Common Pitfalls in Implementing Blowfish Encryption
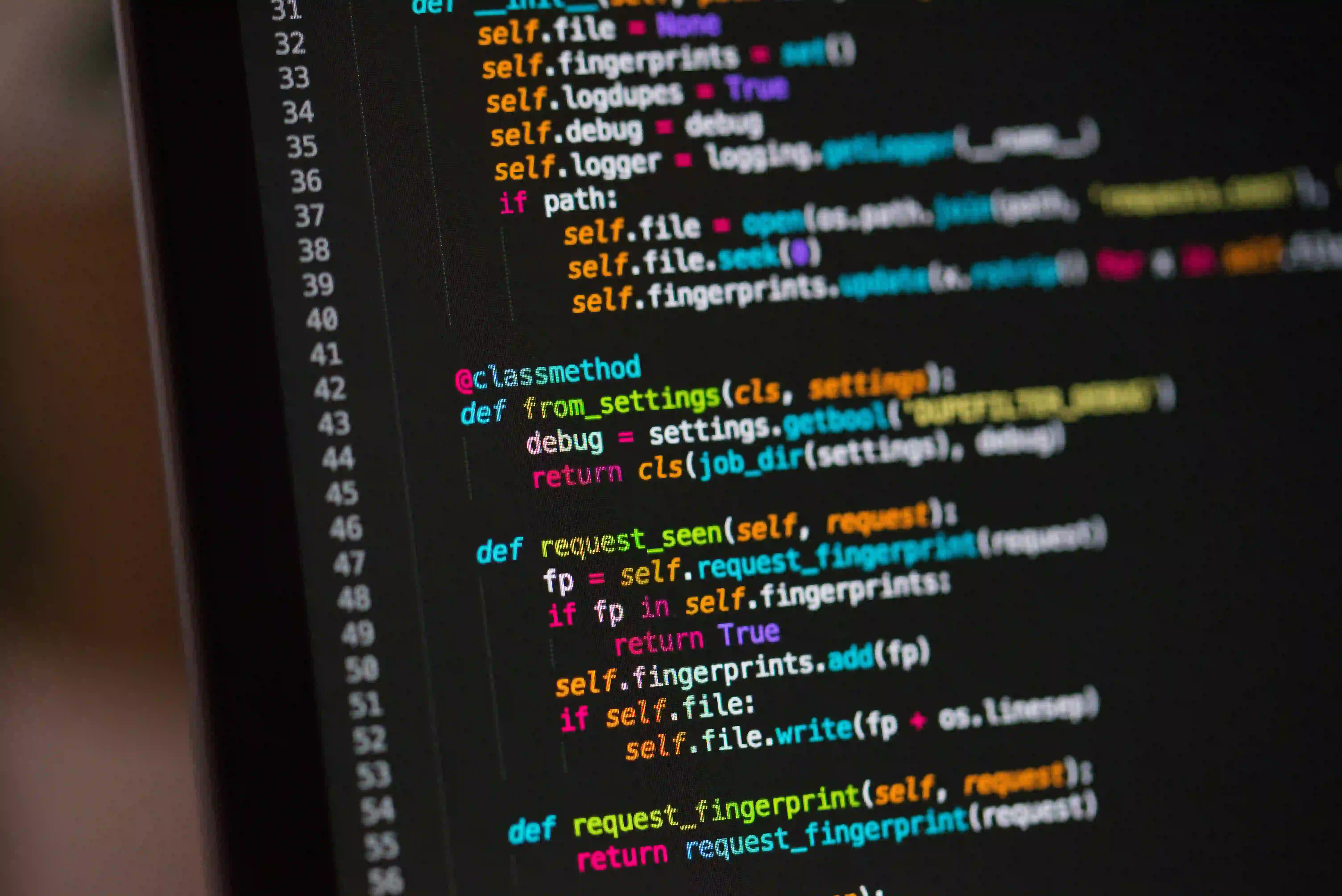
Common Pitfalls in Implementing Blowfish Encryption
Encryption is a critical aspect of securing sensitive data in an increasingly connected world. Among various encryption algorithms, Blowfish is frequently used because of its efficiency and speed. However, its implementation comes with pitfalls that can lead to vulnerabilities. In this blog post, we will discuss some common pitfalls encountered when implementing Blowfish encryption in Java, backed by code examples and clear explanations.
1. Understanding Blowfish
Blowfish is a symmetric-key block cipher that encrypts data in 64-bit blocks using a key ranging from 32 bits to 448 bits in length. Its simplicity and speed make it a go-to choice for many applications. However, the effectiveness of Blowfish largely depends on its implementation.
A Simple Java Implementation
Before diving into common pitfalls, let’s see a straightforward implementation of Blowfish encryption:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public class BlowfishExample {
public static void main(String[] args) throws Exception {
String dataToEncrypt = "SensitiveData";
// Generate a key
KeyGenerator keyGen = KeyGenerator.getInstance("Blowfish");
SecretKey secretKey = keyGen.generateKey();
// Encrypt the data
byte[] encryptedData = encrypt(dataToEncrypt.getBytes(), secretKey);
// Decrypt the data
byte[] decryptedData = decrypt(encryptedData, secretKey);
System.out.println("Original: " + dataToEncrypt);
System.out.println("Decrypted: " + new String(decryptedData));
}
public static byte[] encrypt(byte[] data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance("Blowfish");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data);
}
public static byte[] decrypt(byte[] data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance("Blowfish");
cipher.init(Cipher.DECRYPT_MODE, key);
return cipher.doFinal(data);
}
}
Explanation
- Key Generation: The
KeyGenerator
class generates a random key, which is essential for security. - Encryption/Decryption: The
Cipher
class handles both operations. Proper initialization of the cipher is critical for correct functioning.
2. Common Pitfalls
Pitfall 1: Weak Key Management
Using weak or predictable keys is an enormous risk. A common mistake is hard-coding keys or using default keys.
Solution: Always use secure key generation practices and store keys securely. Avoid hard-coding and consider using a Key Management System (KMS).
Pitfall 2: Poor Padding Scheme
The Blowfish algorithm requires proper padding when the input data size is not a multiple of the block size. Failure to implement the appropriate padding can lead to data corruption.
Example of Padding Implementation:
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import javax.crypto.spec.PaddedBufferedBlockSize;
import java.util.Arrays;
public static byte[] encryptWithPadding(byte[] data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance("Blowfish/CBC/PKCS5Padding");
IvParameterSpec iv = new IvParameterSpec(new byte[8]);
cipher.init(Cipher.ENCRYPT_MODE, key, iv);
return cipher.doFinal(data);
}
Why Padding is Important: Padding ensures that the plaintext size matches the block size requirements, preventing the cipher from crashing.
Pitfall 3: Not Using Initialization Vectors (IV)
Using a static IV can weaken the encryption significantly by making ciphertext patterns predictable. When implementing encryption, always utilize dynamic IVs.
Correct Implementation:
public static byte[] encryptWithIV(byte[] data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance("Blowfish/CBC/PKCS5Padding");
byte[] iv = new byte[cipher.getBlockSize()];
SecureRandom random = new SecureRandom();
random.nextBytes(iv);
IvParameterSpec ivSpec = new IvParameterSpec(iv);
cipher.init(Cipher.ENCRYPT_MODE, key, ivSpec);
byte[] cipherText = cipher.doFinal(data);
return combineIvAndCipherText(iv, cipherText);
}
private static byte[] combineIvAndCipherText(byte[] iv, byte[] cipherText) {
byte[] combined = new byte[iv.length + cipherText.length];
System.arraycopy(iv, 0, combined, 0, iv.length);
System.arraycopy(cipherText, 0, combined, iv.length, cipherText.length);
return combined;
}
Why IVs Are Essential: IVs add randomness to the encryption process, making it resistant to pattern analysis.
Pitfall 4: Inadequate Error Handling
Ignoring exceptions can lead to security vulnerabilities. Network communication errors, input validation errors, and encryption errors should all be handled.
Example of Proper Error Handling:
public static byte[] safeEncrypt(byte[] data, SecretKey key) {
try {
return encrypt(data, key);
} catch (Exception e) {
throw new RuntimeException("Encryption failed", e);
}
}
Why Robust Error Handling Matters: It prevents users from receiving misleading information about operation success and can protect against information leakage.
Pitfall 5: Lack of Testing
Failing to rigorously test the encryption and decryption processes for various input sizes can lead to unexpected behavior.
Recommendation: Write unit tests that cover edge cases, including null inputs, empty strings, and boundary value sizes.
Final Considerations
Blowfish encryption is potent when implemented correctly, but numerous pitfalls can compromise its effectiveness. By being aware of common issues such as weak key management, inadequate padding schemes, misuse of IVs, lack of error handling, and insufficient testing, you can fortify your encryption strategy.
To deepen your understanding of cryptography and enhance your security design, consider exploring resources such as NIST Cryptographic Standards and The OWASP Cryptographic Storage Cheat Sheet.
Embarking on a journey to implement cryptography can be complex, but with awareness and diligence, you can navigate it successfully. Happy coding!