Mastering Java 8: Common Mistakes with Lambda Expressions
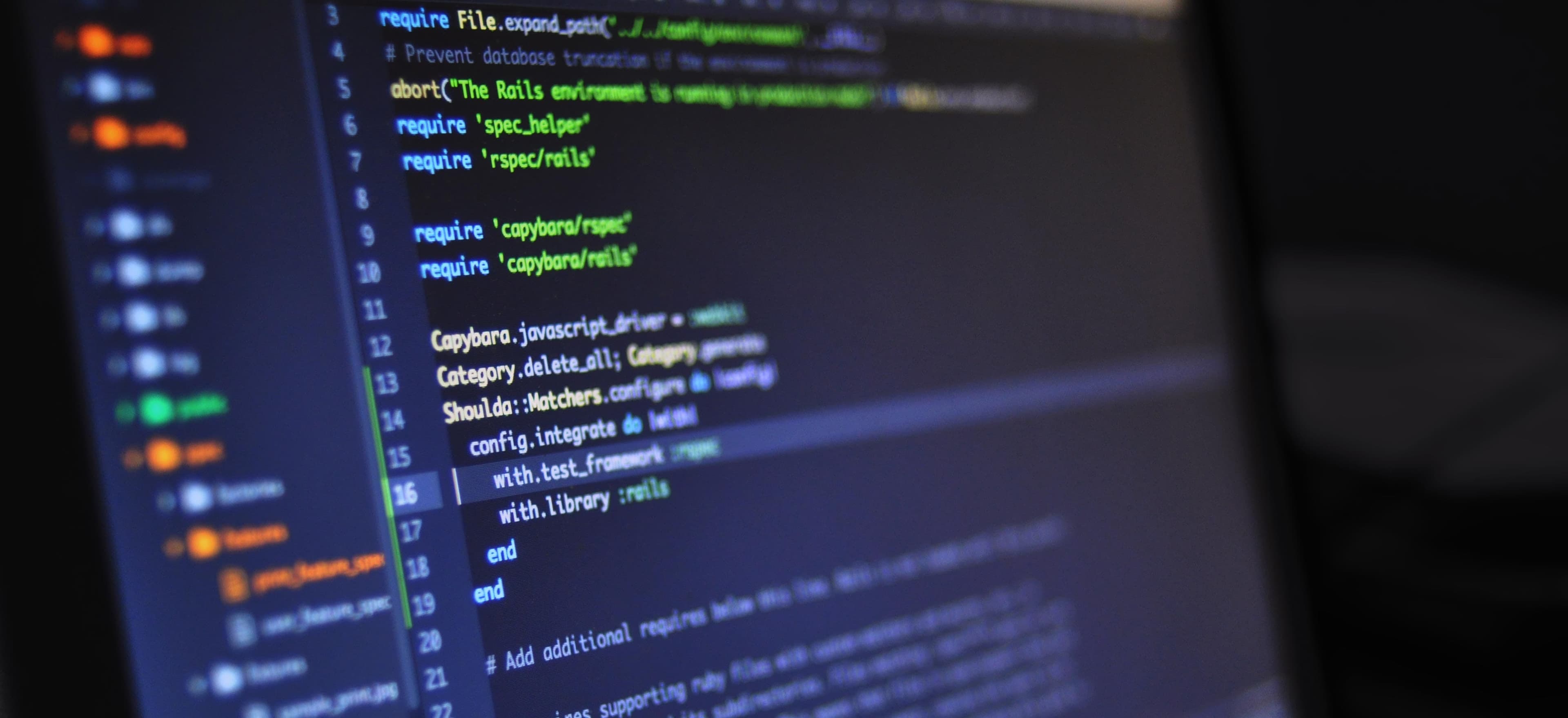
- Published on
Mastering Java 8: Common Mistakes with Lambda Expressions
Java 8 brought significant enhancements to the Java programming language, with one of its most notable features being Lambda expressions. These anonymous functions allow developers to write cleaner, more efficient code. However, as powerful as Lambda expressions are, they can also lead to several common pitfalls for those unfamiliar with this paradigm. In this blog post, we will explore these mistakes, how to avoid them, and best practices for leveraging Lambda expressions effectively in your Java applications.
Understanding Lambda Expressions
Before diving into common mistakes, let's briefly recap what Lambda expressions are. A Lambda expression in Java is a concise way to represent an instance of a functional interface (an interface with a single abstract method). The syntax is straightforward:
(parameters) -> expression
For example, consider the following Lambda expression that adds two numbers:
(int a, int b) -> a + b
Lambdas are often used with the Java Stream API, enabling operations on collections in a more readable manner.
Mistake 1: Forgetting the Type Inference
One of the beauties of Lambda expressions is type inference. Java knows the context, and therefore, does not require explicit type declarations for the parameters.
Incorrect Example:
// Explicit types are redundant here
BinaryOperator<Integer> add = (Integer x, Integer y) -> x + y;
Correct Example:
BinaryOperator<Integer> add = (x, y) -> x + y;
Why This Matters: Keeping your code concise improves readability. Trust the compiler to infer types where appropriate.
Mistake 2: Misunderstanding Scope and this
Reference
When using Lambda expressions, the this
reference behaves differently. Unlike anonymous classes, which create their own scope, Lambdas capture the this
reference from the enclosing class.
Incorrect Example:
public class Outer {
private String name = "Outer";
public void test() {
// This refers to Outer.this.name
Runnable r = () -> System.out.println(this.name);
r.run();
}
}
Correct Example:
If you wanted the this
reference of the inner class or method, consider using a nested class instead if it’s crucial:
public class Outer {
private String name = "Outer";
public void test() {
class Inner implements Runnable {
private String name = "Inner";
public void run() {
System.out.println(this.name); // Refers to Inner's name
}
}
new Inner().run();
}
}
Why This Matters: Understanding the this
reference can prevent confusion and errors in your program. It’s imperative to know which scope you are encapsulating.
Mistake 3: Using Lambdas Where They Don’t Fit
Not all scenarios are suited for Lambda expressions. Sometimes, a traditional implementation is clearer, especially when handling complex logic.
Incorrect Example:
List<String> names = Arrays.asList("John", "Jane", "Jack");
// Complex logic tucked into a Lambda
names.sort((a, b) -> {
if (a.length() == b.length()) return 0;
return a.length() < b.length() ? -1 : 1;
});
Correct Example:
Using a method reference can be clearer and more maintainable:
names.sort(Comparator.comparingInt(String::length));
Why This Matters: While it’s tempting to use Lambda expressions for every case, readability and maintainability should take precedence. Sometimes, clear code is more valuable than concise code.
Mistake 4: Not Handling Exceptions Properly
Lambdas do not permit checked exceptions unless declared. If you are using a Lambda where an exception might arise, you need to handle it properly.
Incorrect Example:
Runnable runnable = () -> {
try {
throw new IOException("Error occurred");
} catch (IOException e) {
e.printStackTrace();
}
};
Correct Example:
With functional interfaces that can throw exceptions, a cleaner approach might be to create a custom functional interface:
@FunctionalInterface
interface ThrowingRunnable {
void run() throws Exception;
}
// Example usage
ThrowingRunnable runnable = () -> {
throw new IOException("Error occurred");
};
try {
runnable.run();
} catch (Exception e) {
e.printStackTrace();
}
Why This Matters: Clean handling of exceptions ensures that your application behaves predictably. Ignoring exceptions can lead to unexpected program crashes.
Mistake 5: Excessive Use of External State
Lambda expressions should ideally be stateless. Relying heavily on mutable external state can lead to tricky bugs and unpredictable behavior.
Incorrect Example:
class Counter {
private int count = 0;
public void increment() {
Runnable r = () -> count++;
// This may lead to race conditions if used in multi-threading context
r.run();
}
}
Correct Example:
It is better to return the result directly:
class Counter {
private int count = 0;
public int increment() {
return ++count; // No external state affected
}
}
Why This Matters: Minimizing mutable state makes Lambdas safer to use, especially in concurrent programming scenarios.
Closing Remarks
Mastering Lambda expressions is essential for any Java developer wishing to leverage the full power of Java 8 and beyond. We covered several common mistakes and their corrections:
- Forget Type Inference: Leverage it for cleaner code.
- Understand
this
: Know the reference scope. - Choose Appropriately: Not every scenario fits well with Lambdas.
- Handle Exceptions: Never omit exception handling.
- Limit External State: Keep Lambdas stateless for predictability.
As you implement Java 8 features, remember to keep these mistakes in mind to enhance code quality and maintainability. For additional context on Lambdas, check out the Official Oracle Documentation on Lambda Expressions and explore deeper insights on Java's Stream API.
With practice and careful attention, you can master Lambda expressions, powering your Java applications to new heights. Happy coding!