Navigating Actor Conflicts in Akka for Seamless Collaboration
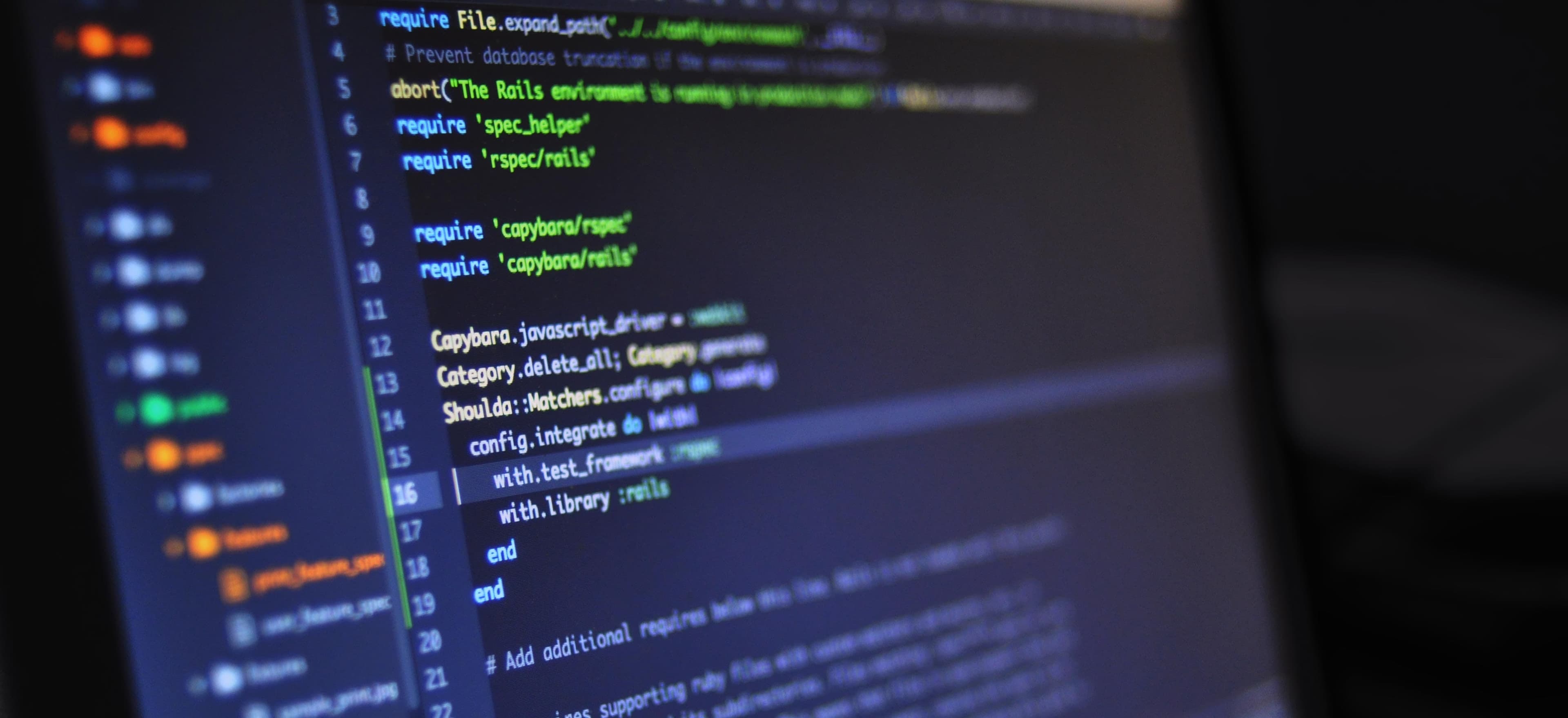
- Published on
Navigating Actor Conflicts in Akka for Seamless Collaboration
In the world of concurrent programming, managing communication and collaboration between multiple components is crucial. One of the most powerful tools available for this purpose in the Java ecosystem is Akka. Akka, built on the Actor model, allows developers to create highly concurrent, distributed, and fault-tolerant applications with ease. However, handling actor conflicts can be a daunting task, particularly in systems with complex interactions. This blog post explores strategies for managing actor conflicts in Akka smoothly.
Understanding the Actor Model
Before diving into conflict resolution, let's revisit the Actor model briefly.
In the Actor model:
- Actors are fundamental units of computation.
- Each actor encapsulates state behavior and communicates through asynchronous messages.
- Actors determine how to respond to incoming messages and can create new actors for handling additional work.
This model brings many benefits, like improved scalability and resilience. However, it can also lead to concurrency-related complications, especially when multiple actors try to access shared resources.
Common Sources of Actor Conflicts
Conflicts in Akka arise from a variety of sources. Understanding these sources can help mitigate potential issues:
-
Shared State: When multiple actors need to read or write to a shared state, conflicts can occur, leading to race conditions and inconsistent data.
-
Message Ordering: Actors process messages asynchronously. Therefore, the order of message arrival can lead to unexpected behavior if not properly managed.
-
Failure Scenarios: Actors can fail independently, affecting others attempting to interact with them.
-
Blocking Calls: If an actor makes a blocking call within its behavior, it can lead to deadlocks or performance bottlenecks.
Strategies for Resolving Actor Conflicts
1. Embrace Immutability
One of the most effective ways to avoid conflicts is to leverage immutability. In Akka, the state should not change after its creation. Instead of modifying existing objects, create new ones.
public class BankAccountActor extends AbstractActor {
private final BigDecimal balance;
public BankAccountActor(BigDecimal initialBalance) {
this.balance = initialBalance;
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(Deposit.class, this::onDeposit)
.match(Withdraw.class, this::onWithdraw)
.build();
}
private void onDeposit(Deposit deposit) {
// Creating a new state instead of mutating the existing one
BigDecimal newBalance = this.balance.add(deposit.amount);
getContext().become(new BankAccountActor(newBalance));
}
private void onWithdraw(Withdraw withdraw) {
if (this.balance.compareTo(withdraw.amount) < 0) {
getSender().tell(new InsufficientFunds(), getSelf());
} else {
// Creating a new instance with the updated balance
BigDecimal newBalance = this.balance.subtract(withdraw.amount);
getContext().become(new BankAccountActor(newBalance));
}
}
}
Why Immutability Matters:
- Each actor has a clear route of state change, improving predictability.
- Reduces unintended side effects and simplifies reasoning about state transitions.
2. Utilize Message Ordering with FIFO Mailboxes
To deal with message ordering, Akka provides mailboxes, allowing developers to dictate how messages are processed. Using a FIFO (First-In-First-Out) mailbox ensures that messages are processed in the order they arrive.
public class MyActor extends AbstractActor {
@Override
public void preStart() {
// Configure a FIFO mailbox
getContext().setMailbox(new PriorityMailbox(
new PriorityComparator(),
otherConfig
));
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(SomeMessage.class, this::handle)
.build();
}
private void handle(SomeMessage msg) {
// processing logic here
}
}
Why Prioritize Mailbox Ordering:
- Ensures that critical messages are processed in the order they are received which is vital in workflows that depend on the sequence of operations.
3. Supervision and Monitoring
In a distributed system, actors can crash. Using Akka's supervision strategies, you can control how your application reacts to such failures. For instance, you can use a Restart strategy that allows actors to restart without compromising the entire application's integrity.
public class SupervisorActor extends AbstractActor {
// Define supervision strategy
@Override
public SupervisorStrategy supervisorStrategy() {
return new OneForOneStrategy(
10, Duration.create("1 minute"),
DeciderBuilder
.match(ArithmeticException.class, e -> SupervisorStrategy.restart())
.match(Exception.class, e -> SupervisorStrategy.resume())
.build()
);
}
@Override
public Receive createReceive() {
return receiveBuilder().match(ChildActor.class, this::createChild).build();
}
private void createChild(ChildActor actor) {
getContext().actorOf(Props.create(ChildActor.class));
}
}
Why Supervision is Critical:
- Enables resilience in the face of errors.
- Allows control over actor lifecycle, leading to a more robust application.
4. Leverage the Akka Persistence Plugin
When dealing with stateful actors, you might consider using Akka Persistence. This plugin allows actors to recover their state after a failure or restart.
public class PersistentBankAccountActor extends AbstractPersistentActor {
private BigDecimal balance = BigDecimal.ZERO;
@Override
public Receive createReceiveRecover() {
return receiveBuilder()
.match(BalanceUpdated.class, this::updateBalance)
.build();
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(Deposit.class, this::handleDeposit)
.match(Withdraw.class, this::handleWithdraw)
.build();
}
private void handleDeposit(Deposit deposit) {
persist(new BalanceUpdated(deposit.amount), evt -> {
updateBalance(evt);
});
}
private void updateBalance(BalanceUpdated evt) {
balance = balance.add(evt.amount);
}
}
Why Akka Persistence is Valuable:
- Simplifies state recovery.
- Helps manage the complexity of concurrent actors by ensuring state consistency.
5. Testing and Monitoring
Finally, thorough testing is essential. Akka provides tools like Akka TestKit for testing actors. Monitoring your actors through tools like Akka Monitoring (with Lightbend Telemetry) or Prometheus can give insights into potential conflicts.
- Akka Documentation for more on actor testing.
- Prometheus for monitoring purposes.
The Bottom Line
Actor conflicts in Akka, if left unmanaged, can result in messy bugs and unpredictable behavior. By embracing strategies such as immutability, messaging order management, supervision, and persistence, you can navigate these conflicts effectively. Whether you're building a microservices architecture or scaling an existing application, Akka's robust features can help ensure seamless collaboration between actors.
As you continue to build your proficiency with Akka, consider these methodologies not just as obstacles to overcome but as integral parts of the design that can lead to more resilient and efficient applications.
Do you have any insights or experiences dealing with actor conflicts in Akka? Share your thoughts in the comments below!
Checkout our other articles