Troubleshooting TLS Errors in Java Mail Sending
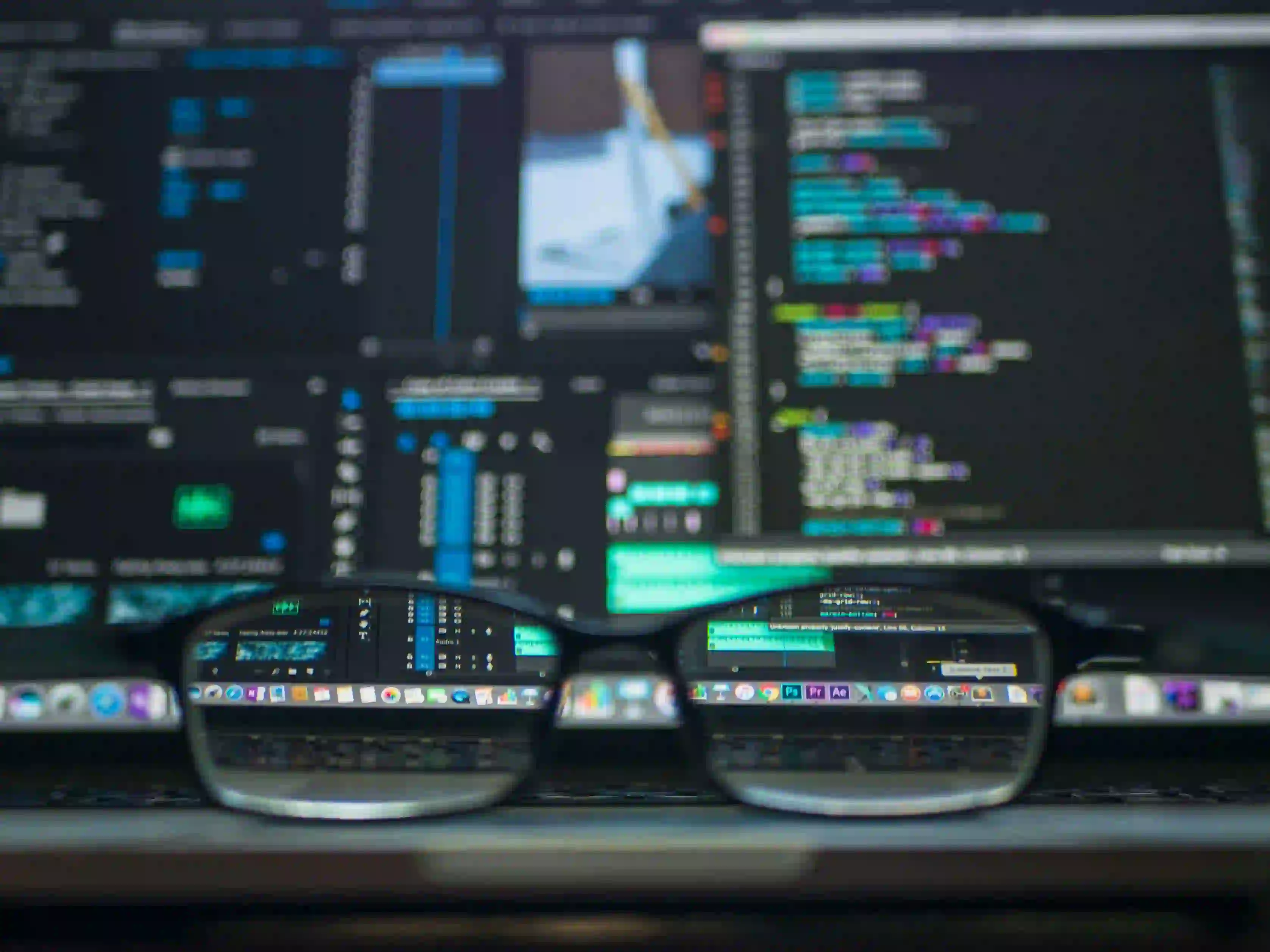
Troubleshooting TLS Errors in Java Mail Sending
Transport Layer Security (TLS) is a critical element for secure email communication, especially when using JavaMail to send emails over SMTP. TLS errors can be frustrating, leading to failed deliveries and hindering your applications. In this article, we will dive into common TLS errors you might encounter while using Java Mail, how to troubleshoot them, and best practices to follow when implementing secure email delivery in your applications.
What is TLS?
Before we delve into troubleshooting, let’s quickly define TLS. TLS is a cryptographic protocol designed to secure communications over a computer network. In the context of email, it ensures that your email and its contents are transmitted securely between the email client and the server.
Common TLS Errors in JavaMail
When sending emails via JavaMail, you may face several TLS-related errors. Here are some frequent culprits:
-
javax.mail.NoSuchProviderException: This typically indicates that JavaMail is unable to find the specified mail provider, often due to misconfigured TLS settings.
-
javax.mail.AuthenticationFailedException: An indication that authentication failed, which can be due to an incorrect username/password or a missing TLS connection.
-
javax.mail.MessagingException: Could not connect to SMTP host: This error may likely arise from TLS settings or a firewall blocking the connection.
To alleviate these errors, a proper understanding of your JavaMail setup is crucial.
Setting Up JavaMail with TLS Support
To effectively use TLS for sending emails, ensure that your JavaMail configuration is correctly set up. Here’s a sample Java code snippet illustrating how to configure JavaMail with TLS:
import java.util.Properties;
import javax.mail.*;
import javax.mail.internet.*;
public class EmailSender {
public static void main(String[] args) {
// Set up properties for the mail session
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.example.com"); // Your SMTP server
props.put("mail.smtp.port", "587"); // TLS port
// Create a session with an authenticator
Session session = Session.getInstance(props, new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your_email@example.com", "your_password");
}
});
try {
// Create a MimeMessage
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("from@example.com"));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse("to@example.com"));
message.setSubject("Test Email");
message.setText("Hello, this is a test email!");
// Send the message
Transport.send(message);
System.out.println("Email Sent Successfully");
} catch (MessagingException e) {
// Handle any exceptions
e.printStackTrace();
}
}
}
Why This Configuration?
-
TLS parameters:
- Setting
mail.smtp.starttls.enable
totrue
allows the connection to automatically switch to a secure TLS connection. - Specifying the SMTP port (587) is essential since it is commonly used for TLS connections in many email services.
- Setting
-
Authentication:
- An authenticator is created to handle both username and password, ensuring that your credentials are utilized securely.
Testing Your Setup
Once you have configured the above code, try running the application. If it executes without errors, congratulations! You have successfully set up your JavaMail with TLS support. If you still face issues, examine the error output carefully.
Common Issues and Resolutions
1. Certificate Verification Failure
A common TLS error when sending emails is related to certificate problems. If JavaMail fails to verify the SMTP server's certificate, you might see:
javax.mail.MessagingException: Could not connect to SMTP host: smtp.example.com, port: 587
Resolution:
- Ensure that your Java environment recognizes the certificate of the SMTP server.
- Import the server certificate into your Java keystore using the following command:
keytool -import -alias smtp-cert -file your_cert_file.crt -keystore cacerts -storepass changeit
2. Old TLS Version
Sometimes, the TLS version used by JavaMail may not be accepted by the server due to security policies. Modern email servers require TLS 1.2 or TLS 1.3.
Resolution:
- Ensure that your Java version supports TLS 1.2 or 1.3. You can check the version with:
java -version
If necessary, consider upgrading to a more recent JDK.
3. Firewall Issues
A firewall may block your connection attempts, leading to an inability to send emails.
Resolution:
- Check firewall rules and ensure TCP port 587 (or the port you are using) is open for outgoing connections.
Best Practices for Secure Email Sending in Java
-
Secure Your Credentials: Never hard-code your email credentials in the code. Instead, consider using environment variables or a secure vault.
-
Stay Updated: Use the latest version of Java and JavaMail to benefit from security and functionality improvements.
-
Test in a Safe Environment: Before deploying email functionality in production, thoroughly test email sending in a controlled environment.
A Final Look
Troubleshooting TLS errors in JavaMail can be daunting, but understanding configurations and common pitfalls can ease the process. By following the guidance provided in this article, you will be better prepared to handle TLS-related challenges in your Java email applications.
For further reading, check out JavaMail API Documentation for insights into more advanced features and capabilities.
With the right setup and best practices, you can ensure that your email functionalities are reliable, secure, and ultimately successful. Happy coding!