Mastering Error Handling in Functional Java Programming
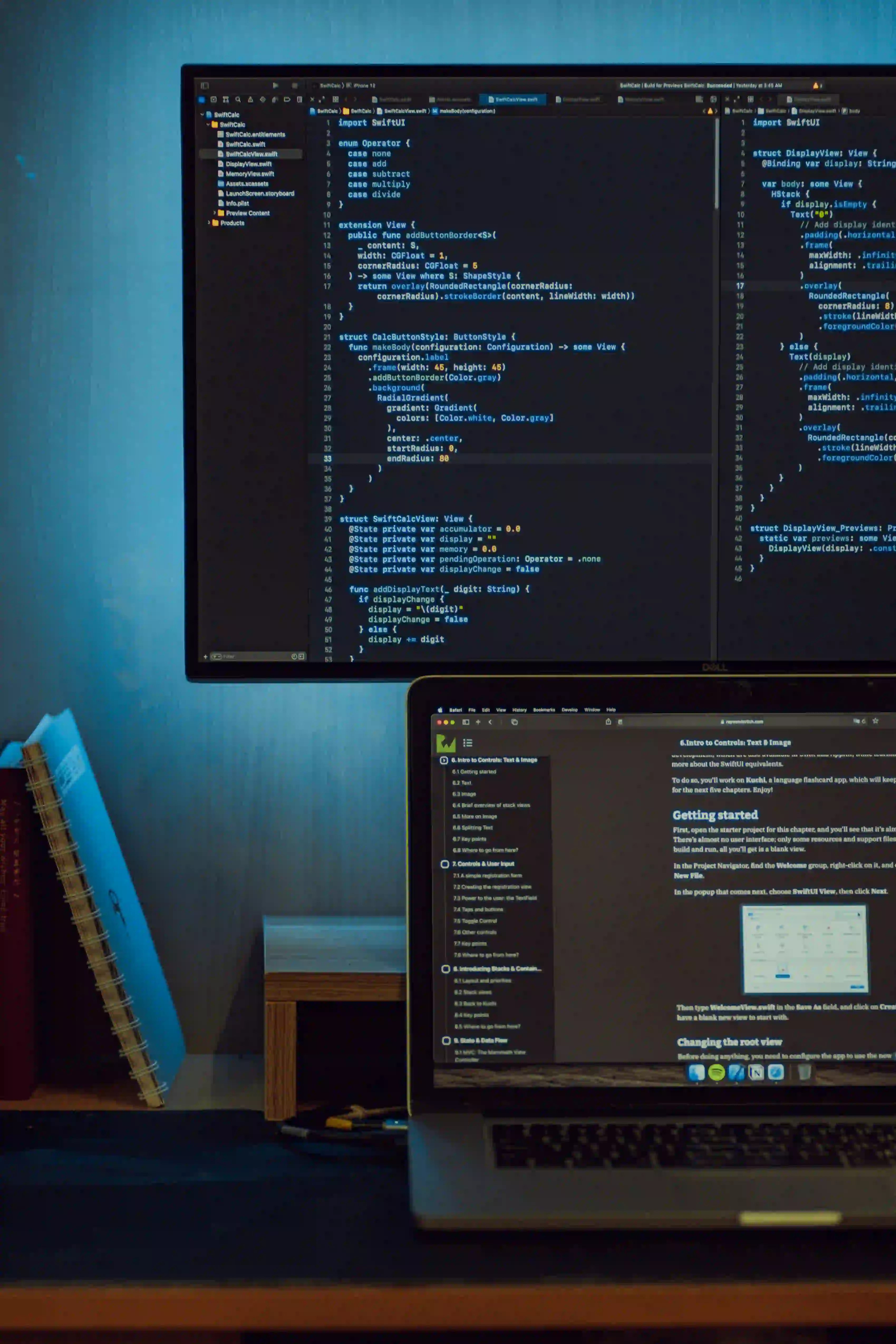
Mastering Error Handling in Functional Java Programming
Error handling has always been an essential part of writing robust applications. In the context of functional programming, especially when using Java, it requires a paradigm shift in how we think about errors. This blog post will delve into advanced error handling techniques in functional Java, exploring concepts, code examples, and best practices.
Understanding Functional Programming
Functional programming (FP) is a programming paradigm where computation is treated as the evaluation of mathematical functions. Unlike imperative programming, where state and mutable data play central roles, FP emphasizes immutability and function composition. This shift encourages programmers to write cleaner, more maintainable code.
In Java, FP principles were brought to the forefront with the introduction of lambdas, streams, and method references in Java 8. However, the traditional exception-handling mechanisms often fall short in functional scenarios, leading to potential pitfalls if not addressed properly.
The Challenge of Error Handling in Functional Programming
In traditional Java programming, errors and exceptions are handled through try-catch blocks. This imperative approach can clutter the code with boilerplate and lead to the infamous "spaghetti code." In functional programming, especially with the use of Streams, using imperative constructs to handle errors can defeat the purpose of writing functional code.
Key Concepts
To effectively manage errors in functional programming with Java, several key concepts should be understood:
-
Option Type: Instead of returning null or throwing exceptions, consider using an
Optional<T>
. This type encapsulates a value that might or might not be present, forcing clients to handle both cases. -
Either Type: This is a data type that can hold one of two possible values: a success (Right) or a failure (Left). Libraries such as Vavr provide implementations of the Either type.
-
Functional Interfaces: Leveraging functional interfaces allows you to define methods that can execute code blocks and handle results more elegantly.
Implementing Option Type
The Optional
class is a container for values that may be present or absent. It forces users to explicitly handle the absence of a value.
Example: Using Optional for Error Handling
Here's an example of how to apply Optional
for error handling. Let's say we have a method to retrieve a user by their ID.
import java.util.Optional;
public class UserService {
public Optional<User> findUserById(String userId) {
// Simulating a user lookup
User user = userRepository.findById(userId);
return Optional.ofNullable(user);
}
}
Why Use Optional?
Using Optional
here eliminates the possibility of a NullPointerException
. It forces the caller to handle the absence of a user explicitly.
Optional<User> user = userService.findUserById("123");
user.ifPresent(u -> System.out.println("User found: " + u.getName()));
In this snippet, we can safely operate on user
knowing that it either contains a value or is empty.
Implementing Either Type
The Either
type provides a robust way to signal successes and failures without throwing exceptions. This pattern helps to maintain flow while handling both outcomes effectively.
Example: Using Either for Error Handling
Using an Either
type to represent operations that may result in failures can significantly streamline error handling:
import io.vavr.control.Either;
public class UserService {
public Either<String, User> findUserById(String userId) {
User user = userRepository.findById(userId);
if (user == null) {
return Either.left("User not found");
} else {
return Either.right(user);
}
}
}
Why Use Either?
This implementation provides a clear contract: Either a meaningful error message, or the expected result. The caller handles both cases cleanly.
Either<String, User> result = userService.findUserById("123");
result.peek(user -> System.out.println(user.getName()))
.onLeft(error -> System.err.println("Error: " + error));
This concise approach prevents deep nesting and improves code readability.
Composable Error Handling
Error handling doesn't have to be linear. You can combine error handling and business logic through methods composition.
Example: Chaining Operations
Consider a scenario where you need to validate a user before processing their data:
import io.vavr.control.Either;
public class UserService {
public Either<String, User> getUserData(String userId) {
return findUserById(userId)
.flatMap(user -> validateUser(user)
.map(validUser -> fetchUserData(validUser));
}
private Either<String, User> validateUser(User user) {
if (!user.isActive()) {
return Either.left("User is not active");
}
return Either.right(user);
}
private User fetchUserData(User user) {
// Fetches user data...
return userData;
}
}
Why Use Chaining?
Chaining operations allows for a clear view of the business logic while also providing a robust error handling pathway. Each function in the chain can handle errors in context.
In Conclusion, Here is What Matters
Mastering error handling in functional Java programming not only streamlines your code but also adds layers of robustness and elegance. By utilizing types such as Optional
and Either
, you can effectively manage errors without resorting to cluttered try-catch blocks.
In functional programming, the goal is to increase clarity and maintainability. By adopting these practices and embracing the functional paradigm, your Java code can transform significantly. As you implement these techniques, consider examining existing libraries that use these concepts — for instance, Vavr has fantastic resources to further explore functional programming in Java.
Remember, efficient error handling is paramount in building resilient applications. The more you familiarize yourself with these patterns, the more adept you will be at writing idiomatic Java that leverages functional concepts. Embrace the change and start your journey toward mastering error handling in functional Java programming today!