Troubleshooting Common Issues with Maven Jetty Plugin
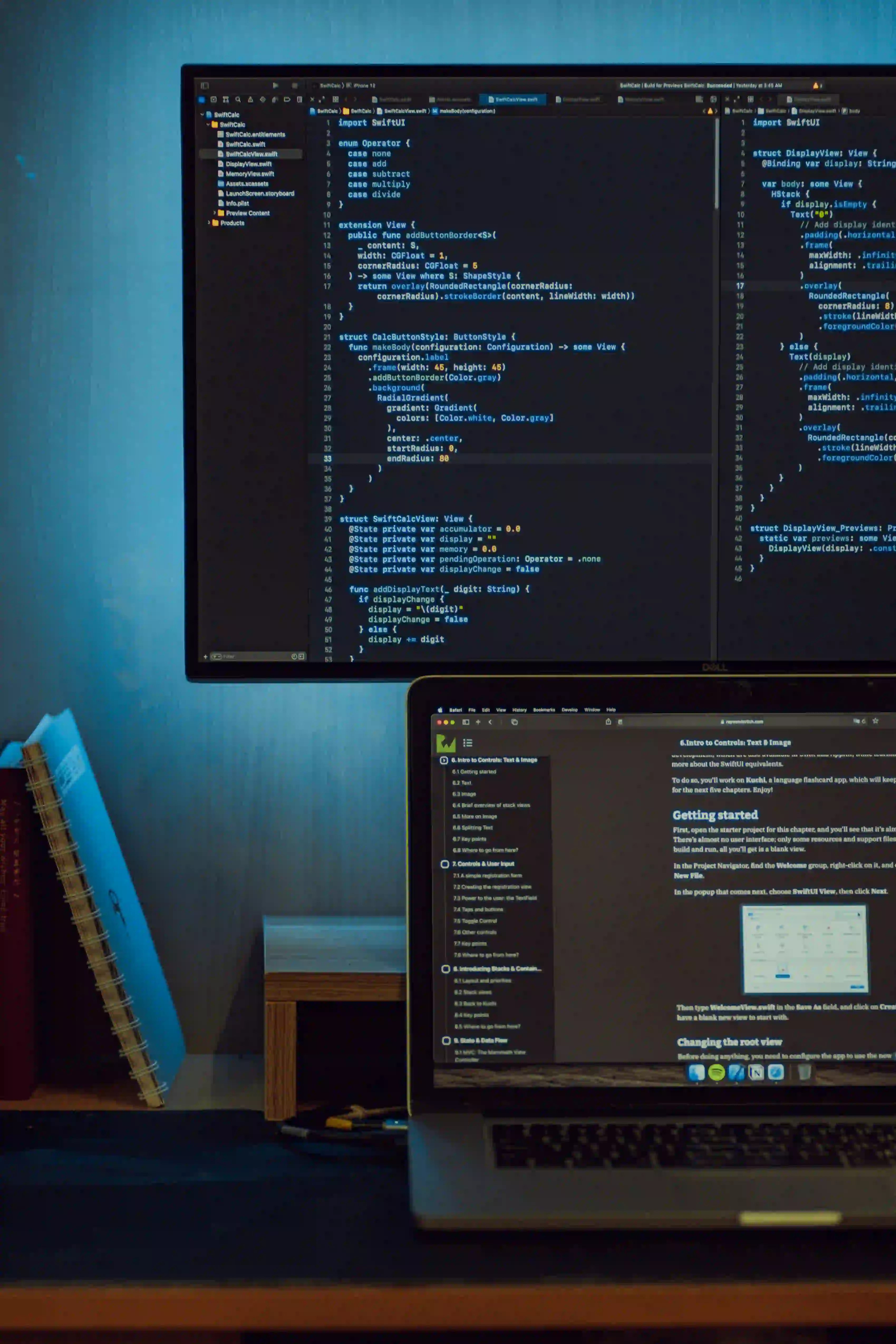
Troubleshooting Common Issues with Maven Jetty Plugin
Maven Jetty Plugin is an indispensable tool for Java developers who want to test and run their web applications in a local environment effortlessly. The plugin allows developers to operate an embedded Jetty server from the command line. However, as with any technology, issues may arise. This blog post will cover common problems encountered while using the Maven Jetty Plugin and their solutions.
What is the Maven Jetty Plugin?
The Maven Jetty Plugin makes it easy to run a Jetty web application server in a Maven build process. It allows developers to deploy their applications locally, facilitating real-time testing and development cycles. Learn more about Maven Jetty Plugin here.
Setting Up the Maven Jetty Plugin
To get started, you must first configure the Jetty plugin in your pom.xml
. Here is a basic setup:
<build>
<plugins>
<plugin>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-maven-plugin</artifactId>
<version>9.4.43.v20210629</version>
<configuration>
<webApp>
<contextPath>/my-app</contextPath>
</webApp>
</configuration>
</plugin>
</plugins>
</build>
Why Use Jetty in Development?
- Quick Iterations: Changes can be reflected immediately.
- Lightweight: Jetty consumes fewer resources than other servers like Tomcat, making it ideal for local development.
- Configuration Flexibility: Custom configurations can be applied mindfully for various project needs.
Common Issues and Their Solutions
1. Dependencies Version Conflicts
Issue:
The Jetty server fails to start due to conflicting versions of dependencies.
Solution:
Ensure that all your dependencies are compatible with the Jetty version you are using. You can use the mvn dependency:tree
command to analyze your dependency tree and identify version conflicts.
Example:
mvn dependency:tree
Commentary:
Dependency conflicts often arise when transitive dependencies pull in versions incompatible with the Jetty server. Resolve these by overruling specific dependency versions in your pom.xml
.
2. Port Already in Use
Issue:
You receive an error stating that the port is already in use when starting the Jetty server.
Solution:
You can either stop the process using the port or change the port in the Jetty configuration.
Changing the Port Example:
<configuration>
<port>8081</port>
</configuration>
Commentary:
Port conflicts occur frequently in development environments where multiple applications are running. It's a good practice to keep track of the ports you're using.
3. Application Not Accessible at Context Path
Issue:
After starting Jetty, you cannot access your application at the desired context path.
Solution:
Double-check the contextPath
in your plugin configuration and ensure your application is being deployed within the correct path.
Example:
<configuration>
<webApp>
<contextPath>/my-app</contextPath>
</webApp>
</configuration>
Commentary:
If your browser shows a 404 error, it often indicates a mismatch between the configured context path and the URL you are attempting to access.
4. Servlet Not Found
Issue:
The application fails to find certain servlets you've configured.
Solution:
Verify that you've mapped your servlets correctly and that they are included in the web application structure.
<servlet>
<servlet-name>MyServlet</servlet-name>
<servlet-class>com.example.MyServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>MyServlet</servlet-name>
<url-pattern>/example</url-pattern>
</servlet-mapping>
Commentary:
Misconfigurations in the web.xml
can lead to your servlets not being recognized. Ensure that you correctly follow the Servlet specification.
5. Improper Cleanup on Shutdown
Issue:
When using the command mvn jetty:stop
, the Jetty server does not shut down properly.
Solution:
Make sure you are using the correct lifecycle calls. Sometimes, configurations can lead to failure in clean shutdown.
Example Command:
mvn jetty:stop
Commentary:
Failure to stop the Jetty server leaves orphan processes running, which can interfere with subsequent runs. Proper lifecycle management is paramount.
Additional Tips for a Smooth Experience
- Always Check Logs: Jetty provides detailed logging information that can help diagnose issues more effectively.
- Set up Automatic Testing: Integrating JUnit and Maven Surefire Plugin can help automate testing during the Jetty lifecycle.
- Network Configuration: Ensure your firewall settings allow local connections on the configured port.
My Closing Thoughts on the Matter
The Maven Jetty Plugin is a powerful tool for Java developers. Although it simplifies local development and testing, it can be prone to common issues that disrupt your workflow. By understanding these problems and implementing their solutions, you can streamline your development process and maximize your productivity.
As you continue to work with the Maven Jetty Plugin, remember that tackling problems head-on can be an educational experience. Each issue resolved adds to your skill set and prepares you for more robust application development.
For additional information, consider checking the official Jetty Documentation, which provides more extensive resources on advanced configurations and troubleshooting techniques. Happy coding!