Understanding HTTP Content Negotiation: Common Pitfalls
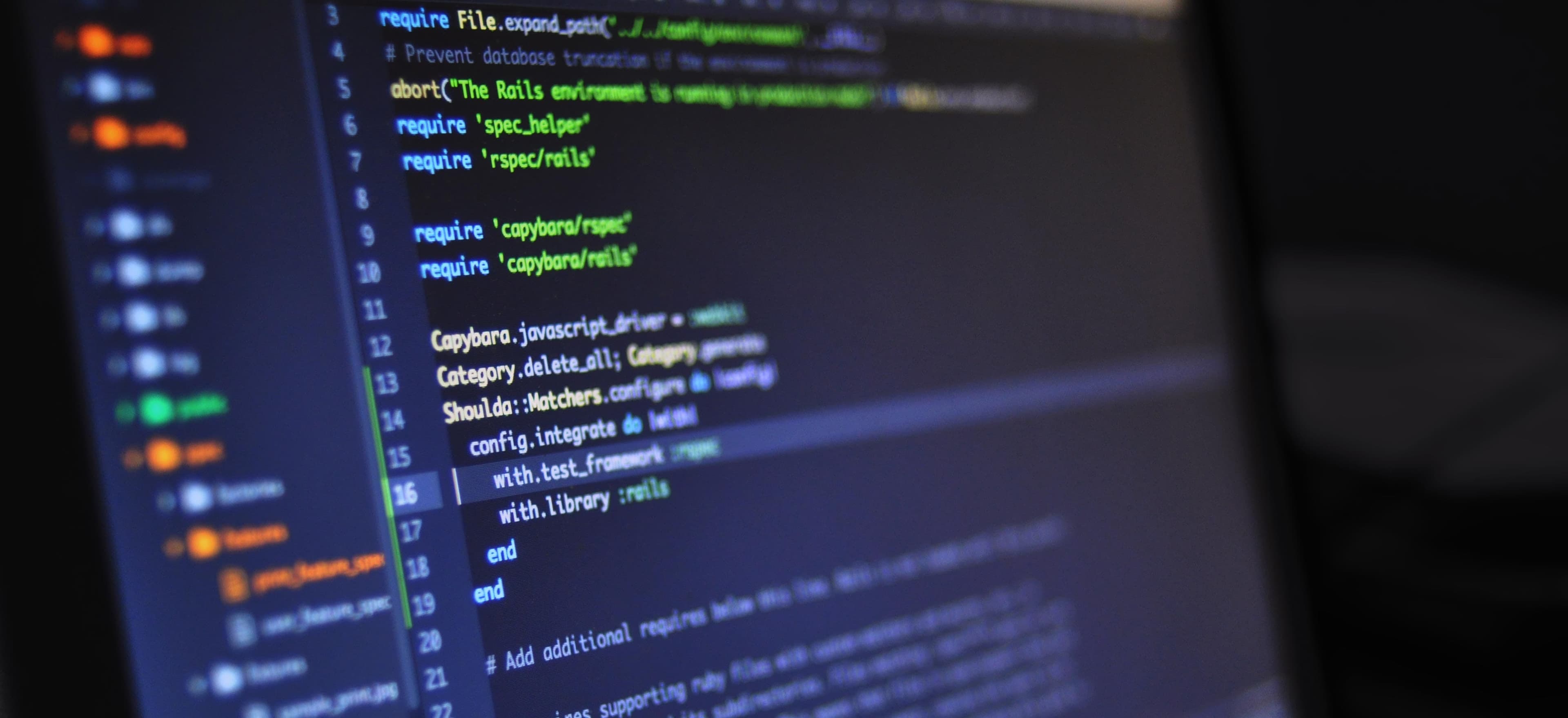
- Published on
Understanding HTTP Content Negotiation: Common Pitfalls
In today's API-driven landscape, HTTP content negotiation plays a crucial role in serving various types of clients and ensuring a seamless user experience. As developers, we must grasp the intricacies of this concept to avoid common pitfalls that can lead to inefficient data delivery or broken interfaces.
In this blog post, we will:
- Define HTTP content negotiation.
- Discuss the different types of content negotiation.
- Highlight common pitfalls in content negotiation.
- Provide practical coding examples in Java to illustrate best practices.
What is HTTP Content Negotiation?
HTTP content negotiation is a mechanism that enables clients and servers to agree on the media type of the response based on the client's request. It allows a single resource to return different representations, such as JSON or XML, depending on what the client can handle.
For instance, a server could provide a resource at a single URI but deliver it as JSON for web clients and XML for mobile applications. This capability is vital for APIs that serve a diverse audience with varying preferences.
Types of Content Negotiation
There are three primary types of content negotiation:
-
Content Negotiation via HTTP Headers:
- Uses the
Accept
,Accept-Language
, orAccept-Encoding
headers to indicate the client's preferences. - For example, a client can communicate it prefers JSON with the header:
Accept: application/json
- Uses the
-
Content Negotiation via URL:
- In this approach, the preferred media type is part of the URL, like:
/api/resource.json /api/resource.xml
- This method often makes it clearer what type of content the client expects.
-
Content Negotiation via Query Parameters:
- Clients can specify their content preferences using URL query parameters:
/api/resource?format=json
Understanding these different methods is crucial, as each has its own advantages and drawbacks.
Common Pitfalls in Content Negotiation
Despite the utility of content negotiation, there are common pitfalls developers should be aware of:
1. Failing to Handle Unsupported Formats
One of the most common mistakes is not adequately handling unsupported formats. If a user-agent requests a type that the server cannot provide, it is essential to respond with an appropriate status code, like 406 Not Acceptable
.
Example in Java
Here's how you might handle unsupported media types in a simple Java controller:
@GetMapping("/resource")
public ResponseEntity<?> getResource(@RequestHeader(value = "Accept") String acceptHeader) {
if (acceptHeader.contains("application/json")) {
return ResponseEntity.ok(new ResourceJson());
} else if (acceptHeader.contains("application/xml")) {
return ResponseEntity.ok(new ResourceXml());
} else {
// Return 406 Not Acceptable
return ResponseEntity.status(HttpStatus.NOT_ACCEPTABLE)
.body("Unsupported media type requested.");
}
}
Why This Matters: This code checks the Accept
header for supported media types and provides a clear response for unsupported formats, improving robustness.
2. Ignoring Default Media Types
Some developers overlook setting a default media type when the client does not specify any preferences. This can lead to confusion and an unpredictable experience for end-users.
Example in Java
You can define a default response type in your controller like so:
@GetMapping("/resource")
public ResponseEntity<?> getResource(@RequestHeader(value = "Accept", defaultValue = "application/json") String acceptHeader) {
if (acceptHeader.contains("application/json")) {
return ResponseEntity.ok(new ResourceJson());
} else if (acceptHeader.contains("application/xml")) {
return ResponseEntity.ok(new ResourceXml());
} else {
return ResponseEntity.status(HttpStatus.NOT_ACCEPTABLE)
.body("Unsupported media type requested.");
}
}
Why This Matters: Setting a default media type ensures a consistent experience, especially for clients that might not explicitly define their preferred format.
3. Not Considering Language Variants
When dealing with international applications, failing to account for language negotiation can also lead to poor user experience. Make sure to handle Accept-Language
headers appropriately.
Example in Java
Here is a simple example of how you might implement language variation handling:
@GetMapping("/greet")
public ResponseEntity<?> greet(@RequestHeader(value = "Accept-Language", defaultValue = "en-US") String language) {
String greeting;
switch (language) {
case "es":
greeting = "Hola!";
break;
case "fr":
greeting = "Bonjour!";
break;
default:
greeting = "Hello!";
}
return ResponseEntity.ok(greeting);
}
Why This Matters: This ensures users receive responses in their preferred language, enhancing the application's usability.
4. Not Implementing Caching Correctly
Improper caching might lead to outdated content being served when media types change. If your application supports content negotiation, make sure to implement HTTP caching strategies appropriately.
Example in Java
To leverage caching, you might use Cache-Control headers:
@GetMapping("/resource")
public ResponseEntity<?> getResource(@RequestHeader(value = "Accept") String acceptHeader) {
CacheControl cacheControl = CacheControl.maxAge(10, TimeUnit.MINUTES);
if (acceptHeader.contains("application/json")) {
return ResponseEntity.ok()
.cacheControl(cacheControl)
.body(new ResourceJson());
} else if (acceptHeader.contains("application/xml")) {
return ResponseEntity.ok()
.cacheControl(cacheControl)
.body(new ResourceXml());
} else {
return ResponseEntity.status(HttpStatus.NOT_ACCEPTABLE)
.body("Unsupported media type requested.");
}
}
Why This Matters: Setting cache control headers can significantly enhance performance by reducing redundant calls and serving frequently requested resources efficiently.
Wrapping Up
Understanding and implementing HTTP content negotiation correctly is vital for developing APIs that provide flexible and adaptable content delivery. Avoiding common pitfalls, such as failing to handle unsupported formats or neglecting language variants, will ensure a smoother experience for your users.
For further reading, consider exploring the official HTTP documentation or Spring's content negotiation guide.
By being mindful of these challenges and employing best practices in your applications, you're on the right path to mastering the art of content negotiation in HTTP. Happy coding!