Overcoming Common Java Game Development Challenges
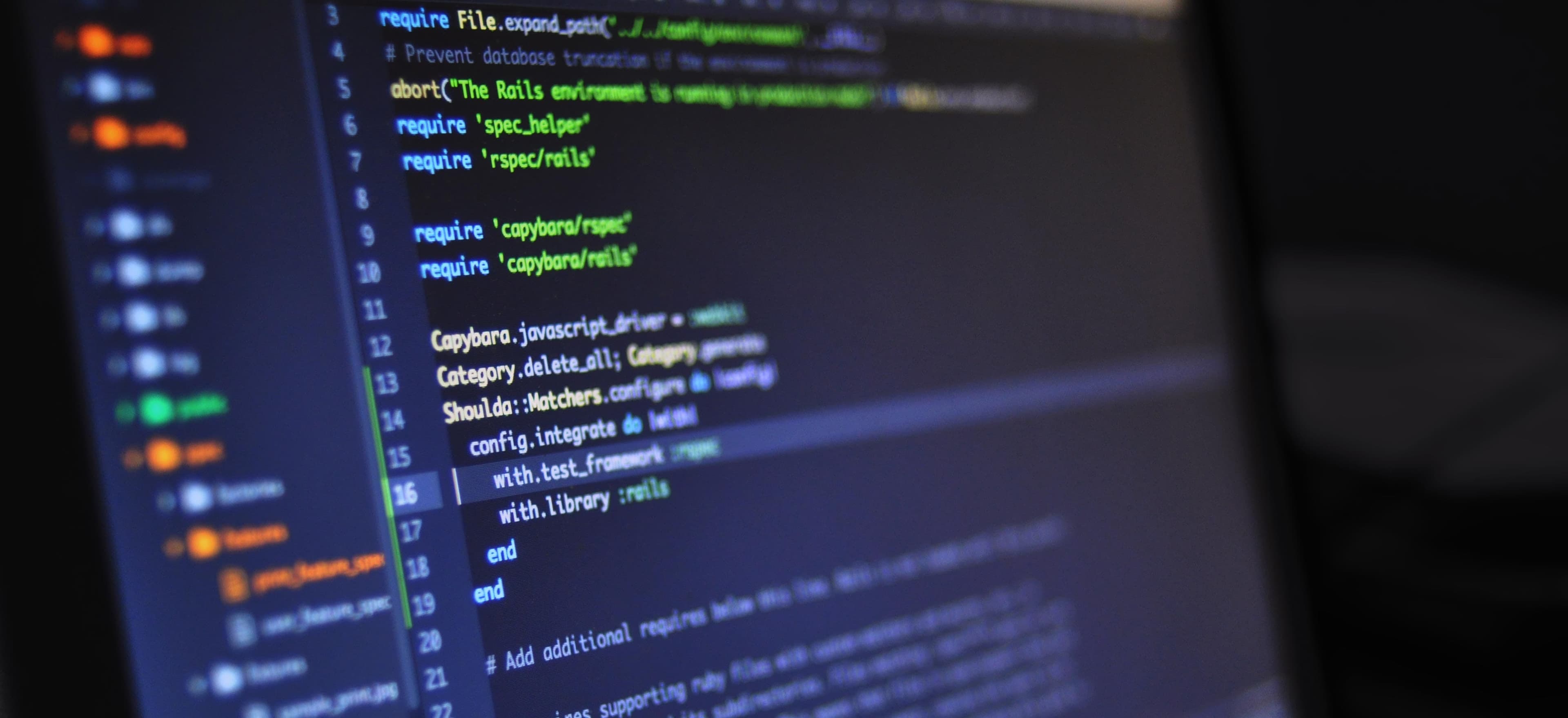
- Published on
Overcoming Common Java Game Development Challenges
Game development is a fascinating field that blends creativity with technical skills. While Java is a powerful language for game development, it can also present its own set of challenges. In this blog post, we will explore common hurdles developers face when creating games in Java and discuss practical solutions.
Diving Into the Subject
Java has gained prominence in game development due to its portability, object-oriented structure, and vast community support. However, as with any technology, certain challenges can arise. By identifying these common issues, we can equip ourselves with the solutions needed for smooth game development.
Common Challenges in Java Game Development
- Memory Management
- Performance Issues
- Collision Detection
- Graphics Rendering
- Game Logic Implementation
Let us dive deeper into each of these challenges and outline ways to overcome them.
1. Memory Management
Memory management is crucial in game development. Games often require substantial resources which can lead to memory leaks if not handled properly.
Solution: Use ByteBuffer and Optimize Resource Loading
Java provides mechanisms like ByteBuffer for efficient memory allocation. Instead of loading resources each time they are needed, load assets once and keep them in memory as long as necessary.
import java.nio.ByteBuffer;
import java.awt.image.BufferedImage;
public class ResourceLoader {
private ByteBuffer buffer;
public BufferedImage loadImage(String path) {
// Load your image file using ImageIO or a similar library.
return ImageIO.read(new File(path));
}
}
Why: By preloading images and storing them in a ByteBuffer, you reduce the overhead that would arise from repeatedly loading files during gameplay.
2. Performance Issues
Game performance is often quick to become a bottleneck, especially in graphics-heavy games.
Solution: Utilize Java’s Built-in Concurrency
Java’s concurrency utilities allow you to perform tasks in parallel. This ensures smoother graphics rendering and game logic execution.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class Game {
private ExecutorService executor = Executors.newFixedThreadPool(4);
public void update() {
executor.submit(() -> {
// Game logic updates
});
executor.submit(() -> {
// Render graphics
});
}
}
Why: By leveraging multithreading, you can run game logic and rendering simultaneously, significantly improving the game's performance.
3. Collision Detection
Collision detection is a critical aspect of any game. Implementing an effective system can be tricky and performance-intensive.
Solution: Use Bounding Box Collision Detection
A straightforward method to ensure efficient collision detection is to use Bounding Boxes. This approach simplifies complex shapes into rectangles for quick checks.
public class GameObject {
public int x, y, width, height;
public boolean collidesWith(GameObject other) {
return this.x < other.x + other.width &&
this.x + this.width > other.x &&
this.y < other.y + other.height &&
this.y + this.height > other.y;
}
}
Why: Bounding box checks allow you to quickly ascertain potential collisions before performing more complex calculations. It’s efficient and reduces computational load.
4. Graphics Rendering
Graphics rendering can be daunting, especially when creating visually appealing graphics using Java’s standard libraries.
Solution: Use Libraries like JavaFX or LibGDX
Consider using libraries specifically designed for game development, like JavaFX or LibGDX. These libraries enhance rendering capabilities and often come with built-in support for animations and transitions.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
Canvas canvas = new Canvas(600, 400);
GraphicsContext gc = canvas.getGraphicsContext2D();
draw(gc);
StackPane root = new StackPane();
root.getChildren().add(canvas);
primaryStage.setScene(new Scene(root));
primaryStage.show();
}
private void draw(GraphicsContext gc) {
gc.fillRect(10, 10, 100, 100);
}
public static void main(String[] args) {
launch(args);
}
}
Why: Libraries like JavaFX provide a higher abstraction level in handling graphics, allowing developers to focus on game design rather than the technical intricacies of rendering.
5. Game Logic Implementation
Implementing game logic can become complex as games scale, leading to convoluted code that is hard to debug and maintain.
Solution: Design Patterns and State Management
Employ design patterns such as the State Pattern or Component-Based Architecture to manage game logic effectively.
public interface GameState {
void enter();
void execute();
void exit();
}
public class PlayingState implements GameState {
public void enter() {
// Initialize game
}
public void execute() {
// Game loop logic
}
public void exit() {
// Cleanup
}
}
Why: The use of design patterns organizes code better, enhances readability, and encourages reusability, making your game logic intuitive and manageable.
The Bottom Line
While Java game development presents unique challenges, implementing strategic solutions allows developers to efficiently overcome these hurdles.
By focusing on memory management, performance, collision detection, graphics rendering, and clean game logic, you can build more robust and enjoyable games. For further reading on Java game development techniques, consider exploring the Java Game Development Wiki or diving into LibGDX's official documentation.
Remember, the goal is to create a game that captivates players. By overcoming these challenges systematically, you can bring your creative vision to life. Happy coding!
Checkout our other articles