Securing Microservices: Protecting Against Exposed Endpoints
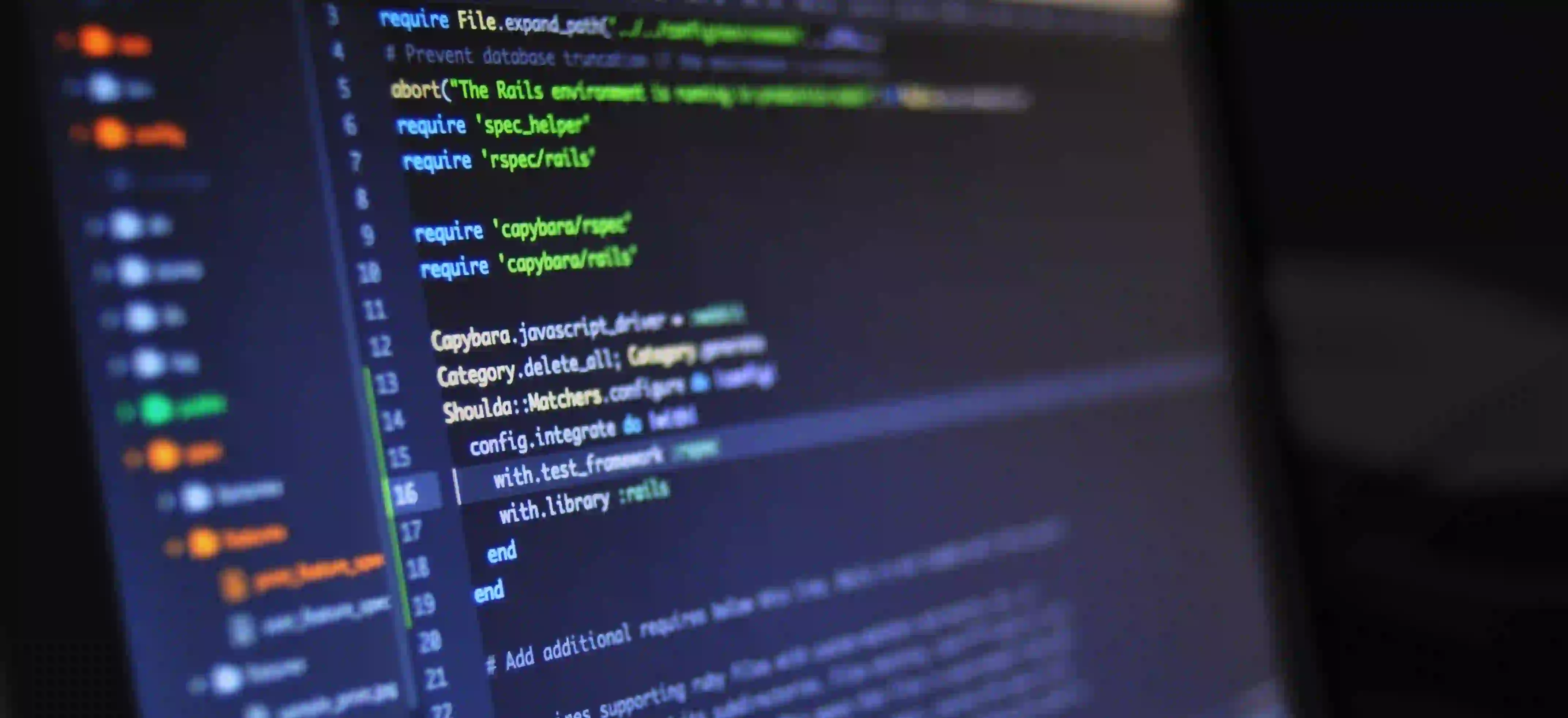
Securing Microservices: Protecting Against Exposed Endpoints
In today's world of software development, the microservices architecture is gaining significant traction. With its advantages such as scalability, flexibility, and resilience, more organizations are adopting microservice patterns. However, this shift also brings along a series of security challenges, particularly the exposure of endpoints which can become prime targets for attackers. In this blog post, we'll discuss how to secure microservices, focusing on protecting against exposed endpoints.
Understanding Microservices Architecture
Microservices are a paradigm that structures software as a collection of loosely coupled services, each handling a specific business functionality. Each service runs independently and communicates through well-defined APIs, often using HTTP or messaging protocols.
Key Benefits of Microservices
- Scalability: Each service can be scaled independently according to its load.
- Flexibility: Development teams can choose different tech stacks according to service requirements.
- Resilience: Failure in one service doesn't necessarily mean the entire system will fail.
However, with these benefits comes the vulnerability of multiple exposed endpoints. Each endpoint is a potential entry point for malicious actors.
Common Threats to Microservices
- Unauthorized Access: Attackers may exploit endpoints to gain unauthorized access to sensitive data.
- Data Breaches: Exposed endpoints can lead to the leakage of personal or proprietary information.
- Denial of Service (DoS): An attacker may flood a service with requests, causing it to become unresponsive.
- Injection Attacks: Endpoints accepting user input are vulnerable to various injection vulnerabilities like SQL injection or command injection.
Why Securing Endpoints is Crucial
Endpoints are the gateways through which clients interact with backend services. If not adequately trained and governed, endpoints can become the weak link in the microservices chain. A compromised endpoint can lead to significant business risks, including financial losses, reputational damage, and regulatory repercussions.
Therefore, it's crucial to implement effective security measures around these endpoints.
Best Practices for Securing Microservice Endpoints
Here are proven strategies and best practices to secure microservices and safeguard exposed endpoints:
1. Implement Authentication and Authorization
Authentication verifies user identities, while authorization governs what authenticated users can access. By implementing robust authentication and authorization strategies, you can significantly minimize the risk of unauthorized access.
Code Snippet - JWT Authentication Example
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
public class JwtUtil {
private String secret = "secretkey"; // Secret key for signing tokens
private long validity = 3600000; // Token validity
public String generateToken(String username) {
Map<String, Object> claims = new HashMap<>();
return Jwts.builder()
.setClaims(claims)
.setSubject(username)
.setIssuedAt(new Date(System.currentTimeMillis()))
.setExpiration(new Date(System.currentTimeMillis() + validity))
.signWith(SignatureAlgorithm.HS256, secret)
.compact();
}
public boolean validateToken(String token, String username) {
final String extractedUsername = extractUsername(token);
return (extractedUsername.equals(username) && !isTokenExpired(token));
}
public String extractUsername(String token) {
return extractAllClaims(token).getSubject();
}
private Claims extractAllClaims(String token) {
return Jwts.parser().setSigningKey(secret).parseClaimsJws(token).getBody();
}
private boolean isTokenExpired(String token) {
return extractAllClaims(token).getExpiration().before(new Date());
}
}
In this example, we use JWT (JSON Web Tokens) for authentication. The generateToken
method creates a signed token using a secret key, which can then be sent to the client.
2. Use API Gateway for Centralized Security
An API gateway functions as a single entry point to your microservices, enabling centralized security, traffic management, and API integration. The gateway can enforce authentication, rate limiting, and logging.
Benefits of API Gateway:
- It can help in throttling requests to protect against DoS attacks.
- It provides a location for implementing secure data transfer protocols such as TLS.
3. Employ Rate Limiting
By limiting the number of API requests a client can make in a certain period, you can protect your services from abuse. Rate limiting acts as a guard against DoS attacks where repetitive requests are sent to exhaust resources.
@RestController
@RequestMapping("/api")
public class ExampleController {
private static final int MAX_REQUESTS = 100;
private static final long TIME_WINDOW = 60000; // 1 minute
private Map<String, RateLimiter> ratelimiters = new ConcurrentHashMap<>();
@GetMapping("/data")
public ResponseEntity<String> getData(@RequestHeader("X-Client-ID") String clientId) {
ratelimiters.putIfAbsent(clientId, RateLimiter.create(MAX_REQUESTS / (TIME_WINDOW / 1000.0)));
if (ratelimiters.get(clientId).tryAcquire()) {
return ResponseEntity.ok("Here is your data!");
} else {
return ResponseEntity.status(HttpStatus.TOO_MANY_REQUESTS).body("Rate limit exceeded.");
}
}
}
In this code, we utilize a rate limiter that allows only a specified number of requests for each client within a defined time window.
4. Validate Input and Sanitize Data
Always validate input from clients to prevent injection attacks. Only accept expected data types and formats. This holds true not only for user input but also for any parameters that can be exploited.
5. Secure Your Communication
Using HTTPS instead of HTTP is a must. SSL/TLS encrypts the data in transit, ensuring that sensitive information is not intercepted during transmission. Whether communications happen between microservices or between clients and services, encryption is key.
6. Monitor and Log API Activity
Monitoring activity can provide insights into potential security issues. By logging access patterns and behaviors, you can quickly identify suspicious activities.
- Use tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus for monitoring service metrics and logs.
My Closing Thoughts on the Matter
As organizations shift towards microservices architectures, securing exposed endpoints has never been more critical. By implementing thorough authentication, API gateways, rate limiting, input validation, encrypted communications, and vigilant monitoring, developers can significantly reduce the surface for potential attacks.
Securing microservices is not a one-time effort but an ongoing process involving constant scrutiny, updates, and optimizations. For further reading on the best practices for API security, refer to OWASP’s API Security Top 10 and Spring Security documentation for comprehensive guidelines.
By adopting these practices, you can help protect your microservices from a wide array of security threats and provide a robust foundation for your digital services.