Debugging Service Call Issues in Knative Serving
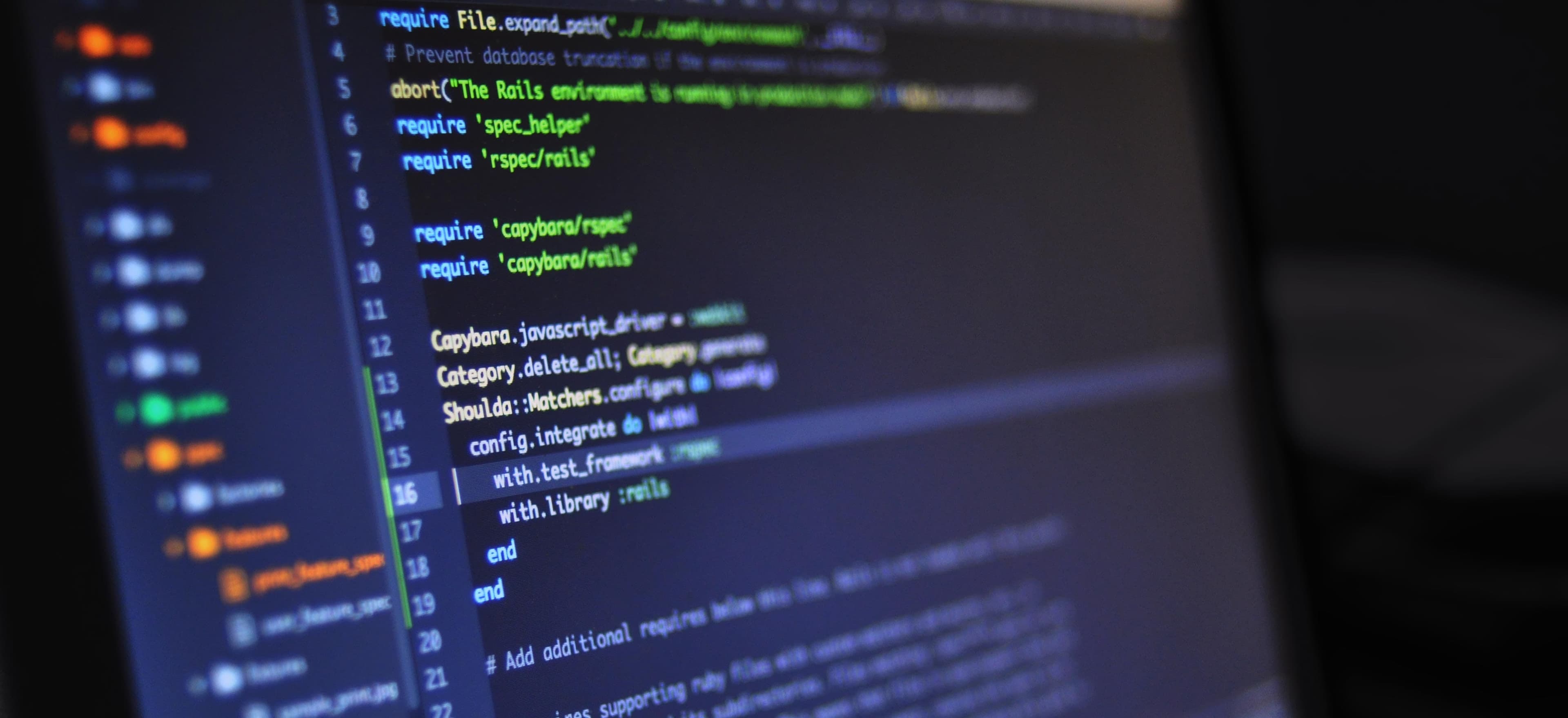
- Published on
Debugging Service Call Issues in Knative Serving
Knative Serving is a powerful framework that abstracts away the complexity of deploying and managing serverless workloads on Kubernetes. However, like any modern infrastructure, issues can arise. This blog post focuses on debugging service call issues within Knative Serving. By the end of this post, you should have a solid grasp of common problems, diagnostic techniques, and troubleshooting steps.
Understanding Knative Serving
Before diving into debugging, it's crucial to comprehend what Knative Serving is and how it operates. At its core, Knative Serving:
- Automates Deployment: Knative automates the creation of deployment resources, allowing developers to focus on writing code instead of managing container orchestration.
- Scales Applications: It can scale applications down to zero when they're not in use, saving resources and costs.
- Routes Traffic: Knative efficiently routes incoming requests to the appropriate service version.
Common Service Call Issues
When working with Knative Serving, service call issues can manifest in various ways. Here are some of the most common problems:
- 503 Service Unavailable
- Timeout Errors
- Incorrect Traffic Routing
- Misconfigured Services
Identifying the source of these errors requires a systematic approach to debugging.
Step-by-Step Debugging Process
Step 1: Check Knative Service Status
The first step in diagnosing service call issues is to check the status of your Knative services. Use the following command to get insights:
kubectl get ksvc
This command lists all Knative services along with their status. Look for any services that are not READY
. Here’s why this is important:
- A service marked as
NOT READY
indicates issues with the underlying deployment or configuration. - If the service has
SKIP
status, that usually results from misconfiguration or incorrect routing.
Step 2: Examine Pod Logs
If a service is not ready, inspecting the logs of the associated pods can provide valuable context. Retrieve pod names with:
kubectl get pods -n your-namespace
Then, use kubectl logs
to inspect the logs of a specific pod:
kubectl logs <pod-name> -n your-namespace
For example:
kubectl logs my-service-xyz-abc -n default
Why Check Logs? Examining pod logs can reveal runtime errors, misconfigurations, or connectivity issues that were not evident at the service status level.
Step 3: Investigate Gateway Configuration
Knative Serving relies on an ingress gateway for routing traffic. Examine the gateway configuration using:
kubectl get istio
If you’re using Istio as the ingress, ensure that the gateway is configured correctly. You may also want to check the Gateway and Virtual Service configurations:
kubectl describe gateway -n knative-serving
kubectl describe virtualservice -n knative-serving
Importance of Gateway Configurations: If the gateways and routes are misconfigured, they can lead to unreachable services and ultimately result in 503 errors.
Step 4: Test with Curl
Sometimes, the best way to diagnose service call issues is to make direct calls to the service. Using curl
, you can mimic client requests to your Knative service:
curl -v -H "Host: my-service.example.com" http://<INGRESS_IP>
Replace the placeholder with the correct ingress IP and service host.
Why Use Curl? Using curl
allows you to test the service call directly through the network. This approach can help identify if the problem lies in the application itself or in routing/ingress configurations.
Step 5: Review Service Configuration
If all else fails, review the manifest files for your Knative services. Misconfiguration can lead to numerous problems, including:
- Incorrect
image
paths - Flawed
env
variable setups - Misconfigured HTTP routes
Check the service configurations with:
kubectl get ksvc my-service -o yaml
Sample Service Configuration
Here’s a basic Knative service YAML configuration as an example:
apiVersion: serving.knative.dev/v1
kind: Service
metadata:
name: my-service
namespace: default
spec:
template:
metadata:
annotations:
autoscaling.knative.dev/minScale: '1'
autoscaling.knative.dev/maxScale: '5'
spec:
container:
image: myregistry/myservice:latest
env:
- name: ENV_VAR_NAME
value: "some-value"
Why Configuration Matters: Each field in your Knative configuration has repercussions on service behavior. An incorrect image name or missing environment variable can easily lead to service failures.
Step 6: Check Health Probes
Lastly, health checks are critical for service readiness. Knative uses Active Readiness and Liveness Probes to determine if a service is functioning correctly. To define these, configure the following in your service YAML:
livenessProbe:
httpGet:
path: /healthz
port: 8080
initialDelaySeconds: 30
periodSeconds: 10
readinessProbe:
httpGet:
path: /ready
port: 8080
initialDelaySeconds: 5
periodSeconds: 5
Why Are Health Probes Important?
Properly configured health probes help ensure that your application is not only functional but also capable of handling traffic appropriately, if not, it may lead to service calls being rejected.
Additional Resources
To further enhance your debugging skills within the Knative ecosystem, consider checking out these resources:
Closing the Chapter
Debugging service call issues in Knative Serving can initially seem daunting. However, by systematically checking service status, examining logs, reviewing configurations, and testing calls directly, you can quickly hone in on the root cause. Remember that proper configuration and understanding of the underlying concepts are key to leveraging the full power of Knative. Happy debugging!
Checkout our other articles