Decoding JVM Crashes: Your Guide to Root Cause Analysis
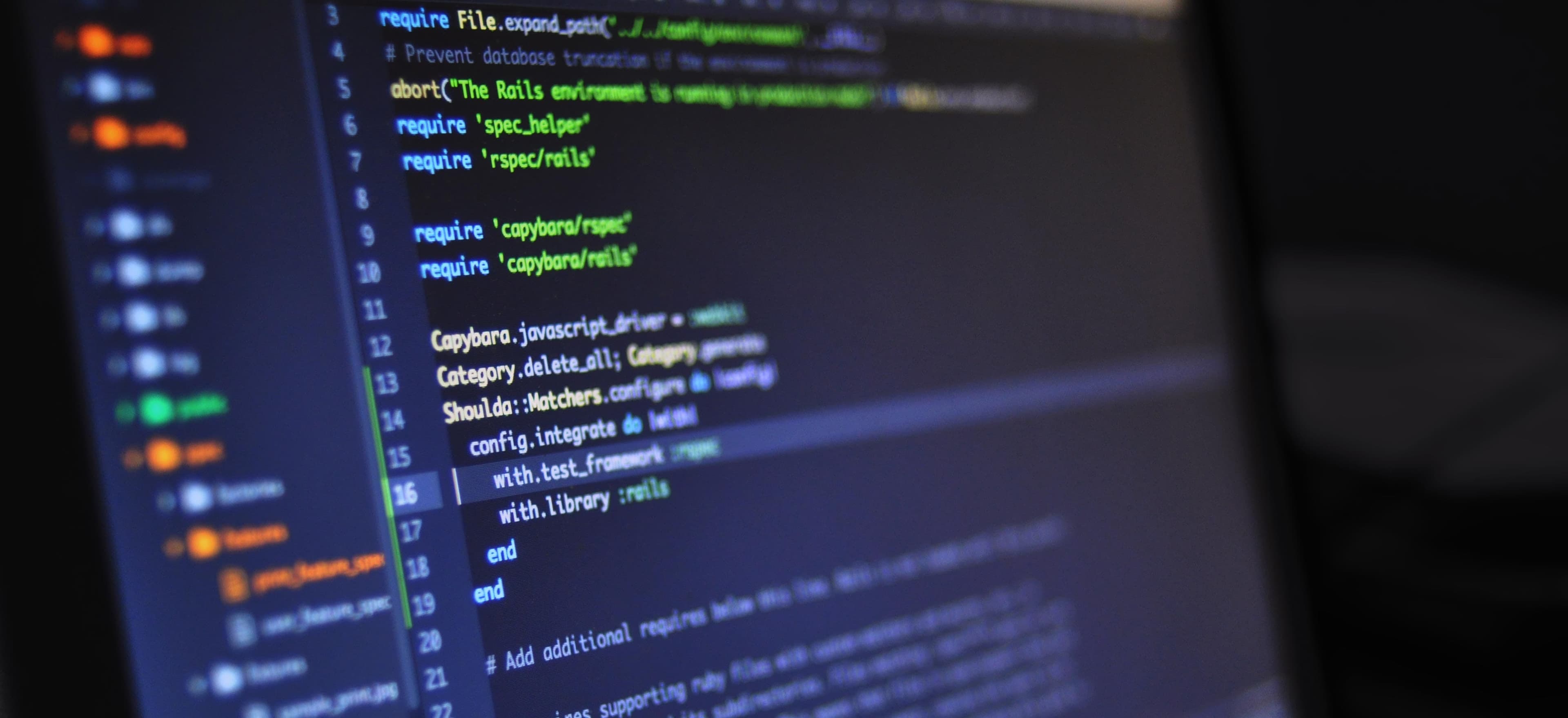
- Published on
Decoding JVM Crashes: Your Guide to Root Cause Analysis
Java Virtual Machine (JVM) crashes can lead to a cascade of issues in any application, resulting in downtimes, data losses, and frustration for both developers and users. The JVM is a powerful engine, but it is not immune to problems. In this blog post, we will delve into the complexities of JVM crashes, why they occur, techniques for diagnosing them, and how to prevent them.
Understanding the JVM
Before diving deep into issues surrounding JVM crashes, it is essential to understand how the JVM operates. The JVM acts as an intermediary between Java applications and the host operating system. It executes Java bytecode, manages system resources, and provides services like garbage collection and exception handling.
What Causes JVM Crashes?
Here are some of the primary reasons that can lead to a JVM crash:
- Native Code Issues: Java allows the integration of native code through Java Native Interface (JNI). If native code contains errors, it can cause crashes.
- Out of Memory Errors: Occurs when the JVM cannot allocate an object due to insufficient memory.
- Thread Issues: Deadlocks, race conditions, and improper thread management can lead to instability.
- Corrupted Installation: If the JVM itself is corrupted or improperly installed, it may behave unpredictably.
Understanding these causes helps in effectively analyzing and resolving JVM crashes.
Diagnosing JVM Crashes
Detection of JVM crashes can often be tricky. Here are the main steps to diagnose a crash effectively:
1. Core Dump Analysis
When a JVM crashes, it can produce a core dump—a snapshot of the memory at the time of the crash. A core dump file contains valuable information for debugging.
Example of Generating a Core Dump
To enable core dumps, you can set the -XX:+HeapDumpOnOutOfMemoryError
JVM flag to create a heap dump when the memory limit is reached.
java -XX:+HeapDumpOnOutOfMemoryError -cp your-classpath YourMainClass
Why This Matters
The heap dump provides insight into what happened just before the crash occurred. It can be analyzed using tools such as Eclipse MAT or VisualVM.
2. Analyzing Logs
JVMs usually generate log files during runtime. A thorough examination of these logs can uncover vital clues about what led to the crash.
Important Logs to Analyze
- gc.log: Shows garbage collection information and memory management.
- system log: Contains runtime exceptions and system-level errors.
3. Using Diagnostic Tools
There are numerous tools available for diagnosing JVM crashes. Some of the most commonly used are:
- JStack: A command-line tool that prints Java thread stack traces.
- JConsole: A graphical tool for monitoring and managing Java applications.
- VisualVM: Integrates several monitoring, troubleshooting, and profiling tools into one.
Example of Using JStack
- Find the process ID (PID) of the Java application:
jps
- Use JStack to print the stack trace:
jstack <PID>
Why Use JStack?
JStack helps identify what each thread was doing at the time of the crash. It can reveal deadlocks and long-running operations.
Best Practices for Preventing JVM Crashes
While diagnosing crashes is crucial, implementing preventive measures is even more vital. Here are some best practices:
1. Proper Memory Management
Out of Memory errors are among the most common causes of JVM crashes. Configurations such as setting the appropriate heap size via -Xmx
and -Xms
flags is essential.
java -Xms512m -Xmx2048m -cp your-classpath YourMainClass
The Significance of Memory Flags
The -Xms
flag specifies the initial heap size, while -Xmx
sets the maximum heap size. This helps ensure that your application has access to enough memory throughout its execution.
2. Regular Updates
Keeping your Java runtime environment updated minimizes the chances of experiencing bugs that could lead to crashes. Updates often include performance improvements and important security fixes.
3. Use of Profiling Tools
Profiling tools can help detect memory leaks and performance bottlenecks in your application. Tools like Java Flight Recorder provide insights into your Java application's performance and can help you identify potential issues before they lead to crashes.
4. Testing and Load Balancing
Conduct rigorous testing, including stress tests, to ensure your application can perform under high load situations. Use load balancers to distribute incoming traffic across multiple instances of your application to prevent overload.
Closing the Chapter: Navigating the Landscape of JVM Crashes
Decoding JVM crashes involves understanding the layers at which these issues can arise. By monitoring JVM behavior, analyzing logs, and employing best coding practices, you can significantly reduce the likelihood of crashes. While it can be a daunting process, having the right tools and techniques at hand makes diagnosing and resolving JVM crashes manageable.
To learn more about JVM architecture, check out the Oracle Documentation. Additionally, studying memory management and garbage collection can enhance your application’s resilience against JVM crashes, as discussed in this informative guide.
In summary, the JVM is a robust platform, but it requires careful calibration and monitoring. By deploying the strategies outlined in this guide, you can navigate the complexities of JVM crashes and maintain a stable, high-performing Java application.
Checkout our other articles