Navigating the Pitfalls of Private Methods in Java 9 Interfaces
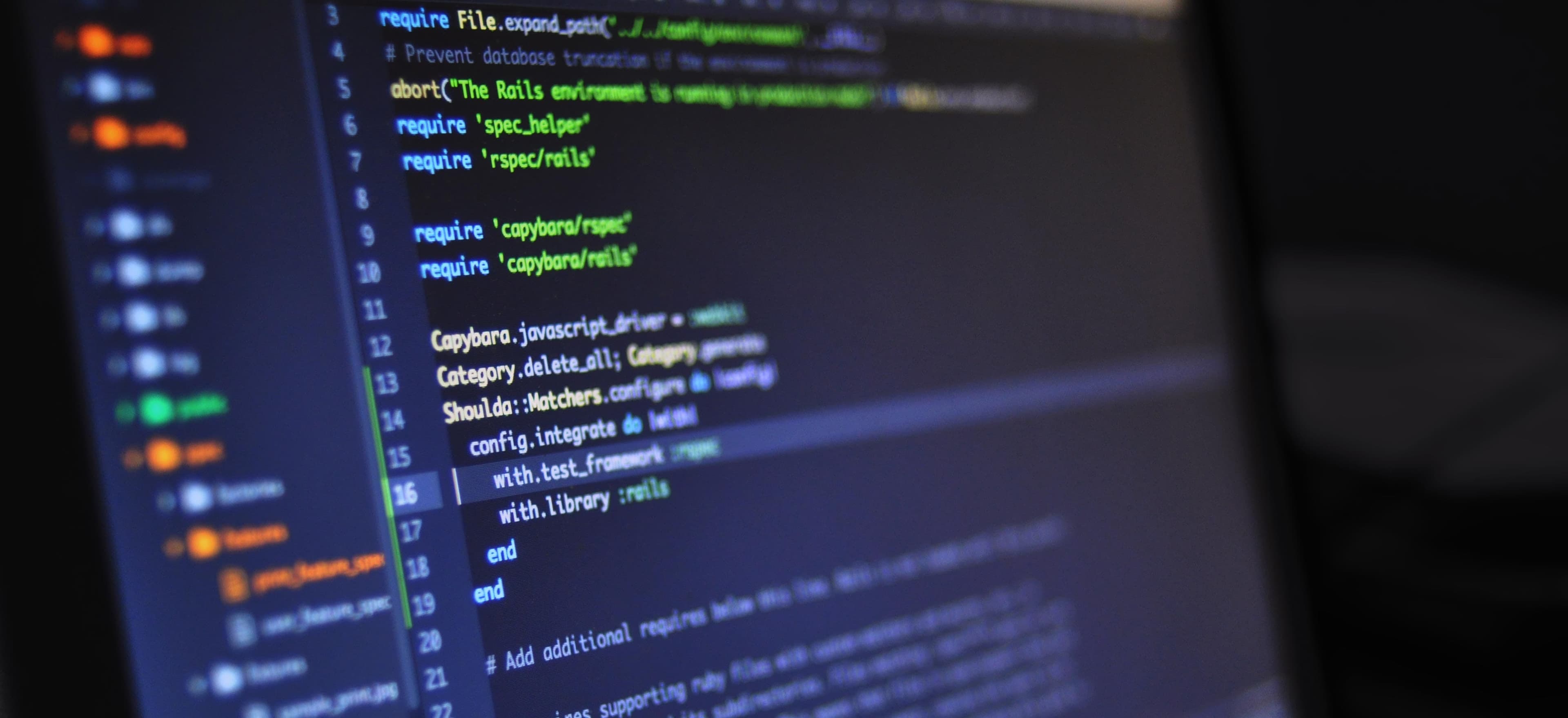
- Published on
Navigating the Pitfalls of Private Methods in Java 9 Interfaces
Java has evolved significantly since its inception. With the introduction of Java 9, a seismic shift occurred with the enhancement of interfaces. One of the most notable features was the ability to include private methods in interfaces. While this development promotes code reuse and cleaner interfaces, it also presents some challenges. This blog post will explore these pitfalls, offering insights on effective navigation and practices for Java developers.
Understanding Private Methods in Interfaces
Before diving into the pitfalls, it’s crucial to understand the role of private methods within Java interfaces. In previous versions of Java, interfaces could only contain public methods. The introduction of private methods allows developers to define helper methods that can be used within the implementing classes.
Example of Private Method in Interface:
public interface Shape {
double area();
default void printInfo() {
System.out.println("Area: " + area());
printBorder();
}
private void printBorder() {
System.out.println("----------");
}
}
Explanation:
- Default Method: The
printInfo
method is a default method that calls another private method,printBorder
. This allows developers to encapsulate behavior that is not exposed publicly, promoting better design. - Reuse: The
printBorder
method can be reused across multiple default methods in the interface.
Advantages of Private Methods
Before we discuss the pitfalls, let’s outline the benefits private methods offer:
- Code Reusability: Developers can avoid code duplication by defining common logic within private methods.
- Encapsulation: Private methods allow for better encapsulation of behavior that should not be exposed outside the interface.
- Improved Readability: By abstracting complex logic into private methods, the readability of public or default methods improves.
Pitfalls of Private Methods in Interfaces
While private methods provide several advantages, they also come with certain challenges. Below are some key pitfalls to consider:
1. Lack of Inheritance
Unlike public methods, private methods in interfaces cannot be inherited by implementing classes. This limitation can lead to unexpected behavior or redundancy in code.
Example:
public class Rectangle implements Shape {
@Override
public double area() {
return 10.0 * 5.0; // Area calculation
}
}
In the above case, Rectangle
cannot access printBorder()
directly. Instead, it has to rely on printInfo
. This may lead to repeated logic across different implementations if not managed properly.
2. Difficulty in Testing
Testing private methods can be problematic. Since these methods are not accessible from implementing classes or external classes, it may lead to challenges in ensuring the reliability of the code.
Suggested Approach:
Use code coverage tools to ensure that the public methods invoking private methods are well-tested. However, rely on good design principles and avoid heavy reliance on the testing of private logic.
3. Increased Complexity
Adding private methods may increase the complexity of an interface. As functions get more sophisticated, the interface can become harder to maintain.
Best Practice:
Keep interfaces simple and focused. Avoid bloating them with too much logic, which can lead to poor design. Instead, delegate complex behavior to separate classes or utility classes where necessary.
4. Misleading API Design
Using private methods incorrectly can lead to a misleading API. Users might assume that certain functionality is part of the public API when, in fact, it is a hidden implementation detail.
Example:
Consider an API that presents a method as simple when it actually uses several layers of private methods. This could confuse other developers who might expect that all behavior is documented.
public interface Logger {
void log(String message);
default void logWithBorder(String message) {
printBorder();
log(message);
printBorder();
}
private void printBorder() {
System.out.println("----------");
}
}
In this case, others using Logger
may not be aware of the printBorder
methodology unless they read through the source code, leading to confusion about its intended behavior.
Best Practices for Navigating Pitfalls
To leverage the benefits of private methods and sidestep their pitfalls, consider the following best practices:
1. Use Descriptive Naming Conventions
Choose method names that clearly convey their purpose. This ensures that even if a method is private, its intent is understandable.
2. Keep Interfaces Focused
Adhering to the Single Responsibility Principle (SRP) ensures that your interfaces remain clean and easy to maintain. Avoid convoluted logic in interfaces by limiting their scope.
3. Document Private Methods
Even though private methods are not publicly accessible, maintaining documentation on their intent and usage can prevent confusion during development.
4. Optimize Testing Strategies
Focus on testing the public API extensively. Make sure that private methods are invoked through public methods to ensure that their functionality is indirectly tested.
5. Refactor Responsibly
If you find that private methods are becoming complex, it might be time to refactor. Consider moving complex logic to dedicated classes or utility classes that adhere to specific responsibilities.
Key Takeaways
In summary, private methods in Java 9 interfaces offer dramatic improvements in code reuse and encapsulation. However, they also come with challenges, including inheritance issues, testing difficulties, increased complexity, and potentially misleading API designs.
Employing best practices such as clear naming conventions, focused interfaces, thorough documentation, strategic testing, and responsible refactoring will help you navigate these pitfalls effectively.
As you embrace the power of private methods, remember: the goal is not just to write functional code but to create clean, maintainable, and user-friendly APIs.
Further Reading
For those interested in delving deeper into Java and its interface capabilities, check out the following resources:
- Java 9 Language Changes
- Effective Java by Joshua Bloch
- Understanding Java Interfaces
Embrace the journey of learning Java, and happy coding!