Mastering Hibernate Criteria API: Common SQL Pitfalls
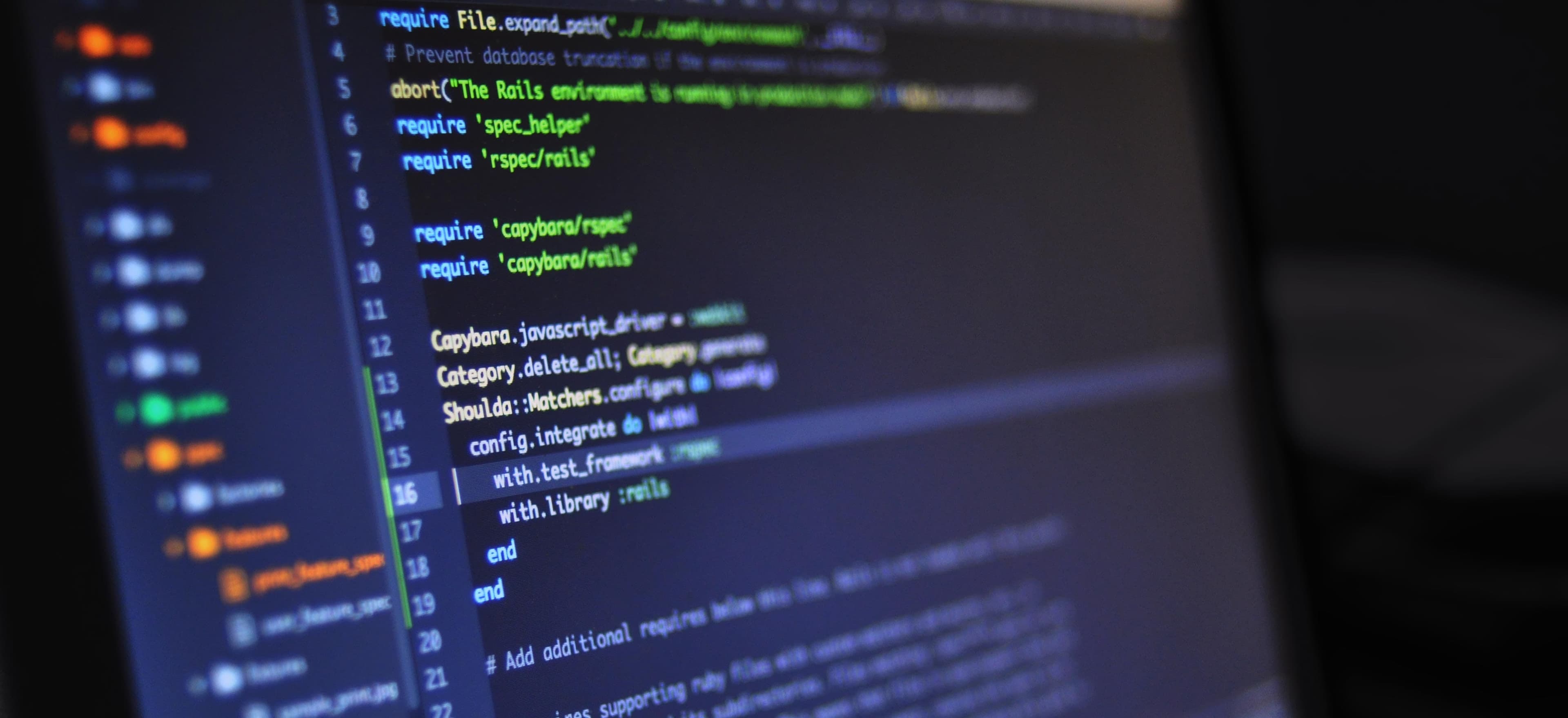
- Published on
Mastering Hibernate Criteria API: Common SQL Pitfalls
When working with Java applications that involve relational databases, Hibernate often becomes an essential ORM (Object-Relational Mapping) tool. While Hibernate simplifies database interactions and abstracts SQL complexities, developers sometimes encounter pitfalls that lead to inefficient queries and unexpected behavior. Among these, the Criteria API provides a powerful and flexible way to query data, yet there are common mistakes that many fall into.
What is Hibernate Criteria API?
The Hibernate Criteria API allows developers to build dynamic queries using a programmatic approach. It abstracts SQL's structure, helping create type-safe queries while maintaining flexibility.
This method is especially useful in scenarios where query requirements evolve or depend on user input. Creating a query dynamically is a breeze with the Criteria API, compared to building complex SQL strings.
How to Get Started with Hibernate Criteria API
Before diving into the pitfalls, let's set the stage with a basic understanding of how to craft a simple Criteria query.
Basic Example of CriteriaQuery
Here's a code snippet to illustrate the use of the Criteria API:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.Criteria;
import org.hibernate.criterion.Restrictions;
public class HibernateExample {
public static void main(String[] args) {
SessionFactory sessionFactory = HibernateUtil.getSessionFactory();
Session session = sessionFactory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
// Create a criteria query instance
Criteria criteria = session.createCriteria(Employee.class);
// Add a restriction
criteria.add(Restrictions.eq("department", "HR"));
// Fetch results
List<Employee> employees = criteria.list();
for (Employee employee : employees) {
System.out.println(employee.getName());
}
tx.commit();
} catch (Exception e) {
if (tx != null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
}
Explanation of the Code Snippet
-
Session Creation: We open a session to interact with the database. Using
SessionFactory
ensures that connections are managed efficiently. -
Criteria Initialization: The
createCriteria
method is used to create a Criteria object specific to theEmployee
entity. -
Adding Restrictions: The
add
method, paired withRestrictions
, allows for filtering records based on specific criteria. In this case, we filter employees from the HR department. -
Executing the Query: Finally, we call
list()
to execute the query and retrieve the results.
Now, let's delve into the more significant pitfalls developers may encounter when using the Hibernate Criteria API.
Common SQL Pitfalls in Hibernate Criteria API
1. Not Using Projections
Using projections can lead to performance issues. If you only need a few fields from an entity, fetching the entire entity can be wasteful.
Pitfall Example:
In cases where only names are needed, a developer might use:
Criteria criteria = session.createCriteria(Employee.class);
List<Employee> employees = criteria.list();
Improved Approach:
Using projections is ideal:
criteria.setProjection(Projections.property("name"));
List<String> employeeNames = criteria.list();
Why This Matters: By utilizing projections, you minimize data transfer between your database and application, improving performance.
2. Ignoring Pagination
Fetching all records can bog down your application, especially with large datasets.
Pitfall Example:
Failing to paginate results can lead to performance degradation:
List<Employee> employees = criteria.list();
Improved Approach:
Implement pagination:
criteria.setFirstResult(0); // start from record 0
criteria.setMaxResults(50); // retrieve only 50 records
List<Employee> employees = criteria.list();
Why This Matters: Pagination reduces memory consumption and improves response times for users interacting with large datasets.
3. Mismanaging Entity Relationships
Hibernate’s entity associations can be tricky. Many developers miss the importance of fetching strategies (e.g., Lazy vs. Eager loading).
Pitfall Example:
Loading an entire Project
entity when only the ProjectName
is needed can cause unnecessary database loading.
Criteria criteria = session.createCriteria(Project.class);
criteria.setFetchMode("employees", FetchMode.LAZY);
Improved Approach:
Ensure that you understand the relationship and use fetch modes appropriately.
Why This Matters: Lazy loading prevents extra data from being loaded into your application, improving efficiency.
4. Creating Inefficient Joins
Using the wrong types of joins can lead to performance issues and convoluted SQL queries.
Pitfall Example:
Using criteria to join entities without considering performance can cause N+1 query issues.
criteria.createAlias("employees", "e");
criteria.add(Restrictions.eq("e.department", "HR"));
Improved Approach:
Consider using setFetchMode
optimally to define how data is fetched.
criteria.createAlias("employees", "e", JoinType.LEFT);
criteria.setFetchMode("employees", FetchMode.JOIN);
Why This Matters: Utilizing the correct join type can mean the difference between loading too many rows and effectively fetching just what you need.
5. Failing to Handle Transactions Properly
Improper transaction management can lead to issues like data inconsistency or memory leaks.
Pitfall Example:
Neglecting to rollback a transaction leads to lingering open sessions:
try {
// Code that may throw exception
} catch (Exception e) {
// No rollback implemented
}
Improved Approach:
Always ensure that transactions are committed or rolled back properly.
try {
tx.commit();
} catch (Exception e) {
if (tx != null) tx.rollback();
}
Why This Matters: Proper transaction management ensures data integrity and releases resources promptly.
Lessons Learned
Utilizing the Hibernate Criteria API can significantly streamline database interactions in your Java applications. However, like any tool, it requires a thoughtful approach.
By avoiding these common pitfalls, you not only optimize your queries for better performance but also enhance the maintainability and scalability of your application.
Remember, a well-structured Criteria API query leads to cleaner code, efficient database operations, and ultimately, a better user experience.
For further insights into Hibernate best practices, consider exploring the Hibernate documentation.
Happy coding!