Securing Your Data: Common Cryptography Pitfalls in Shiro
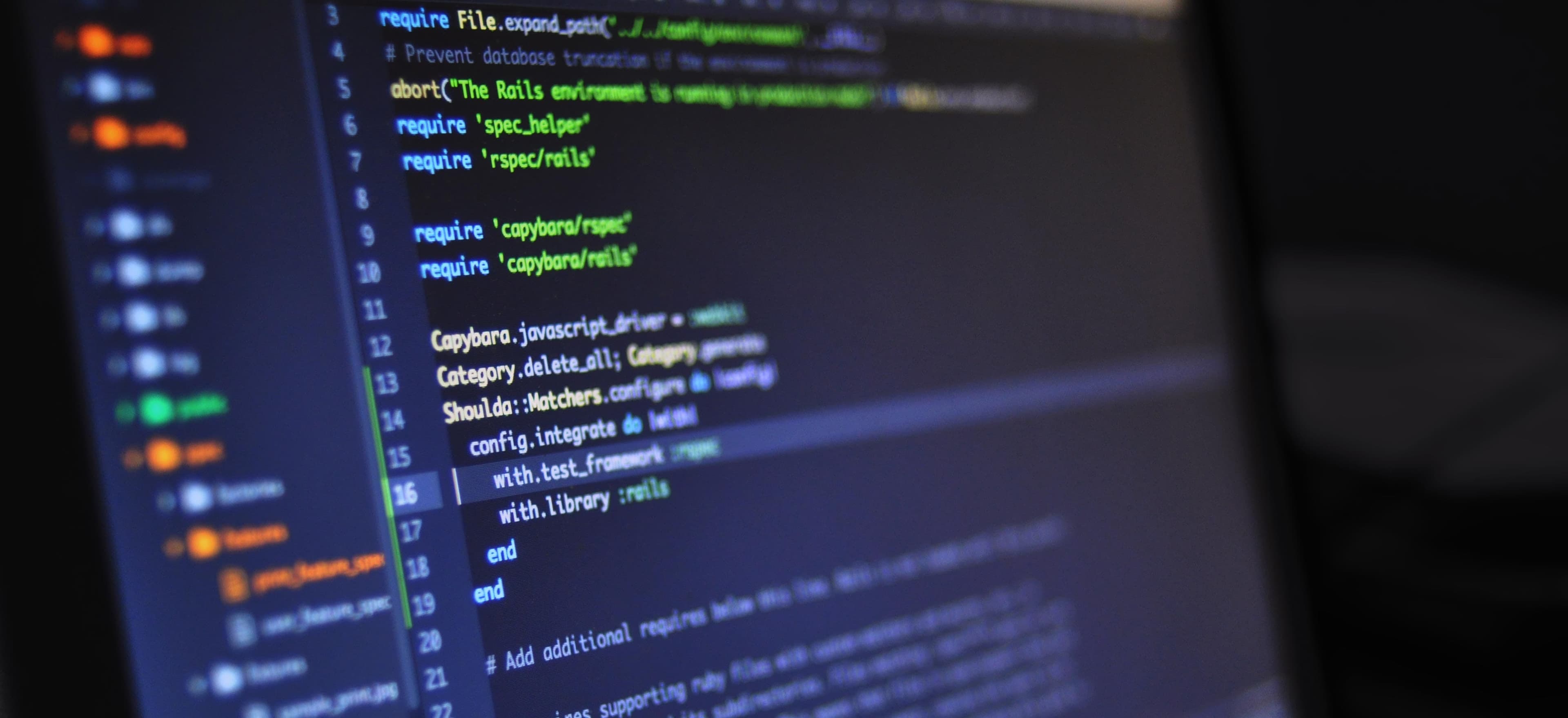
- Published on
Securing Your Data: Common Cryptography Pitfalls in Shiro
Apache Shiro is a powerful framework that allows developers to implement robust security measures in their Java applications. Its flexible architecture makes it a popular choice for handling authentication, authorization, session management, and cryptography. However, even seasoned developers can fall into traps when implementing cryptography within Shiro. In this article, we will explore some common pitfalls and how to avoid them, ensuring your application remains secure.
Understanding Shiro’s Cryptography Features
Before we delve into the pitfalls, it’s essential to review the cryptography features Shiro offers. Shiro provides built-in support for hashing, encryption, and decryption. This means you can securely store passwords, encrypt sensitive data, and manage user sessions effectively.
The primary components of Shiro’s cryptography features include:
- Hashing algorithms: For secure password storage.
- Encryption algorithms: For encrypting data and ensuring confidentiality.
By leveraging these components, you can enhance your application's security posture. However, incorrect usage can lead to significant vulnerabilities.
Common Cryptography Pitfalls
1. Weak Hashing Algorithms
One of the most significant mistakes developers make is selecting a weak hashing algorithm for password storage. Shiro provides several hashing methods as part of its HashService
.
For example, many applications still use SHA-256 for hashing passwords. While SHA-256 is a cryptographically secure hash function, it is not designed for password storage. Attackers can use techniques like rainbow tables or brute-force attacks to reverse-engineer stored hashes quickly.
Instead, you should utilize stronger hashing functions designed specifically for passwords, such as bcrypt, PBKDF2, or Argon2.
Code Example: Using bcrypt with Shiro
import org.apache.shiro.crypto.hash.BCrypt;
import org.apache.shiro.crypto.hash.SimpleHash;
public class PasswordUtil {
public static String hashPassword(String password) {
// Generate a random salt. This should be stored alongside the hashed password.
String salt = BCrypt.gensalt();
// Hash the password using bcrypt with a generated salt.
return BCrypt.hashpw(password, salt);
}
public static boolean verifyPassword(String password, String hashedPassword) {
// Compare the plain password with the hashed password.
return BCrypt.checkpw(password, hashedPassword);
}
}
Why Use bcrypt?: Bcrypt automatically manages the hashing iterations, making it computationally expensive for attackers to perform dictionary and brute-force attacks.
2. Hardcoding Secrets
Another pitfall often encountered in applications is hardcoding cryptographic keys and secrets directly in the code. Not only does this practice expose your secrets in the source code, but it also makes changing those secrets tedious.
Best Practice: External Configuration
Instead of hardcoding sensitive information into the application code, store keys and secrets in external configuration files or environmental variables.
import org.apache.shiro.codec.Base64;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public class EncryptionUtil {
private static final String KEY = System.getenv("APP_SECRET_KEY"); // Retrieve API secret key from environment variable
public static String encrypt(String data) throws Exception {
SecretKeySpec keySpec = new SecretKeySpec(KEY.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, keySpec);
byte[] encrypted = cipher.doFinal(data.getBytes());
return Base64.encodeToString(encrypted);
}
public static String decrypt(String encryptedData) throws Exception {
SecretKeySpec keySpec = new SecretKeySpec(KEY.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, keySpec);
byte[] decrypted = cipher.doFinal(Base64.decode(encryptedData));
return new String(decrypted);
}
}
Why Avoid Hardcoding?: If attackers gain access to your source code repository, they will have immediate access to your secrets. Using environment variables or configurations adds a layer of protection.
3. Not Using Salting
When implementing hashing for passwords, developers sometimes overlook the importance of salting. A salt is a random value added to the password before hashing. This ensures that even if two users have the same password, their hashes will differ, preventing attackers from using precomputed hashes.
Correct Hashing with Salt
By using salts, you increase the complexity for attackers.
public static String hashPasswordWithSalt(String password, String salt) {
// Combine password and salt before hashing
String saltedPassword = password + salt;
// Use a strong hashing algorithm, e.g., SHA-256
return new SimpleHash("SHA-256", saltedPassword).toHex();
}
Why Is Salting Important?: Without salting, identical passwords can yield identical hashes, making your application vulnerable to attacks using precomputed hash tables.
4. Insecure Random Number Generation
If your application relies on cryptographic operations, you need to ensure that random numbers used in cryptography, such as for generating salts or keys, are generated securely. Java’s Math.random()
is not suitable for cryptographic purposes.
Instead, use Java’s SecureRandom
.
Example of Secure Random Generation
import java.security.SecureRandom;
public class SecureRandomUtil {
private static final SecureRandom secureRandom = new SecureRandom();
public static byte[] generateSecureRandomBytes(int numBytes) {
byte[] bytes = new byte[numBytes];
secureRandom.nextBytes(bytes);
return bytes;
}
}
Why Use SecureRandom?:
SecureRandom
uses a secure algorithm to generate random numbers, ensuring a higher level of unpredictability in cryptographic applications.
5. Lack of Regular Updates
Lastly, many developers do not keep their libraries up-to-date, including Shiro itself. Security vulnerabilities are regularly reported and patched, and not upgrading can leave your application exposed to well-known attacks.
Best Practice: Regularly check for updates and release notes for your dependencies. Tools like Dependabot can automate this process.
The Last Word
Cryptography is an essential part of developing secure Java applications with Apache Shiro. However, developers must remain vigilant about common pitfalls. By using strong hashing algorithms, avoiding hardcoding secrets, using proper salting techniques, generating secure random numbers, and ensuring libraries are regularly updated, you can significantly increase the security of your application.
For more information on how to implement Shiro effectively, visit the Apache Shiro documentation. Remember, when it comes to security, attention to detail is crucial. Every little mistake can lead to vulnerabilities in your system.
Ensuring robust cryptography practices not only protects your data but also reinforces trust in your application among users. Stay informed and proactive about security, and your Java applications will withstand potential threats more effectively.
By implementing the strategies outlined above and avoiding common pitfalls, you can develop applications that not only utilize Shiro for effective security but also stand resilient against evolving security threats.
Checkout our other articles