Why Decomposed Apps Fail Performance Testing
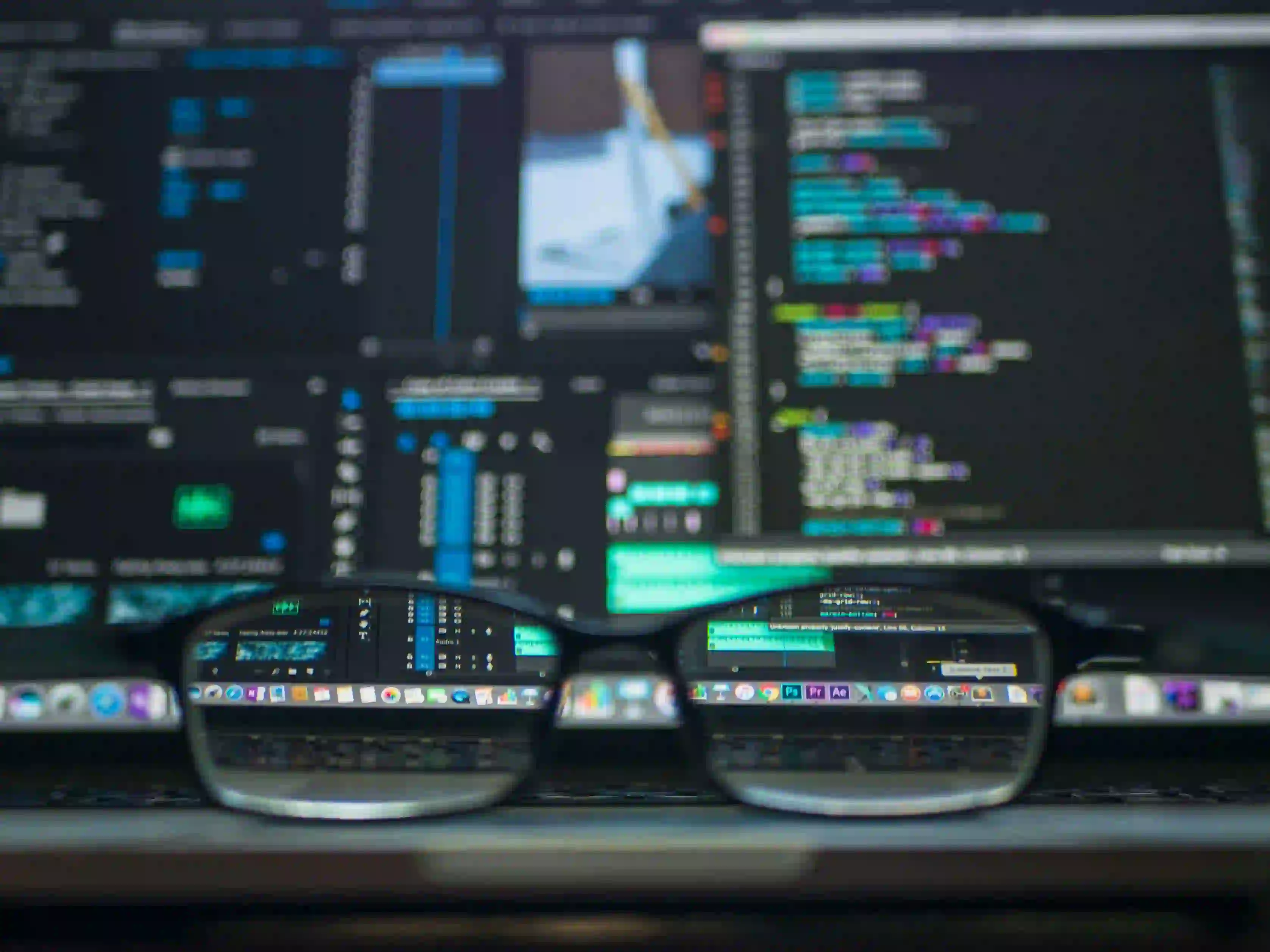
Why Decomposed Apps Fail Performance Testing
In the modern software development landscape, the trend toward building decomposed applications, often referred to as microservices, has gained notable traction. However, despite the architectural advantages of decomposed apps, they often encounter performance failures during testing. Understanding why these failures occur is crucial for developers and quality assurance professionals alike. This blog post delves into the common pitfalls of decomposed applications in performance testing, elucidates the underlying causes, and offers practical solutions for overcoming these challenges.
The Rise of Decomposed Applications
Decomposed applications break down monolithic applications into smaller, independent services. This architectural shift aims to improve scalability, enhance team productivity, and facilitate continuous delivery. Each service can be developed, deployed, and scaled independently, offering flexibility and resilience. However, the very characteristics that make microservices attractive can also lead to vulnerabilities in performance testing.
Common Pitfalls of Decomposed Applications in Performance Testing
1. Increased Latency Due to Network Calls
Decomposed architectures frequently require services to communicate over the network. Each service call introduces latency, especially when relying on synchronous communication patterns.
Why it Matters:
High latency can significantly affect user experience. If one service is slower due to network conditions, the entire application may suffer.
Example:
// A typical REST call in Java
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.getForEntity("http://example.com/api/service", String.class);
In this snippet, if the service being called has high latency, the overall response time for the requesting service inflates. Consider an asynchronous mechanism or a caching strategy to alleviate this issue.
2. Service Dependencies and Bottlenecks
In a microservices architecture, services are interdependent. If one service fails or slows down, it can create a bottleneck for others depending on it.
Why it Matters:
Identifying and managing dependencies is critical, as a bottle-necked service can cascade failures throughout the application.
Example:
// Service A calls Service B
public void callServiceB() {
// Call to service B
String result = serviceBClient.getData();
// Further processing
}
If Service B is under heavy load and cannot respond promptly, Service A will stall. Implementing circuit breakers or flowing requests through message queues can help mitigate these risks.
3. Insufficient Test Scenarios
Performance testing of decomposed applications often falls short when it comes to creating realistic test scenarios. Many tests merely mimic happy paths without considering how services interact under stress.
Why it Matters:
Real-world usage patterns significantly differ from simplistic test scenarios. This discrepancy can lead to false confidence in application performance.
Example:
// Simulating load testing scenario
for (int i = 0; i < 1000; i++) {
callServiceB();
}
This loop tests Service A calling Service B without considering concurrent users or service load. Including diverse scenarios such as spike testing or steady state analysis provides a more accurate representation of service performance.
4. Monitoring Complexity
Monitoring a decomposed application can be complex due to the distributed nature of microservices. Lack of centralized logging and monitoring can obscure performance issues.
Why it Matters:
Without proper visibility, spotting bottlenecks or slow services becomes tremendously difficult.
Example:
Utilizing Spring Cloud Sleuth can help improve the traceability of requests through microservices.
@EnableZuulProxy
@EnableDiscoveryClient
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In this case, adding Sleuth helps in correlating logs from various services, ultimately assisting developers in tracking down performance issues.
5. Inconsistent Resource Management
Microservices often have different resource management requirements. If not properly allocated, some services might be over-provisioned while others are starved of resources.
Why it Matters:
Improper resource allocation can lead to performance degradation, even if the application runs smoothly under nominal load.
Example:
resources:
requests:
memory: "128Mi"
cpu: "500m"
limits:
memory: "256Mi"
cpu: "1"
Configuring resource requests and limits in Kubernetes can optimize performance based on service needs. Monitor each microservice and adjust accordingly to prevent starvation and ensure efficient performance.
Best Practices for Performance Testing of Decomposed Apps
1. Adopt Asynchronous Communication
Utilizing asynchronous communication methods, such as messaging queues, can help mitigate latency issues associated with synchronous calls. Technologies like RabbitMQ or Apache Kafka can be employed to decouple services and reduce wait times.
2. Throttle and Retries with Circuit Breaker Patterns
Encapsulate service calls using the circuit breaker pattern to prevent cascading failures. If a downstream service is slow or failing, the circuit breaker can 'trip' to switch to a fallback method, ensuring application continuity.
3. Implement Chaos Engineering
Integrating chaos engineering practices can expose weaknesses in service performance by intentionally introducing failures and monitoring resultant behaviors. Tools such as Netflix’s Chaos Monkey can assist in this approach.
4. Comprehensive Load Testing
Conduct thorough load testing that includes various scenarios simulating real user behavior and transactions. Use tools such as JMeter or Gatling to analyze multi-service interactions accurately.
5. Centralized Monitoring System
Adopt a centralized logging and monitoring system using tools like Prometheus, Grafana, or ELK Stack. This gives a holistic view of performance metrics, allowing for faster identification of issues across services.
Final Considerations
Decomposed applications offer multiple advantages, but they also bring unique challenges that can lead to performance failures during testing. By understanding the core reasons for these failures and implementing best practices, developers can create more efficient, resilient applications. Ultimately, performance testing should mirror real-world scenarios to ensure that decomposed applications can withstand user demands.
Additional Resources
- Microservices Patterns by Chris Richardson
- Spring Cloud Documentation
- Introduction to Chaos Engineering
By embracing the challenges that come with decomposed applications, teams can improve performance testing strategies, ensuring high-quality software delivery and an optimal user experience.