Mastering Pagination in Spring Data JPA: Common Pitfalls
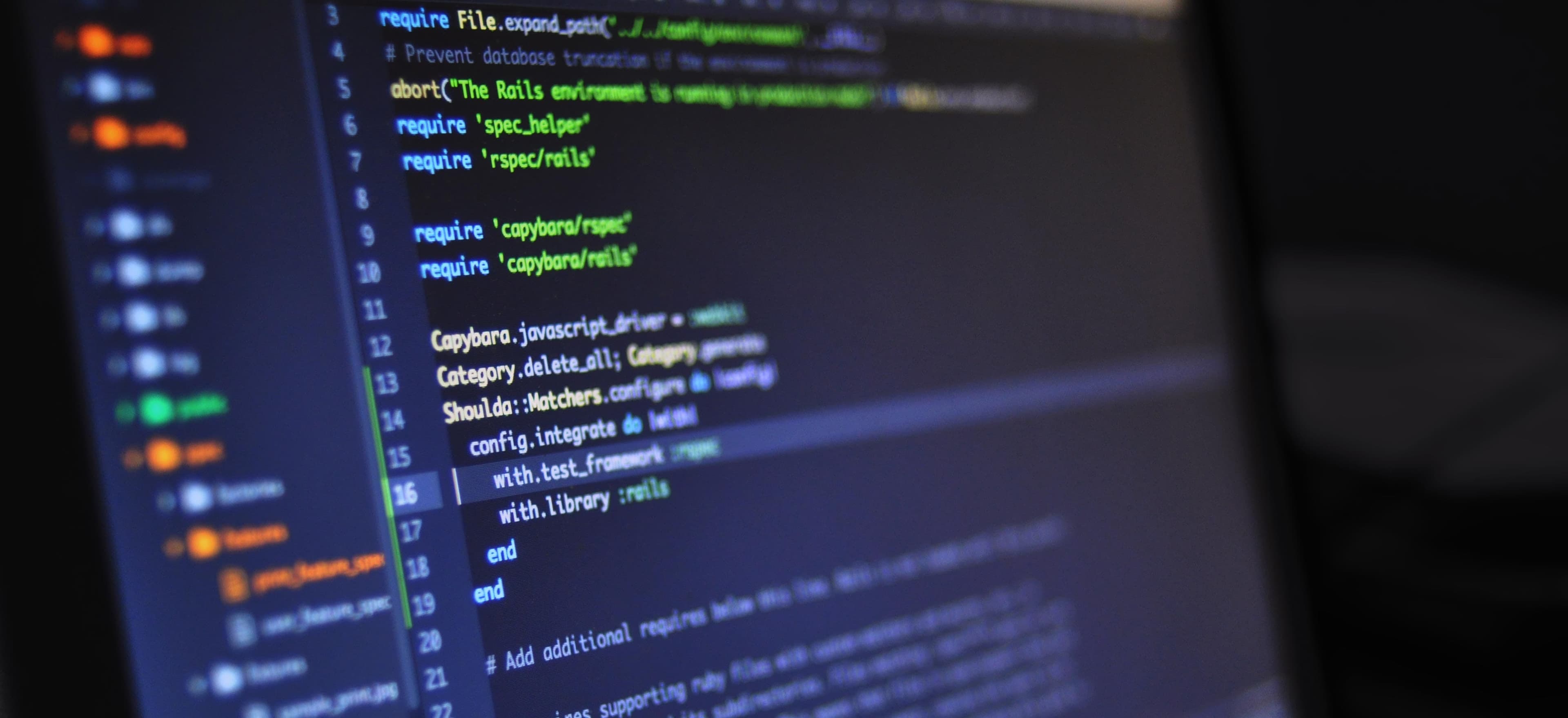
- Published on
Mastering Pagination in Spring Data JPA: Common Pitfalls
In the world of web applications, the need for efficient data retrieval cannot be overstated. As your dataset grows, the necessity for paginated results becomes apparent. Pagination helps in managing large amounts of data efficiently, enhancing performance, and improving user experience. In the context of Spring Data JPA, mastering pagination is essential, but it’s also fraught with potential pitfalls.
This blog post will guide you through common pitfalls associated with pagination in Spring Data JPA, providing examples and best practices along the way.
Table of Contents
- What is Pagination?
- Setting up Spring Data JPA
- Implementing Pagination
- Common Pitfalls
- Ignoring Offsets
- Fetching Too Much Data
- Performance Issues with Large Datasets
- Conclusion
What is Pagination?
Pagination refers to dividing data into discrete pages, allowing users to navigate through data without being overwhelmed. Instead of loading thousands of records, pagination loads a limited number of records at a time, making data easier to read and interact with.
With Spring Data JPA, implementing pagination is straightforward, allowing developers to retrieve subsets of data simply and efficiently.
Setting up Spring Data JPA
Before diving into pagination, let’s ensure that we have the necessary dependencies. You will need to include the following dependencies in your pom.xml
file if you are using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Ensure that your Spring Boot application is configured to use JPA and a database of your choice, e.g., H2 for simplicity.
Example Entity
Here’s a quick definition of a simple entity:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and Setters
}
User Repository
Next, let's define a repository interface to interact with our User
entity:
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
}
Implementing Pagination
With our entity and repository in place, we can look at how to implement pagination. Spring Data JPA makes this seamless through the Pageable
interface.
Example of Pagination
Here’s how you can retrieve a paginated list of users:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.web.PageableDefault;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/users")
public Page<User> getUsers(@PageableDefault(size = 5) Pageable pageable) {
return userRepository.findAll(pageable);
}
}
Explanation
- We use
@PageableDefault(size = 5)
to specify that each page should contain 5 users. - The repository method
findAll(pageable)
efficiently handles the pagination.
Make sure to test your endpoint by calling GET /users?page=0&size=5
to view the first page of users.
Common Pitfalls
While using pagination with Spring Data JPA is straightforward, several pitfalls can arise. Understanding these issues will help you avoid common mistakes.
Ignoring Offsets
One of the frequent mistakes developers make is neglecting that pagination involves offsets. The page starts at zero. If you request page one with size five, it will fetch records 0-4.
Incorrect Usage Example:
@GetMapping("/users/incorrect")
public Page<User> getIncorrectUsers(@RequestParam int page) {
return userRepository.findAll(PageRequest.of(page, 5));
}
If you access this endpoint with page=1
, it will fetch users from record 0-4 instead of 5-9. Always remember that pagination starts from zero.
Fetching Too Much Data
It can be tempting to increase the page size to reduce the number of requests. However, fetching too much data at once can lead to performance issues.
Example of Fetching Too Much Data:
@GetMapping("/users/largePage")
public Page<User> getLargePageUsers(@RequestParam int page) {
return userRepository.findAll(PageRequest.of(page, 100));
}
Here, if page size is set to 100, and the dataset is large, it could lead to memory overflow, degrading performance. Instead, stick to manageable sizes, like 5 or 10.
Performance Issues with Large Datasets
When operating on large datasets, naïve pagination techniques can perform poorly. Problems may stem from inefficient querying, especially if you’re using JOINs or have complex SQL queries.
Consider using Keyset Pagination instead of Offset pagination in complex queries. With keyset pagination, you retrieve records based on a “bookmark” (the last record of the previous page), which can significantly boost performance.
Example of Keyset Pagination:
This approach prevents the database from having to count the offset every time.
@GetMapping("/users/keyset")
public List<User> getUsersAfter(@RequestParam Long lastId) {
return userRepository.findUsersAfterId(lastId);
}
In your repository, create a custom method:
@Query("SELECT u FROM User u WHERE u.id > :lastId ORDER BY u.id")
List<User> findUsersAfterId(@Param("lastId") Long lastId);
By implementing keyset pagination, you will notice increased performance, especially for large datasets.
Final Considerations
Pagination is vital for efficient data management in large applications. By understanding its implementation and potential pitfalls, you can significantly enhance the performance and user experience of your Spring Data JPA application.
Key Takeaways:
- Always account for offsets when using pagination.
- Be cautious about fetching too much data at once.
- For large datasets, consider using advanced techniques like keyset pagination.
For further reading, consider visiting Spring Data JPA Reference Documentation or explore JPA Pagination Best Practices to deepen your understanding.
Happy coding, and remember to paginate wisely!
Checkout our other articles