Top SQL Mistakes Java Developers Make: Avoid These!
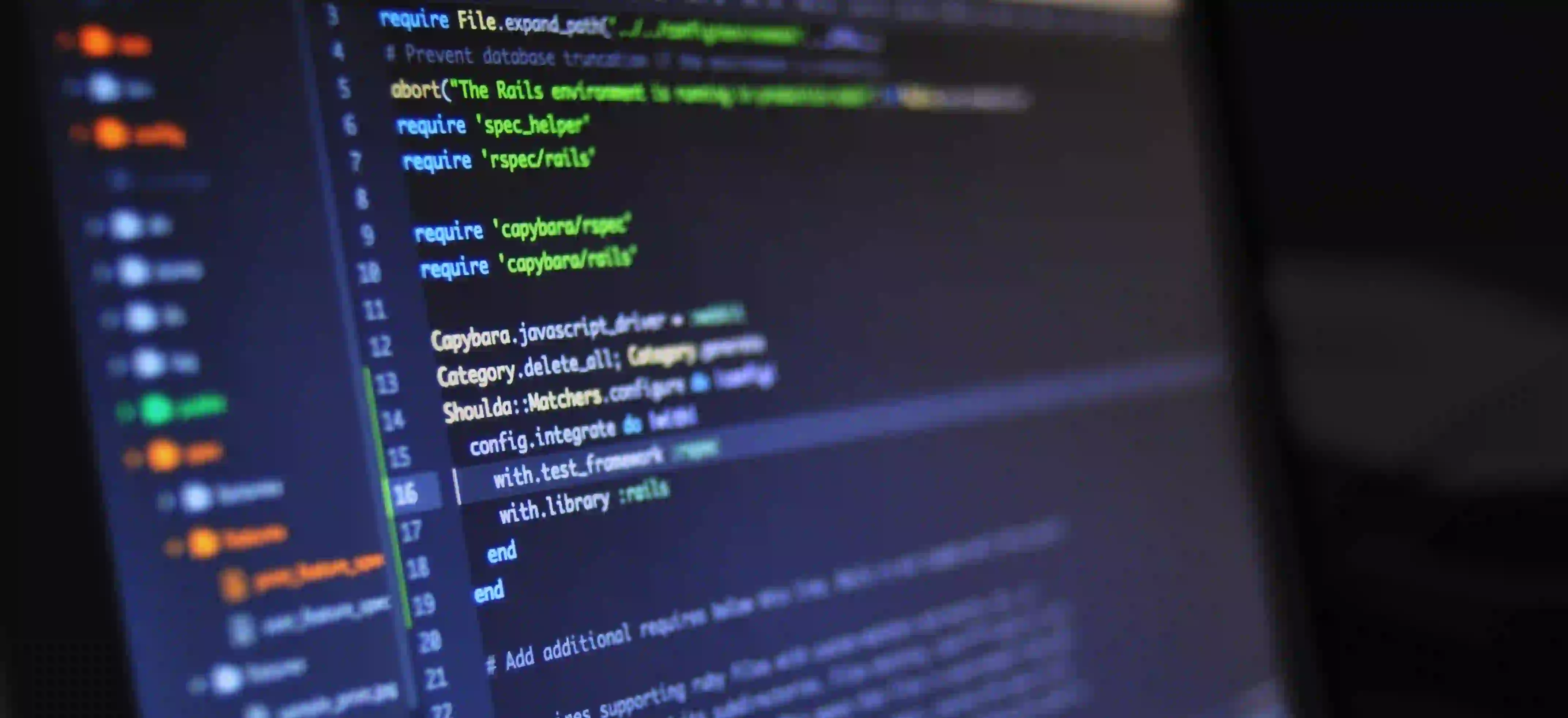
Top SQL Mistakes Java Developers Make: Avoid These!
As a Java developer venturing into the world of databases, it’s crucial to learn the ins and outs of SQL. While Java and SQL may seem worlds apart, they often converge in backend development. Missteps in SQL can lead to performance bottlenecks, data inconsistency, and security vulnerabilities. In this blog post, we will discuss the top SQL mistakes Java developers make and how to avoid them.
1. Not Using Prepared Statements
What are Prepared Statements?
Prepared statements allow you to execute the same SQL query multiple times with different parameters securely. They help prevent SQL injection attacks and often improve performance.
Why It Matters
Using prepared statements ensures that your SQL queries are safe from malicious input, which is critical in today’s security-conscious environment. Additionally, they can be precompiled, leading to faster execution for repetitive queries.
Example Code
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class PreparedStatementExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "root";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, user, password)) {
String sql = "SELECT * FROM users WHERE username = ?";
try (PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, "Alice");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
System.out.println("User: " + rs.getString("username"));
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
In this example, the ?
is a placeholder that gets replaced by the user's input safely. If you use concatenated strings to create queries, you risk SQL injections.
2. Ignoring Connection Pooling
What is Connection Pooling?
Connection pooling is a technique used to maintain a pool of database connections, which can be reused by applications instead of opening a new connection every time.
Why It Matters
Creating a new database connection for every request is costly in terms of performance. Connection pooling improves response times and resource utilization.
Example Code
import javax.sql.DataSource;
import org.apache.commons.dbcp2.BasicDataSource;
public class ConnectionPoolingExample {
public static void main(String[] args) {
BasicDataSource ds = new BasicDataSource();
ds.setUrl("jdbc:mysql://localhost:3306/mydb");
ds.setUsername("root");
ds.setPassword("password");
try (Connection conn = ds.getConnection()) {
// Use the connection ...
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Commentary
In this code snippet, we utilize Apache Commons DBCP to create a data source with pooling capabilities. This provides a significant performance boost in high-load scenarios.
3. Poor Error Handling
The Importance of Error Handling
SQL queries can fail for various reasons, including connection issues, syntax errors, or data integrity violations.
Why It Matters
Robust error handling allows your application to respond gracefully to failures instead of crashing unexpectedly, leading to a better user experience.
Example Code
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class ErrorHandlingExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "root";
String password = "wrongPassword";
try (Connection conn = DriverManager.getConnection(url, user, password)) {
// Perform database operations
} catch (SQLException e) {
System.err.println("Database operation failed: " + e.getMessage());
}
}
}
Commentary
The example above shows basic error handling. Notice how it captures any SQL exception and prints an error message. You should always log these errors for troubleshooting.
4. Neglecting Transaction Management
What are Transactions?
A transaction is a sequence of operations performed as a single logical unit of work. It ensures data integrity and consistency.
Why It Matters
Neglecting transaction management can lead to partial updates in your database, causing data inconsistency.
Example Code
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class TransactionManagementExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "root";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, user, password)) {
conn.setAutoCommit(false); // Start transaction
// Perform multiple database operations
// if all operations succeed
conn.commit(); // Commit transaction
} catch (SQLException e) {
System.err.println("Transaction failed: " + e.getMessage());
// rollback if transaction fails
try { conn.rollback(); } catch (SQLException rollbackEx) {
System.err.println("Rollback failed: " + rollbackEx.getMessage());
}
}
}
}
Commentary
In the code snippet above, we manually manage the transaction. If any operation fails, we roll back to maintain data integrity. Always consider transaction management in your applications.
5. Overusing SELECT *
What is SELECT *?
SELECT * retrieves all columns from a database table.
Why It Matters
Using SELECT * can slow down your queries, especially with large datasets, and makes your application less explicit about what data it needs.
Example Code
Instead of:
SELECT * FROM users;
Use:
SELECT username, email FROM users;
Commentary
By specifying exactly which columns you want, you reduce data transfer between the application and database, leading to better performance.
6. Forgetting to Close Resources
The Importance of Closing Resources
Database connections, statements, and result sets consume resources. Not closing them can lead to memory leaks and exhausted database connections.
Why It Matters
Properly closing resources ensures efficient resource management in your application.
Example Code
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class CloseResourcesExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "root";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement("SELECT * FROM users");
ResultSet rs = pstmt.executeQuery()) {
while (rs.next()) {
System.out.println("User: " + rs.getString("username"));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Commentary
Using a "try with resources" statement ensures that all resources are closed automatically at the end of the block, reducing the risk of leaks.
To Wrap Things Up
SQL is an essential skill for Java developers, but common pitfalls can hinder development. By avoiding these top SQL mistakes, you can write more efficient, secure, and maintainable database interactions.
Key Takeaways
- Use Prepared Statements to prevent SQL injection.
- Implement Connection Pooling for improved performance.
- Handle Errors Gracefully to enhance user experience.
- Manage Transactions properly for data integrity.
- Be Specific in SELECT Queries to optimize performance.
- Always Close Resources to avoid leaks.
For Java developers looking to deepen their understanding of SQL, consider reading more about SQL Best Practices or consult the official Java JDBC documentation for further insight.
Remember, mastering SQL is not just about learning syntax—it's about understanding how to apply it effectively and securely in your Java applications. Happy coding!