Mastering Memory Leaks: Secrets to HProf Analysis
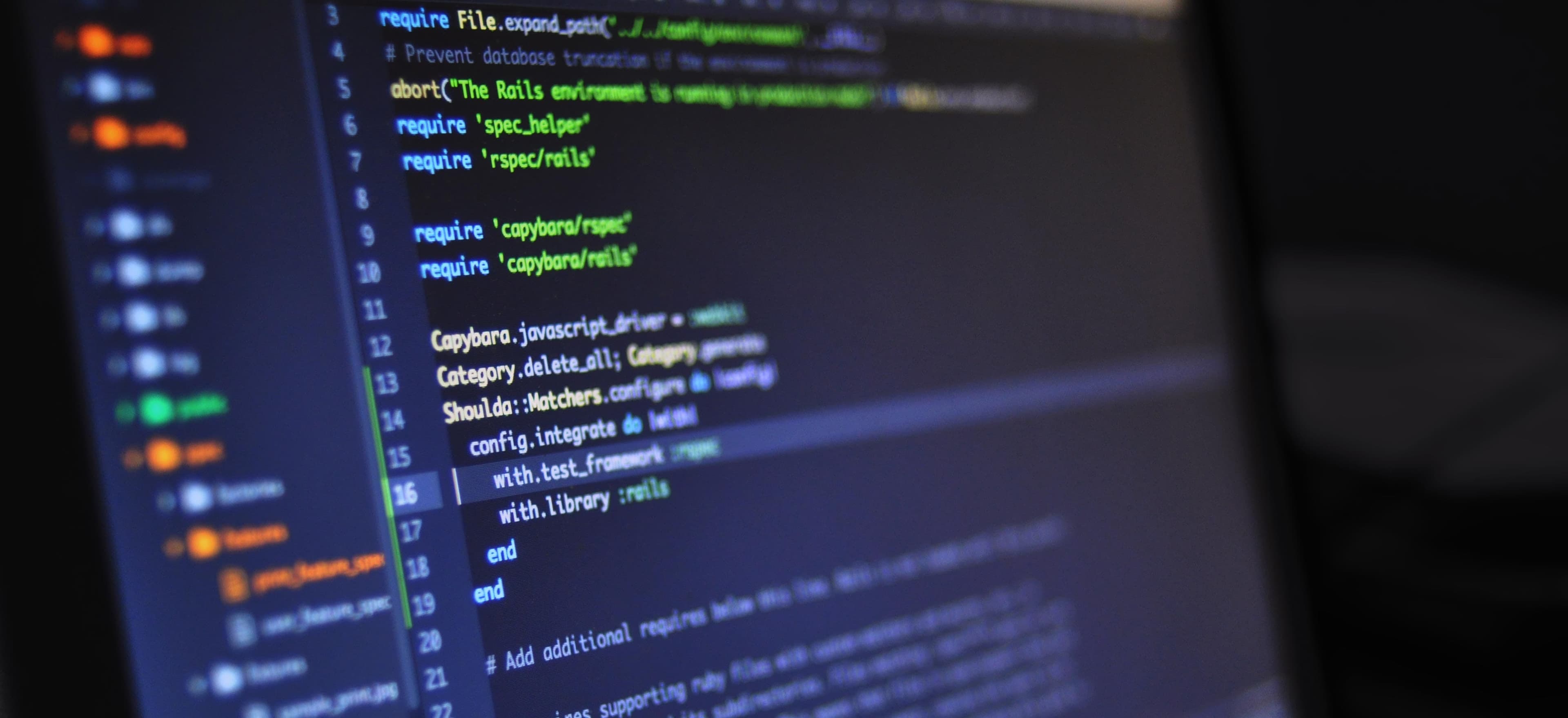
- Published on
Mastering Memory Leaks: Secrets to HProf Analysis
Memory management is one of the most critical aspects of software development, particularly in Java. An effective understanding of memory leaks and the tools available to analyze them can significantly improve application performance and reliability. In this blog post, we will dive into memory leaks in Java, exploring how to identify and analyze them using HProf, a profiling tool that provides insight into memory consumption.
What Are Memory Leaks?
Definition
A memory leak in Java occurs when an application inadvertently holds references to objects that are no longer needed, preventing the Java Garbage Collector (GC) from reclaiming that memory. Over time, memory leaks can lead to increased memory consumption, slow performance, and eventually, application crashes.
Symptoms
Typical symptoms include:
- Increasing memory usage over time.
- Slower response times.
- Frequent "Out of Memory" errors during normal operation.
Common Causes of Memory Leaks
Before delving into analysis techniques, it's essential to understand common causes of memory leaks in Java applications:
- Static Collections: Storing references in static collections prevents the objects from being garbage-collected.
- Listeners and Callbacks: Not unregistering listeners can keep objects in memory when they're no longer needed.
- Thread Locals: Misusing ThreadLocal may cause instances to pile up, especially in long-lived threads.
- Unclosed Resources: Failing to close resources like database connections can lead to leaks.
How to Detect Memory Leaks
Detecting memory leaks involves monitoring the application’s memory usage over time. Here are a few strategies to help identify leaks:
- Use Profiling Tools: Java profilers such as VisualVM, JProfiler, and HProf can help identify memory usage patterns.
- Analyze Heap Dumps: A heap dump captures the entire memory of the Java Virtual Machine (JVM) at a certain point; analyzing it can reveal objects that are unnecessarily retained.
Why HProf?
HProf is a built-in Java profiler that comes with the JDK. It allows developers to analyze memory usage and track down memory leaks efficiently. HProf generates a heap dump that describes every object on the heap along with references to other objects, making it invaluable for identifying leaks.
Setting Up HProf
To use HProf in your application, you must first enable it. Here’s how to do that:
java -Xrunhprof:heap=sites,yourApp.jar
In the command above:
-Xrunhprof
enables HProf.heap=sites
specifies that you want to include heap site data.yourApp.jar
is the name of your Java application.
Once you've started your application with HProf enabled, it will automatically generate a heap dump when the application terminates. The output file is named something like java.hprof.txt
.
Analyzing HProf Output
After obtaining the HProf output, the next step is to analyze it. The HProf output consists of a detailed breakdown of memory usage.
Key Sections
- Heap Summary: Offers a general overview of memory consumption, including the number of objects and their sizes.
- All Classes: Lists all classes present in the heap along with their instances and memory consumed.
Here is a naming convention for memory analysis through HProf output:
index: 1
class: java.lang.String
instances: 1500
total-size: 120,000
In this example, java.lang.String
occupies a notable amount of memory (120KB) with 1500 instances. The next step is evaluating what holds these references.
Using the Output for Leak Identification
When analyzing the HProf file, it’s crucial to look for the Object Retained Size. Here's a fictive code snippet that illustrates how to do this in a practical scenario:
Example Code
Assume we have a class where we might store user sessions in a static collection:
import java.util.HashMap;
public class SessionManager {
private static HashMap<String, UserSession> sessions = new HashMap<>();
public static void addSession(String userId, UserSession session) {
sessions.put(userId, session);
}
// Forget to clear sessions after usage
}
In this code, if sessions are never removed after a user logs out, they will accumulate, leading to a memory leak.
Analyzing Evidence from HProf
When we examine the HProf output, if we see UserSession
has hundreds of instances with no corresponding memory release over time, we might confirm a memory leak.
Fixing Memory Leaks
When you identify a leaking object, you will need to ensure that references to it are disposed of correctly. Below, we modify our example to remove sessions based on certain criteria:
public static void removeSession(String userId) {
sessions.remove(userId);
}
Make sure to call removeSession
at appropriate times (like when the session has expired or when a user logs out).
Best Practices to Avoid Memory Leaks
- Avoid Static References: Unless absolutely necessary, refrain from holding onto strong references in static fields.
- Cleanup Code: Always implement cleanup logic in components that maintain state, such as listeners and database connections.
- Weak References: Use
WeakReference
for caches or listeners when appropriate.
A Final Look
Memory leaks can plague Java applications, but understanding how to analyze and address these issues is key to robust performance. Tools like HProf provide a powerful means to investigate memory usage, revealing pain points in your applications.
By following best practices, regularly analyzing your application's memory, and utilizing profiling tools, you can keep memory leaks at bay and ensure your applications perform at their best. For deeper insights, consider Oracle's Memory Management documentation for further refining your understanding.
Embrace these techniques and transform your Java applications into models of efficiency. Happy coding!
Checkout our other articles