Overcoming Complexity in Declarative Data Pipelines
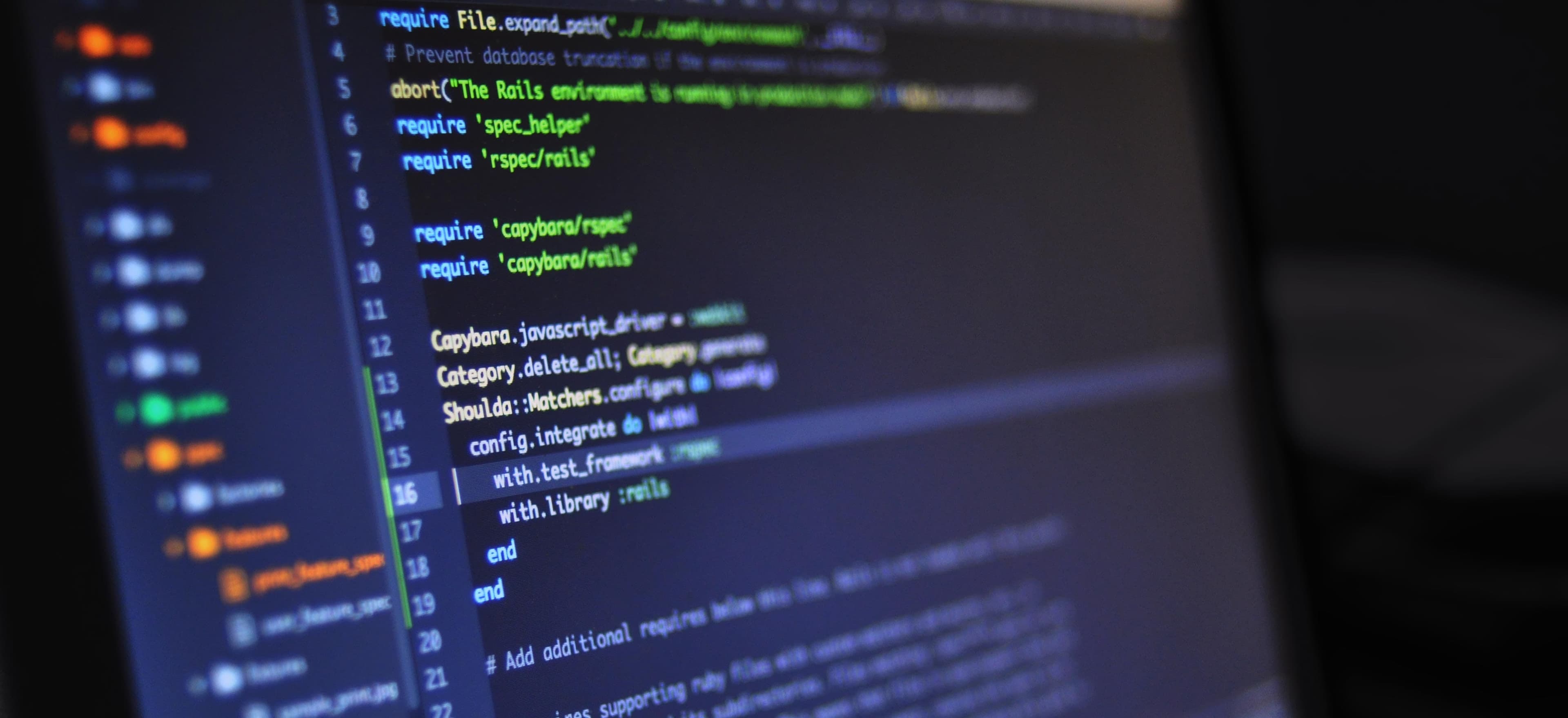
- Published on
Overcoming Complexity in Declarative Data Pipelines
In the era of big data, organizations are grappling with the challenges of data processing, transformation, and integration. As the complexity of data pipelines increases, teams find themselves seeking more efficient strategies to manage these complexities. Declarative programming models offer a compelling solution. This blog post explores how to effectively use declarative data pipelines to overcome complexity, with practical insights, Java code snippets, and resources for further learning.
Understanding Declarative Data Pipelines
Before diving into the specifics, let’s clarify what declarative data pipelines are. Unlike imperative programming, where you explicitly outline every step, declarative programming lets you specify what you want to accomplish without detailing how to achieve it. This abstraction can significantly reduce complexity.
Benefits of Declarative Data Pipelines
- Simplicity: You describe data transformations succinctly, making it easier to write and understand pipelines.
- Maintainability: Changes in requirements or data structure require less effort, as you focus on the output rather than the process.
- Parallelization: Many frameworks that employ declarative models can easily parallelize tasks, leading to improved performance.
Use Case 1: Streamlining ETL Processes
Extract, Transform, Load (ETL) processes form the backbone of data handling in many organizations. Let's see how declarative pipelines simplify ETL.
Example ETL in Java using Apache Beam
Apache Beam is an excellent framework for building data pipelines using a declarative approach. Below is an example using Java.
import org.apache.beam.sdk.Pipeline;
import org.apache.beam.sdk.transforms.MapElements;
import org.apache.beam.sdk.transforms.SimpleFunction;
import org.apache.beam.sdk.values.TypeDescriptor;
public class ETLPipeline {
public static void main(String[] args) {
Pipeline pipeline = Pipeline.create();
pipeline.apply("ReadfromSource", /* Your source here */)
.apply("TransformData", MapElements.via(new SimpleFunction<MyInputType, MyOutputType>() {
@Override
public MyOutputType apply(MyInputType input) {
// Transform logic
return new MyOutputType(input.getField1(), input.getField2());
}
}).withOutputType(TypeDescriptor.of(MyOutputType.class)))
.apply("LoadToSink", /* Your sink here */);
pipeline.run();
}
}
Commentary on the Code
- Here applying transformations is straightforward. You define a series of operations without diving into implementation details such as how the source or sink is managed.
- The
MapElements
function encapsulates the transformation logic, allowing us to focus on what data we want to manipulate rather than how it flows through our system.
Additional Resource
For more on Apache Beam, check out the Apache Beam documentation.
Use Case 2: Real-time Data Processing
Declarative pipelines shine in real-time use cases like event stream processing. Frameworks like Apache Flink and KSQL allow users to articulate data flows succinctly.
Example of Streaming with Apache Flink
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
public class StreamingPipeline {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
env.socketTextStream("localhost", 9999)
.map(new MapFunction<String, Integer>() {
@Override
public Integer map(String value) {
// Process line and extract a metric like word count
return value.split(" ").length;
}
})
.print();
env.execute("Real-time Text Processing");
}
}
Commentary on the Code
- The ability to define the pipeline with a few lines of code demonstrates Flink’s declarative approach.
- Processing the input stream and deriving a simple metric (like word count) requires minimal boilerplate, enhancing readability.
Additional Resource
For more on Apache Flink, refer to their official website.
Overcoming Common Challenges
Data Schema Evolution
One of the complexities in data pipelines is managing data schema evolution. As your data model changes, you need a strategy for maintaining backward compatibility. Declarative pipelines can help here by employing schema-on-read techniques.
Performance Optimization
While declarative pipelines offer simplicity, they may introduce performance bottlenecks if not properly optimized. Always benchmark your pipelines, and leverage techniques like:
- Partitioning: Helps read and write data in parallel.
- Predicate Pushdown: Filters data at the storage layer to reduce data volume.
Error Handling and Monitoring
Error handling in declarative pipelines can be tricky. Many frameworks provide hooks for error logging and recovery, which you should leverage. Consider implementing monitoring systems that notify you when a pipeline fails, as early detection is crucial.
The Last Word
Navigating the intricacies of data processing is a task best approached with simplicity and clarity in mind. Declarative data pipelines empower developers to focus on what they want to achieve, reducing overhead and enhancing maintainability. By employing frameworks like Apache Beam and Apache Flink, you can streamline your ETL processes and real-time data handling, ultimately leading to a more robust data strategy.
Staying informed about new developments in declarative programming models will bolster your ability to adapt and innovate. For further learning, explore resources like Martin Fowler’s blog on Data Pipelines and Google Cloud’s Guide to Data Processing.
As you embark on your journey to leverage declarative data pipelines, remember: The goal is to reduce complexity and enrich outcomes. Happy coding!