Securing Your APIs: Opaque Token Challenges in Spring Boot
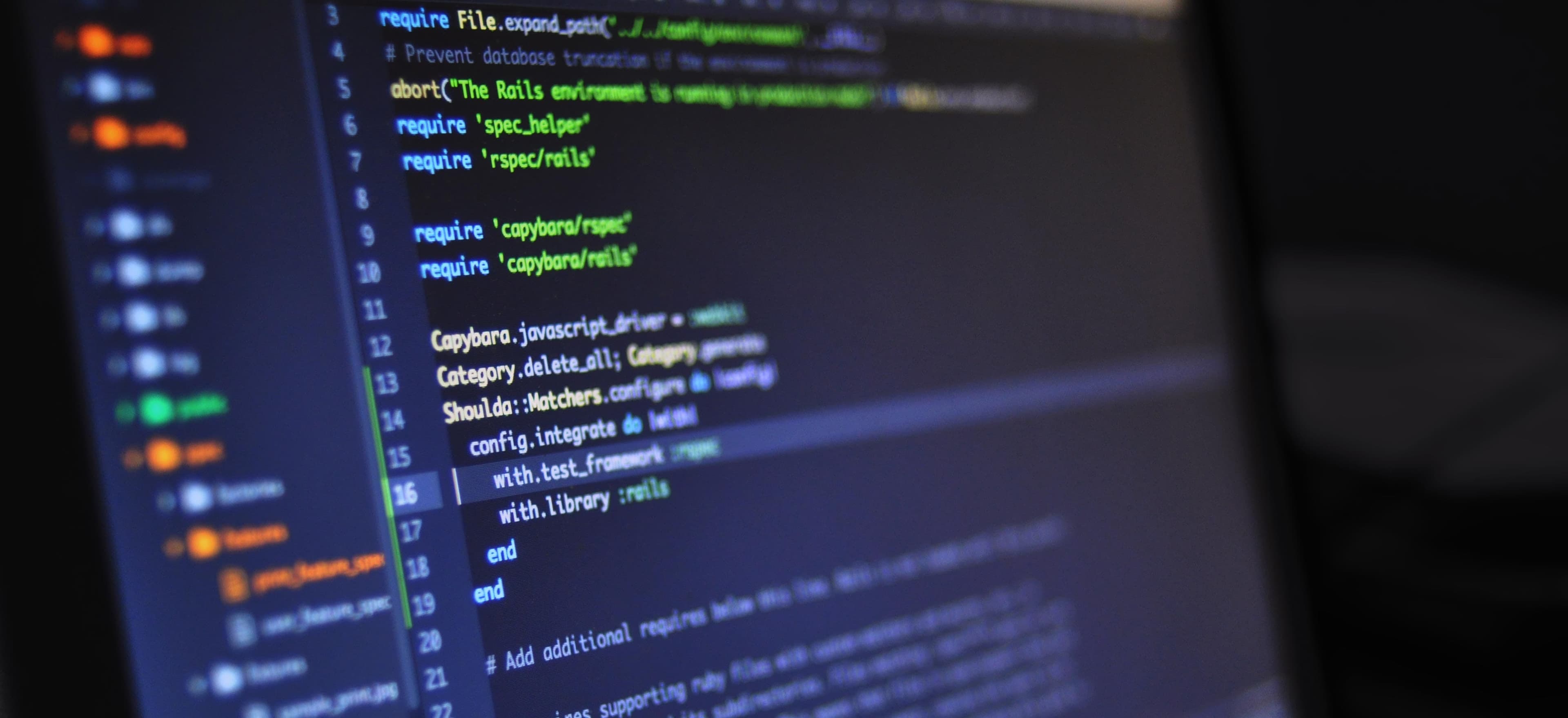
- Published on
Securing Your APIs: Opaque Token Challenges in Spring Boot
In today's digital landscape, API security has become a pressing concern. With cyber threats growing in sophistication, developers must ensure that their APIs are secure against attacks. One such solution gaining traction is the use of opaque tokens in API authentication. In this blog post, we will discuss opaque tokens, their benefits, and how to implement them effectively in a Spring Boot application.
Understanding Opaque Tokens
Opaque tokens are security tokens that a client application uses to access resources on behalf of a user. Unlike JWT (JSON Web Tokens), opaque tokens do not carry any information about the user or the session within themselves. Instead, they serve as a reference to an authentication session stored on the server.
Benefits of Using Opaque Tokens
-
Increased Security: Since opaque tokens do not expose any user details, even if an attacker intercepts the token, they cannot derive any meaningful information from it.
-
Token Revocation: Opaque tokens can be easily revoked on the server-side. This allows developers to invalidate a user's session without requiring them to change their entire token structure, enhancing security.
-
Simplified Token Management: Developers only need to manage server-side session data, making the implementation straightforward.
Challenges with Opaque Tokens
While opaque tokens present several security advantages, several challenges also arise when implementing them in a Spring Boot application:
-
Server-side Storage: Opaque tokens require the server to maintain a database or a data store, creating an overhead in management.
-
Performance Concerns: Constantly querying the database to validate tokens can lead to performance bottlenecks.
-
Scalability: As the user base grows, the server must efficiently handle a large number of token validation requests.
In the following sections, we will address these challenges by implementing opaque tokens in a Spring Boot application step-by-step.
Implementing Opaque Tokens in Spring Boot
Prerequisites
- Java: Version 11 or above.
- Spring Boot: Version 2.5 or above.
- Maven: Latest version.
- Database: Choose between H2 (for development) or any other RDBMS for production.
Step 1: Setting Up the Spring Boot Application
Start by creating a new Spring Boot application. You can use Spring Initializr to bootstrap your project with the necessary dependencies:
- Spring Web
- Spring Security
- Spring Data JPA (for database interactions)
- H2 Database (optional, for quick testing)
Pom.xml Configuration
Ensure your pom.xml
includes the following dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Step 2: Creating User and Token Models
Create user and token entity classes to represent the data stored in the database.
User Entity
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
// Getters and Setters
}
Opaque Token Entity
@Entity
public class OpaqueToken {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String token;
@ManyToOne
private User user;
private LocalDateTime expiryDate;
// Getters and Setters
}
Step 3: Token Generation and Storage
When users log in, generate the opaque token and store it in the database alongside its corresponding user and expiration date.
Token Generation Service
@Service
public class TokenService {
@Autowired
private OpaqueTokenRepository tokenRepository;
@Autowired
private UserRepository userRepository;
public String generateToken(String username) {
User user = userRepository.findByUsername(username);
String token = UUID.randomUUID().toString();
OpaqueToken opaqueToken = new OpaqueToken();
opaqueToken.setToken(token);
opaqueToken.setUser(user);
opaqueToken.setExpiryDate(LocalDateTime.now().plusHours(1)); // Token lifespan
tokenRepository.save(opaqueToken);
return token;
}
}
Step 4: Authenticating Users
You need to implement user authentication. Here’s a simple example using Spring Security to check user credentials.
Security Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private TokenService tokenService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(username -> {
// Load user from database and return UserDetails
User user = userRepository.findByUsername(username);
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), new ArrayList<>());
});
}
}
Step 5: Using the Opaque Token
During the authentication process, create the token and send it back to the client as a part of the response.
Authentication Controller
@RestController
public class AuthController {
@Autowired
private AuthenticationManager authenticationManager;
@Autowired
private TokenService tokenService;
@PostMapping("/login")
public ResponseEntity<String> login(@RequestBody AuthRequest authRequest) {
authenticationManager.authenticate(new UsernamePasswordAuthenticationToken(authRequest.getUsername(), authRequest.getPassword()));
String token = tokenService.generateToken(authRequest.getUsername());
return ResponseEntity.ok(token);
}
}
Step 6: Token Validation
To secure your endpoints, you need to validate the opaque token with middleware.
Token Filter
@Component
public class TokenFilter extends OncePerRequestFilter {
@Autowired
private OpaqueTokenRepository tokenRepository;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
String token = request.getHeader("Authorization");
if (token != null && tokenRepository.existsByToken(token)) {
// Token is valid; proceed with the request
} else {
response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Invalid Token");
return;
}
filterChain.doFilter(request, response);
}
}
Key Takeaways
Implementing opaque tokens in your Spring Boot application significantly enhances security. While it introduces challenges, such as needing to manage tokens in the database, the benefits far outweigh these hurdles. By leveraging tools like Spring Security and JPA, developers can create robust, secure APIs that guard against unauthorized access effectively.
For further reading on security best practices, you might find OWASP API Security Top 10 incredibly useful. Additionally, don’t hesitate to explore the official Spring Security Documentation for more advanced configurations and features.
By following these guidelines, you can take decisive steps toward enforcing solid security measures in your applications while harnessing the power of Spring Boot and opaque token authentication. Happy coding!
Checkout our other articles