Mastering Java Servlets: Common Questions Unpacked
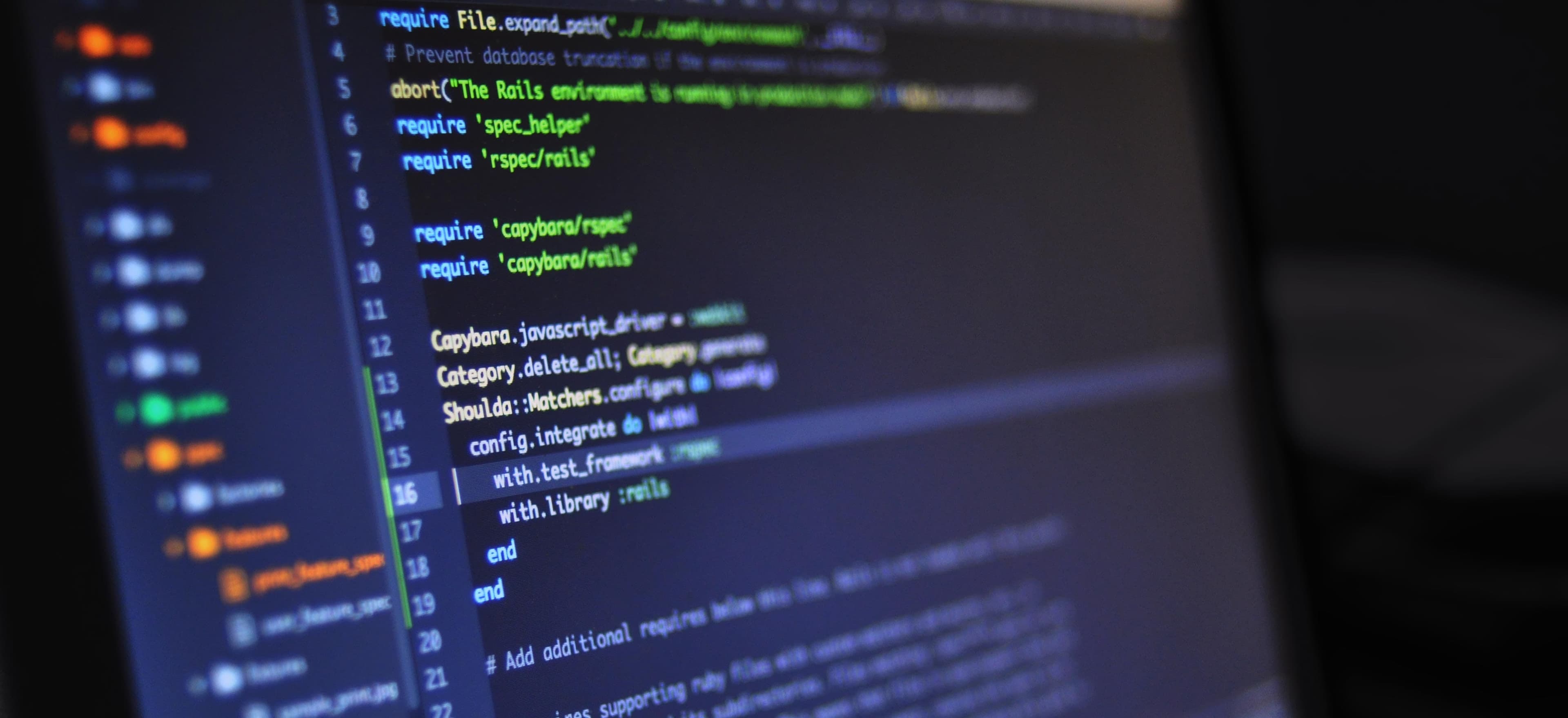
- Published on
Mastering Java Servlets: Common Questions Unpacked
Java Servlets form an integral part of web application development, laying the groundwork for dynamic web content generation. Whether you are a newcomer seeking to understand the basics, or an experienced developer needing a refresher, this guide aims to help you master the key concepts of Java Servlets.
We’ll unpack some of the most commonly asked questions, delve into examples, and provide insights that will enhance your understanding of Servlets and their role in Java-based web frameworks.
What is a Java Servlet?
A Java Servlet is a server-side Java program that handles client requests and generates dynamic responses. It operates within a servlet container, acting as an intermediary between the client and the server.
Basic Servlet Lifecycle
Understanding the Servlet lifecycle is crucial. The lifecycle can be broken down into four main phases:
- Loading and Instantiation: When the server starts, it loads the servlet class and creates an instance.
- Initialization: The
init()
method is invoked, where you can set up resources or configuration. - Request Handling: The
service()
method is called for each request, where the servlet processes requests and responses. - Destruction: The
destroy()
method is called when the servlet is taken out of service, allowing for cleanup.
Example Code: Simple Servlet
Here’s a basic example of a Servlet that returns a simple "Hello, World!" message:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.PrintWriter;
public class HelloWorldServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<h1>Hello, World!</h1>");
}
}
Why Use Servlets?
- Efficiency: Servlets execute in a server-side environment which can handle many requests simultaneously.
- Portability: Being Java-based, servlets can run on any platform that supports Java.
- Integration: Servlets seamlessly interact with other Java technologies like JSP (JavaServer Pages).
What are the Differences Between Servlets and JSP?
While both Servlets and JSP serve a similar purpose, they cater to different scenarios.
-
Servlets: They are Java code which can be more complex and verbose for HTML generation. They are primarily used for controlling application logic.
-
JSP: Offers a simpler syntax for the presentation layer. HTML intermingled with Java code is much cleaner and preferred for dynamic web content.
Example Code: Simple JSP
Here’s a simple JSP page that outputs "Hello, World!":
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Hello JSP</title>
</head>
<body>
<h1>Hello, World!</h1>
</body>
</html>
When to Use Each?
Use Servlets when you need to handle complex business logic and JSP for the presentation layer. They often work together in a web application.
How do Servlets Handle Requests?
Servlets handle requests through overridden doGet()
or doPost()
methods. These methods are designed to respond to HTTP GET and POST requests respectively.
Example of Handling POST Request
Here is a simple example of a Servlet that processes user input via POST request:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.PrintWriter;
public class UserServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String userName = request.getParameter("username");
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<h1>Hello, " + userName + "!</h1>");
}
}
Why Understand Request Handling?
Understanding request handling is crucial for building interactive web applications. By effectively managing requests, you can create applications that respond to user inputs dynamically.
What are Request and Response Objects?
The HttpServletRequest
and HttpServletResponse
objects play a vital role in Servlets by providing necessary details about the request made by the client and the response that the server sends back.
Request Object
The request object encapsulates all incoming client data, allowing you to retrieve parameters, headers, and session information.
Response Object
The response object is used to control how the server responds to a client, including status codes, headers, and response body content.
Example: Using Request and Response
Here’s a snippet demonstrating how to use these objects:
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String name = request.getParameter("name");
response.setContentType("text/plain");
response.getWriter().write("Welcome, " + name);
}
How to manage Session in Servlets?
Session management is a crucial part of web application development. Servlets can track user sessions using the HttpSession
object.
Example of Session Management
The following snippet shows how to create and retrieve session attributes:
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
HttpSession session = request.getSession();
session.setAttribute("username", "JohnDoe");
String user = (String) session.getAttribute("username");
response.getWriter().write("Logged in as: " + user);
}
Why Track Sessions?
Session tracking (via cookies or URL rewriting) allows you to maintain user state across multiple requests, enhancing user experience by keeping data consistent.
Are Java Servlets Multithreaded?
Yes! Servlets are inherently multithreaded, meaning multiple threads can invoke the service method simultaneously. This makes servlets highly efficient in handling multiple requests.
Thread Safety Considerations
While servlets can handle multiple requests, be cautious about shared resources. Always ensure that shared data is appropriately synchronized to avoid race conditions.
A Final Look
Mastering Java Servlets is vital for anyone in web development using Java-based frameworks. By understanding the servlet lifecycle, request and response handling, and session management, you can build powerful web applications.
Further Reading
- Oracle Servlet Documentation
- JavaServer Pages (JSP) Guide
As your journey into Java Servlets continues, keep experimenting and building. The foundational knowledge you've gained here will serve you well as you create more complex and robust web applications. Happy coding!
Checkout our other articles