Mastering Error Handling in Spring Integration: Common Pitfalls
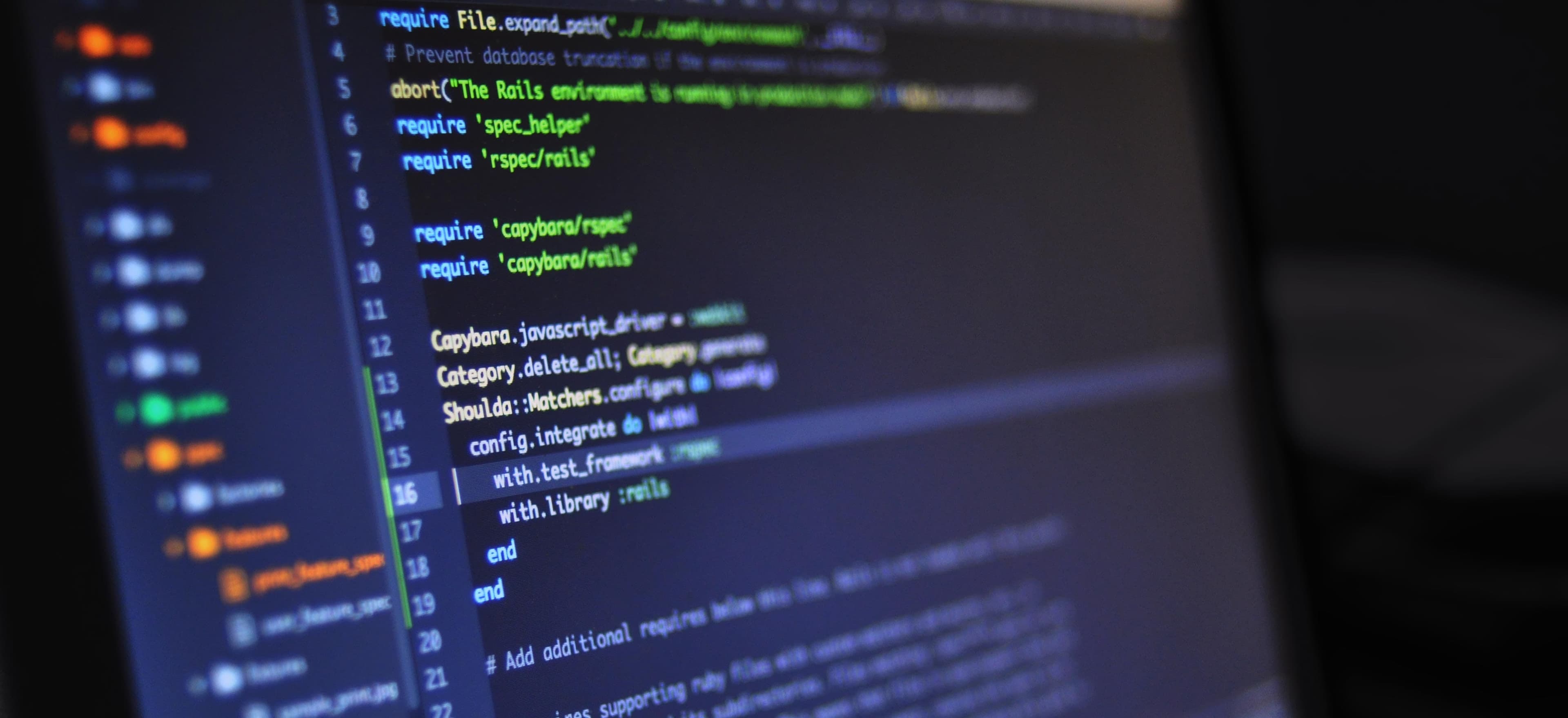
- Published on
Mastering Error Handling in Spring Integration: Common Pitfalls
Error handling is an essential aspect of robust application development, particularly in the context of Spring Integration. When integrating disparate systems, services, or APIs, it's crucial to properly manage errors to ensure that your application remains resilient and maintainable. This blog post will delve into common pitfalls in error handling within Spring Integration and provide practical advice and strategies on how to master this critical aspect of your Spring applications.
Understanding Spring Integration
Before we dive into error handling practices, it’s vital to understand the fundamentals of Spring Integration. In simple terms, Spring Integration is a framework that provides a programming model for building enterprise integration solutions. It allows you to connect different applications and services seamlessly while supporting various protocols and messaging formats.
Spring Integration employs message-driven architectures and focuses on creating reusable components, such as channels and endpoints, to streamline communication between these components.
Importance of Proper Error Handling
Effective error handling is vital for several reasons:
- Maintainability: Proper error management makes your system easier to maintain, reducing the likelihood of issues in production.
- User Experience: It enhances user experience by providing meaningful feedback when things go wrong.
- System Resilience: Robust error handling helps ensure your application can gracefully recover from unforeseen failures.
Now that we understand the importance, let’s explore common pitfalls in error handling within Spring Integration.
Common Pitfalls in Error Handling
1. Ignoring Error Channels
One of the biggest mistakes developers make is neglecting to configure error channels. Spring Integration provides a dedicated error channel for handling failures gracefully.
Consider the following example of a message flow with an error channel:
@Bean
public IntegrationFlow errorFlow() {
return IntegrationFlows.from("errorChannel")
.handle(message -> {
System.err.println("Error occurred: " + message.getPayload());
})
.get();
}
In this example, the errorChannel
listens for any errors emitted by the application. However, if you forget to define or connect an error channel in your integration flow, errors may propagate up the stack in an uncontrolled manner, leading to unhandled exceptions.
2. Using Generic Exception Handling
While it is tempting to use a generic exception handler, this approach often lacks specificity. A one-size-fits-all error handler can obscure the root causes of different errors, making it complicated to troubleshoot issues.
Instead, use more specific exception handling strategies. Here is an optimized code snippet:
@Bean
public IntegrationFlow specificErrorFlow() {
return IntegrationFlows.from("specificErrorChannel")
.handle(Message.class, message -> {
if (message.getPayload() instanceof MySpecificException) {
// Handle specific exception
} else if (message.getPayload() instanceof AnotherException) {
// Handle different exception
} else {
// Fallback for unexpected exceptions
}
})
.get();
}
In this code, you can see how we differentiate between various exceptions, leading to precise error handling. This enables you to take specific actions based on the type of error encountered.
3. Failing to Use Retry Mechanisms
Another common pitfall is the failure to implement retry mechanisms. Temporary issues, such as network outages, may lead to transient errors. In these cases, a retry mechanism can prove invaluable.
Consider this example using Spring's built-in RetryTemplate:
@Bean
public IntegrationFlow retryFlow() {
return IntegrationFlows.from("inputChannel")
.handle(message -> {
RetryTemplate retryTemplate = RetryTemplate.builder()
.maxAttempts(3)
.fixedBackoff(Duration.ofMillis(1000))
.build();
retryTemplate.execute(context -> {
// Your message processing logic here
return null;
}, context -> {
// Handle failure after retries
throw new RuntimeException("Message processing failed after retries");
});
})
.get();
}
In this example, the RetryTemplate
attempts to process the message up to three times before failing, thereby providing the system a chance to recover from intermittent issues.
4. Lack of Logging
Effective error logging is sometimes overlooked. Inadequate or no logging makes troubleshooting difficult. Proper logging captures critical information and enables quick diagnosis of issues.
It is recommended to log detailed error messages along with timestamps and context. For instance:
@Bean
public IntegrationFlow loggingErrorFlow() {
return IntegrationFlows.from("loggingErrorChannel")
.handle(message -> {
Exception e = (Exception) message.getPayload();
System.err.printf("Error occurred at %s: %s%n", LocalDateTime.now(), e.getMessage());
})
.get();
}
5. Not Implementing Global Error Handling
Global error handling can help centralize your error management, making it easier to manage and audit errors consistently across your application. To define a global error handler, you can use the @ControllerAdvice
annotation.
Here's a basic implementation:
@ControllerAdvice
public class GlobalErrorHandler {
@ExceptionHandler(Exception.class)
public void handleException(Exception ex) {
// Log and handle the exception
System.err.println("Global error occurred: " + ex.getMessage());
}
}
With this global handler, you can ensure that any unhandled exceptions from your integration flows are captured appropriately.
The Bottom Line: Best Practices for Error Handling
Mastering error handling in Spring Integration requires a keen understanding of various components and patterns. Here are some best practices to reinforce your error-handling strategies:
- Always Configure Error Channels: Ensure you have proper channels to handle errors.
- Be Specific: Avoid generic handlers; distinguish between error types.
- Implement Retries: Utilize retries for transient failures.
- Log Errors Effectively: Keep logs with meaningful context.
- Centralize Error Handling: Use global handlers when applicable.
Leveraging these practices will not only enhance the reliability of your Spring Integration applications but also improve the overall user experience.
For additional resources on Spring Integration, consider checking out the official Spring Integration Documentation and Spring Framework Documentation.
By mastering error handling, you empower your application to withstand various issues, leading to a smoother integration experience and enhancing overall operational efficiency. Happy coding!
Checkout our other articles