Troubleshooting JMS Connection Issues in GlassFish v4
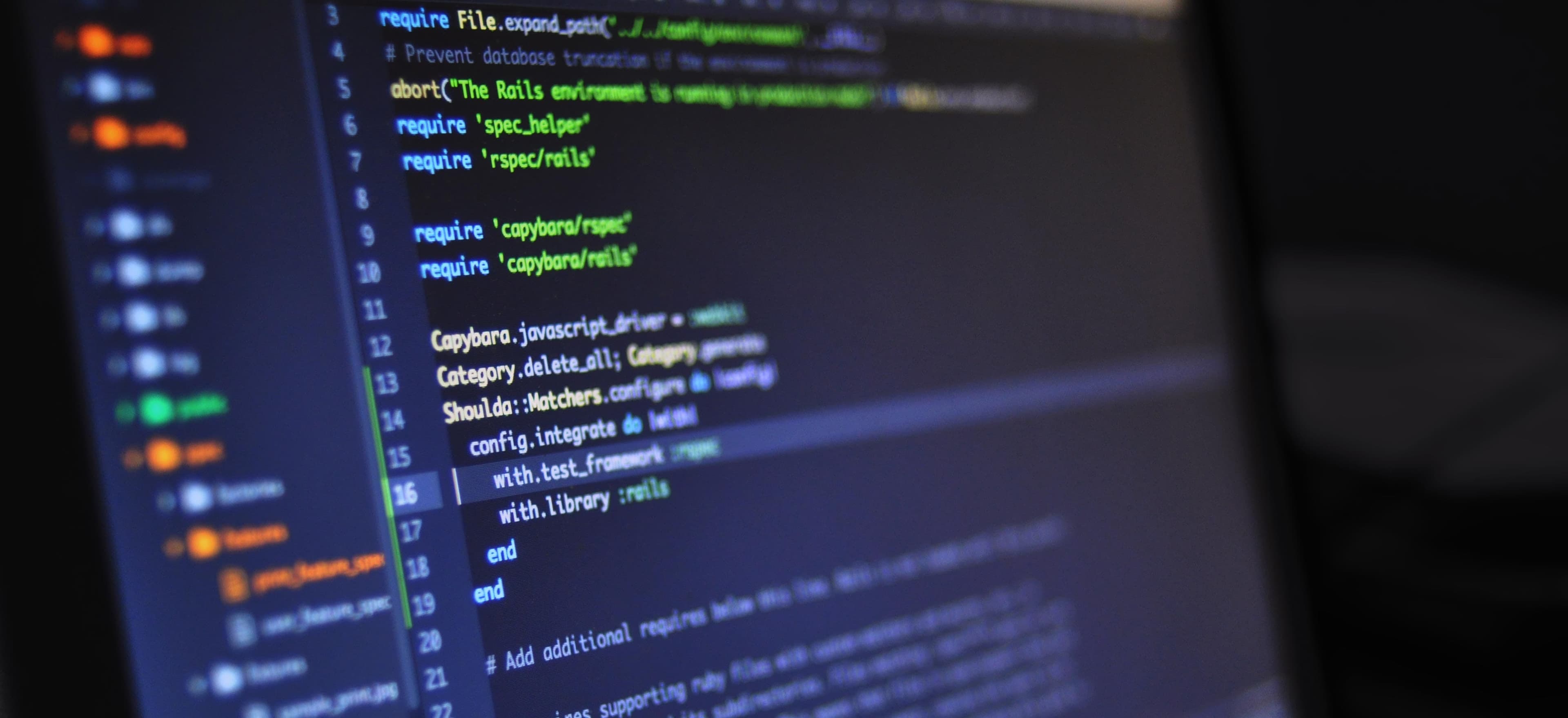
- Published on
Troubleshooting JMS Connection Issues in GlassFish v4
Java Message Service (JMS) is a crucial component in Java EE applications that require messaging capabilities. When developing applications using JMS in GlassFish v4, developers might occasionally encounter connection issues. This blog aims to provide a comprehensive guide to troubleshooting these issues effectively.
What is JMS?
Before diving into troubleshooting, let's briefly understand what JMS is. JMS provides a way for Java applications to create, send, receive, and read messages. It is based on the publish-subscribe and point-to-point messaging models, essential for building scalable, distributed systems. Oracle's official JMS documentation offers further insights into its capabilities.
Common JMS Connection Issues
When working with JMS in GlassFish, you may encounter several connection-related issues. Here are some common scenarios:
- Failed to Create Connection: This usually occurs due to network problems or incorrect configuration.
- Connection Timeout: Often a result of improper setup of the broker or network settings.
- Authentication Errors: Credentials provided for the JMS provider might be incorrect.
Steps to Troubleshoot JMS Connection Issues
1. Verify JMS Resources Configuration
To begin troubleshooting, ensure that all JMS resources are correctly configured.
Example: Check the configuration of your JMS Connection Factory.
- Login to the GlassFish Admin Console (default is
http://localhost:4848
). - Navigate to Resources > JMS Resources > Connection Factories.
Ensure that the connection factory's settings are correct. Here’s an example configuration snippet:
<jms-connection-factory>
<jndi-name>jms/MyConnectionFactory</jndi-name>
<property name="ConnectionURL">tcp://localhost:61616</property>
<property name="UserName">admin</property>
<property name="Password">admin123</property>
</jms-connection-factory>
Why: The URL, username, and password must match your messaging broker’s settings. Any discrepancy leads to connection failures.
2. Check Messaging Broker Status
If your configuration is correct, the next step is to verify that the messaging broker (such as ActiveMQ, RabbitMQ, etc.) is up and running.
- For ActiveMQ, you might access the web interface at
http://localhost:8161/admin
. - Ensure the status indicates that the broker is running, and you can send/receive messages through the interface.
Why: If the messaging broker isn’t operational, no JMS connections can be established, leading to connection issues.
3. Investigate Network Connectivity
Network issues can lead to problems with JMS connections. Use tools like ping
or telnet
to diagnose connectivity.
Command Example:
ping localhost
telnet localhost 61616 # For ActiveMQ
Why: Ensuring that your server is reachable and the necessary ports are open is critical for JMS.
4. Review Application Logs
Checking the application and server logs can provide insights into any exceptions or errors encountered when attempting to connect to JMS.
- Access logs located at
glassfish/domains/domain1/logs/server.log
.
Search for JMS
or connection
keywords in the log file. Error messages will often point you in the right direction.
Why: Logs often contain specific error messages that highlight underlying problems, assisting you in identifying the root cause.
5. Check Client Code for Errors
Sometimes the issue lies in the way the client code is written. Here's an example snippet:
import javax.jms.*;
import javax.naming.InitialContext;
import javax.naming.NamingException;
public class JMSProducer {
public static void main(String[] args) {
try {
InitialContext ctx = new InitialContext();
ConnectionFactory factory = (ConnectionFactory) ctx.lookup("jms/MyConnectionFactory");
Connection connection = factory.createConnection("admin", "admin123");
connection.start();
// Creating session
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Destination destination = session.createQueue("exampleQueue");
MessageProducer producer = session.createProducer(destination);
TextMessage message = session.createTextMessage("Hello JMS");
producer.send(message);
System.out.println("Message sent successfully!");
producer.close();
session.close();
connection.close();
} catch (NamingException ne) {
ne.printStackTrace();
} catch (JMSException jmse) {
jmse.printStackTrace();
}
}
}
Why: Double-check the usernames, passwords, and JNDI lookup names. An incorrect configuration or credentials in the code can result in connection failures.
Additional Tips
- Connection Pooling: Ensure that your application is correctly utilizing connection pooling to minimize the risk of connection saturation.
- Security Settings: If SSL is in play, confirm that certificates and security settings are correctly configured.
- GlassFish Version: Ensure you are running the latest version of GlassFish, as updates often fix bugs and improve stability.
Wrapping Up
Troubleshooting JMS connection issues in GlassFish v4 can initially appear daunting, but methodical checking of configurations, network settings, and logs brings clarity. Whether it's verifying your JMS resource configurations, checking your messaging broker's health, or ensuring your client code is correct, each step brings you closer to resolution.
For further reading and an in-depth discussion about JMS with GlassFish, check out the GlassFish documentation for comprehensive guidelines.
By following these troubleshooting steps, you can efficiently diagnose and resolve JMS connection issues, improving the resilience and reliability of your Java applications. Happy coding!