Struggling with Multiple Assertions in Tests? Here's How!
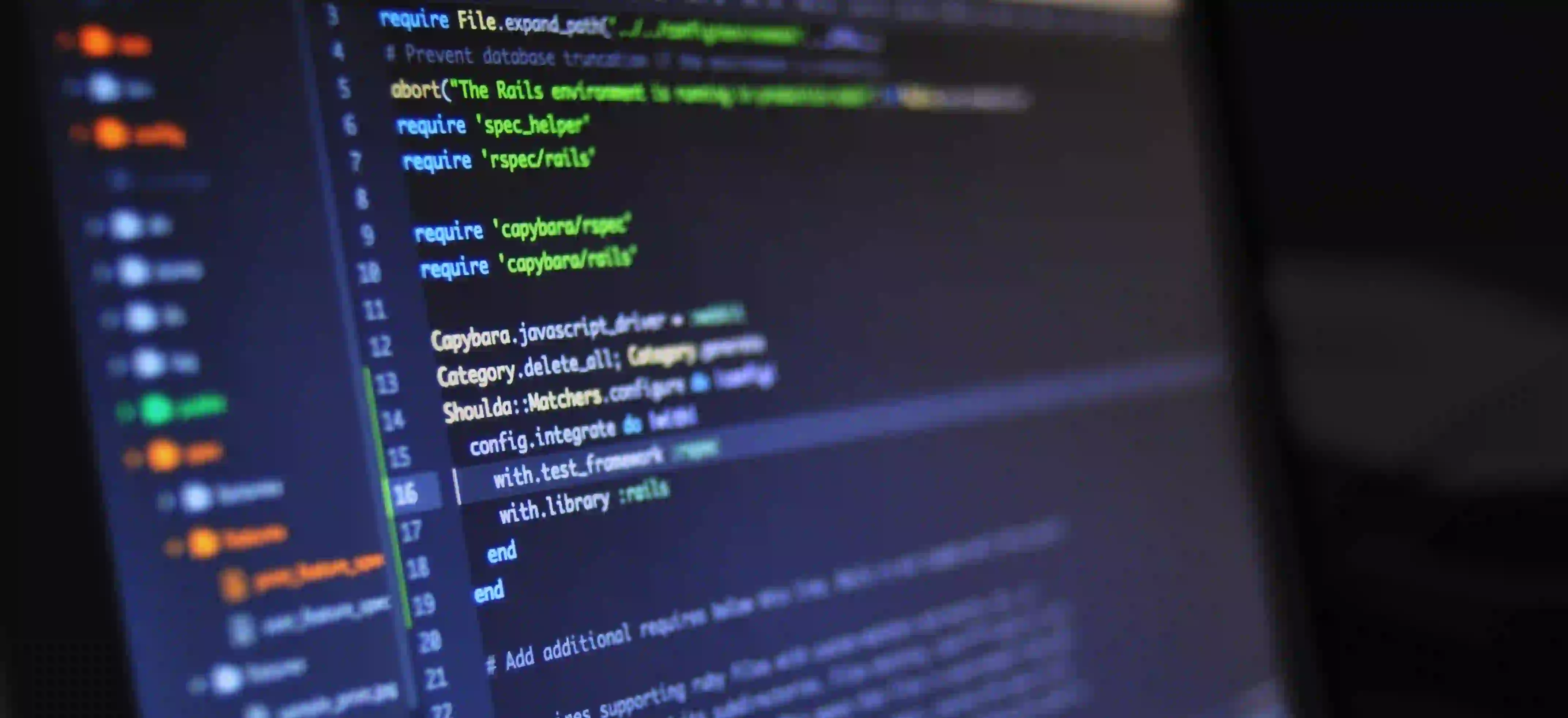
Struggling with Multiple Assertions in Tests? Here's How!
Testing is an integral part of software development. It ensures that the code behaves as expected and helps to identify bugs early in the development cycle. However, when it comes to writing tests in Java, things can get tricky, particularly when dealing with multiple assertions. This guide aims to simplify your understanding of multiple assertions in tests and provide you with effective strategies to handle them.
Why Assertions are Important
Assertions serve as checkpoints within your tests. They verify that the application behaves as intended. Multiple assertions can be useful for testing different aspects of a single unit of work, but they can introduce complexity.
The main issues you might face with multiple assertions include:
- Test Failures: Understanding which assertion failed can be challenging.
- Test Readability: A long list of assertions can make tests harder to read.
- Test Independence: Unrelated failures can mislead you about the source of the error.
Understanding the implications of multiple assertions can help you write better, more maintainable tests.
Setting Up Your Java Testing Environment
Before diving deep, ensure you have your Java testing environment set up. You will generally use JUnit, the most widely used testing framework in Java. If you haven't set this up yet, you can do so via Maven:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
Best Practices for Handling Multiple Assertions
1. Group Related Assertions
When writing tests, it is often beneficial to group related assertions together. This can help you logically segregate the checks based on what they are verifying.
For instance, if you're validating the properties of an object, consider bundling all assertions related to that object in one logical grouping:
@Test
public void testEmployeeDetails() {
Employee employee = new Employee("John", 30, "Developer");
assertEquals("John", employee.getName());
assertEquals(30, employee.getAge());
assertEquals("Developer", employee.getJobTitle());
}
Here, we are testing the fields of an Employee
object in a cohesive manner. If one assertion fails, you immediately understand that it relates to the same object, which helps in debugging.
2. Use AssertAll for JUnit 5
If you're using JUnit 5, take advantage of the Assertions.assertAll()
method that provides a structured way of handling multiple assertions. This method collects failures rather than stopping at the first failed assertion:
import static org.junit.jupiter.api.Assertions.assertAll;
import static org.junit.jupiter.api.Assertions.assertEquals;
@Test
void testAssertAll() {
Employee employee = new Employee("Jane", 25, "Designer");
assertAll("employee",
() -> assertEquals("Jane", employee.getName()),
() -> assertEquals(25, employee.getAge()),
() -> assertEquals("Designer", employee.getJobTitle())
);
}
By using assertAll
, you can pinpoint multiple assertion failures in a single test run. It's a more efficient way to debug your tests.
3. Separate Tests for Independent Assertions
When assertions are functionally independent, it's often better to separate them into individual tests. This makes it easier to identify what is failing and why.
@Test
public void testEmployeeName() {
Employee employee = new Employee("John", 30, "Developer");
assertEquals("John", employee.getName());
}
@Test
public void testEmployeeAge() {
Employee employee = new Employee("John", 30, "Developer");
assertEquals(30, employee.getAge());
}
@Test
public void testEmployeeJobTitle() {
Employee employee = new Employee("John", 30, "Developer");
assertEquals("Developer", employee.getJobTitle());
}
By breaking down the tests, you ensure that failures are more straightforward to diagnose, improving overall test readability.
4. Use Test Case Names Meaningfully
When you write multiple assertions, make sure to provide meaningful names for test methods to describe what each test is checking.
@Test
public void employeeNameShouldBeJohn() {
Employee employee = new Employee("John", 30, "Developer");
assertEquals("John", employee.getName());
}
A descriptive name can provide immediate context, which helps future developers (or even yourself) understand the purpose of the test at a glance.
5. Consider Using Hamcrest Matchers
Hamcrest library provides matching capabilities. Using matchers can make assertions more expressive and easier to read. For example:
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
@Test
public void testEmployeeDetailsUsingHamcrest() {
Employee employee = new Employee("John", 30, "Developer");
assertThat(employee.getName(), is("John"));
assertThat(employee.getAge(), is(30));
assertThat(employee.getJobTitle(), is("Developer"));
}
With Hamcrest, your assertions can convey your intent more effectively. It helps to keep tests organized and understandable.
Summary
In conclusion, when dealing with multiple assertions in Java tests, it is essential to maintain clarity and maintainability throughout your code. By grouping related assertions, utilizing JUnit 5's assertAll()
, separating tests for independent cases, using meaningful method names, and leveraging libraries like Hamcrest, you can improve both the quality of your tests and the ease with which they can be maintained.
Ultimately, effective testing not only helps to identify bugs but can also contribute significantly to the design and architecture of your code. Remember, clean tests lead to clean code!
Additional Resources
With these strategies at your disposal, you'll find that handling multiple assertions in your tests becomes a much simpler and more rewarding task. Happy testing!