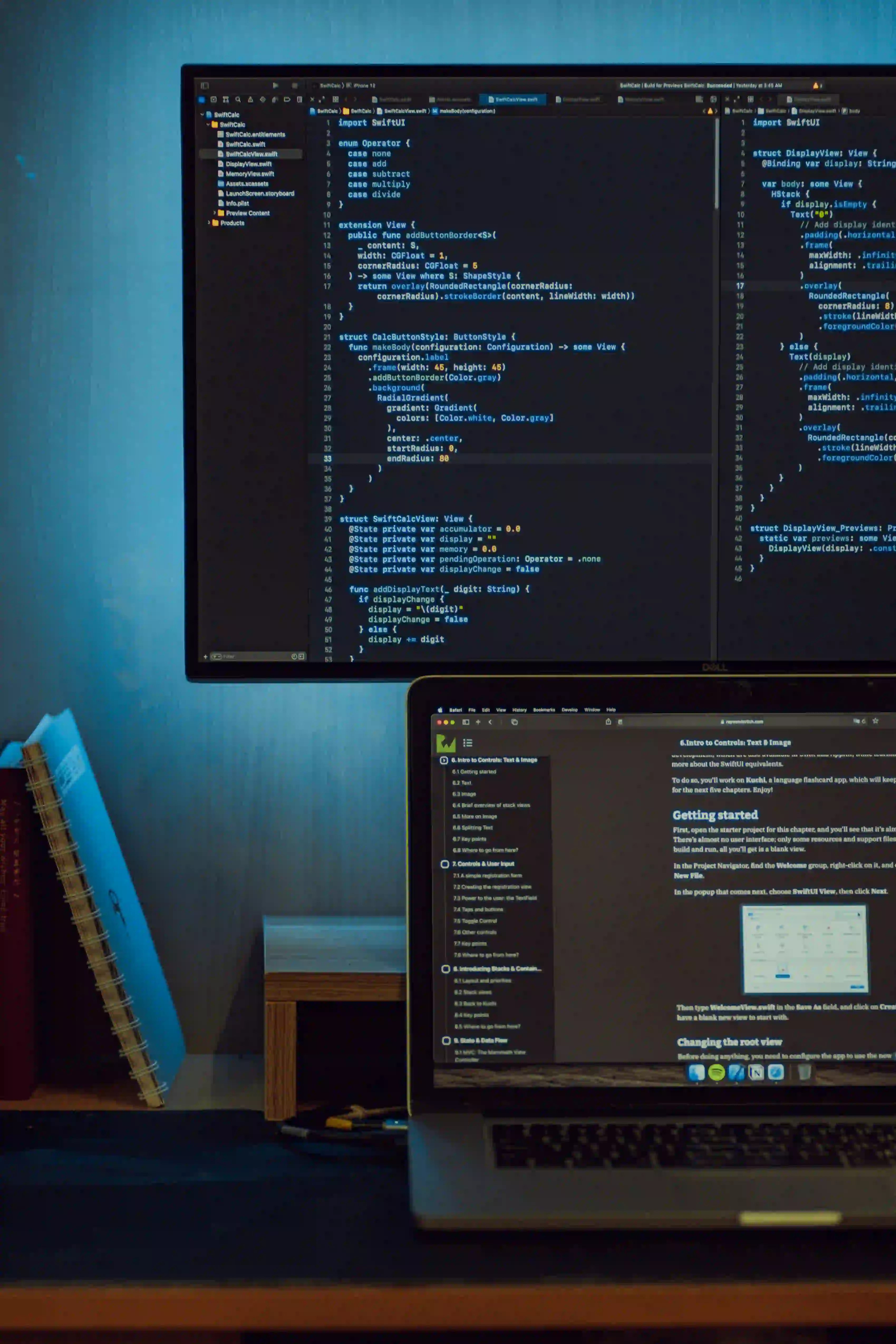
Mastering JDK 9: Overcoming Stream API Confusion
Starting Off
Java Development Kit (JDK) 9 brought many improvements to the Java programming landscape, and one of the most significant enhancements was the addition of various features to the Stream API.
If you're a novice or an experienced Java developer, you might find yourself in a constant struggle to leverage the full power of the Stream API. In this blog post, we'll dissect the Stream API, clarify its purpose, and illuminate its newly introduced functionalities in JDK 9. By the end, you’ll be equipped with the knowledge to utilize streams effectively in your projects.
Understanding the Stream API
The Stream API, introduced in JDK 8, is designed to simplify bulk operations on collections of data. It's essential to understand that a Stream is not a data structure; rather, it's a sequence of elements that allows you to process data in a functional style.
Benefits of Using Streams
- Declarative Approach: Streams enable writing code that's easier to read and understand without the need for boilerplate loops.
- Parallel Processing: They provide built-in support for parallel processing, which can lead to performance improvements.
- Lazy Evaluation: Streams perform operations on-demand, effectively managing resources.
Key Features Introduced in JDK 9
In JDK 9, several enhancements add to the power of the Stream API. Some notable features include:
- TakeWhile() and DropWhile() Methods
- OfNullable() Method
- Iterate() Method Enhancements
Let's explore each of these features in detail.
1. takeWhile()
and dropWhile()
These two methods allow you to process data based on a predicate. takeWhile()
reads elements until a condition fails, and dropWhile()
skips elements while the condition holds true.
Example of takeWhile()
import java.util.List;
import java.util.stream.Collectors;
public class StreamTakeWhileExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 5, 7, 9, 11);
// Elements taken while they are less than 5
List<Integer> result = numbers.stream()
.takeWhile(n -> n < 5)
.collect(Collectors.toList());
System.out.println(result); // Output: [1, 2, 3]
}
}
Commentary
In this example, takeWhile(n -> n < 5)
means that we are collecting elements as long as the numbers are less than 5. Once it encounters 5, it stops. This enables a seamless way to filter data based on conditions.
Example of dropWhile()
import java.util.List;
import java.util.stream.Collectors;
public class StreamDropWhileExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 5, 7, 9, 11);
// Elements dropped while they are less than 5
List<Integer> result = numbers.stream()
.dropWhile(n -> n < 5)
.collect(Collectors.toList());
System.out.println(result); // Output: [5, 7, 9, 11]
}
}
Commentary
In contrast, dropWhile(n -> n < 5)
will ignore all elements that are less than 5, returning the remaining elements. This is beneficial when you only want the data that meets certain criteria after a condition fails.
2. ofNullable()
The ofNullable()
method allows you to create a Stream from a potentially null value. This is a great way to avoid null checks and provide a more robust code.
Example
import java.util.Optional;
import java.util.stream.Stream;
public class StreamOfNullableExample {
public static void main(String[] args) {
String value = null;
// Create Stream from nullable value
Stream<String> stream = Stream.ofNullable(value);
// Collecting non-null values
List<String> result = stream.collect(Collectors.toList());
System.out.println(result); // Output: []
}
}
Commentary
In this example, using Stream.ofNullable(value)
allows for the creation of a Stream without throwing a NullPointerException
. Even if the variable is null, the stream operation completes smoothly.
3. Enhanced iterate()
Before JDK 9, the Stream.iterate()
method could create infinite streams. JDK 9 has introduced a second parameter that serves as a predicate to stop the iteration.
Example
import java.util.stream.Stream;
public class StreamIterateExample {
public static void main(String[] args) {
// Generating a finite stream of even numbers
Stream<Integer> evenNumbers = Stream.iterate(0, n -> n < 20, n -> n + 2);
evenNumbers.forEach(System.out::println);
// Output: 0, 2, 4, 6, 8, 10, 12, 14, 16, 18
}
}
Commentary
In this example, Stream.iterate(0, n -> n < 20, n -> n + 2)
creates a stream of even numbers starting from 0 and terminating before reaching 20. The addition of a stopping condition makes it much safer, preventing infinite loops.
Practical Use Cases
Understanding the features of the Stream API is only part of the battle. Knowing when to use them can impact the efficiency and clarity of your code.
Data Transformation
When you need to transform data in collections, streams are your best bet. For example, if you have a list of items and want to extract certain properties, you can chain Stream methods.
import java.util.List;
import java.util.stream.Collectors;
class Person {
String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
public class StreamTransformationExample {
public static void main(String[] args) {
List<Person> people = List.of(new Person("Alice"), new Person("Bob"));
List<String> names = people.stream()
.map(Person::getName)
.collect(Collectors.toList());
System.out.println(names); // Output: [Alice, Bob]
}
}
Filtering Data
The Stream API shines when you need to filter a collection based on specific criteria.
import java.util.List;
import java.util.stream.Collectors;
public class StreamFilterExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4, 6, 8, 10]
}
}
My Closing Thoughts on the Matter
Navigating the Stream API can initially seem daunting, but the enhancements provided in JDK 9 offer critical functionalities that can simplify your code significantly. With methods like takeWhile()
, dropWhile()
, and ofNullable()
, you can handle data processing tasks with elegance and efficiency.
Incorporating these features into your Java applications is a step towards more functional programming paradigms and can lead to better maintainability and clarity in your code.
If you're looking to deepen your understanding even further, consider exploring the official Java Documentation and experimenting with other advanced features that the Stream API has to offer. Happy coding!