Troubleshooting Common Jersey and Gson Integration Issues
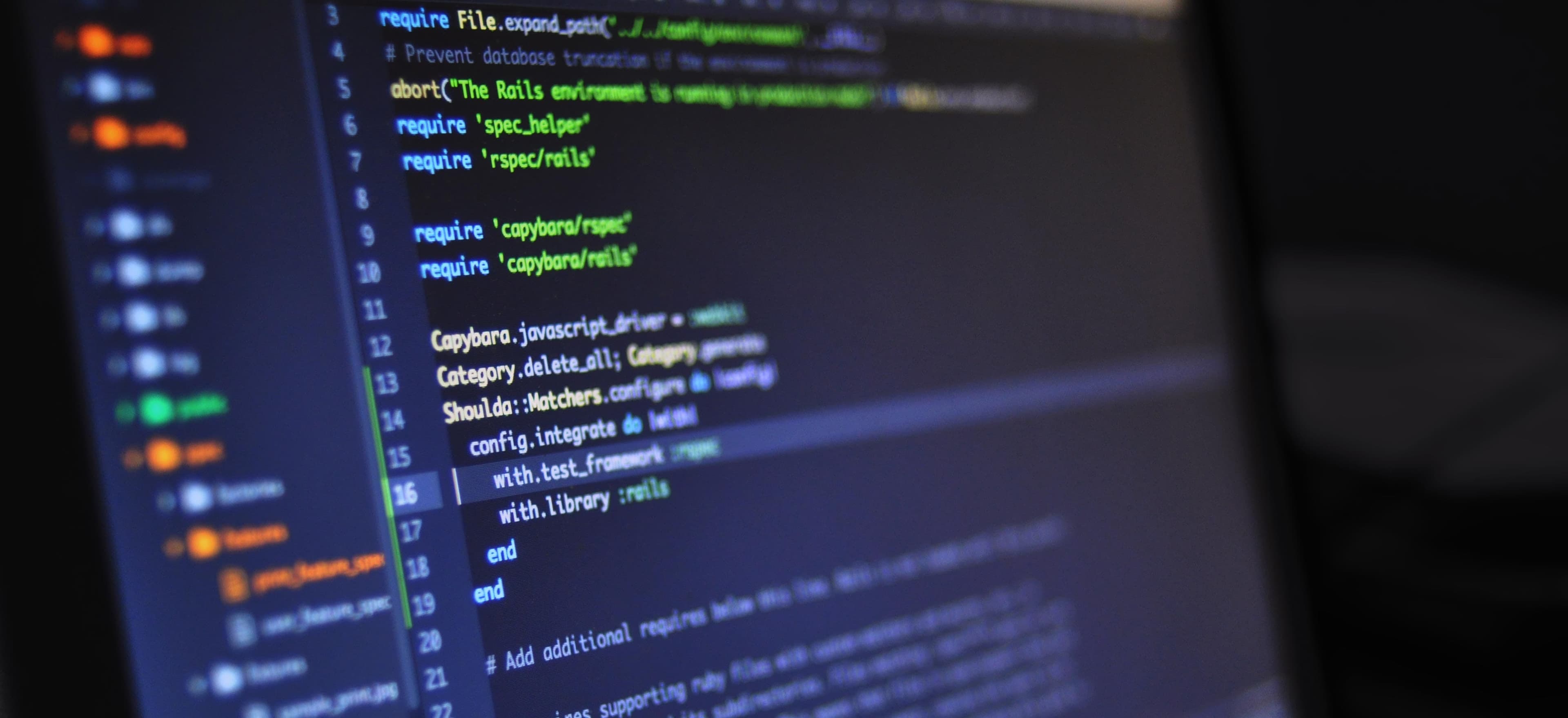
- Published on
Troubleshooting Common Jersey and Gson Integration Issues
Jersey is a popular framework for developing RESTful web services in Java, and Gson is a powerful library for converting Java objects into their JSON representation and vice versa. When using Jersey with Gson for JSON serialization and deserialization, developers may encounter several issues. In this blog post, we will troubleshoot some common integration problems and offer effective solutions.
What is Jersey?
Jersey is the reference implementation of the Jakarta EE (formerly Java EE) JAX-RS (Java API for RESTful Web Services). It provides a simple, effective way to create RESTful web services in Java.
What is Gson?
Gson is a Java library created by Google to convert Java objects into JSON and back. It is well-suited for converting Java objects to JSON strings, making it a popular choice for Java developers dealing with RESTful services.
Common Integration Issues
1. Not Properly Configuring Jersey with Gson
When integrating Jersey with Gson, the first step is to ensure that both frameworks are correctly configured. Jersey does not provide support for Gson out of the box, so you will need to register the Gson provider explicitly.
Solution
To configure Gson in Jersey, you need to register the GsonFeature
. Here is an example of how to do this in your Application
class.
import org.glassfish.jersey.server.ResourceConfig;
import org.glassfish.jersey.jackson.JacksonFeature;
import com.google.gson.Gson;
public class MyApplication extends ResourceConfig {
public MyApplication() {
// Register the Gson feature
register(GsonFeature.class);
}
}
By adding the register(GsonFeature.class);
line in your MyApplication
class, you tell Jersey to use Gson for JSON processing.
2. Data Serialization Issues
Another common issue occurs when the Java objects being serialized do not match the expected JSON format. This can be caused by various factors, such as visibility of fields or use of complex objects.
Solution
Ensure that your POJOs (Plain Old Java Objects) are properly set up for serialization. For example:
public class User {
private String name;
private int age;
public User() {
// No-args constructor for Gson
}
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In the above code, we have a no-args constructor, which is a requirement for Gson to create instances of our class during deserialization. Without it, Gson may throw an exception.
3. Java Collections Not Serializing Correctly
Complex Java structures like collections may not serialize correctly if their generic types are not properly specified. This can lead to issues where the serialized JSON does not reflect the expected structure.
Solution
For collections, ensure you use meaningful generic types in your Java classes. Consider the example below:
import java.util.List;
public class Group {
private List<User> users;
public Group() {
// No-args constructor for Gson
}
public Group(List<User> users) {
this.users = users;
}
public List<User> getUsers() {
return users;
}
}
Make sure the field type List<User>
is declared properly. Gson can now correctly serialize and deserialize it.
4. Circular References
If your model contains circular references, Gson can throw a stack overflow error or convert it into an incorrectly formatted JSON.
Solution
You can resolve this issue by using the @Expose
annotation to limit which fields Gson uses when serializing/deserializing. Here’s an example:
import com.google.gson.annotations.Expose;
public class User {
@Expose
private String name;
@Expose(serialize = false)
private Group group; // Prevent circular reference serialization
// Constructor and getters/setters...
}
In this example, the group
field is excluded from serialization, breaking the circular reference.
5. Handling Null Values
When dealing with null values, Gson serialization may omit those fields, potentially leading to unexpected results.
Solution
To handle null fields appropriately, configure Gson with the following option:
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
Gson gson = new GsonBuilder()
.serializeNulls()
.create();
This will ensure that null values are included in the serialized JSON, making it consistent with your Java object's structure.
6. Version Mismatch
Another often-overlooked issue is mismatched versions of Jersey and Gson. This could result in compatibility issues when integrating both libraries.
Solution
Make sure that you are using compatible versions. You can check the latest versions on their respective repositories:
Update your pom.xml
file in Maven accordingly:
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.x</version> <!-- Use the latest stable version -->
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.x</version> <!-- Use the latest stable version -->
</dependency>
7. Deserialization Error Handling
When deserializing JSON into Java objects, malformed or unexpected JSON structures can lead to exceptions or incorrect data formats.
Solution
To handle deserialization errors, use try-catch
blocks in your code:
import com.google.gson.JsonParseException;
public User deserializeUser(String json) {
try {
return gson.fromJson(json, User.class);
} catch (JsonParseException e) {
// Log error or throw a custom exception
System.err.println("Failed to parse JSON: " + e.getMessage());
return null;
}
}
This way, you can gracefully handle any issues during the deserialization process.
The Last Word
Integrating Jersey and Gson can lead to powerful RESTful web service applications but is not without its challenges. By paying attention to configuration, data structure, and error handling, you can avoid common pitfalls.
If you run into issues, utilize the solutions outlined above to troubleshoot effectively.
For further reading, explore the official Jersey Documentation and the Gson Documentation.
By addressing these integration challenges proactively, you can build robust and maintainable applications that leverage the strengths of both Jersey and Gson.
Now, you've successfully navigated through common Jersey and Gson integration issues. Happy coding!