Tackling Memory Leaks in OpenJPA: A Developer's Guide
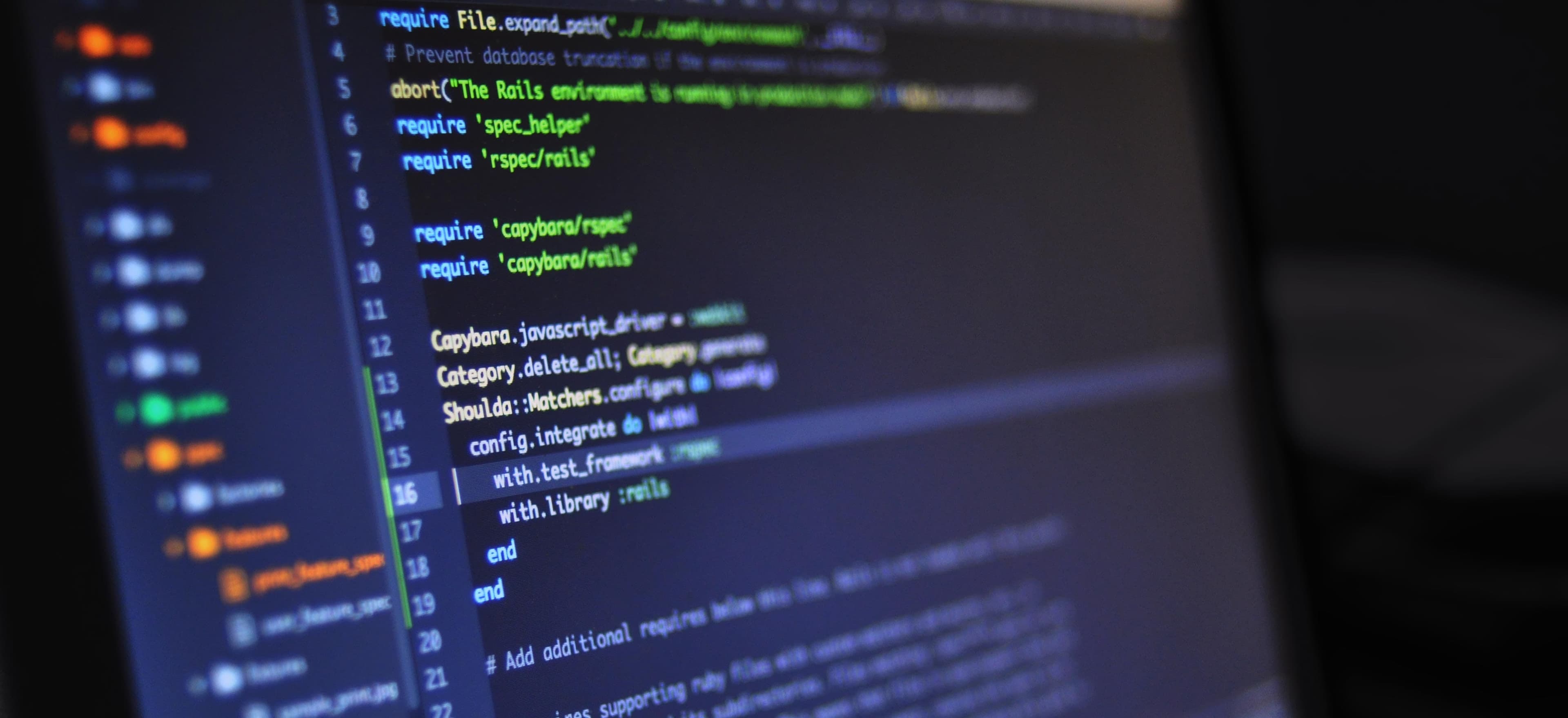
- Published on
Tackling Memory Leaks in OpenJPA: A Developer's Guide
Java Persistence API (JPA) is a powerful specification for working with database operations in Java applications. OpenJPA, an implementation of JPA, offers great flexibility, but like any robust framework, it comes with its own set of challenges. One prominent issue developers may face is memory leaks. In this blog post, we will explore the causes of memory leaks in OpenJPA, techniques for identifying them, and strategies for avoiding them.
Understanding Memory Leaks in OpenJPA
Memory leaks happen when an application retains references to objects that are no longer required, preventing the Java garbage collector from freeing up memory. In an OpenJPA context, various factors can lead to memory leaks.
- Uncleared Entity Managers: If Entity Managers are not closed properly, they can retain references to entities.
- Poorly Managed Caches: OpenJPA uses caching to enhance performance, but improper configuration can lead to leaks.
- Listener References: Event listeners can hold on to entities that should otherwise be collected by the garbage collector.
What Are the Symptoms?
Detecting memory leaks can be tricky. However, some typical symptoms include:
- Increased memory usage over time.
- OutOfMemoryError exceptions on the server.
- Gradual performance degradation.
Strategies to Identify Memory Leaks
1. Use a Memory Profiler
Tools like VisualVM or YourKit can help visualize memory usage in your application. By analyzing heap dumps, you can identify which objects are consuming excessive memory.
// For example, in VisualVM you would see a graph like this:
Memory Usage over Time
This can help confirm which object types are not being cleared correctly.
2. Enable OpenJPA Logging
By turning on OpenJPA logging, you can gather insights into your persistence context and cache usage. Set the logging level to DEBUG in the persistence.xml
.
<persistence-unit name="myPersistenceUnit">
<properties>
<property name="openjpa.Log" value="DefaultLevel=DEBUG"/>
</properties>
</persistence-unit>
3. Analyze JVM Memory with jconsole
JConsole, included with the JDK, can also diagnose memory leak issues. Connect to your application and monitor memory consumption using the Memory tab.
!JConsole Memory Tab
Common Causes of Memory Leaks in OpenJPA
1. Unmanaged Entity Managers
It is vital to ensure Entity Managers are closed after use. Failing to do this often leads to Word Count leakage. Use try-with-resources for automatic closure.
EntityManagerFactory emf = Persistence.createEntityManagerFactory("myPersistenceUnit");
try (EntityManager em = emf.createEntityManager()) {
// Perform operations
} catch (Exception e) {
// Handle exceptions
}
The try-with-resources statement is crucial because it guarantees the closure of the EntityManager even in the case of exceptions.
2. Cache Misconfiguration
OpenJPA's cache is beneficial by storing frequently accessed entities, which can improve performance. Nonetheless, if it is configured improperly, it may cache too many entities.
Solution: Regularly review the cache configuration in persistence.xml
and make sure to set appropriate limits for caching.
<property name="openjpa.jdbc.Schema" value="public"/>
<property name="openjpa.Cache" value="true"/>
<property name="openjpa.Cache.size" value="1000"/> <!-- Adjust the cache size -->
3. Event Listeners
Event listeners need to be managed correctly. They can hold references to tracked entities; if these listeners are not deregistered, this can cause memory leaks.
public class MyEventListener implements EntityListener {
@PersistenceContext
EntityManager em;
@Override
public void afterPersist(Object entity) {
// Process entity
}
}
// Use weak references for listeners
WeakReference<MyEventListener> weakListener = new WeakReference<>(new MyEventListener());
// Ensure to clean up listener references on exit
Weak references allow the garbage collector to clean up resources while you still have a way to handle events when necessary.
Best Practices for Avoiding Memory Leaks
1. Regular Profiling and Monitoring
Establish a routine to profile the application. Running tools regularly will help catch memory leaks before they become a critical issue.
2. Configure JPA Properly
A well-structured persistence.xml
file will reduce the risk of memory leaks:
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence
http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd"
version="2.1">
<persistence-unit name="myPersistenceUnit">
<jta-data-source>jdbc/MyDS</jta-data-source>
<properties>
<property name="openjpa.RuntimeUnenhancedClasses" value="unsupported"/>
<property name="openjpa.flushBeforeQueries" value="true"/>
</properties>
</persistence-unit>
</persistence>
3. Cleanup Operations
Ensure that entity objects are cleared after their use. This will not only help with memory management but also boost application performance.
em.clear(); // This method detaches all entities from the EntityManager
4. Understand Object Lifecycles
Educate yourself on how JPA manages objects through various states: transient, managed, detached, and removed. Understanding this flow is essential in ensuring you mitigate memory leaks effectively.
My Closing Thoughts on the Matter
Memory leaks can severely impact the performance of an OpenJPA-based application, leading to OutOfMemoryError and degraded user experience. By understanding the causes, employing the right tools for identification, and implementing best practices, developers can mitigate the risks of memory leaks effectively.
Memory management requires vigilance and understanding, but with these strategies, you can create a more robust OpenJPA application.
If you're interested in further exploring performance optimizations in OpenJPA, consider reading Optimizing Performance with OpenJPA where you'll find an array of strategies tailored for performance.
Happy coding!
Checkout our other articles