Fixing UnsupportedTemporalTypeException in Java Time API
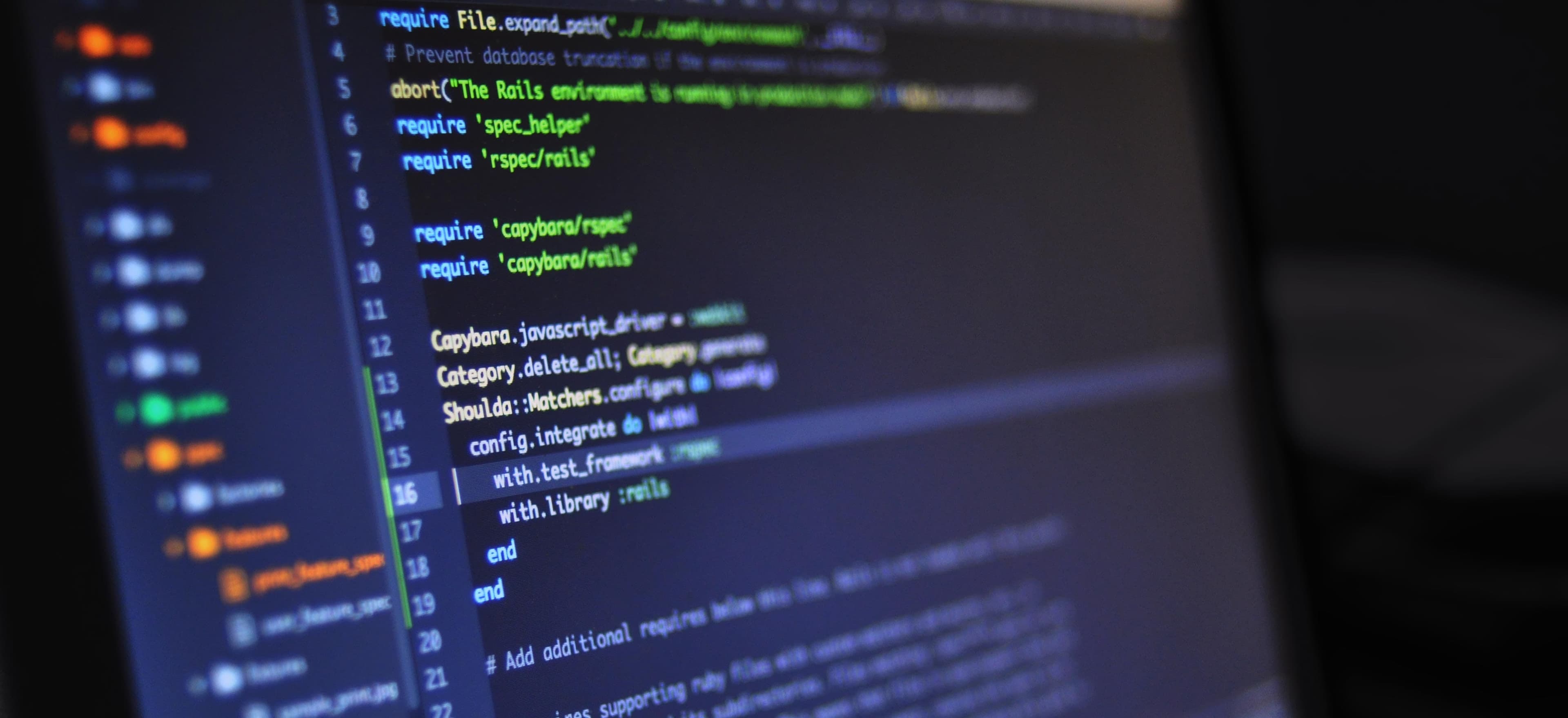
- Published on
Fixing UnsupportedTemporalTypeException in Java Time API
The Java Time API, introduced in Java 8, has made date and time handling more manageable and intuitive. However, as with any robust library, developers may face various exceptions when utilizing these new features. One common issue is the UnsupportedTemporalTypeException
, which can arise under certain conditions while manipulating date and time objects. In this blog post, we'll delve into what this exception means, when it occurs, and how to effectively fix it.
What is UnsupportedTemporalTypeException?
UnsupportedTemporalTypeException
is part of the java.time.temporal
package. This exception is thrown when a temporal query, adjustment, or formatting request contains an unsupported type. Essentially, it indicates that a particular operation cannot be completed because the temporal type does not support the requested feature.
For example, if you're trying to manipulate a LocalDate
object by retrieving its time component, you'll encounter this exception since LocalDate
only encompasses date information.
Common Scenarios Leading to UnsupportedTemporalTypeException
Here are a few situations that might trigger this exception:
- Incompatibility of Temporal Types: Using a time-related method on a date type.
- Excessive Querying: Attempting to extract values that a specific temporal type does not hold.
- Manipulating Unsupported Fields: Trying to manipulate fields that are not applicable for the given temporal type.
Let's explore a few examples that produce this exception and ways to address them effectively.
Example 1: Extracting Time from LocalDate
Code Snippet
import java.time.LocalDate;
import java.time.temporal.ChronoField;
public class TemporalExample {
public static void main(String[] args) {
try {
LocalDate localDate = LocalDate.now();
// Attempting to get hour from LocalDate will throw UnsupportedTemporalTypeException
int hour = localDate.get(ChronoField.HOUR_OF_DAY);
System.out.println("Hour: " + hour);
} catch (UnsupportedTemporalTypeException e) {
System.out.println("Caught exception: " + e.getMessage());
}
}
}
Explanation
Here, we're trying to retrieve the hour of a LocalDate
instance. Since LocalDate
encompasses only date information (year, month, and day), trying to access the time field results in an UnsupportedTemporalTypeException
.
Solution
To fix this, you can either:
- Use
LocalDateTime
orZonedDateTime
if time components are necessary:
import java.time.LocalDateTime;
public class TemporalExample {
public static void main(String[] args) {
LocalDateTime localDateTime = LocalDateTime.now();
int hour = localDateTime.getHour();
System.out.println("Hour: " + hour);
}
}
- Avoid unnecessary calls to non-supported fields based on the type you're working with.
Example 2: Using Instant
with LocalDate
Code Snippet
import java.time.Instant;
import java.time.LocalDate;
public class DateExample {
public static void main(String[] args) {
LocalDate localDate = LocalDate.of(2023, 10, 25);
Instant instant = localDate.atStartOfDay().toInstant(java.time.ZoneOffset.UTC);
System.out.println("Instant: " + instant);
// Trying to get epochs from LocalDate directly
try {
long epochMilli = localDate.toEpochDay();
System.out.println("Epoch Milliseconds: " + epochMilli);
} catch (UnsupportedTemporalTypeException e) {
System.out.println("Caught exception: " + e.getMessage());
}
}
}
Explanation
In this scenario, we first create a LocalDate
and then correctly convert it to Instant
for a point in time. However, directly trying to get epochMilli
from LocalDate
raises an exception. This is because epoch time is represented in milliseconds, and LocalDate
was designed primarily for date handling without a time context.
Solution
To correctly obtain the epoch time, utilize conversion methods or work with the correct type:
import java.time.LocalDate;
import java.time.ZoneId;
public class DateExample {
public static void main(String[] args) {
LocalDate localDate = LocalDate.of(2023, 10, 25);
long epochDay = localDate.toEpochDay(); // Correct: returns days since epoch.
System.out.println("Epoch Days: " + epochDay);
// Correctly obtaining an Instant
Instant instant = localDate.atStartOfDay(ZoneId.systemDefault()).toInstant();
System.out.println("Instant: " + instant);
}
}
Avoiding UnsupportedTemporalTypeException
To consistently avoid UnsupportedTemporalTypeException
, you should adhere to these best practices:
- Check Type Compatibility: Always ensure the method you choose aligns with the temporal type you are working with.
- Use Alternative Types: If you need both date and time, consider using
LocalDateTime
,ZonedDateTime
orInstant
depending on your needs. - Consult the Documentation: The official Java documentation includes comprehensive descriptions of each temporal type and the operations they support.
My Closing Thoughts on the Matter
Navigating the landscape of Java's Time API can be challenging due to the complexities of temporal types and their respective functionalities. Understanding and handling exceptions like UnsupportedTemporalTypeException
is imperative for writing robust applications.
Throughout this discussion, we've examined common scenarios leading to this exception. Armed with this knowledge, you can prevent runtime issues in your Java applications related to date and time manipulations. For more advanced lessons, check out tutorials on Java Time API that enhance your understanding of temporal types and their intricacies.
Remember, the key to mastering the Java Time API is practice and continuous learning. Happy coding!
Checkout our other articles