Eliminating Weak Links: Scanning Vulnerabilities in DevSecOps
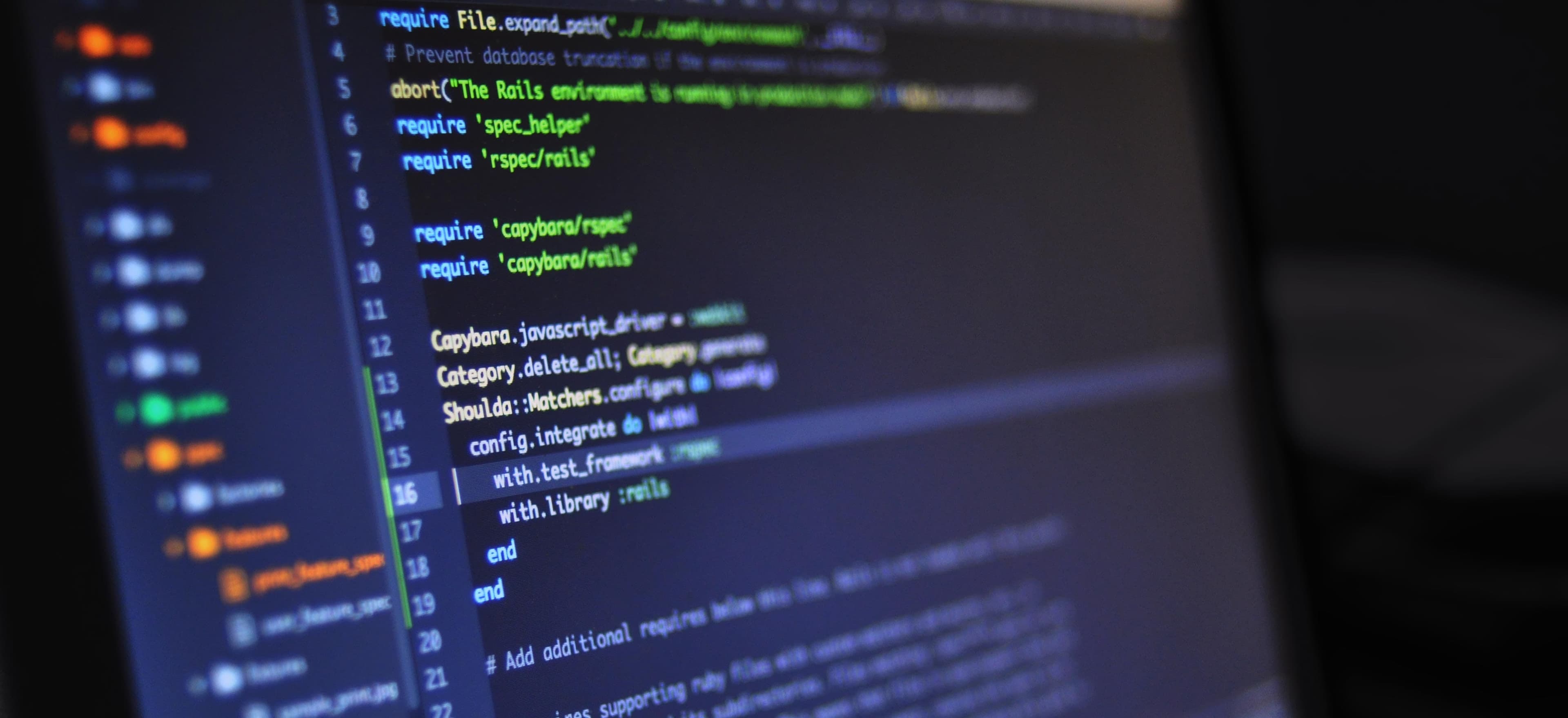
- Published on
Eliminating Weak Links: Scanning Vulnerabilities in DevSecOps
In today's fast-paced software development landscape, security cannot be an afterthought. With more organizations adopting DevSecOps practices, it's essential to integrate security throughout the software development lifecycle (SDLC). This blog post aims to explore how vulnerability scanning plays a crucial role in DevSecOps and how developers can implement it effectively.
Understanding DevSecOps
DevSecOps is the practice of integrating security measures into the DevOps pipeline. It promotes a culture where security is considered everyone's responsibility, not just the security team. In a typical DevSecOps model, security checks are embedded into every stage of development, from code writing to production deployment.
Why Vulnerability Scanning is Vital
- Early Detection: Identifying vulnerabilities early in the development lifecycle can save time and resources in the long run.
- Continuous Improvement: Automated scans allow for continuous monitoring, enabling teams to resolve vulnerabilities promptly.
- Regulatory Compliance: Many industries have strict compliance requirements; scanning for vulnerabilities ensures adherence to these standards.
Key Vulnerability Scanning Techniques
1. Static Application Security Testing (SAST)
Static Application Security Testing involves scanning the source code without executing it to find security vulnerabilities during the coding phase. It analyzes the codebase for common vulnerabilities, such as SQL injection or cross-site scripting (XSS).
Example of SAST Implementation in Java
Consider a simple Java code snippet that reads user input:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class UserData {
public boolean addUser(String username, String password) {
String query = "INSERT INTO users (username, password) VALUES (?, ?)";
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "pass");
PreparedStatement pstmt = conn.prepareStatement(query)) {
pstmt.setString(1, username); // Safe way to insert user input
pstmt.setString(2, password);
pstmt.executeUpdate();
return true;
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
}
Commentary on SAST
The above code uses a PreparedStatement
, which helps mitigate SQL injection risks. By using parameterized queries, you ensure that user inputs do not interfere with SQL commands. Implementing SAST can help identify structures like this in your code and emphasize best practices.
2. Dynamic Application Security Testing (DAST)
Dynamic Application Security Testing evaluates a running application by simulating attacks and scanning for vulnerabilities during execution. DAST tools assess inputs and outputs without requiring access to source code.
Using DAST Tools
Popular DAST tools include:
- OWASP ZAP
- Burp Suite
- Qualys
Integrating a DAST tool into your CI/CD pipeline enables security testing to happen automatically whenever new code is deployed, further instilling a security-first approach.
3. Software Composition Analysis (SCA)
Software Composition Analysis helps identify vulnerabilities in open-source libraries used in your applications. With the popularity of third-party libraries, keeping track of their vulnerabilities is essential.
Example Using an SCA Tool
Tools like Snyk and Nexus Vulnerability Scanner allow developers to scan their project dependencies easily. For example, after adding a new library to your Maven project, you can run:
mvn dependency:tree
Then use the SCA tool to check for vulnerabilities in the libraries listed.
4. Container Security Scanning
In modern development, Docker containers encapsulate applications and their dependencies. Hence, it's crucial to scan containers for known vulnerabilities before deployment.
Container Scanning Example
Using Clair
, you can scan Docker images. Here's a command line to scan an image:
clair-scanner --ip <server-ip> <registry/image:tag>
Commentary on Container Scanning
Container scanning ensures that security vulnerabilities are not introduced during the containerization process. By implementing this practice early, organizations can significantly reduce their risk exposure.
Integrating Scanning into CI/CD Pipelines
To maximize the benefits of vulnerability scanning, it’s crucial to integrate these tools into your CI/CD pipeline. Most CI/CD platforms offer an array of plugins and integrations for scanning tools.
Jenkins Example
Here's how to integrate a SAST tool (e.g., SonarQube) into a Jenkins pipeline:
pipeline {
agent any
stages {
stage('Code Analysis') {
steps {
script {
sh 'mvn clean install sonar:sonar -Dsonar.host.url=http://<sonar-server>:9000 -Dsonar.projectKey=your-project'
}
}
}
}
}
Commentary on Continuous Scanning
Incorporating scanning into your CI/CD pipeline allows for automated checks against production-ready code. Doing so elevates your code's quality and reduces the chances of vulnerabilities making it into production.
Best Practices for Vulnerability Scanning
- Automate as Much as Possible: Automate your scans to ensure regular and timely checks.
- Educate Your Team: Make sure all team members understand security best practices, as vulnerabilities often stem from human error.
- Prioritize Findings: Not all vulnerabilities are created equal. Focus on those that present an actual risk to your application and organization.
- Incorporate Manual Testing: While automated tools are essential, manual testing helps catch vulnerabilities that automated tools may overlook.
The Last Word
Security is a shared responsibility, and vulnerability scanning is an essential component of a successful DevSecOps strategy. Employing effective scanning techniques—ranging from SAST and DAST to SCA and container security—can eliminate weak links in your software development process. Moreover, integrating these practices into your CI/CD pipelines ensures that security checks are continuous and proactive.
By making security a priority, organizations not only protect their applications but also boost stakeholder confidence. It's crucial to stay informed about the latest tools and vulnerabilities and continuously evolve your security practices.
For further reading, consider the following resources:
- OWASP DevSecOps
- DevSecOps Guide
Taking proactive measures today can protect your application from the threats of tomorrow. Let’s start eliminating those weak links!
Checkout our other articles