Solving Hibernate's Field Value Update Issues During Insert
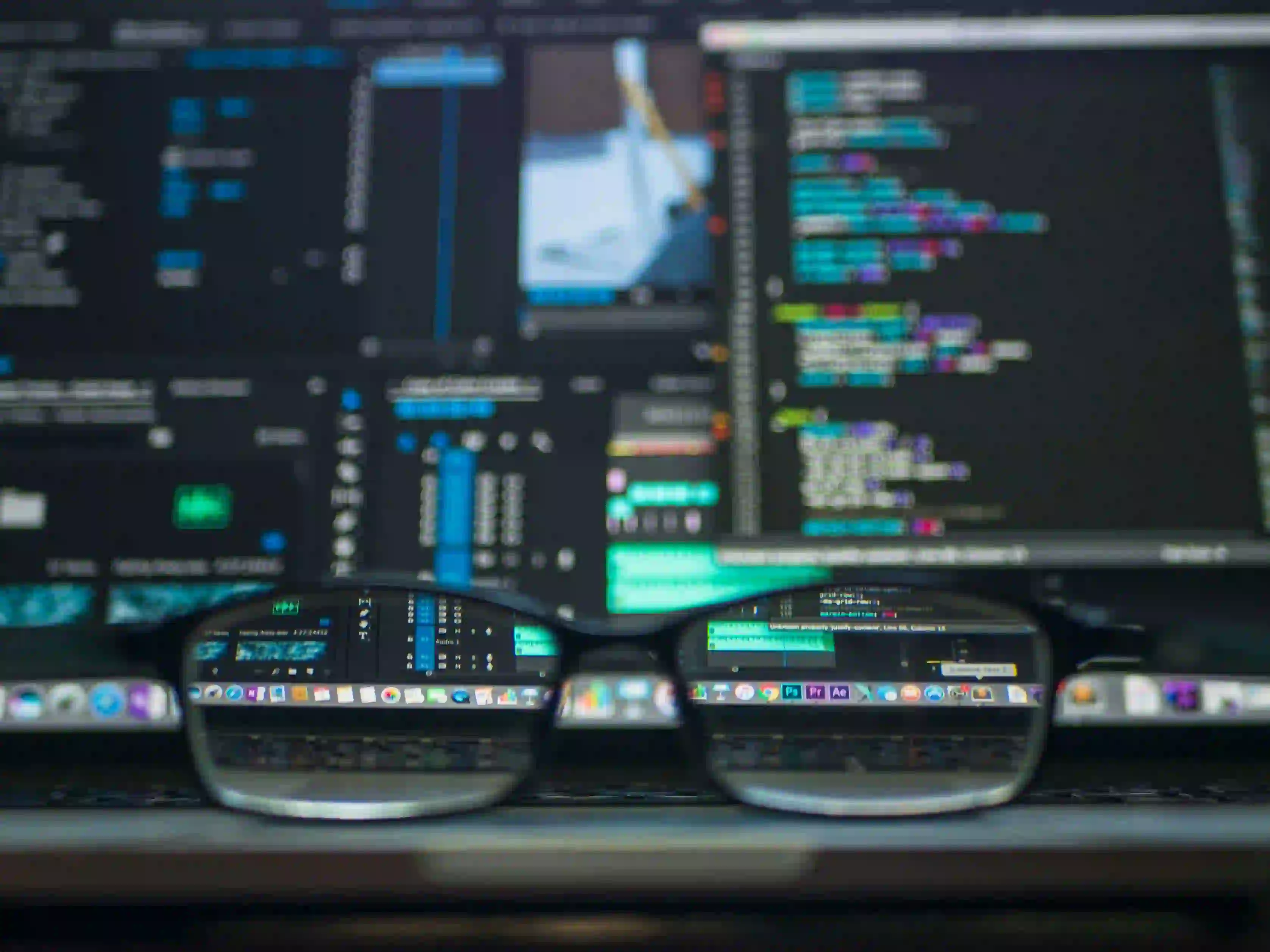
Solving Hibernate's Field Value Update Issues During Insert
Hibernate is a powerful object-relational mapping (ORM) tool that simplifies database interactions in Java applications. However, it’s not without its quirks. One common issue developers encounter is the field value update problem during insert operations. This problem often leads to unexpected behavior and can be particularly troubling when dealing with audit logs or maintaining data integrity.
In this blog post, we’ll dive deep into what causes these issues, how to identify them, and most importantly, ways to solve them effectively.
Understanding Hibernate's Behavior
Before we tackle solutions, it's paramount to understand how Hibernate operates. Hibernate manages the life cycle of entities, and it caches states—pending changes, updates, and deletions.
The Problem Explained
Consider the following scenario: You have an entity class, and you are trying to set certain values in that entity before persisting it. You may find that these values are unexpectedly altered or lost during the save()
or persist()
operations.
For instance, let’s say we have a simple User
class:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and Setters
}
Now, if you try to create a new instance of User
, set its attributes, and then save it, you may encounter issues if you are calling methods that also manipulate the state of the entity after it has been persisted.
User user = new User();
user.setName("Alice");
user.setEmail("alice@example.com");
session.save(user);
// If you manipulate user here, it might update the field values incorrectly.
Identifying the Issue
Common Symptoms
- Values in the database do not match the values set before saving.
- Hibernate throws
TransientObjectException
when trying to save an entity which is in an unexpected state. - Changes reflected in the console during debugging do not match the final outcome after the transaction commits.
Use Logging to Identify State Changes
Using logging can be particularly beneficial in tracking state changes. You can configure Hibernate to show SQL and binding parameters:
# hibernate.cfg.xml or application.properties
hibernate.show_sql=true
hibernate.format_sql=true
hibernate.use_sql_comments=true
Solutions to Field Value Update Issues
1. Use @DynamicInsert
Annotation
One of the most efficient ways to prevent unwanted updates of default values is to use the @DynamicInsert
annotation. This denotes that Hibernate will generate insert SQL dynamically, applying only the fields that have changed.
Here’s how to implement it:
@Entity
@DynamicInsert
public class User {
// Same fields as before
}
With this annotation, if a field's value is null, it won't be included in the SQL insert statement, and Hibernate won’t alter the default value that might be set in your database schema.
2. Entity Lifecycle Callbacks
Utilizing lifecycle callbacks can help manage the state before persisting entities:
@Entity
public class User {
// Fields...
@PrePersist
public void prePersist() {
if (name == null) {
name = "Default Name";
}
// Add any default or calculated attributes here before saving
}
}
With @PrePersist
, you can ensure the entity is in a valid state before being saved.
3. Validate Before Save
Implementing validation logic can prevent attempting to save entities with unexpected values.
public void saveUser(User user) {
if (user.getEmail() == null || !isValidEmail(user.getEmail())) {
throw new IllegalArgumentException("Invalid user email");
}
session.save(user);
}
In the hypothetical isValidEmail()
method, you can add further email validation logic to control the state and integrity of user data.
4. Use Hibernate Interceptors
Hibernate Interceptors allow you to hook into the lifecycle of entity states. By overriding the onSave
method, you can manipulate the entity’s fields before they are persisted.
public class UserInterceptor extends EmptyInterceptor {
@Override
public boolean onSave(Object entity, Serializable id, Object[] state, String[] propertyNames, Type[] types) {
if (entity instanceof User) {
User user = (User) entity;
// Adjust fields before they get persisted
if (user.getName() == null) {
user.setName("Default Name");
}
}
return super.onSave(entity, id, state, propertyNames, types);
}
}
Register the interceptor within your Hibernate configuration.
5. Utilize Versioning for Optimistic Locking
In scenarios where multiple transactions may interfere, implementing optimistic locking with a version field can save you from overwriting concurrent changes.
Add a version field to your entity:
@Entity
public class User {
// Fields...
@Version
private Integer version;
}
With this, Hibernate helps manage concurrency, ensuring that if someone tries to modify a user after another transaction has changed it, an exception will be thrown.
Handling the Transaction
Remember to wrap your session operations in a transaction. Here’s a simple example:
Transaction transaction = null;
try {
transaction = session.beginTransaction();
session.save(user);
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
} finally {
session.close();
}
Ensuring that you commit or rollback as needed can further secure your application against unintended data manipulations.
In Conclusion, Here is What Matters
While Hibernate simplifies database interactions, understanding its internal workings is crucial to successfully handle various behaviors, such as field value update issues during inserts. Using strategies like @DynamicInsert
, lifecycle callbacks, and interceptors will not only help mitigate issues but will also improve the robustness of your data handling.
For more on how Hibernate works, check their official documentation. Additionally, consider joining community forums like Stack Overflow to discuss Hibernate-related queries.
By implementing these best practices and techniques, you'll be much better equipped to handle the nuances of data persistence with Hibernate in your Java applications. Happy coding!