Mastering the Open-Closed Principle for Flexible Code Design
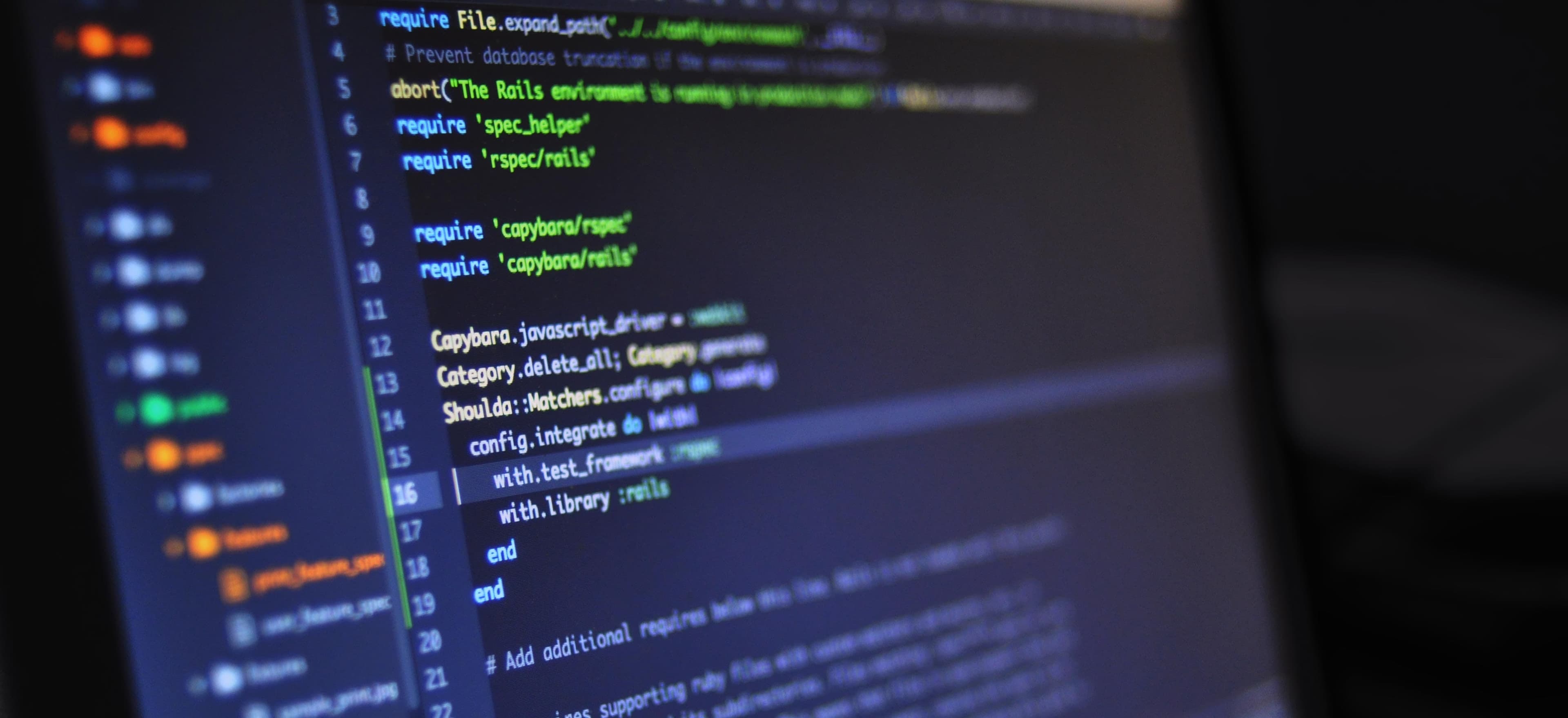
- Published on
Mastering the Open-Closed Principle for Flexible Code Design
In the world of software development, designing flexible and maintainable code is essential for long-term success. One guiding principle that has stood the test of time is the Open-Closed Principle (OCP). This principle encourages developers to write code that is open for extension but closed for modification. In this article, we'll explore the concepts behind the Open-Closed Principle, its importance in Java programming, and how to implement it effectively.
What is the Open-Closed Principle?
The Open-Closed Principle is one of the five SOLID design principles. Coined by Bertrand Meyer in 1988, the OCP states that software entities (such as classes, modules, and functions) should be open for extension but closed for modification. This means that a class should allow for new functionality to be added without altering its existing code structure.
The Need for OCP
As codebases grow, making changes to existing code can lead to unforeseen bugs and increased complexity. By adhering to OCP, developers can introduce new features without risking the integrity of existing code, thereby improving maintainability and flexibility.
Why OCP Matters in Java
Java, one of the most popular programming languages, benefits immensely from applying the Open-Closed Principle. Java's object-oriented nature makes it conducive to implementing extensions through abstraction and polymorphism. When we design our Java applications with OCP in mind, we can:
- Encourage Reusability: Code that adheres to OCP is more likely to be reusable across different parts of a project.
- Reduce Risk of Bugs: By not modifying the existing code base, the likelihood of introducing new bugs decreases.
- Promote Clean Architecture: OCP supports a clean separation of concerns, making your application easier to manage.
Implementing OCP in Java
Let's walk through implementing the Open-Closed Principle in Java using a simple example: a payment processing system. We'll start with a basic implementation and then refactor it to comply with the OCP.
Step 1: Initial Implementation
Suppose we have a class that processes payments:
public class PaymentProcessor {
public void processPayment(String type, double amount) {
if (type.equals("credit")) {
System.out.println("Processing credit payment of $" + amount);
} else if (type.equals("debit")) {
System.out.println("Processing debit payment of $" + amount);
} else {
System.out.println("Invalid payment type");
}
}
}
Issues with the Initial Implementation
- Modifications Risk: Adding a new payment type (like PayPal) requires modifying the
processPayment
method, introducing a risk of bugs. - Inverse Dependency: The method directly exposes the payment types, violating the OCP principles.
Step 2: Refactoring for OCP
To adhere to OCP, we'll introduce an interface and concrete class implementations for payment types.
// PaymentStrategy interface
public interface PaymentStrategy {
void pay(double amount);
}
// Credit payment implementation
public class CreditPayment implements PaymentStrategy {
@Override
public void pay(double amount) {
System.out.println("Processing credit payment of $" + amount);
}
}
// Debit payment implementation
public class DebitPayment implements PaymentStrategy {
@Override
public void pay(double amount) {
System.out.println("Processing debit payment of $" + amount);
}
}
// New class for PayPal payment
public class PaypalPayment implements PaymentStrategy {
@Override
public void pay(double amount) {
System.out.println("Processing PayPal payment of $" + amount);
}
}
// PaymentProcessor class now adheres to OCP
public class PaymentProcessor {
public void processPayment(PaymentStrategy paymentStrategy, double amount) {
paymentStrategy.pay(amount);
}
}
Explanation of Changes
- Interface Segregation: We defined a
PaymentStrategy
interface. Each payment type implements this interface. - Flexibility: You can easily add new payment types (like
PaypalPayment
) without modifying the existingPaymentProcessor
class. - Decoupling: The
PaymentProcessor
is now loosely coupled to specific payment types, adhering to OCP.
Step 3: Using the Refactored Code
Now you can instantiate the PaymentProcessor
and choose any payment strategy dynamically:
public class Main {
public static void main(String[] args) {
PaymentProcessor processor = new PaymentProcessor();
// Using Credit Payment
PaymentStrategy credit = new CreditPayment();
processor.processPayment(credit, 100.00);
// Using Debit Payment
PaymentStrategy debit = new DebitPayment();
processor.processPayment(debit, 75.50);
// Using PayPal Payment
PaymentStrategy paypal = new PaypalPayment();
processor.processPayment(paypal, 50.00);
}
}
Step 4: Class Diagram Representation
Visualizing the structure can help clarify how OCP was implemented. The class diagram below illustrates the relationship between the PaymentProcessor
and its payment strategies:
+-------------------+
| PaymentProcessor |
+-------------------+
| +processPayment() |
+-------------------+
▲ ▲ ▲
| | |
+------+ +---+---+ +--------+
| CreditPayment | | DebitPayment |
+--------------+ +--------------+
| +pay() | +pay() |
+--------------+ +--------------+
| PaypalPayment |
+--------------+
| +pay() |
+--------------+
This diagram underscores a clean architecture that supports easy extension for future payment types.
The Last Word
The Open-Closed Principle is vital for creating flexible and maintainable Java applications. By designing our classes to be open for extension but closed for modification, we encourage reusability, reduce bugs, and promote a clean architecture.
As you practice implementing OCP, remember that design patterns, such as the Strategy Pattern showcased above, can be invaluable. Their ability to facilitate adherence to OCP will save you time and effort in the long run.
For those looking to dive deeper, consider exploring resources like Martin Fowler's article on Patterns of Enterprise Application Architecture and the SOLID principles overview on Refactoring Guru.
Keep experimenting, and soon you'll master the Open-Closed Principle and elevate your Java coding skills to new heights!