Common Pitfalls in Software Application Design and How to Avoid Them
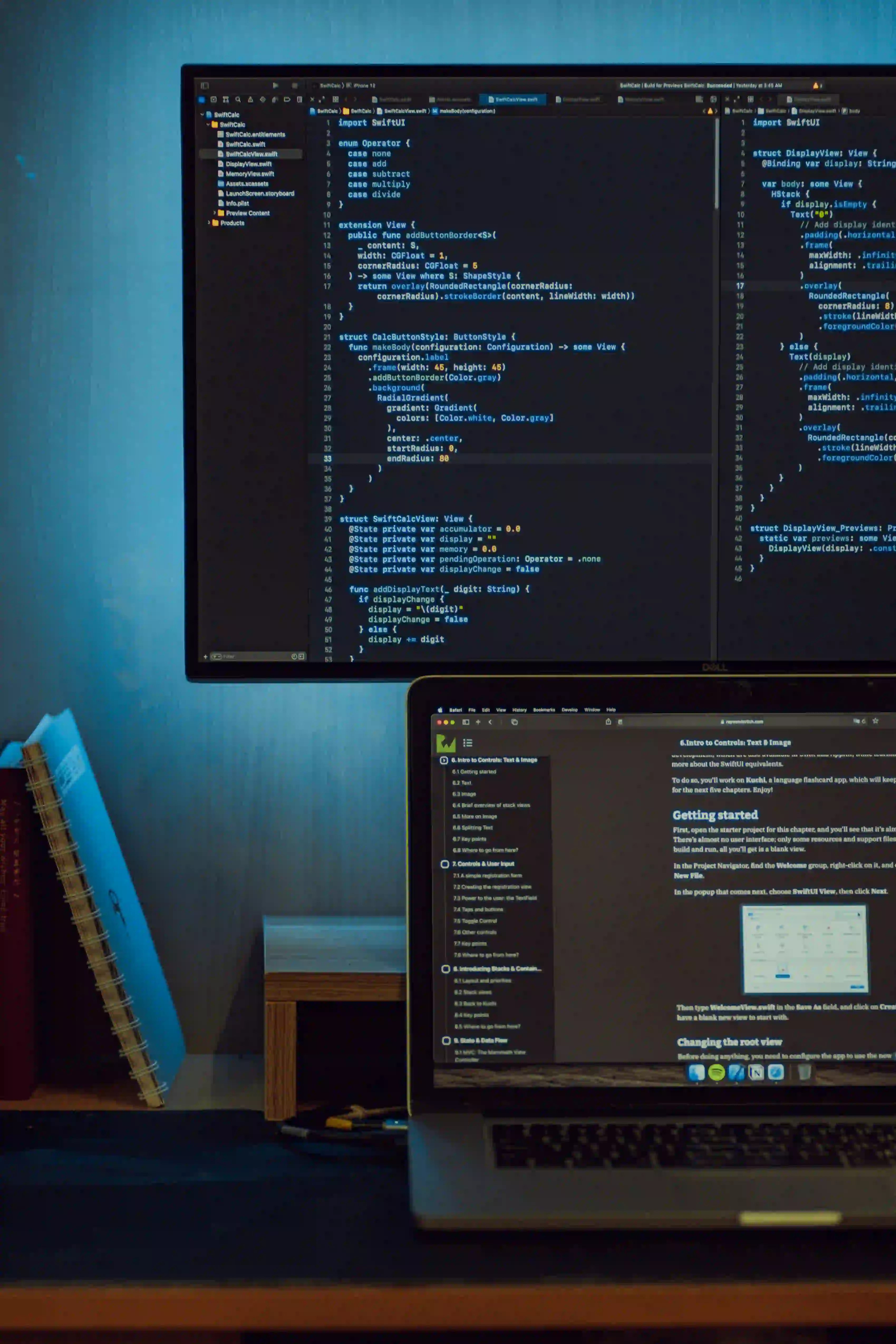
Common Pitfalls in Software Application Design and How to Avoid Them
Software application design is a complex field, often rife with pitfalls that can lead to increased costs, poor user experience, and even project failure. Whether you’re a budding developer or a seasoned professional, understanding these pitfalls—and how to navigate them—can make a significant difference in the success of your projects.
In this blog post, we’ll explore common design pitfalls, why they happen, and ways to avoid them. We’ll also include Java code snippets to illustrate some of the discussed concepts.
1. Lack of User-Centric Design
One of the most significant mistakes in software design is neglecting the end-user. It’s easy to become too focused on technical specifications or system architecture while forgetting for whom you are building the application.
Why It Happens
Often, project stakeholders may not fully understand their users' needs. They might assume what features are necessary without conducting proper research.
Solution: User Research
Conduct user research and usability testing. Utilize methodologies such as surveys, interviews, and A/B testing to identify user preferences and pain points.
Code Example: Simple User Feedback Loop
Here’s a simplistic approach to gather user feedback via a command line application:
import java.util.Scanner;
public class UserFeedback {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please rate your experience from 1 to 5:");
int rating = scanner.nextInt();
switch (rating) {
case 5:
System.out.println("Thank you! We're glad you loved it!");
break;
case 1:
System.out.println("We're sorry to hear that. Please share your feedback:");
scanner.nextLine(); // Consume newline
String feedback = scanner.nextLine();
System.out.println("Your feedback has been recorded: " + feedback);
break;
default:
System.out.println("Thank you for your rating!");
break;
}
scanner.close();
}
}
In the example above, this code helps to gather user experience ratings and even allows for feedback collection for those who rated poorly. For more information on user-centric design, check out NNG's Guide on User Research.
2. Ignoring Scalability
Designing an application without considering scalability can be disastrous. As user demands increase, the application should be able to handle greater loads without performance issues.
Why It Happens
Often, developers focus on immediate functionality and overlook future growth, leading to a patchwork of solutions that can complicate maintenance.
Solution: Design for Scalability
Design your architecture with scalability in mind. A common practice is to use microservices or event-driven architecture to allow components to scale independently.
Code Example: Follow the SOLID Principles
Here’s a Java example demonstrating the Interface Segregation Principle, one of the SOLID principles that fosters scalability:
interface UserService {
void createUser(String name);
}
interface AdminService {
void deleteUser(String userId);
}
class User implements UserService {
public void createUser(String name) {
// Implementation to create user
System.out.println("User " + name + " created.");
}
}
class Admin implements AdminService {
public void deleteUser(String userId) {
// Implementation to delete user
System.out.println("User with ID " + userId + " deleted.");
}
}
Here, separating the User and Admin functionalities allows for different components to scale independently. For additional insights into scalable architectures, visit Martin Fowler’s website for relevant resources.
3. Over-Engineering
In an effort to future-proof applications, developers may over-engineer solutions, adding unnecessary complexity.
Why It Happens
A desire to use the latest technology or desire for perfection can cloud judgment, leading to overly complicated designs.
Solution: Adopt KISS Principle
Keep it simple, stupid (KISS). Focus on the minimum viable product (MVP) that meets core user needs without unnecessary features.
Code Example: Minimalist Design
Here’s an example of a simple calculator application that adheres to minimal design:
public class SimpleCalculator {
public static int add(int a, int b) {
return a + b;
}
public static int subtract(int a, int b) {
return a - b;
}
public static void main(String[] args) {
int sum = add(4, 5);
int difference = subtract(9, 3);
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
}
}
In this case, the calculator includes only essential functionalities—addition and subtraction—demonstrating a minimal approach.
4. Inefficient Communication Among Teams
Software projects often involve cross-functional teams. If communication remains inefficient, it can lead to misunderstandings, misaligned goals, and ultimately project failure.
Why It Happens
Lack of defined processes and tools can hinder effective communication among team members.
Solution: Use Collaborative Tools
Implement chat platforms, project management tools, and regular stand-up meetings to keep all stakeholders aligned.
Code Example: Documentation Best Practices
Use concise comments in your code to promote understanding:
public class Rectangle {
double length;
double width;
// Constructor initializes length and width
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
// Method to calculate area of rectangle
public double calculateArea() {
return length * width; // Area = Length x Width
}
}
Structured comments help clarify the purpose and functionality, ensuring better communication for anyone reviewing the code later.
5. Ignoring Security Fundamentals
With cyber threats constantly evolving, neglecting security in your design could have dire consequences.
Why It Happens
Sometimes the focus on development speed overshadows a thorough review of security protocols.
Solution: Incorporate Security by Design
Make security an integral part of your design process. Use encryption, validate input, and regularly update software dependencies.
Code Example: Input Validation
Java offers mechanisms for validating user inputs to enhance security:
import java.util.Scanner;
public class InputValidator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter your username (only letters):");
String username = scanner.nextLine();
// Validate username
if (!username.matches("[a-zA-Z]+")) {
System.out.println("Invalid username! Only letters are allowed.");
} else {
System.out.println("Username accepted.");
}
scanner.close();
}
}
Input validation helps prevent potential security breaches by ensuring that only acceptable data is processed.
Final Thoughts
Software application design is a multi-faceted process, and being aware of common pitfalls can significantly enhance your approach. Avoiding user-centric design focuses, planning for scalability, resisting the temptation to over-engineer, ensuring effective communication, and embedding security practices are essential steps toward successful software development.
As you continue your journey in software development, remember that learning from existing pitfalls and implementing practical solutions will pave the way for creating robust, user-friendly applications.
Continue honing your skills and don’t hesitate to revisit the principles discussed here when tackling your next project. For more insights on software development, check out Martin Fowler's blog or explore user-centered design methodologies at Nielsen Norman Group.
Happy coding!