Why End-of-Line Issues Ruin Your Code and How to Fix Them
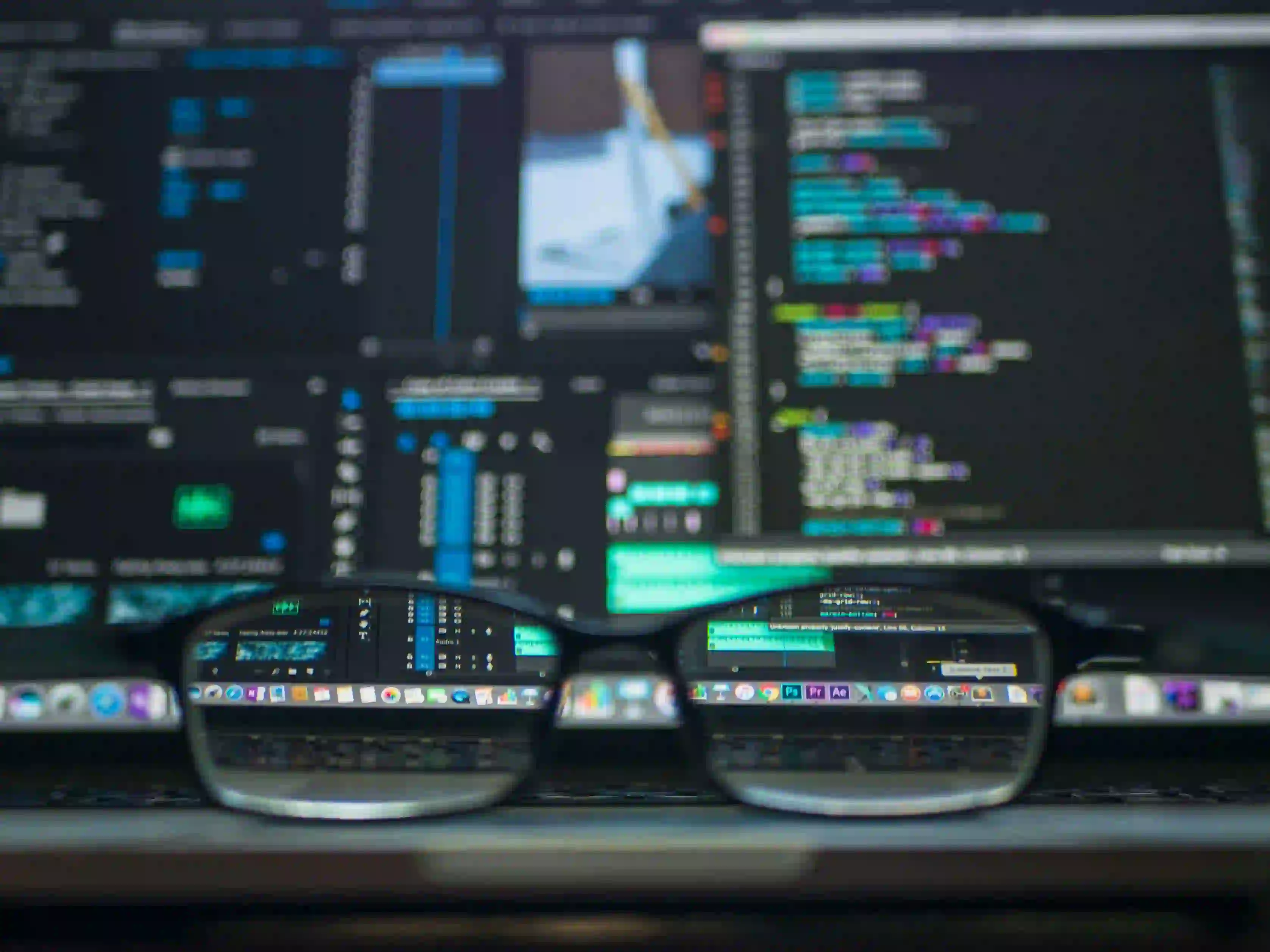
Why End-of-Line Issues Ruin Your Code and How to Fix Them
In the world of programming, even the smallest issues can lead to significant hurdles. One of the lesser-discussed but still prevalent issues is that of end-of-line (EOL) characters. End-of-line problems can lead to unexpected behavior, debugging headaches, and even failures in critical parts of your application. In this post, we will explore why EOL issues can tarnish your code and how to fix them effectively.
Understanding End-of-Line Characters
End-of-line (EOL) characters define the end of a line in a text file. Different operating systems use different characters for this purpose. For instance:
- Windows uses a carriage return followed by a line feed (
\r\n
). - Linux/Unix uses a single line feed (
\n
). - Old Mac systems used a carriage return (
\r
), though this is largely obsolete now.
This inconsistency across platforms can lead to compatibility issues when sharing files between different systems. Let's illustrate with a simple example:
String text = "Hello, World!\r\nThis is a test.";
System.out.println(text);
If you run this code on a Windows system, it will display correctly, with "Hello, World!" and "This is a test." on separate lines. But if you run it on a Linux system without adjusting the EOL characters, it may not render as expected.
The Problems Created by EOL Issues
1. Compatibility Issues
When developers collaborate across different platforms, inconsistencies in EOL characters can lead to significant problems. For instance, if you wrote code in a Windows environment and checked it into a version control system, a Linux user pulling the code might encounter unexpected behavior, failing tests, or worse.
2. Unwanted Characters in Output
If your code isn't designed to handle multiple EOL characters, it can lead to unwanted characters appearing in the output. Suppose you concatenate strings with different EOL characters:
String str1 = "Line 1\r\n"; // Windows EOL
String str2 = "Line 2\n"; // Linux EOL
String result = str1 + str2;
System.out.println(result);
The output may include unexpected line breaks or spacing, making your code difficult to read or debug.
3. Source Control Chaos
Version control systems (VCS) like Git try to manage these differences, but they can still lead to complications. Configurations may be necessary to ensure that EOL characters are consistent across the team's workflow. For example, without proper .gitattributes settings, Git might attribute changes to files just due to EOL differences.
Solutions for EOL Issues
Now that we've discussed the problems, let's explore how to resolve EOL issues effectively.
1. Use a Standard EOL Character
The easiest solution is to agree on a standard EOL character among all team members. For most development environments, adopting UNIX-style line endings (\n
) is often a good compromise since it’s widely supported and is generally considered the industry standard.
2. Configure Your Text Editor/IDE
Most text editors and integrated development environments (IDEs) allow you to configure EOL settings. Make sure to check these settings and standardize your approach. Here’s how you can adjust the EOL settings in some popular editors:
-
Visual Studio Code: You can set the default EOL character by adding the following line to your
settings.json
:📋snippet.json"files.eol": "\n"
-
IntelliJ IDEA: Navigate to File > Settings > Editor > Code Style, then select Line Separator.
3. Set Up .gitattributes
To ensure consistent line endings in Git, you can configure the repository to manage EOL characters automatically by creating or modifying a .gitattributes
file.
Example of .gitattributes
:
* text=auto
This instructs Git to handle line endings automatically based on the platform the files are being checked out to.
4. Convert EOL Characters Programmatically
To handle different line endings in your Java code, you can use utility methods to ensure that all lines have the correct EOL character.
Here’s a simple approach:
public static String normalizeEOL(String input) {
return input.replace("\r\n", "\n").replace("\r", "\n");
}
public static void main(String[] args) {
String mixedEOLText = "Hello, World!\r\nThis is a test.\nEnjoy coding!";
String normalizedText = normalizeEOL(mixedEOLText);
System.out.println(normalizedText);
}
This will replace all occurrences of \r\n
and \r
with \n
.
5. Automated Formatting Tools
Using automated formatting tools like Prettier or EditorConfig can be beneficial. These tools can help maintain consistent EOL characters in your codebase across various contributors.
To use EditorConfig, for example, simply create a .editorconfig
file in your project root:
root = true
[*]
end_of_line = lf
This setting ensures that all files in the project will use LF as their end-of-line character.
To Wrap Things Up
End-of-line issues can seem trivial at first, but they can cause significant headaches in collaborative programming environments and lead to unexpected behavior in your applications. It is crucial to standardize EOL characters among team members, configure development environments accordingly, manage settings in version control systems, and utilize programming utilities to handle EOL normalization in your code.
By being proactive about these issues, you can ensure that your code remains clean, maintainable, and free of EOL-related pitfalls.
For more information on EOL characters and how to manage them, explore the Git Documentation or check out resources on code formatting tools like Prettier.
Happy coding!