Solving Spring Logging Dependency Conflicts Made Easy
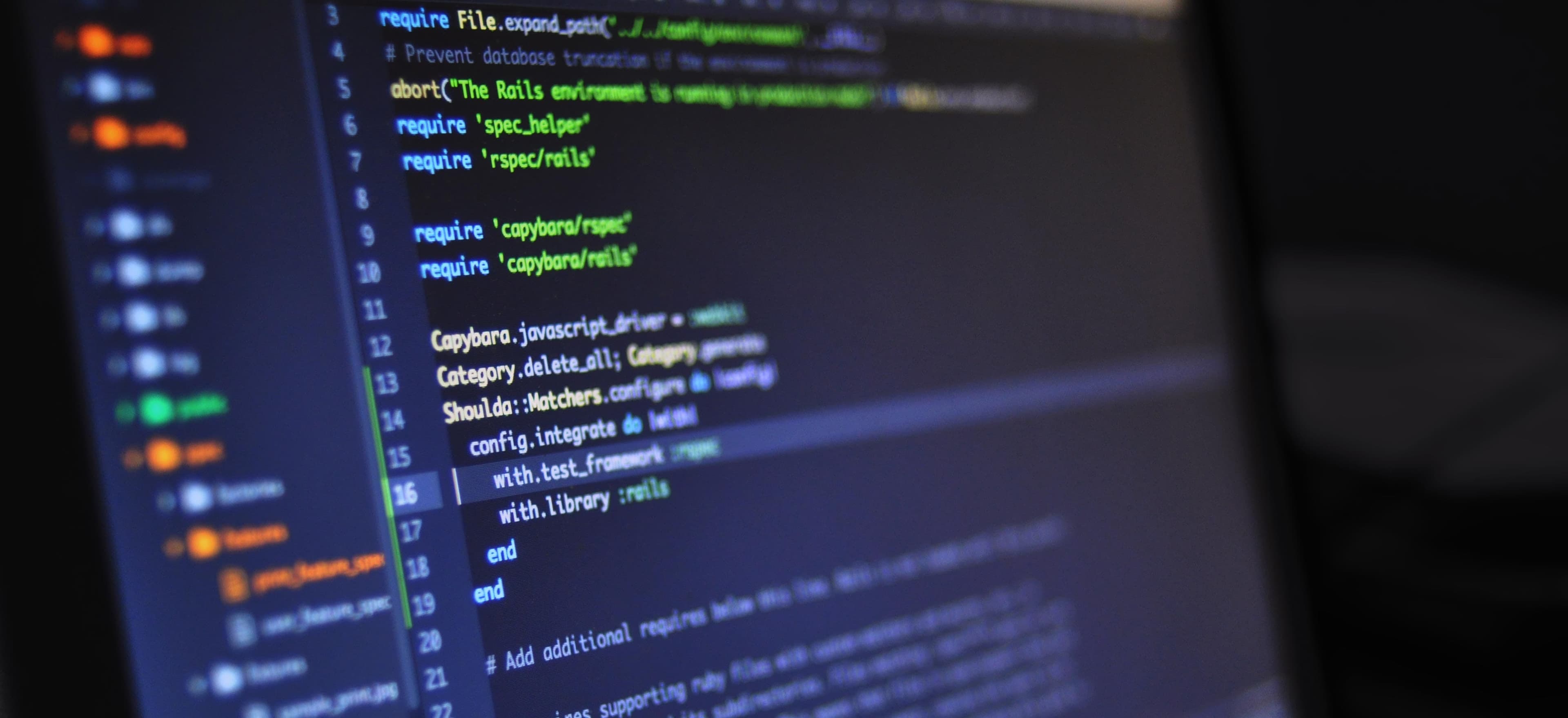
- Published on
Solving Spring Logging Dependency Conflicts Made Easy
Spring Framework is a powerful tool for building Java applications. One of its many features is logging, which plays a crucial role in debugging and monitoring applications. However, developers often encounter dependency conflicts that complicate the logging setup, particularly when using different logging frameworks and libraries.
In this blog post, we will explore how to solve logging dependency conflicts in Spring applications effectively. This guide will cover the necessary tools and methods to identify and manage these conflicts and present code snippets to illustrate the solutions.
Understanding Logging in Spring
Before diving into conflict resolution, let's take a moment to understand how logging works in Spring. Spring uses the SLF4J (Simple Logging Facade for Java) as a logging abstraction layer. This integration allows developers to plug in any logging frameworks, such as Logback, Log4j, or java.util.logging.
While the flexibility of SLF4J is essential, it can sometimes lead to problems, specifically when multiple libraries impose conflicting dependencies. These conflicts manifest in various ways, such as runtime exceptions, log messages not showing up, or being improperly formatted.
Identifying Dependency Conflicts
The first step in solving dependency conflicts is to identify them. The easiest method to view your project's dependency tree in a Maven project is by using the command:
mvn dependency:tree
For Gradle, you can use:
gradle dependencies
By analyzing the dependency tree, you can spot conflicting logging libraries. Common problematic scenarios include having multiple versions of SLF4J or having both Logback and Log4j on the classpath.
Example Dependency Tree Output
[INFO] com.example:myapp:jar:1.0-SNAPSHOT
[INFO] +- org.springframework:spring-context:jar:5.3.10:compile
[INFO] | +- org.slf4j:slf4j-api:jar:1.7.32:compile
[INFO] | +- ch.qos.logback:logback-classic:jar:1.2.6:compile
[INFO] | \- ch.qos.logback:logback-core:jar:1.2.6:compile
[INFO] +- org.apache.logging.log4j:log4j-core:jar:2.14.1:compile
...
In this output, you can observe conflicting logging frameworks: Logback and Log4j.
Resolving Dependency Conflicts
Once you identify the conflicts, you can resolve them systematically. Here are effective strategies you can employ.
1. Exclude Unwanted Dependencies
One straightforward approach to resolve conflicts is to exclude the unwanted libraries from your dependency tree. You can do this in Maven or Gradle.
Maven Example
If you want to use Logback but find Log4j included accidentally, modify your pom.xml
as follows:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.10</version>
<exclusions>
<exclusion>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
</exclusion>
</exclusions>
</dependency>
Gradle Example
For Gradle, you exclude dependencies like this:
implementation('org.springframework:spring-context:5.3.10') {
exclude group: 'org.apache.logging.log4j', module: 'log4j-core'
}
2. Ensure Consistent Versions
Another common source of conflicts arises from using inconsistent versions of the same library. Make sure all dependencies related to logging frameworks use the same version. The Spring Boot Starter includes a curated set of dependencies, which ensures compatibility. If you're using Spring Boot, always prefer the Spring Boot Starter dependencies.
3. Use Spring Boot’s Dependency Management
If you are using Spring Boot, take advantage of its built-in dependency management. By including the Spring Boot Starter, you automatically handle many dependency conflicts. The starter includes the appropriate logging libraries.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
4. Verify Classpath Order
Sometimes, even with the correct dependencies, issues may arise if they aren’t loaded in the right order. Java loads classes in the order they appear in the classpath. To troubleshoot class loading issues, checking the classpath order may yield results.
Practical Example of Implementing Logging
Let's take a look at a practical example of a Spring Boot application that utilizes SLF4J and Logback.
Here’s a simple structure for your application:
Project Structure
my-spring-app
|-- src
| |-- main
| | |-- java
| | | |-- com
| | | | `-- example
| | | | `-- myapp
| | | | `-- MyApplication.java
| | | `-- application.properties
|-- pom.xml
Simple Logging Setup
MyApplication.java
package com.example.myapp;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication implements CommandLineRunner {
private static final Logger logger = LoggerFactory.getLogger(MyApplication.class);
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
logger.info("Application started with args: {}", (Object) args);
}
}
Explanation:
Here, we leverage SLF4J
for logging. The LoggerFactory
creates a logger instance that can be used for various logging levels (info, debug, error). The logging framework allows you to control the output and format via configuration files.
Configuring Logback
You can configure how Logback manages logs by creating a logback.xml
file in the src/main/resources
directory.
logback.xml
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="STDOUT" />
</root>
</configuration>
Explanation:
This configuration specifies that logs should be printed to the console with a timestamp. You can adjust the logging level as needed (DEBUG, WARN, ERROR, etc.) to suit your development or production needs.
My Closing Thoughts on the Matter
Dependency conflicts, particularly in logging frameworks, can hinder the development process. This article has provided you with a structured method to identify and resolve these conflicts in Spring applications.
By utilizing Maven or Gradle effectively, ensuring consistent versions, and leveraging Spring Boot’s built-in features, you can ease the complexities associated with logging dependencies.
For more on managing Spring dependencies effectively, check the Spring Framework Documentation and SLF4J Documentation.
By following these practices, you can keep your logging setup clean, consistent, and efficient, paving the way for smoother development and easier debugging. Happy coding!