Choosing the Right Play Framework for Your Next Project
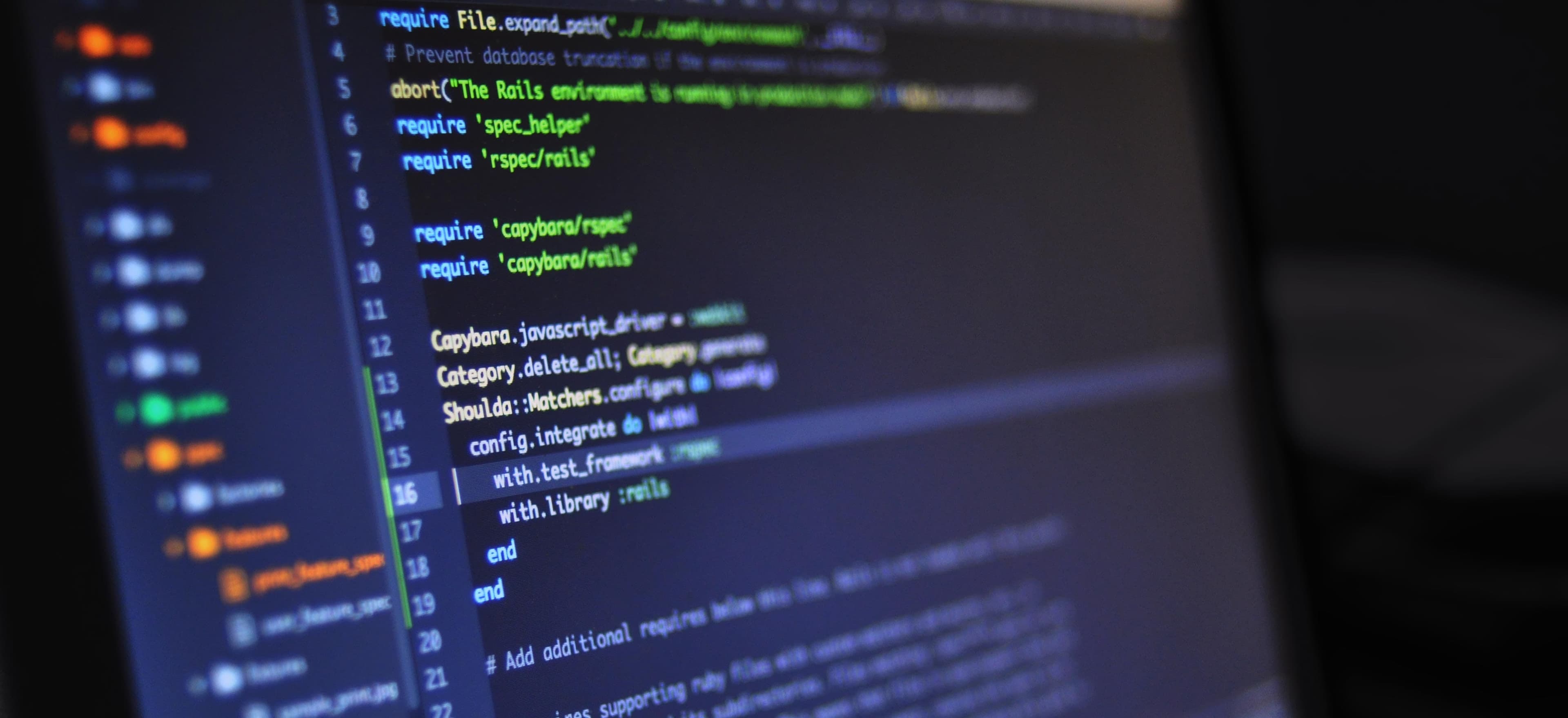
- Published on
Choosing the Right Play Framework for Your Next Project
When embarking on a new web project, the choices of frameworks can be overwhelming. Among these choices, the Play Framework stands out for its robust features, simplicity, and scalability. In this blog post, we'll explore why you might consider the Play Framework, its strengths, and how to decide if it's the right fit for your next project.
What is Play Framework?
The Play Framework is an open-source web application framework that follows the MVC (Model-View-Controller) architectural pattern. It is primarily designed for Java and Scala applications. One of its main objectives is to make development a smooth and enjoyable process with minimal configuration, enabling developers to focus on what matters most: building applications.
Key Features of Play Framework
-
Reactive Architecture: Designed for asynchronous programming, Play allows you to build high-performance applications. This is particularly important in an era where user experience is paramount.
-
Hot Reloading: This feature allows developers to see changes in real-time without restarting the server. It speeds up the development process considerably.
-
Built-in Testing: Play provides testing support out of the box, making it easier to implement unit tests and ensure code quality.
-
RESTful Support: Play promotes a RESTful approach, allowing you to create APIs quickly and efficiently.
-
Support for WebSockets: The ability to handle WebSockets enables real-time web applications.
-
Integration: Play integrates seamlessly with popular front-end frameworks and libraries, allowing developers to utilize the best tools available.
When to Choose Play Framework
While the Play Framework offers numerous advantages, it may not be suitable for every project. Here are key aspects to consider when choosing Play:
1. Application Type
If you are developing a real-time application, such as a chat application or a gaming platform, the reactive principles of Play will be beneficial. Here's a simple example showing how you might set up a controller in Play to handle WebSocket connections:
import play.libs.streams.ActorFlow;
import akka.actor.ActorRef;
import play.mvc.Controller;
import play.mvc.WebSocket;
public class ChatController extends Controller {
public WebSocket chat() {
return WebSocket.sayHello(request ->
ActorFlow.actorRef(out -> ChatActor.props(out))
);
}
}
Why this matters: This code snippet demonstrates how easy it is to set up a WebSocket connection in Play. The integration of Akka actors makes real-time handling straightforward.
2. Team Experience
Is your team familiar with Java or Scala? If so, Play is a natural fit. If you have a diverse team with various experience levels among programming languages, you may want to consider a framework that accommodates a broader skill set.
3. Performance Requirements
If your application needs to handle a large number of concurrent users, Play's non-blocking architecture is advantageous. It's among the best choices for applications that require high throughput and low latency.
4. Scalability
The Play framework succinctly supports cloud applications and microservices. If scaling is a critical element of your project, you will find Play's architecture conducive to seamless growth.
5. Development Cycle
Play’s features, such as hot reloading and built-in testing, can substantially shorten the development cycle. If you aim for rapid iterations and frequent deployments, Play can fit the bill.
Setting Up Your Play Framework Project
Setting up a Play Framework project is typically straightforward. You start by using the command line to create a new project scaffold. Here’s how to do it with the latest Play version.
Step 1: Install Play
You can download the Play Framework from the official site Play Framework Download. Ensure that you have Java JDK 8 or higher installed.
Step 2: Create a New Project
Run the following command:
sbt new playframework/play-java-seed.g8
This command initializes a new Java Play project filled with a basic structure.
Step 3: Running Your Application
Navigate to the new project directory and run:
sbt run
The server typically runs on http://localhost:9000
. You can easily view your project in action.
Code Structure in Play Framework
Understanding the code structure is essential for leveraging Play effectively. Here’s a brief overview of a standard Play Framework project layout:
- app: Contains the main application code, including controllers, models, and views.
- conf: Configuration files, such as
application.conf
androutes
. - public: Static assets like JavaScript, CSS, and images.
- test: This directory contains your test files.
Here's an example of a simple controller in Play:
package controllers;
import play.mvc.Controller;
import play.mvc.Result;
public class HomeController extends Controller {
public Result index() {
return ok("Welcome to the Play Framework!");
}
}
Why this matters: The HomeController
returns a simple "Welcome" message when the index route is hit. Creating controllers in Play is straightforward and follows standard patterns.
Testing in Play Framework
One of the strengths of Play is its built-in support for testing. Play supports both unit and functional testing. Here’s how you can write a simple test:
package test;
import org.junit.Test;
import play.test.WithApplication;
import play.mvc.Http.Response;
import static play.mvc.Http.Status.OK;
public class HomeControllerTest extends WithApplication {
@Test
public void testIndex() {
Response response = route(controllers.routes.HomeController.index());
assertEquals(OK, response.status());
}
}
Why this matters: Testing ensures your code behaves as expected. The above snippet tests if the index
route returns a status of 200 OK
. Automated tests keep your code reliable over time.
Moving Forward with Play
As you venture into the world of the Play Framework, consider checking out Play Framework Documentation for more in-depth knowledge. Experiment with building a small application to get familiar with its structure and functionalities.
When to Look Elsewhere
While Play has its merits, there are instances where alternative frameworks may serve you better. Consider the following situations:
- Simplicity Needs: For smaller, less complex applications, simpler frameworks like Spring Boot may be more appropriate.
- Time Constraints: If you need a quick MVP, you might lean towards frameworks that require less boilerplate.
Final Considerations
Choosing the right framework is crucial for the success of your project. The Play Framework offers a modern, feature-rich environment, particularly suited for applications requiring high performance and scalability.
In summary, evaluate your project requirements against what Play brings to the table. If ready to build high-performance, scalable, and reactive web applications, the Play Framework is a powerful ally.
Explore, experiment, and let your next project not only meet goals but exceed expectations with the Play Framework. Happy coding!
Checkout our other articles