Common Pitfalls in JUnit Testing with JBoss Drools
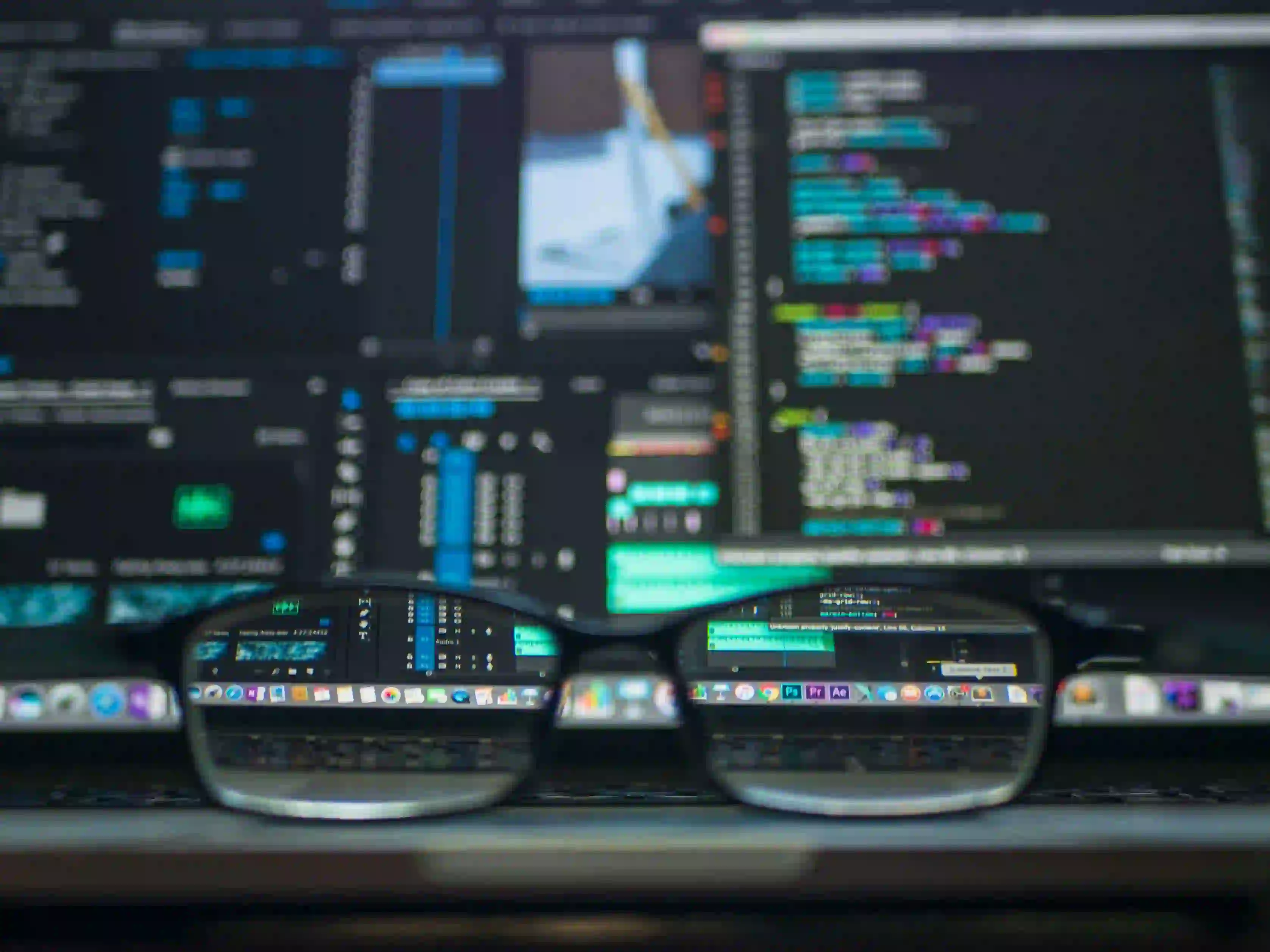
Common Pitfalls in JUnit Testing with JBoss Drools
JUnit testing is an essential part of the development lifecycle, especially when working with complex rule engines like JBoss Drools. Despite its advantages, using JUnit with Drools can present challenges that developers need to navigate carefully. In this blog post, we will explore some common pitfalls you may encounter while writing JUnit tests for JBoss Drools and how to avert them.
Understanding JBoss Drools
JBoss Drools is a Business Rules Management System (BRMS) solution that allows businesses to define, deploy, and manage business rules through a user-friendly interface. It can process complex event patterns and make decisions based on predefined rules. When combined with JUnit tests, it ensures that these rules function as intended.
Pitfall 1: Ignoring the Rule Execution Environment
One of the first pitfalls in JUnit testing with Drools is not thoroughly understanding the rule execution environment. Drools rules rely on a working memory framework, which means that they operate within a certain context.
The Importance of KieSession
When working with rule engines, the KieSession is crucial. It manages the state and allows you to execute rules against facts. Here's an example of how to initialize your KieSession in a JUnit test:
import org.kie.api.KieServices;
import org.kie.api.runtime.KieContainer;
import org.kie.api.runtime.KieSession;
public class DroolsTest {
private KieContainer kieContainer;
private KieSession kieSession;
@Before
public void setUp() {
kieContainer = KieServices.Factory.get().getKieClasspathContainer();
kieSession = kieContainer.newKieSession("sessionName");
}
@Test
public void testBusinessRules() {
// Execute your rules here
}
@After
public void tearDown() {
kieSession.dispose();
}
}
Why this matters:
Initializations within the correct KieSession context help maintain the quality of rule execution. Misconfiguring a session can lead to inconsistent or incorrect results.
Pitfall 2: Neglecting to Clean Up After Tests
Another common issue arises from not releasing resources after tests. Failing to dispose of KieSession can lead to memory leaks and other unwanted behaviors in larger test suites.
Cleaning Up Resources
Ensure that you always call kieSession.dispose()
in an @After
method to free up resources:
@After
public void tearDown() {
kieSession.dispose(); // Clean up resources
}
Pitfall 3: Improper Use of Fact Objects
In Drools, rules operate on facts, which are objects placed in KieSession. A common mistake is not creating or populating these facts correctly.
Example of Adding Facts
Here's how you can correctly add facts to your KieSession:
@Test
public void testRuleWithFacts() {
MyFact fact = new MyFact();
fact.setValue(100);
kieSession.insert(fact); // Add fact to the session
kieSession.fireAllRules(); // Trigger rule execution
}
Why this matters:
Incorrectly configured facts may lead to rules being skipped or fired improperly. Always test with accurate representations of your domain objects.
Pitfall 4: Not Asserting Outcomes
JUnit tests are only as good as the assertions they include. Neglecting to assert the expected outcome after executing Drools rules can lead to failures not being detected, ultimately masking issues in your business logic.
Assertion Documentation
Utilize proper assertions to validate the state of your facts post-rule execution:
@Test
public void testExpectedOutcome() {
MyFact fact = new MyFact();
fact.setValue(100);
kieSession.insert(fact);
kieSession.fireAllRules();
// Check if the expected changes occurred
assertTrue(fact.getValue() > 100);
}
Why this matters:
Assertions provide a safety net to catch any failures in rule logic and ensure that business requirements are met.
Pitfall 5: Testing the Rule Engine Instead of Business Logic
A subtle yet crucial pitfall is testing the Drools engine rather than focusing on the business logic represented by the rules.
Best Practices for Testing
When writing tests, concentrate on the scenarios and expected behaviors rather than the mechanics of the rule engine. A good test clarifies what rules should accomplish rather than how they are processed.
@Test
public void testBusinessRuleBehavior() {
// Arrange: Set up the facts
MyFact fact = new MyFact(50);
kieSession.insert(fact);
// Act: Trigger the rule engine
kieSession.fireAllRules();
// Assert: Check business expectations
assertEquals("Expected outcome not achieved", expectedValue, fact.getCalculatedValue());
}
Why this matters:
By focusing solely on business outcomes, you enhance the clarity and robustness of tests, ensuring they validate business functionality, not implementation.
Pitfall 6: Over-Complicating Test Cases
Sometimes, tests can be over-complicated, making them harder to maintain and understand. Keep tests straightforward and focused.
Keeping it Simple
Avoid lengthy test cases with multiple assertions. Break them down into smaller, focused tests:
@Test
public void testRuleOne() {
// Test for first rule
}
@Test
public void testRuleTwo() {
// Test for second rule
}
Why this matters:
Easier-to-understand tests enhance maintainability and help identify where things go wrong faster.
The Bottom Line
JUnit testing with JBoss Drools can be straightforward if you are aware of common pitfalls. Avoiding misconfigurations, ensuring proper cleanup, and focusing on business logic will guide you toward a solid testing framework.
For more in-depth reading about JBoss Drools and its capabilities, consider reviewing the official Drools documentation. And to learn about best practices in JUnit testing, checkout JUnit documentation.
Happy testing!