Overcoming Common Pitfalls in Flyway Database Migrations
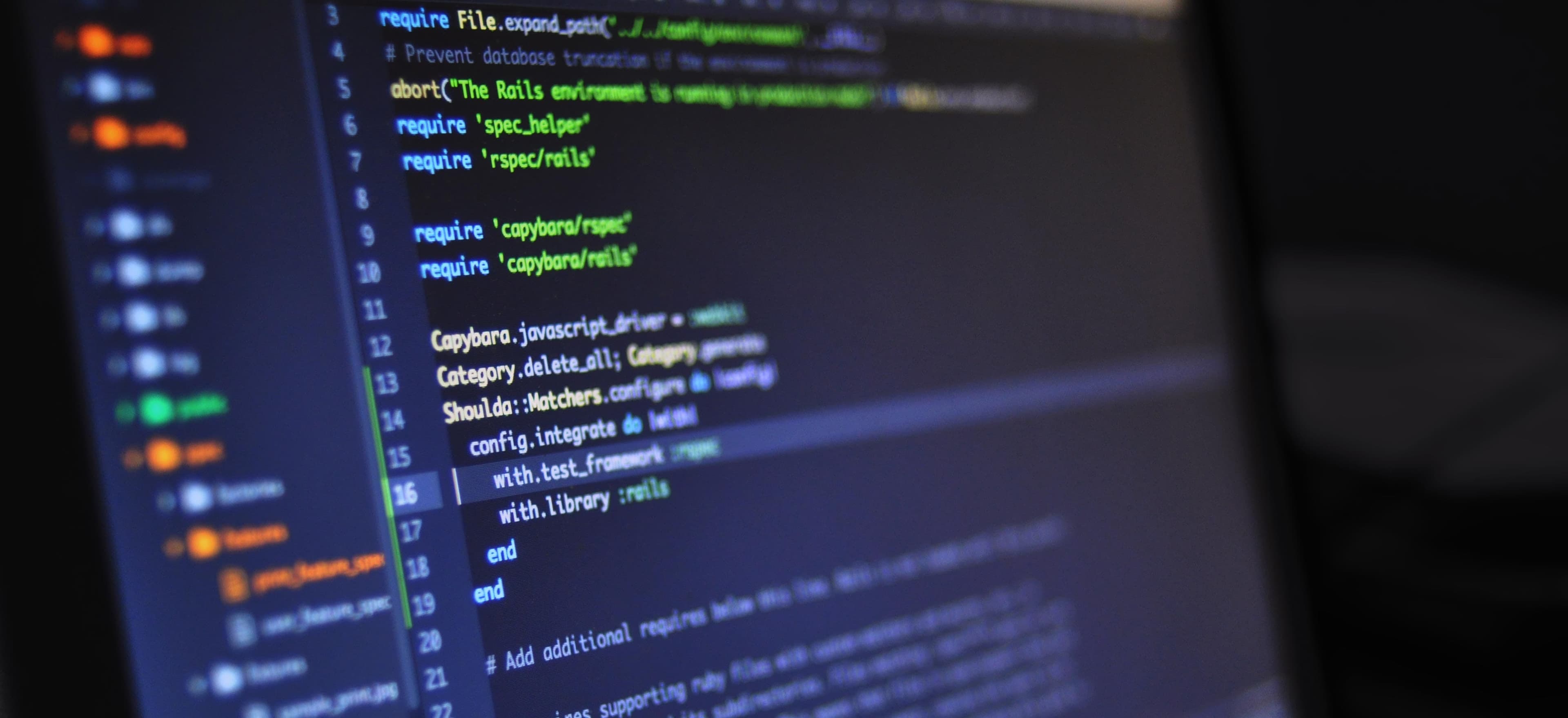
- Published on
Overcoming Common Pitfalls in Flyway Database Migrations
Flyway is a powerful tool for managing database migrations, allowing developers to apply version control features to their databases in a straightforward and reliable manner. However, working with Flyway is not without its challenges. Many developers encounter pitfalls that can cause frustrating delays and unexpected behavior. This blog post will discuss common issues in Flyway migrations and provide strategies to mitigate them. By understanding these pitfalls, you can ensure smoother database migrations and maintain a clean database schema.
Understanding Flyway
Before diving into specific challenges, it’s important to understand what Flyway does. Flyway operates by applying migrations—SQL scripts or Java-based migrations—that alter the state of the database schema. It tracks which migrations have been applied through a dedicated table called flyway_schema_history
.
One of Flyway’s strengths is its ability to maintain the integrity and consistency of your database, especially in development and production environments. However, issues can arise based on how Flyway interacts with your database.
Common Pitfalls
Let's delve into common pitfalls developers face using Flyway and discuss how to overcome them.
1. Out-of-Order Migrations
One of the most common pitfalls developers face is applying migrations out of order. This often happens when developers create new migrations without careful tracking. The result can be broken migrations or, at worst, data corruption.
Solution: Use versioned migrations. Flyway uses a naming convention for its migration files typically prefixed with a version number. For example, naming a migration file V1__Create_users_table.sql
ensures that Flyway applies it in order.
-- V1__Create_users_table.sql
CREATE TABLE users (
id INT PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(255) NOT NULL,
password VARCHAR(255) NOT NULL
);
By following this pattern, Flyway helps enforce that migrations are applied in the correct order, thus reducing out-of-order migration issues.
2. Ignoring the Baseline Version
When introducing Flyway to an existing project, it is crucial to set a baseline version. Failing to do so can lead to Flyway treating the entire database as needing migrations, resulting in historical data loss or corruption.
Solution: Set a baseline version using the Flyway baselineVersion
property. This sets a point from which Flyway starts tracking migrations.
flyway.baselineVersion=1
flyway.baselineOnMigrate=true
By establishing this baseline, Flyway will recognize which migrations to apply and will not attempt to query existing schema states.
3. Mixing Migration Types
Mixing SQL-based and Java-based migrations can create confusion. SQL migrations are generally easier to handle and less prone to errors; however, there are situations where Java migrations are necessary.
Solution: Use SQL migrations where possible. Reserve Java migrations for more complex logic that cannot easily be represented in SQL. If a migration requires changing data or executing conditional logic, a Java migration might be appropriate.
Here’s how you create a basic Java migration:
import org.flywaydb.core.api.migration.BaseJavaMigration;
import org.flywaydb.core.api.migration.Context;
public class V2__Update_user_passwords extends BaseJavaMigration {
@Override
public void migrate(Context context) throws Exception {
// Logic to update user passwords
try (var statement = context.getConnection().createStatement()) {
statement.executeUpdate("UPDATE users SET password = 'newPassword' WHERE username = 'johnDoe'");
}
}
}
In this case, using Java for behavior that SQL cannot easily provide ensures your migrations remain maintainable and clear.
4. Missing Rollback Strategies
Database migrations typically represent changes that should ideally be reversible. However, many migrations lack explicit ROLLBACK
strategies. If a migration fails, this can lead to database states that are hard to trace.
Solution: Implement rollback strategies for every migration. This may involve writing additional scripts that undo changes. While Flyway does not support automatic rollbacks, having a clear plan to handle failures can save time.
For example:
-- V3__Add_email_to_users.sql
ALTER TABLE users ADD email VARCHAR(255);
-- Rollback strategy
-- V3__Remove_email_from_users.sql
ALTER TABLE users DROP COLUMN email;
Maintaining complementary rollback scripts provides a safety net if deployment does not go as planned.
5. Not Testing Migrations in a Sandbox Environment
Deploying migrations directly to production without testing can lead to critical errors and data loss. This is a standard pitfall in any database management practice.
Solution: Always test migrations in a controlled environment before applying them to the production database. Utilize transient databases to simulate real-world scenarios.
Ensure that your CI/CD pipeline includes a step that applies the migration in a test environment before it is applied in production. This adds a layer of assurance that your migrations will run smoothly.
6. Not Checking Compatibility with Database Versions
Different versions of databases may handle migrations differently. Developers often overlook compatibility and encounter a lot of unexpected behaviors due to differences in SQL dialects.
Solution: Always verify SQL commands against the target database version's documentation. Test your migration scripts against the database version you are using in production.
You might want to consider using tools like Docker to create an environment that matches production closely. This practice helps in identifying issues during migration that you might not have anticipated.
Closing Remarks
As you work with Flyway for your database migrations, being aware of common pitfalls will significantly enhance your development experience. From issues related to migration order to the importance of rollback strategies, each topic discussed here serves to underscore best practices that foster reliable database evolution.
Implement these strategies and techniques, and you'll not only avoid frustrating scenarios but also contribute to more stable and maintainable code. To further your understanding, check out the Flyway Documentation for more in-depth guidance and the latest updates.
By overcoming these common pitfalls, you will elevate your database management skills and maintain cleaner workflows with Flyway. Happy coding!