Understanding HttpStatus in Spring: Common Missteps
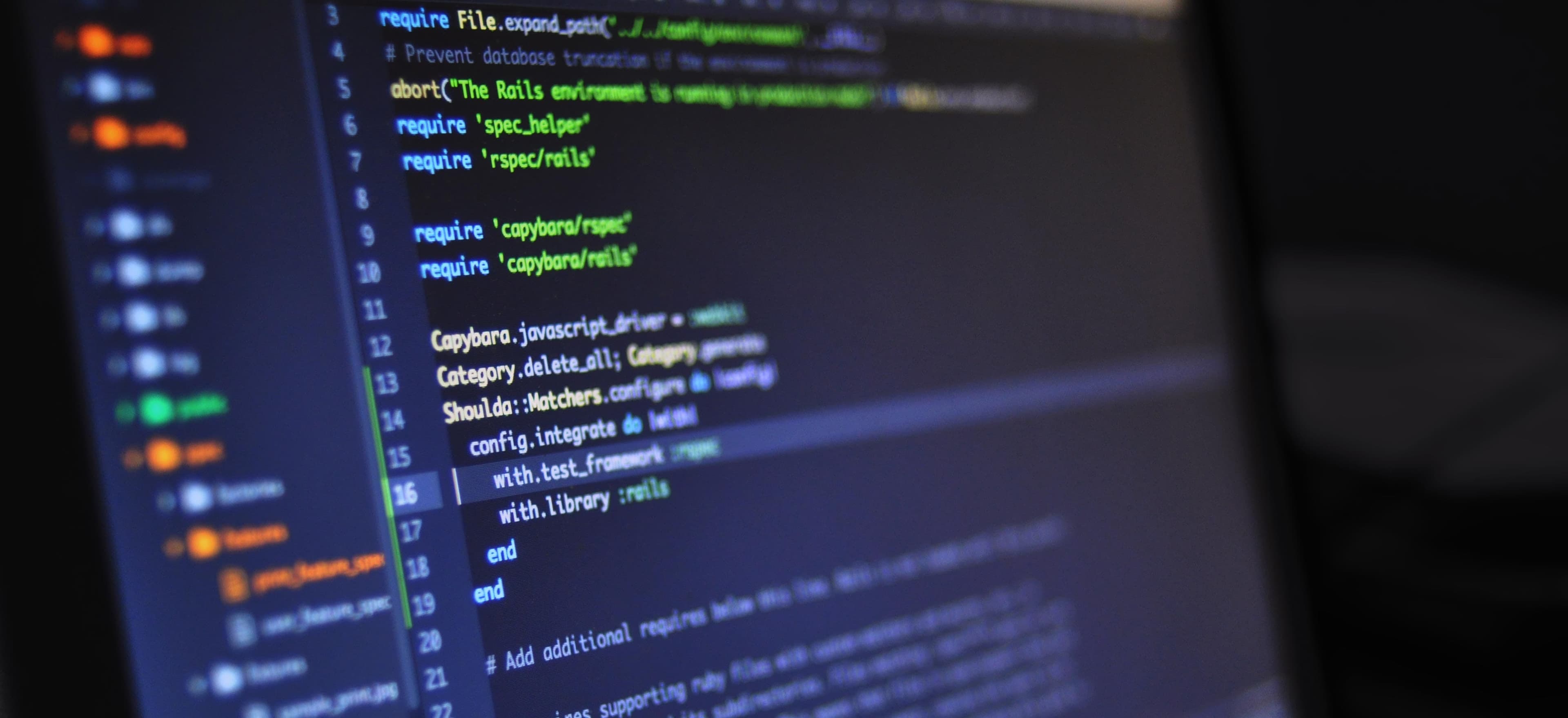
- Published on
Understanding HttpStatus in Spring: Common Missteps
Spring is a powerful framework for building Java applications, especially when it comes to developing web services. One of the pivotal concepts in web development is HTTP status codes. These codes, which indicate the result of an HTTP request, are essential for both client and server communication.
In this blog post, we will delve deep into the HttpStatus
enumeration in Spring, highlighting common missteps developers tend to make when dealing with HTTP status codes.
What is HttpStatus?
HttpStatus
is an enumeration in Spring, representing the various status codes defined by the HTTP specification. These codes are crucial for indicating different states of a response. They range from success responses (like 200 OK) to client and server error responses (like 404 Not Found and 500 Internal Server Error).
In Spring, HttpStatus
is part of the org.springframework.http
package. Using HttpStatus
consistently can simplify the way you handle responses in your application.
Common Missteps with HttpStatus
-
Ignoring the Importance of Status Codes
Many developers tend to overlook the significance of using appropriate HTTP status codes. Using a generic status like 200 OK may seem easier, but it doesn't provide meaningful context to clients regarding what happened with their request. For example, a created resource should ideally return a 201 Created status.
@PostMapping("/create") public ResponseEntity<MyResource> createResource(@RequestBody MyResource resource) { MyResource createdResource = resourceService.create(resource); return new ResponseEntity<>(createdResource, HttpStatus.CREATED); }
In this code, we're explicitly returning
HttpStatus.CREATED
to signal that a resource has been successfully created. Without this, clients might be left guessing the outcome. -
Misuse of 200 Status Code
While it might be tempting to return 200 OK for every successful operation, it's not always appropriate. For instance, when the requested resource is not found, returning 200 would signify success, which is misleading.
Here’s an example of the right way to handle a resource that can be retrieved:
@GetMapping("/resource/{id}") public ResponseEntity<MyResource> getResource(@PathVariable("id") Long id) { MyResource resource = resourceService.findById(id); if (resource == null) { return new ResponseEntity<>(HttpStatus.NOT_FOUND); } return new ResponseEntity<>(resource, HttpStatus.OK); }
This method ensures that if the resource is not found, the server communicates this via a 404 Not Found status.
-
Neglecting the Use of ResponseEntity
Another common misstep is failing to use
ResponseEntity
to encapsulate your HTTP responses. UsingResponseEntity
offers more flexibility than returning an object directly. It allows you to define not just the body of the response, but also the status and headers.@GetMapping("/greet") public ResponseEntity<String> greet(@RequestParam(required = false) String name) { String greeting = "Hello, " + (name != null ? name : "World") + "!"; return ResponseEntity.ok(greeting); }
Here, we're leveraging
ResponseEntity.ok()
which conveniently sets the HTTP status to 200 OK and encapsulates our greeting message. -
Inconsistent Status Codes in Error Handlers
When implementing global exception handling, it's critical to ensure that your application returns uniform HTTP status codes. This prevents confusion for the clients, as they won't know how to interpret different error responses.
A common approach is to use
@ControllerAdvice
along with@ExceptionHandler
. Here's an example:@ControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(ResourceNotFoundException.class) public ResponseEntity<String> handleResourceNotFound(ResourceNotFoundException ex) { return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage()); } @ExceptionHandler(Exception.class) public ResponseEntity<String> handleGeneralException(Exception ex) { return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An unexpected error occurred"); } }
By returning consistent status codes in response to different exceptions, you create a predictable API behavior, improving the overall developer experience for anyone consuming your API.
-
Confusing Client-Side Errors with Server-Side Errors
It's crucial to differentiate between client-side and server-side errors when choosing your HTTP status codes. HTTP status code 400 Bad Request indicates that the client sent a malformed or invalid request, while 500 Internal Server Error signifies that the server encountered an unexpected issue.
Always ensure that your application communicates the exact nature of the problem through appropriate status codes:
@PostMapping("/process") public ResponseEntity<String> processRequest(@RequestBody MyRequest request) { if (request.isInvalid()) { return ResponseEntity.badRequest().body("Invalid request data"); } // process the request return ResponseEntity.ok("Request processed successfully"); }
-
Overlooking the Role of 3xx Redirection Codes
Redirection is another important aspect of HTTP responses that developers often overlook. The
3xx
series of status codes signifies that further action is needed to complete the request. One common situation is the 301 Moved Permanently, which can be useful if your API has changed endpoints.@GetMapping("/old-endpoint") @ResponseStatus(HttpStatus.MOVED_PERMANENTLY) public void redirectToNewEndpoint(HttpServletResponse response) throws IOException { response.sendRedirect("/new-endpoint"); }
By implementing redirect responses correctly, you help clients seamlessly transition to your updated endpoint structures.
Best Practices for Using HttpStatus in Spring
Having covered these common missteps, let’s outline some best practices to follow for effectively using HttpStatus
in your Spring applications:
- Always use the correct status code: Reflect the outcome of the operation accurately—success, errors, or redirections.
- Use ResponseEntity: Encapsulate the response body, status, and headers effectively.
- Implement global exception handling: Create a unified strategy for dealing with errors and provide meaningful messages alongside appropriate status codes.
- Monitor client responses: Ensure your API clients review the status codes and messages returned, facilitating the debugging process.
For more comprehensive information regarding HTTP status codes, consult the RFC 7231 Specification.
My Closing Thoughts on the Matter
Understanding HttpStatus
is integral to developing robust Spring web applications. Missteps in handling status codes can lead to client miscommunication, debugging difficulties, and a less than seamless experience. By adhering to the guidelines outlined in this blog post, you'll create a clearer, more effective API that clearly communicates its behavior to clients.
If you're looking to deepen your knowledge of Spring and its capabilities, consider exploring Spring's official documentation for more insights.
Embrace the right practices, and take the time to ensure your API communicates effectively through correct HTTP status codes!
Checkout our other articles