Troubleshooting Common Issues with Mockito Templates in Eclipse
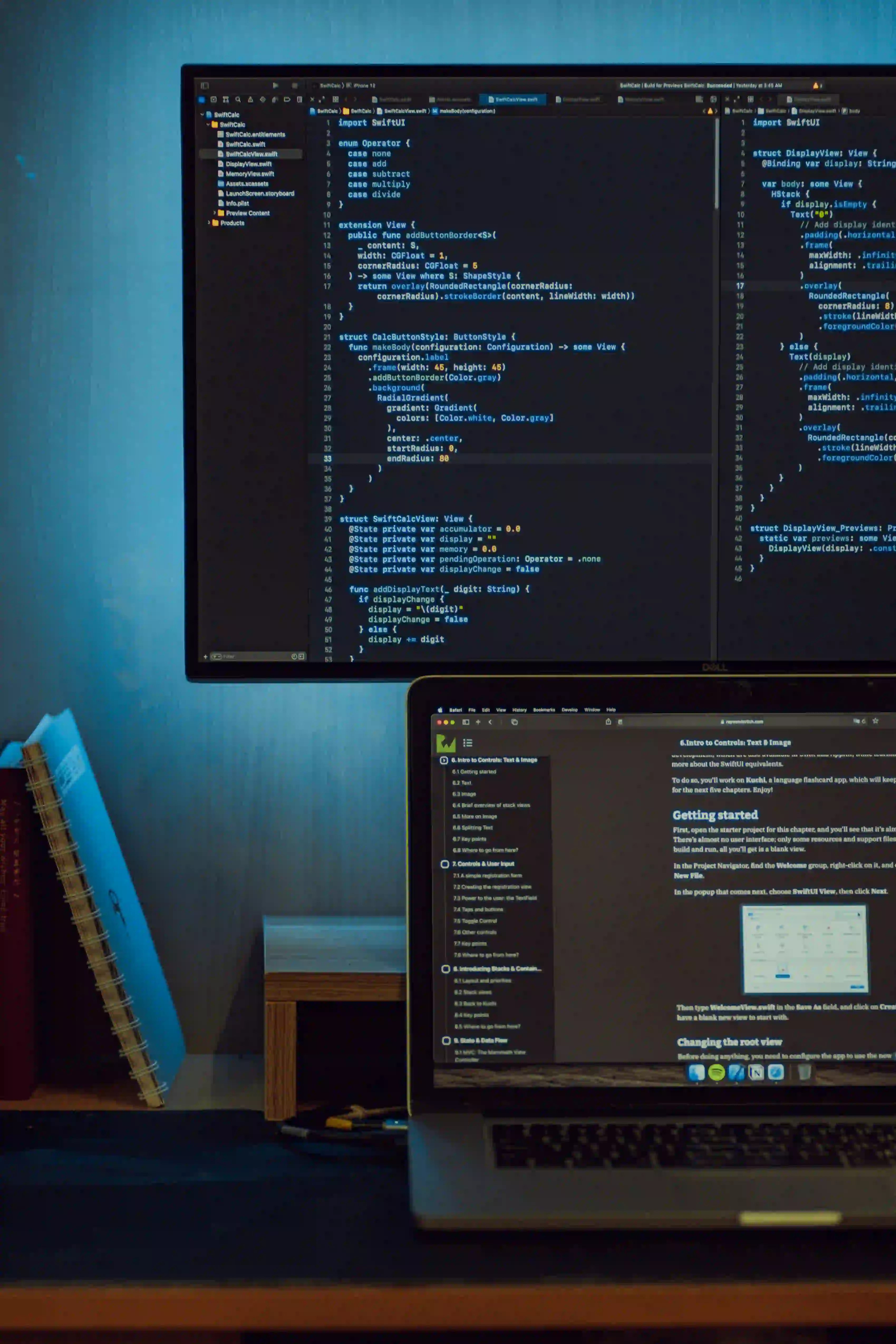
Troubleshooting Common Issues with Mockito Templates in Eclipse
Mockito is one of the most popular mocking frameworks in the Java ecosystem, making it essential for unit testing. However, like any tool, it can present challenges, particularly when working within an IDE like Eclipse. This article explores common issues you might encounter when using Mockito templates in Eclipse and provides troubleshooting steps to overcome them.
Table of Contents
- Setting Up Mockito in Eclipse
- Common Problems with Mockito Templates
- Troubleshooting Steps
- Best Practices for Using Mockito
- Conclusion
Setting Up Mockito in Eclipse
Before troubleshooting, ensure that you have set up Mockito correctly in your Eclipse environment. Follow these steps to configure Mockito in your Java project:
- Create a Java Project in Eclipse.
- Add the Mockito Library:
- Right-click on your project in the Project Explorer.
- Select Build Path > Add Libraries....
- Choose *Maven *and Junit.
- Update Your
pom.xml
(if you are using Maven):📄snippet.txt<dependency> <groupId>org.mockito</groupId> <artifactId>mockito-core</artifactId> <version>4.0.0</version> <!-- Specify the latest version here --> <scope>test</scope> </dependency>
- Refresh the Project to download the necessary dependencies.
Common Problems with Mockito Templates
JUnit and Mockito Integration
One common issue developers face is the integration between JUnit and Mockito. For instance, JUnit 4 annotations must be properly configured:
- Annotations: Use the
@RunWith(MockitoJUnitRunner.class)
annotation for your test class.
import org.junit.runner.RunWith;
import org.mockito.junit.MockitoJUnitRunner;
@RunWith(MockitoJUnitRunner.class)
public class MyServiceTest {
// Test class implementation
}
Importing Mockito Libraries
Ensure that the Mockito libraries are imported correctly. If Mockito classes are not recognized, you may see compilation errors that prevent your tests from running. In this case:
- Check your project's external libraries in the Project Explorer.
- Make sure the Mockito dependency is listed.
Code Completion Issues
Another common issue in Eclipse is the lack of code completion (IntelliSense) for Mockito. If code suggestions do not pop up when entering Mockito methods, try the following:
-
Clean and Build the Project:
- From the menu, choose Project > Clean... and rebuild.
-
Check Project Settings:
- Right-click on the project, go to Properties > Java Build Path and ensure your libraries are listed correctly.
Troubleshooting Steps
If you encounter issues, these steps can help you troubleshoot effectively:
-
Check Compatibility: Make sure that the versions of JUnit and Mockito are compatible. Refer to the JUnit documentation and Mockito documentation for version compatibility.
-
Re-import the Project:
- Sometimes, re-importing the project can resolve underlying issues. Right-click on the project and select Refresh to ensure all dependencies are loaded.
-
Inspect the Console for Errors:
- Keep an eye on the Eclipse Console view for any runtime exceptions or errors related to Mockito. Understanding these errors can often point you directly to the problem.
-
Use Mockito's Inline Mocking:
- If you frequently find yourself needing to mock final classes or static methods, consider using Mockito with the mockito-inline capability. Add it to your
pom.xml
within the dependencies.
📄snippet.txt<dependency> <groupId>org.mockito</groupId> <artifactId>mockito-inline</artifactId> <version>4.0.0</version> <scope>test</scope> </dependency>
- If you frequently find yourself needing to mock final classes or static methods, consider using Mockito with the mockito-inline capability. Add it to your
Exemplary Code Snippet
Here is a simple example demonstrating how to use Mockito in a JUnit test:
import static org.mockito.Mockito.*;
import static org.junit.Assert.*;
import org.junit.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
// Arrange
UserDAO mockUserDao = mock(UserDAO.class);
UserService userService = new UserService(mockUserDao);
User user = new User("John");
when(mockUserDao.getUserById(1)).thenReturn(user);
// Act
User result = userService.getUser(1);
// Assert
assertEquals("John", result.getName());
verify(mockUserDao).getUserById(1); // Verifying the interaction
}
}
Commentary on the Code
-
Mocking: The
mock(UserDAO.class)
creates a mock instance of theUserDAO
. This is crucial for isolating theUserService
during testing. -
Stubbing: The
when(...).thenReturn(...)
construct allows you to specify what should be returned when the specified method is called. -
Assertions: The
assertEquals
method checks the actual output against the expected value. This is a core part of any unit test, ensuring that your test validates a real-world scenario. -
Verification: The
verify(mockUserDao)
method checks that the mock'sgetUserById
method was indeed called during the test execution.
Best Practices for Using Mockito
- Keep Tests Isolated: Ensure that your tests do not depend on external factors. Use mocks to isolate the unit being tested.
- Limit Mock Usage: Avoid excessive mocking. Mocks should be used when necessary, particularly when interfacing with third-party libraries or systems.
- Clear Naming Conventions: Name your mock objects clearly, so it’s easy to identify their purpose in tests.
Key Takeaways
Using Mockito with Eclipse can empower your testing process by ensuring that your code is functioning as intended. Familiarize yourself with common issues and best practices highlighted in this article to minimize frustrations and maximize productivity.
Whether you encounter integration issues, import problems, or code completion hiccups, following the troubleshooting steps provided can help you get back on track quickly. Happy testing!
For more resources, check out the official Mockito documentation and the JUnit Guide.