Avoiding Scientific Notation in Java Decimal Formatting
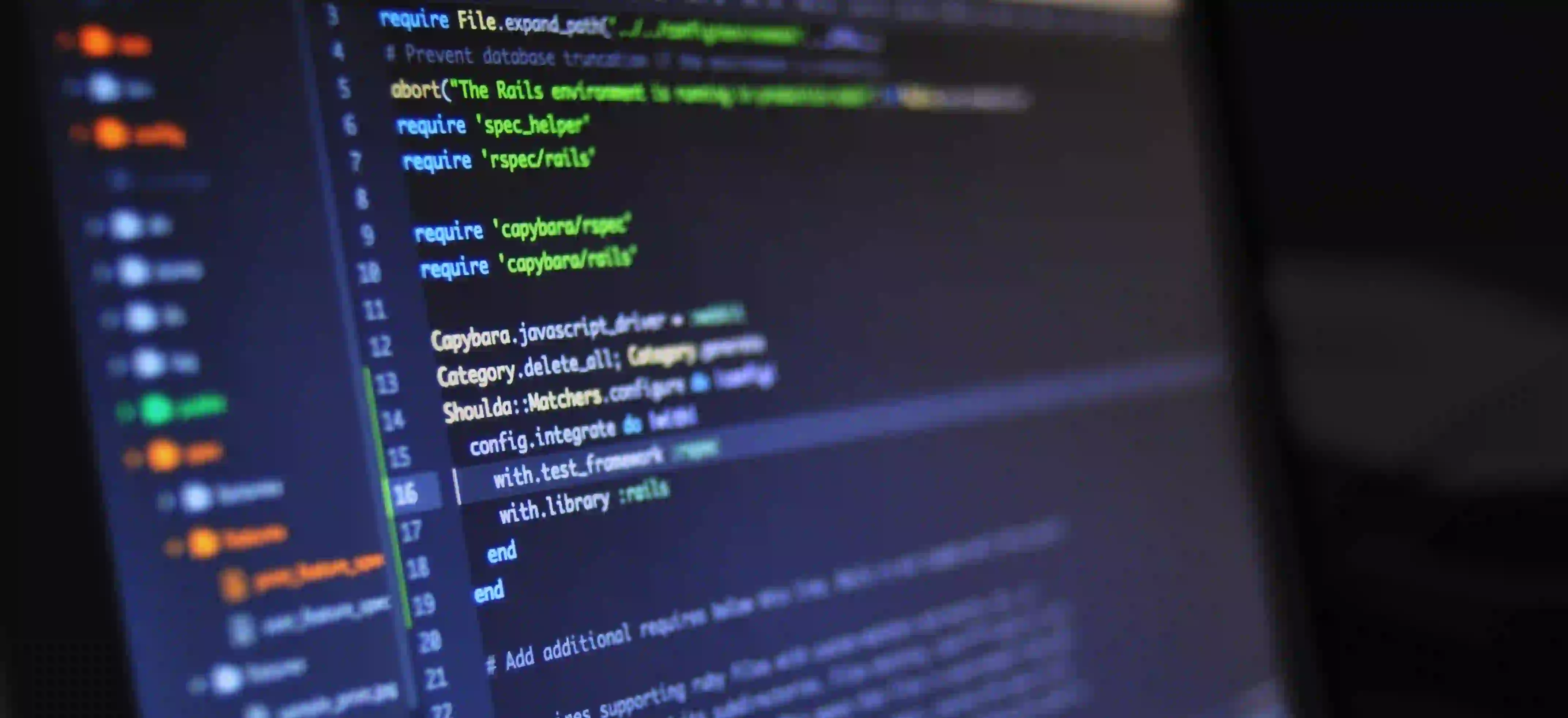
Avoiding Scientific Notation in Java Decimal Formatting
In the world of programming, we often deal with numerical data. When presenting this data, particularly in reports or user interfaces, the formatting can significantly impact readability. One common issue that arises is the use of scientific notation when displaying decimal numbers, especially large or small values. In this blog post, we will explore how to avoid scientific notation in Java decimal formatting, ensuring that your numerical outputs are clear, concise, and user-friendly.
Understanding Scientific Notation
Scientific notation is a shorthand way of representing very large or very small numbers. For example:
- 0.000123 can be represented as 1.23E-4
- 1230000 can be represented as 1.23E6
While scientific notation is useful in certain fields, it can confuse general audiences or users who are not familiar with it. Avoiding scientific notation can lead to improved comprehension of numerical data.
Why Use Decimal Formatting?
Using proper decimal formatting not only enhances readability but also ensures that the values are presented in a contextually appropriate manner. For instance, financial applications generally require two decimal places to accurately represent currency, while scientific applications might prioritize precision over formatting.
Core Java Decimal Formatting Techniques
Java provides various ways to format decimals, mainly through the DecimalFormat
class and the NumberFormat
class. In this section, we will focus on utilizing these tools.
1. Using DecimalFormat
DecimalFormat
is a concrete subclass of NumberFormat
that formats decimal numbers. Here is how you can use it in your Java application:
import java.text.DecimalFormat;
public class DecimalFormattingExample {
public static void main(String[] args) {
double number = 1234567890.987654;
// Create a DecimalFormat instance with desired pattern
DecimalFormat decimalFormat = new DecimalFormat("#,###.00");
// Format the decimal number
String formattedNumber = decimalFormat.format(number);
// Output the formatted number
System.out.println("Formatted Number: " + formattedNumber);
}
}
Why Use This Code?
- The
#
character in the format pattern allows optional digits, while0
means to always show a digit. - The
,
character adds a grouping separator (thousands separator). - The
.00
ensures that the decimal part always displays two digits.
When you run this code, the output will be:
Formatted Number: 1,234,567,890.99
This formatting effectively avoids scientific notation and presents the number in a user's preferred style.
2. Using NumberFormat
If you want to format numbers based on the locale (which can be useful for international applications), you can use NumberFormat
:
import java.text.NumberFormat;
import java.util.Locale;
public class LocaleDecimalFormattingExample {
public static void main(String[] args) {
double number = 1234567890.987654;
// Create a NumberFormat instance based on locale (e.g., US)
NumberFormat numberFormat = NumberFormat.getNumberInstance(Locale.US);
numberFormat.setMaximumFractionDigits(2);
numberFormat.setMinimumFractionDigits(2);
// Format the decimal number
String formattedNumber = numberFormat.format(number);
// Output the formatted number
System.out.println("Formatted Number: " + formattedNumber);
}
}
Why Use This Code?
NumberFormat.getNumberInstance()
allows you to adopt formatting preferences for specific regions.- You can customize the number of maximum and minimum fraction digits, ensuring you maintain control over the output.
When executed, this will produce a similarly formatted output:
Formatted Number: 1,234,567,890.99
3. Avoiding Scientific Notation with BigDecimal
When dealing with very large or small decimal numbers, you might use BigDecimal
, which is designed to handle arbitrary precision. Hereβs a demonstration:
import java.math.BigDecimal;
import java.text.DecimalFormat;
public class BigDecimalFormattingExample {
public static void main(String[] args) {
BigDecimal bigDecimalNumber = new BigDecimal("0.000000123456789");
// Create DecimalFormat instance
DecimalFormat decimalFormat = new DecimalFormat("#.##########");
// Format BigDecimal
String formattedNumber = decimalFormat.format(bigDecimalNumber);
// Output the formatted number
System.out.println("Formatted Number: " + formattedNumber);
}
}
Why Use This Code?
BigDecimal
allows for high precision in numerical data.- The formatting with
#
ensures that unnecessary zeroes are not added, yielding a clean, non-scientific notation output.
The output in this case will be:
Formatted Number: 0.0000001235
Practical Applications
Avoiding scientific notation is especially crucial in the following areas:
- Financial applications: Where precision is vital, and users are accustomed to numeric forms without scientific formatting.
- User interfaces: Ensuring all displayed data is understandable, particularly to non-technical users.
- Reporting tools: Clear reports increase user trust and clarity, making data easily consumable.
For further reading, you may explore the official Java documentation on NumberFormat and DecimalFormat.
The Last Word
In summary, avoiding scientific notation in Java decimal formatting can streamline the presentation of numerical data, enhancing its accessibility and comprehension. Using classes such as DecimalFormat
and NumberFormat
, and leveraging BigDecimal
for high-precision values, will help you achieve clear and professional number displays.
Whether you're developing a financial application or creating a user-friendly interface, implementing these techniques can make a significant difference in how end-users interpret your data. Happy coding!