Common Pitfalls When Implementing Proxy Pattern in Java
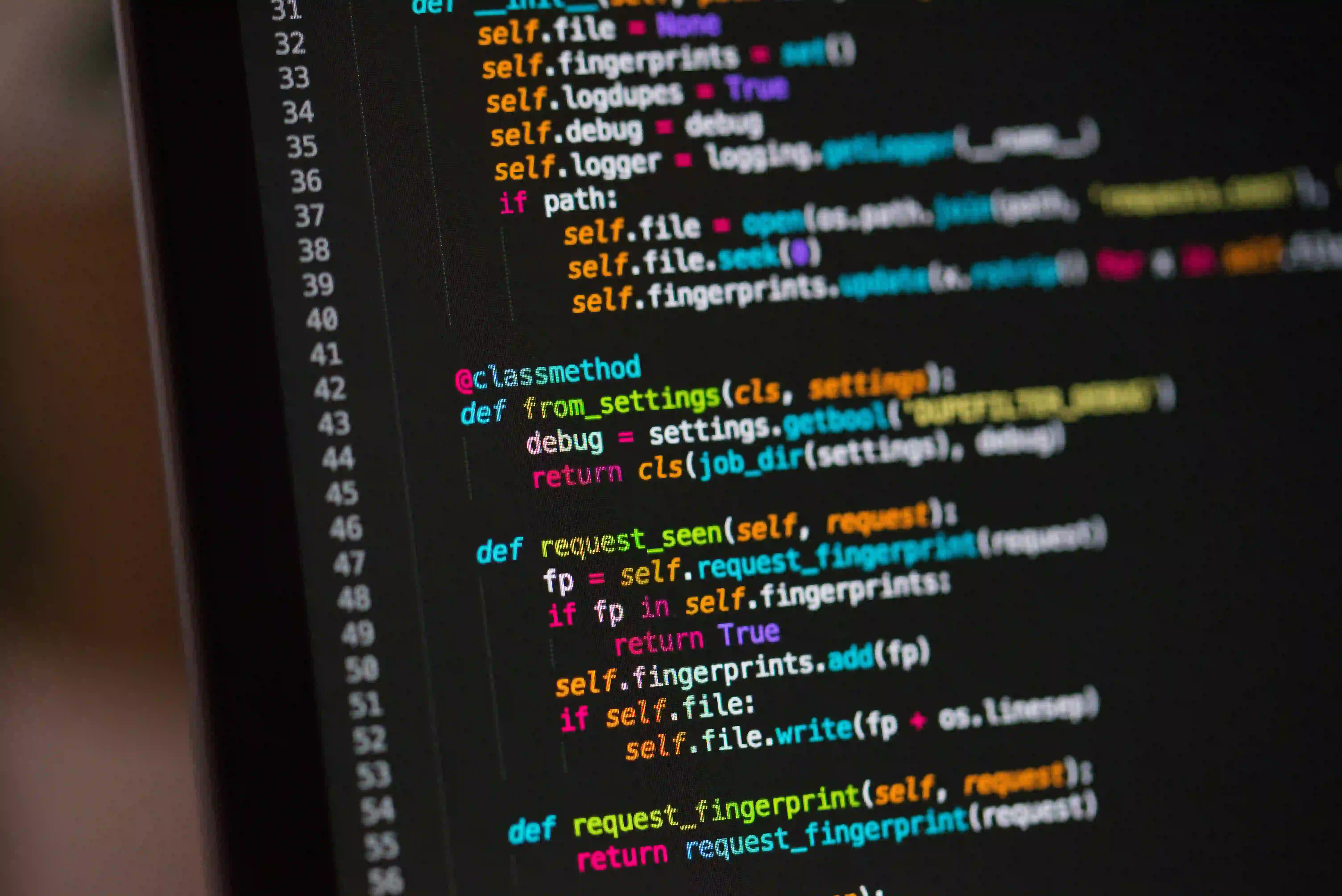
Common Pitfalls When Implementing Proxy Pattern in Java
In the realm of software design, patterns serve as essential building blocks. One such design pattern is the Proxy Pattern, which provides a surrogate or placeholder for another object to control access to it. This pattern can be marvelous for various use-cases—such as lazy initialization, access control, logging, and more. However, it is not without its challenges. In this blog post, we will explore the common pitfalls when implementing the Proxy Pattern in Java, providing insights and code snippets along the way.
What is the Proxy Pattern?
The Proxy Pattern is particularly useful when you want to:
- Control access to an object.
- Perform actions before or after an object is accessed.
- Manage resource-heavy processes by lazy loading them.
Types of Proxy Patterns
- Virtual Proxy: Involves lazy initialization; the real subject is created only when needed.
- Remote Proxy: Represents an object that is in a different address space.
- Protection Proxy: Controls access based on permissions.
Let’s dive into the common pitfalls of implementing the Proxy Pattern in Java.
Common Pitfalls
1. Overcomplicating the Structure
One of the most common mistakes developers make is overengineering the Proxy Pattern implementation by adding unnecessary layers of complexity.
Example:
Imagine trying to use the Proxy Pattern to add logging to each method of a service:
// Real subject
class RealService {
public void performAction() {
System.out.println("Action performed.");
}
}
// Proxy
class ProxyService {
private RealService realService;
public ProxyService() {
this.realService = new RealService();
}
public void performAction() {
// Logging
System.out.println("Before action");
realService.performAction();
System.out.println("After action");
}
}
In this scenario, while the proxy does provide logging, introducing other concerns (like error handling or security) into the ProxyService
can lead to a cumbersome and tightly coupled structure.
Solution:
Keep the proxy class focused on its primary responsibility. If you need logging, consider using an Aspect Oriented Programming (AOP) approach for cleaner separation.
2. Ignoring Performance Implications
Another pitfall is not considering the performance overhead introduced by the proxy. Every method call that goes through the proxy adds layers of method calls, which may not be trivial.
Logical Overhead Example:
Imagine a service that retrieves and processes data:
class DataService {
public String fetchData() {
// Simulating data fetching
return "Data retrieved.";
}
}
class ProxyDataService {
private DataService dataService;
public ProxyDataService() {
this.dataService = new DataService();
}
public String fetchData() {
long startTime = System.nanoTime();
String result = dataService.fetchData();
long endTime = System.nanoTime();
System.out.println("Data fetch took: " + (endTime - startTime) + "ns");
return result;
}
}
While logging the time taken might be useful, if fetchData()
is called frequently, the overhead of capturing timestamps can accumulate.
Solution:
Profile your application and use proxies judiciously. If performance is critical, evaluate whether the overhead introduced by methods in the proxy class is justified.
3. Not Handling Exception Propagation
When using proxy patterns, many developers neglect exception handling. Exceptions occurring in the real subject may need to be caught and handled properly in the proxy.
Example:
Consider a scenario where the real subject might throw an exception:
class UnreliableService {
public String riskyOperation() throws Exception {
// Randomly throw an exception
if (Math.random() > 0.5) {
throw new Exception("Service failed.");
}
return "Service succeeded.";
}
}
class ProxyUnreliableService {
private UnreliableService service;
public ProxyUnreliableService() {
this.service = new UnreliableService();
}
public String riskyOperation() {
try {
return service.riskyOperation();
} catch (Exception e) {
System.err.println("Handled in Proxy: " + e.getMessage());
return null;
}
}
}
By ignoring proper exception propagation, you risk creating a false sense of security, as exceptions may not be adequately handled.
Solution:
Include robust exception handling in the proxy, ensuring that both the proxy and the real subject can report errors effectively.
4. Unintended Side Effects
The proxy can introduce side effects, affecting the behavior of the original subject. For instance, a virtual proxy might lead to repeated initialization if not managed correctly.
Example:
class HeavyResource {
public HeavyResource() {
// Heavy initialization logic
System.out.println("Heavy resource initialized.");
}
}
// Virtual Proxy
class ResourceProxy {
private HeavyResource heavyResource;
public HeavyResource getHeavyResource() {
if (heavyResource == null) {
heavyResource = new HeavyResource(); // Only initialize when needed
}
return heavyResource;
}
}
If getHeavyResource()
is called multiple times, you may expect a single initialization, but if not managed well, it could lead to multiple initializations unintentionally.
Solution:
Ensure that your lazy initialization is correctly implemented to prevent toggling the state of your application inadvertently.
5. Losing Type Information
When using interfaces, you might lose type-specific functionality. Proxy classes should ideally implement the same interface as the real subject.
Example:
interface Service {
void performAction();
}
class RealService implements Service {
public void performAction() {
System.out.println("Real action performed.");
}
}
class ProxyService implements Service {
private RealService realService;
public ProxyService() {
this.realService = new RealService();
}
public void performAction() {
System.out.println("Proxy; before action.");
realService.performAction();
System.out.println("Proxy; after action.");
}
}
If ProxyService
returns only the interface Service
, you ensure that specific features of RealService
will not be accessible unless they are part of the Service
interface.
Solution:
Choose interfaces wisely and ensure that necessary methods are defined in the interface itself.
In Conclusion, Here is What Matters
The Proxy Pattern can serve as a powerful mechanism for managing object access and responsibilities in your Java applications. However, it is fraught with pitfalls that can lead to poor performance, maintenance challenges, or unintended side effects.
By avoiding common mistakes such as overcomplicating your implementation, ignoring performance concerns, neglecting exception handling, and losing type information, you can leverage the full potential of the Proxy Pattern. Keep your implementations clean and focused to promote maintainability and performance.
Additional Resources
- Design Patterns: Elements of Reusable Object-Oriented Software
- Java 8 in Action: Lambdas, Streams, Functional Interfaces
By adhering to best practices and raising awareness about the pitfalls discussed above, you will be well-equipped to employ the Proxy Pattern effectively in your Java endeavors. Happy coding!